TypeScript
Enum Type
enum Direction {
Up,
Down,
Left,
Right,
}
const up: Direction = Direction.Up;
const leftOrRight: Direction.Left | Direction.Right = Direction.Left;
enum Direction {
Up = "UP",
Down = "DOWN",
Left = "LEFT",
Right = "RIGHT",
}
enum BooleanLikeHeterogeneousEnum {
No = 0,
Yes = "YES",
}
유니온 Type
- 유니온(Union) 타입 : (A || B)
- 자바스크립트의 OR 연산자
||
와 같은 의미
|
연산자를 이용하여 타입을 여러 개 연결하는 방식
const printOut = (input: string | number) => {
console.log(input);
}
printOut('문자열');
printOut(20);
printOut(true);
function getAge(age: any) {
age.toFixed();
return age;
}
getAge('20');
getAge(20);
function getAge(age: number | string) {
if (typeof age === 'number') {
age.toFixed();
return age;
}
if (typeof age === 'string') {
return age;
}
}
getAge('20');
getAge(20);
function padLeft(value: string, padding: any) {
if (typeof padding === 'number') {
return Array(padding + 1).join(' ') + value;
}
if (typeof padding === 'string') {
return padding + value;
}
throw new Error(`Expected string or number, got '${padding}'.`);
}
console.log(padLeft('Hello world', 4));
console.log(padLeft('Hello world', '!!!'));
console.log(padLeft('Hello world', true));
Type Alias (사용자 정의 타입)
const hero1: { name: string; power: number; height: number } = {
name: '슈퍼맨',
power: 1000,
height: 190,
};
const printHero = (hero: { name: string; power: number; height: number }) => {
console.log(hero.name, hero.power);
};
console.log(printHero(hero1));
- 매번 타입을 새로 작성하는 건 번거롭고 재사용이 불가능하다.
type Hero = {
name: string;
power: number;
height: number;
};
import type { Hero } from './type';
const hero1: Hero = {
name: '슈퍼맨',
power: 1000,
height: 190,
};
const printHero = (hero: Hero) => {
console.log(hero.name, hero.power);
};
console.log(printHero(hero1));
type Direction = 'UP' | 'DOWN' | 'LEFT' | 'RIGHT';
const printDirection = (direction: Direction) => {
console.log(direction);
};
printDirection('UP');
printDirection('UP!');
interface
interface Person {
name: string;
age: number;
}
const person1: Person = { name: 'js', age: 20 };
const person2: Person = { name: 'ljs', age: 'twenty' };
interface Person {
name: string;
age?: number;
}
const person1: Person = { name: 'js' };
interface Person {
readonly name: string;
age?: number;
}
const person1: Person = { name: 'js' };
person1.name = 'ljs';
let readOnlyArr: ReadonlyArray<number> = [1,2,3];
readOnlyArr.splice(0,1);
readOnlyArr.push(4);
readOnlyArr[0] = 100;
interface Person {
readonly name: string;
[key: string]: string | number;
}
const p1: Person = { name: 'js', birthday: '비밀', age: 20 };
interface Print {
(name: string, age: number): string;
}
const getNameAndAge: Print = function (name, age) {
return `name: ${name}, age: ${age}`;
};
interface Person {
name: string;
age: number;
}
interface Korean extends Person {
birth: 'KOR';
}
interface Korean {
name: string;
age: number;
birth: 'KOR';
}
interface Developer {
job: 'developer';
}
interface KorAndDev extends Korean, Developer {}
interface KorAndDev {
name: string;
age: number;
birth: 'KOR';
job: 'developer';
}
- intersection Type: 여러 타입을 모두 만족하는 하나의 타입
interface Person {
name: string;
age: number;
}
interface Developer {
name: string;
skill: string;
}
type DevJob = Person & Developer;
const nbcPerson: DevJob = {
name: 'a',
age: 20,
skill: 'ts',
};
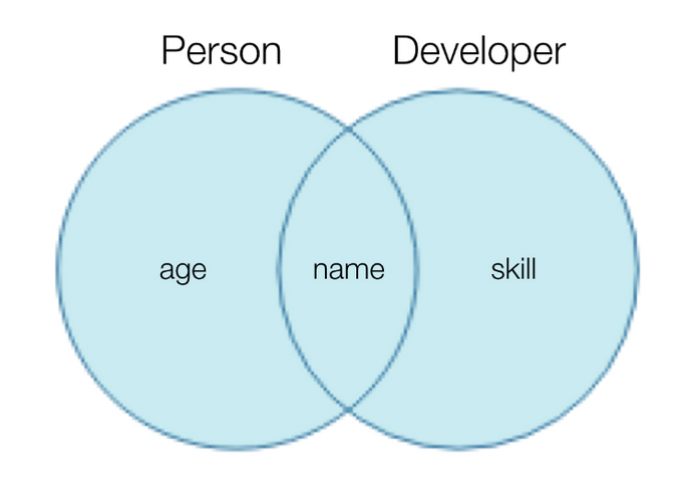
- type vs interface
- 타입 별칭과 인터페이스의 가장 큰 차이점은 타입의 확장 가능 / 불가능 여부이다.
- 인터페이스는 확장이 가능한데 반해 타입 별칭은 확장이 불가능하다.
- 따라서, 가능한한
type
보다는 interface
로 선언해서 사용하는 것을 추천합니다.
제네릭
- 제네릭이란 타입을 마치 함수의 파라미터처럼 사용하는 것
function getText(text: any): any {
return text;
}
getText('hi');
getText(10);
getText(true);
function getText<T>(text: T): T {
return text;
}
getText<string>('hi');
getText<number>(10);
getText<boolean>(true);
function getItemArray(arr: any[], index: number): any {
return arr[index];
}
function pushItemArray(arr: any[], item: any): void {
arr.push(item);
}
const techStack = ['js', 'react'];
const nums = [1, 2, 3, 4];
getItemArray(techStack, 0);
pushItemArray(techStack, 'ts');
getItemArray(nums, 0);
pushItemArray(nums, 5);
function getItemArray<T>(arr: T[], index: number): T {
return arr[index];
}
function pushItemArray<T>(arr: T[], item: T): void {
arr.push(item);
}
const techStack = ['js', 'react'];
const nums = [1, 2, 3, 4];
getItemArray(techStack, 0);
pushItemArray<string>(techStack, 'ts');
getItemArray(nums, 0);
pushItemArray(nums, 5);
function printOut<T>(input: T): T {
console.log(input.length);
return input;
}
function printOut<T>(input: T[]): T[] {
console.log(input.length);
return input;
}
printOut([1, 2, 3]);
function printOut<T>(input: T): T {
console.log(input.length);
return input;
}
interface LengthWise {
length: number;
}
function printOut<T extends LengthWise>(input: T): T {
console.log(input.length);
return input;
}
타입 추론
let a = 123;
let b = 'abc';
a = 'abc';
b = 123;
const c1 = 123;
const c2 = 'abc';
const arr = [1, 2, 3];
const [n1, n2, n3] = arr;
arr.push('a');
const obj = { numId: 1, stringId: '1' };
const { numId, stringId } = obj;
console.log(numId === stringId);
const func1 = (a = 'a', b = 1) => {
return `${a} ${b};`;
};
func1(3, 6);
const v1: number = func1('a', 1);
const func2 = (value: number) => {
if (value < 10) {
return value;
} else {
return `${value} is big`;
}
};