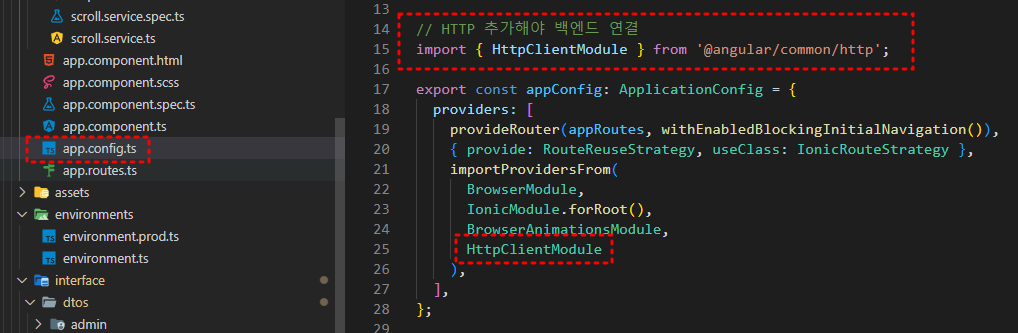
- HTTP 통신을 위해 app.config 에 모듈을 로드합니다.
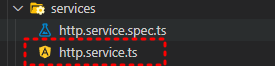
npx nx generate service --project 프로젝트명
import { Injectable } from '@angular/core';
import {
HttpClient,
HttpContext,
HttpHeaders,
HttpParams,
} from '@angular/common/http';
import { environment } from '../../environments/environment';
export type HttpServiceOptions = {
headers?:
| HttpHeaders
| {
[header: string]: string | string[];
};
context?: HttpContext;
observe?: 'body';
params?:
| HttpParams
| {
[param: string]:
| string
| number
| boolean
| ReadonlyArray<string | number | boolean>;
};
reportProgress?: boolean;
responseType?: 'json';
withCredentials?: boolean;
};
@Injectable({
providedIn: 'root',
})
export class HttpService {
private readonly baseUrl = `${environment.baseUrl}`;
constructor(private readonly httpClient: HttpClient) {}
get<T>(url: string, option?: HttpServiceOptions) {
return this.httpClient.get<T>(`${this.baseUrl}/${url}`, {
...option,
});
}
post<T>(url: string, body: any, option?: HttpServiceOptions) {
return this.httpClient.post<T>(`${this.baseUrl}/${url}`, body, {
...option,
});
}
put<T>(url: string, body: any, option?: HttpServiceOptions) {
return this.httpClient.put<T>(`${this.baseUrl}/${url}`, body, {
...option,
});
}
patch<T>(url: string, body: any, option?: HttpServiceOptions) {
return this.httpClient.patch<T>(`${this.baseUrl}/${url}`, body, {
...option,
});
}
delete<T>(url: string, option?: HttpServiceOptions) {
return this.httpClient.delete<T>(`${this.baseUrl}/${url}`, {
...option,
});
}
}
import { HttpService } from '../../services/http.service';
import { environment } from 'apps/client/src/environments/environment';
constructor(
private scrollService: ScrollService,
private router: Router,
private httpService: HttpService
) {}
ngOnInit() {
this.getItems();
}
getItems() {
this.httpService
.get<any>(`${environment.projectId}`)
.subscribe({
next: (res) => {
console.log(res);
},
});
}