Meeting Rooms II
- Difficulty: Medium
- Type: Heap/Priority Que
- link
Problem
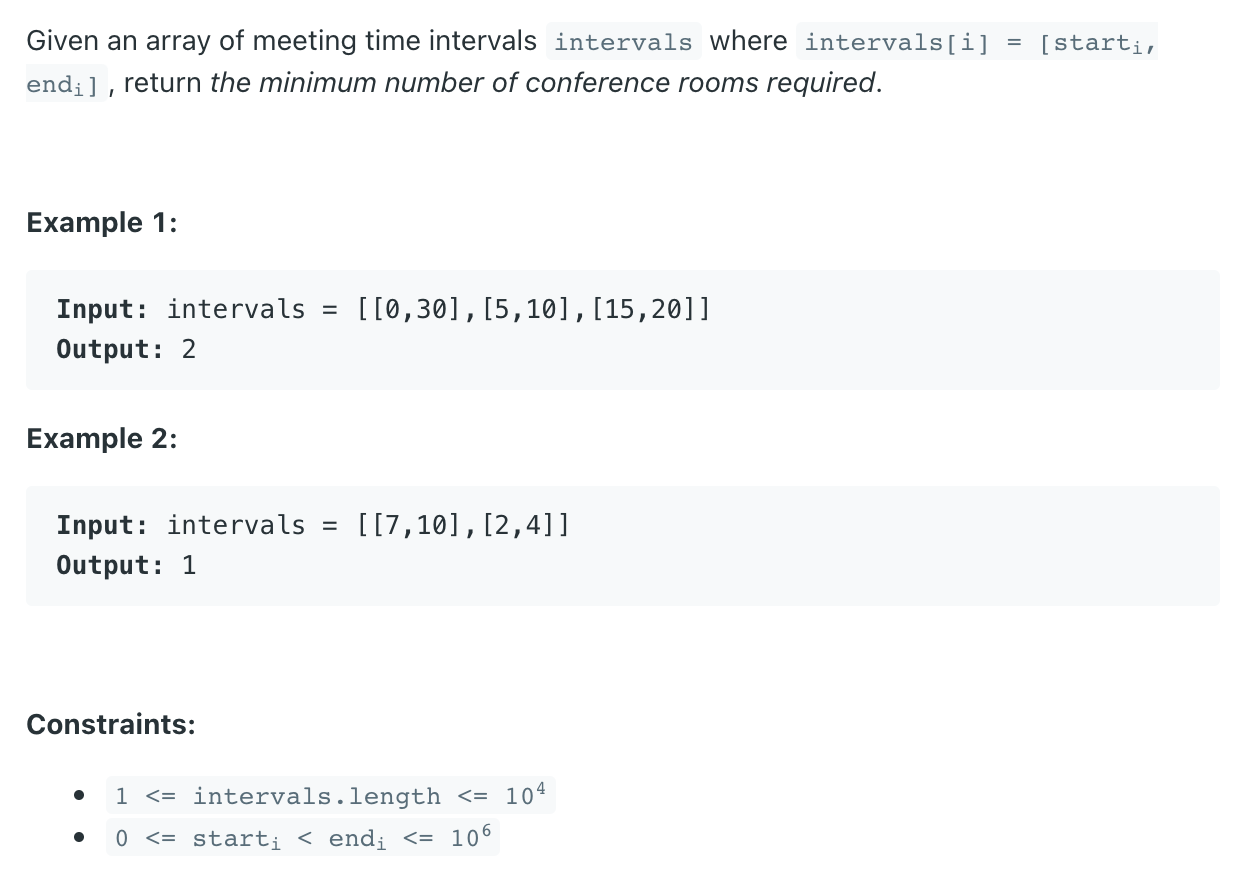
Solution
- Data structure: min heap
- Algorithm: priority que
- Sort the array first in ascending start time
- Save the end time in the min heap
- Rational: whenever the new meeting start time is greater than the minimum end time of the past, a room can be reused to host the new meeting
- Compare meeting start times with the minimum value in the min heap
- Pop from min heap when start time is greater than the minimum end time in the heap
import heapq
class Solution:
def minMeetingRooms(self, intervals: List[List[int]]) -> int:
intervals.sort(key=lambda x: (x[0],x[1]))
rooms = []
for meeting in intervals:
if not rooms:
heapq.heappush(rooms,meeting[1])
elif rooms[0] <= meeting[0]:
heapq.heappop(rooms)
heapq.heappush(rooms,meeting[1])
else:
heapq.heappush(rooms,meeting[1])
return len(rooms)
Even if you're working remotely, it's important to have occasional meetings, and sometimes you need to find a large space for a presentation. With the best room booking software Unspot, finding a place won't take much time. A modern office booking system helps you find the optimal space by price, time, and location.