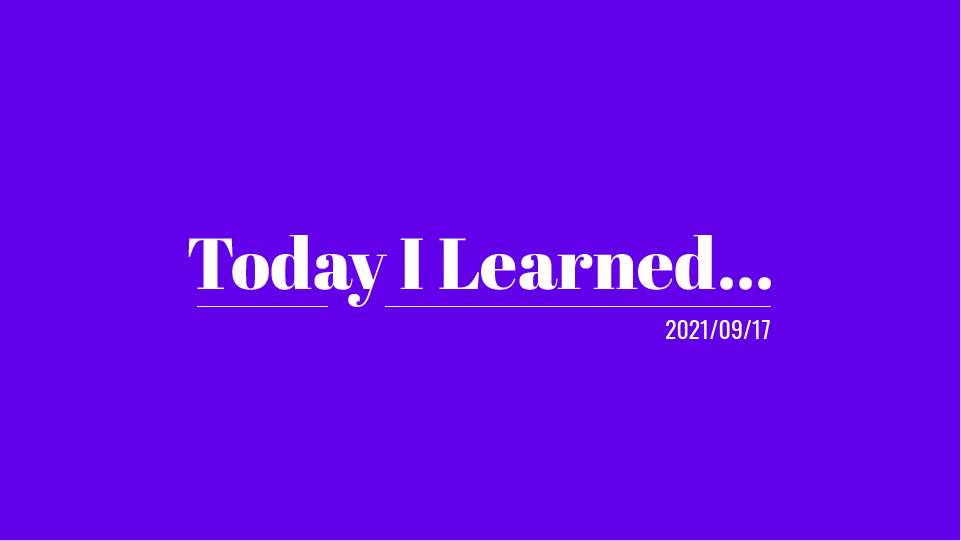
Today I Learned
Props
- 해당 컴포넌트의 속성(property)
- 부모 컴포넌트로부터 전달받은 변경할 수 없는 값
- 컴포넌트는 props를 전달인자처럼 받아 사용할 수 있다. props는 객체의 형태를 가진다.
- React의 단방향 하향식 데이터 흐름 원칙에 따라 읽을 수만 있고 변경할 수 없다 (read-only). 모든 컴포넌트는 자신의 props를 다룰 때 순수 함수(입력값을 바꾸지 않고 동일 입력값에 동일 결과를 반환하는 함수)처럼 동작해야 한다.
props를 사용하는 방법
const TweetList = () => {
const tweets = [
{ username: "alexl", content: "you are so great" },
{ username: "lewisk", content: "and i love you" },
];
return (
<article className="tweets-list-container">
<ul>
{tweets.map((el) => (
<Tweet username={el.username} content={el.content} />
))}
</ul>
</article>
);
};
const Tweet = (userInfo) => {
return (
<article className="tweet-container">
<p className="tweet-username">{userInfo.username}</p>
<p className="tweet-content">{userInfo.content}</p>
</article>
);
};
props.children
- 컴포넌트의 여는 태그와 닫는 태그 사이에 있는 값은
props.children
에 담겨 전달된다.
const Parent = () => {
return (
<div className="parent">
<Child>
<p>I am your father</p>
<p>No, that's not true!!!</p>
</Child>
</div>
)
}
const Child = (props) => {
return (
<div className="child">
<p>Length: {props.children.length}</p>
{props.children}
</div>
)
}
props를 전달하는 여러가지 방법
const Child = (props) => {
return (
<p>Welcome, {props.name} from {props.nationality}</p>
)
}
const Parent = () => {
return (
<div className="parent">
<Child name={"Alex"} nationality={"South Korea"} />
</div>
)
}
const Parent = () => {
return (
<div className="parent">
<Child name="Alex" nationality="South Korea" />
</div>
)
}
const Parent = () => {
const userInfo = {
name: "Alex",
nationality: "South Korea"
}
return (
<div className="parent">
<Child {...userInfo} />
</div>
)
}
State
- 컴포넌트 내에서 사용하는 변경할 수 있는 값 (동적 데이터)
- 상태(state)가 변경되면 해당 컴포넌트(& 자식 컴포넌트)가 리렌더링되면서 화면에 새로 그려진다.
- React v16.8에서 Hook이 추가되면서 함수 컴포넌트에서도 state를 사용할 수 있게 되었다.
useState를 사용하는 방법
useState()
는 React에서 제공하는 Hook으로 이를 이용해 state를 다룰 수 있다. useState()
는 받은 인자를 초기값으로 state를 생성하고 state 변수와 그 변수를 업데이트하는 함수를 요소로 하는 배열을 반환한다.
- state 변수는 직접 변경할 수 없고 반드시 연결된 함수를 통해 변경하여야 한다.
- 왜 일반 변수가 아닌 state를 사용할까? => 일반 변수는 함수가 끝날 때 사라지지만 state 변수는 React에 의해 사라지지 않는다.
- state 변수를 선언할 때
const
를 사용할 수 있는 이유는? => 컴포넌트가 리랜더링될 때마다 새로운 scope에서 새로운 변수를 만들기 때문에 const
를 사용할 수 있다. 리랜더링 전과 후의 state 변수는 서로 다른 변수다. 다만 React에서 해당 state 변수를 기억하고 가장 최근값을 전달한다.
import { useState } from 'react';
const Checkbox = () => {
const [isChecked, setIsChecked] = useState(false);
const handleChecked = (evt) => {
setIsChecked(evt.target.checked);
}
return (
<div>
<input type="checkbox" checked={isChecked} onChange={handleChecked} />
<p>{isChecked ? "Checked" : "Unchecked"}</p>
</div>
)
}