11.1 JSTL이란?
JSP에서 자주 사용되는 조건문, 반복문 등을 처리해주는 태그를 모아 표준으로 만들어 놓은 라이브러리
11.3 Core 태그
11.3.1 <c:set> 태그
<c:set var="변수명" value="값" scope="영역" />
<c:set var="변수명" scope="영역">
value 속성에 들어갈 값
</c:set>
속성명 | 기능 |
---|
var | 변수명을 설정 |
value | 변수에 할당할 값 |
scope | 변수를 생성할 영역을 지정. page가 기본값 |
target | 자바빈즈를 설정 |
property | 자바빈즈의 속성, 멤버 변수의 값을 지정 |
Set1.jsp
<%@ page import="java.util.Date"%>
<%@ page import="common.Person"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head><title>JSTL - set 1</title></head>
<body>
<c:set var="directVar" value="100" />
<c:set var="elVar" value="${ directVar mod 5}" />
<c:set var="expVar" value="<%= new Date() %>" />
<c:set var="betweenVar">변수값 요렇게 설정</c:set>
<h4>EL을 이용해 변수 출력</h4>
<ul>
<li>directVar : ${ pageScope.directVar }</li>
<li>elVar : ${ elVar }</li>
<li>expVar : ${ expVar }</li>
<li>betweenVar : ${ betweenVar }</li>
</ul>
<h4>자바빈즈 생성 1 - 생성자 사용</h4>
<c:set var="personVar1" value='<%= new Person("박문수", 50) %>'
scope="request" />
<ul>
<li>이름 : ${ requestScope.personVar1.name }</li>
<li>나이 : ${ personVar1.age}</li>
</ul>
<h4>자바빈즈 생성 2 - target, property 사용</h4>
<c:set var="personVar2" value="<%= new Person() %>" scope="request" />
<c:set target="${personVar2 }" property="name" value="정약용" />
<c:set target="${personVar2 }" property="age" value="60" />
<ul>
<li>이름 : ${ personVar2.name }</li>
<li>나이 : ${ requestScope.personVar2.age }</li>
</ul>
</body>
</html>
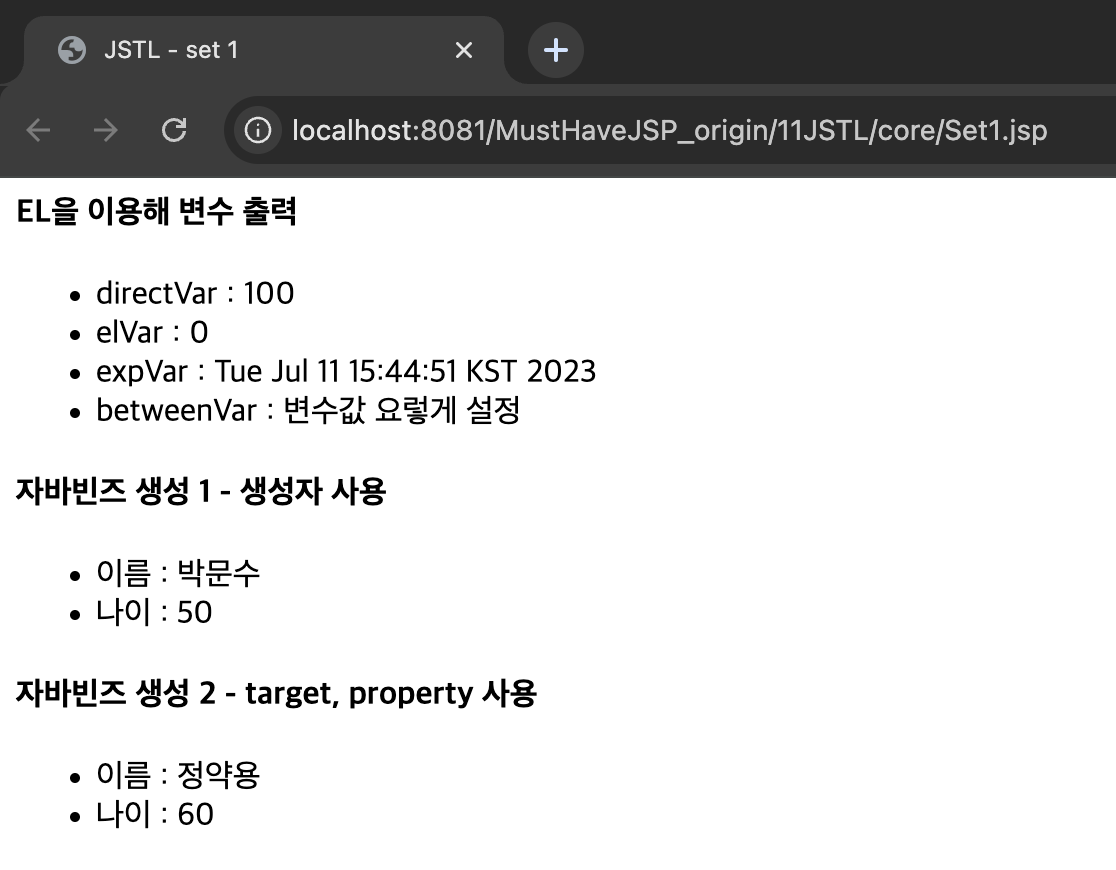
Set2.jsp
<%@ page import="common.Person"%>
<%@ page import="java.util.ArrayList"%>
<%@ page import="java.util.HashMap"%>
<%@ page import="java.util.Map"%>
<%@ page import="java.util.Vector"%>
<%@ page import="java.util.List"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head><title>JSTL - set 2</title></head>
<body>
<h4>List 컬렉션 이용하기</h4>
<%
ArrayList<Person> pList = new ArrayList<Person>();
pList.add(new Person("성삼문", 55));
pList.add(new Person("박팽년", 60));
%>
<c:set var="personList" value="<%= pList %>" scope="request" />
<ul>
<li>이름 : ${ requestScope.personList[0].name }</li>
<li>나이 : ${ personList[0].age }</li>
</ul>
<h4>Map 컬렉션 이용하기</h4>
<%
Map<String, Person> pMap = new HashMap<String, Person>();
pMap.put("personArgs1", new Person("하위지", 65));
pMap.put("personArgs2", new Person("이개", 67));
%>
<c:set var="personMap" value="<%= pMap %>" scope="request" />
<ul>
<li>아이디 : ${ requestScope.personMap.personArgs2.name }</li>
<li>비번 : ${ personMap.personArgs2.age }</li>
</ul>
</body>
</html>
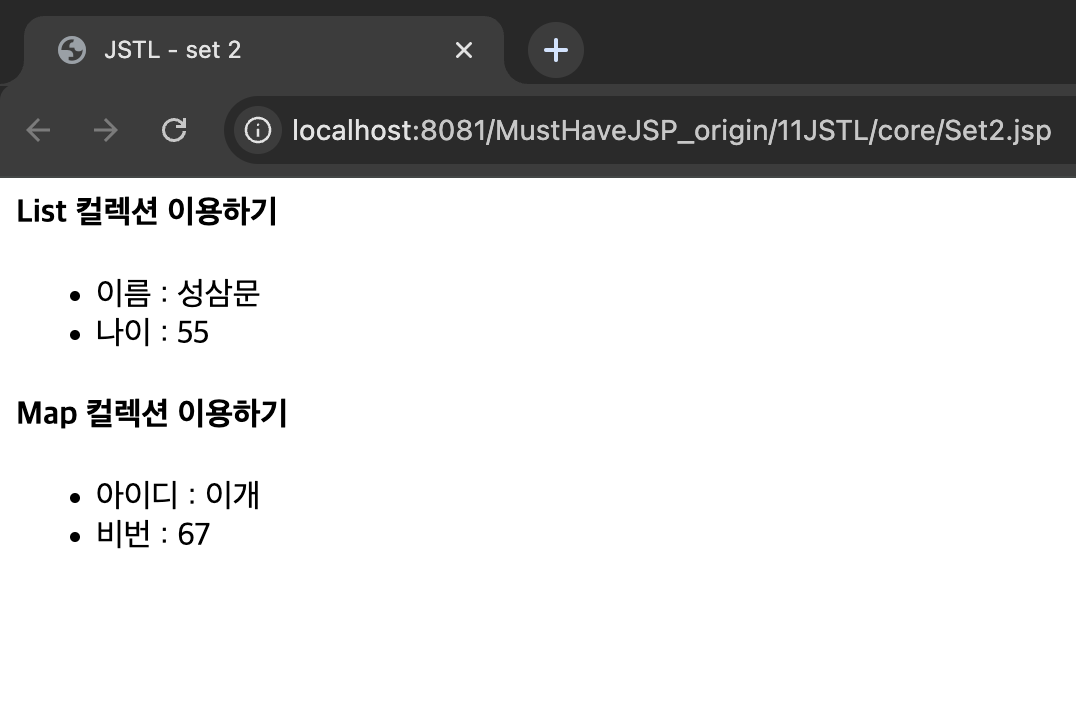
11.3.2 <c:remove> 태그
<c:remove var="변수명" scope="영역" />
속성명 | 기능 |
---|
var | 삭제할 변수명 |
scope | 삭제할 변수의 영역. 없다면 모든 영역의 변수가 삭제 |
Remove.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<c:set var="scopeVar" value="Page Value" />
<c:set var="scopeVar" value="Request Value" scope="request" />
<c:set var="scopeVar" value="Session Value" scope="session" />
<c:set var="scopeVar" value="Application Value" scope="application" />
<html>
<head><title>JSTL - remove</title></head>
<body>
<h4>출력하기</h4>
<ul>
<li>scopeVar : ${ scopeVar }</li>
<li>requestScope.scopeVar : ${ requestScope.scopeVar }</li>
<li>sessionScope.scopeVar : ${ sessionScope.scopeVar }</li>
<li>applicationScope.scopeVar : ${ applicationScope.scopeVar }</li>
</ul>
<h4>session 영역에서 삭제하기</h4>
<c:remove var="scopeVar" scope="session" />
<ul>
<li>sessionScope.scopeVar : ${ sessionScope.scopeVar }</li>
</ul>
<h4>scope 지정 없이 삭제하기</h4>
<c:remove var="scopeVar" />
<ul>
<li>scopeVar : ${ scopeVar }</li>
<li>requestScope.scopeVar : ${ requestScope.scopeVar }</li>
<li>applicationScope.scopeVar : ${ applicationScope.scopeVar }</li>
</ul>
</body>
</html>
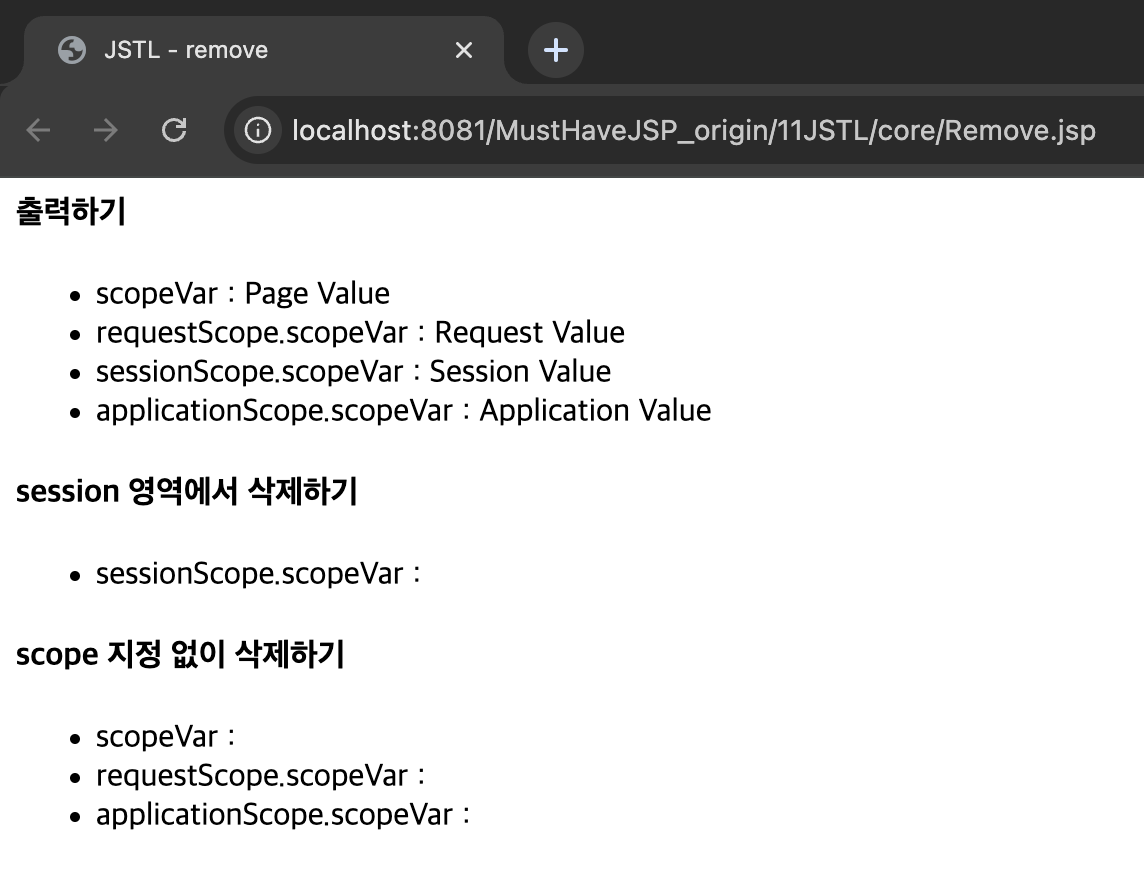
11.3.3 <c:if> 태그
<c:if test="조건" var="변수명" scope="영역">
조건이 true일 때 출력할 문장
</c:if>
속성명 | 기능 |
---|
test | if문에서 사용할 조건 |
var | 조건의 결과를 저장할 변수명 |
scope | 변수가 저장될 영역 |
If.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head><title>JSTL - if</title></head>
<body>
<c:set var="number" value="100" />
<c:set var="string" value="JSP" />
<h4>JSTL의 if 태그로 짝수/홀수 판단하기</h4>
<c:if test="${ number mod 2 eq 0 }" var="result">
${ number }는 짝수입니다. <br />
</c:if>
result : ${ result } <br />
<h4>문자열 비교와 else 구문 흉내내기</h4>
<c:if test="${ string eq 'Java' }" var="result2">
문자열은 Java입니다. <br />
</c:if>
<c:if test="${ not result2 }">
'Java'가 아닙니다. <br />
</c:if>
<h4>조건식 주의사항</h4>
<c:if test="100" var="result3">
EL이 아닌 정수를 지정하면 false
</c:if>
result3 : ${result3 } <br />
<c:if test="tRuE" var="result4">
대소문자 구분 없이 "tRuE"인 경우 true <br />
</c:if>
result4 : ${ result4 } <br />
<c:if test=" ${ true } " var="result5">
EL 양쪽에 빈 공백이 있는 경우 false <br />
</c:if>
result5 : ${ result5 } <br />
</body>
</html>
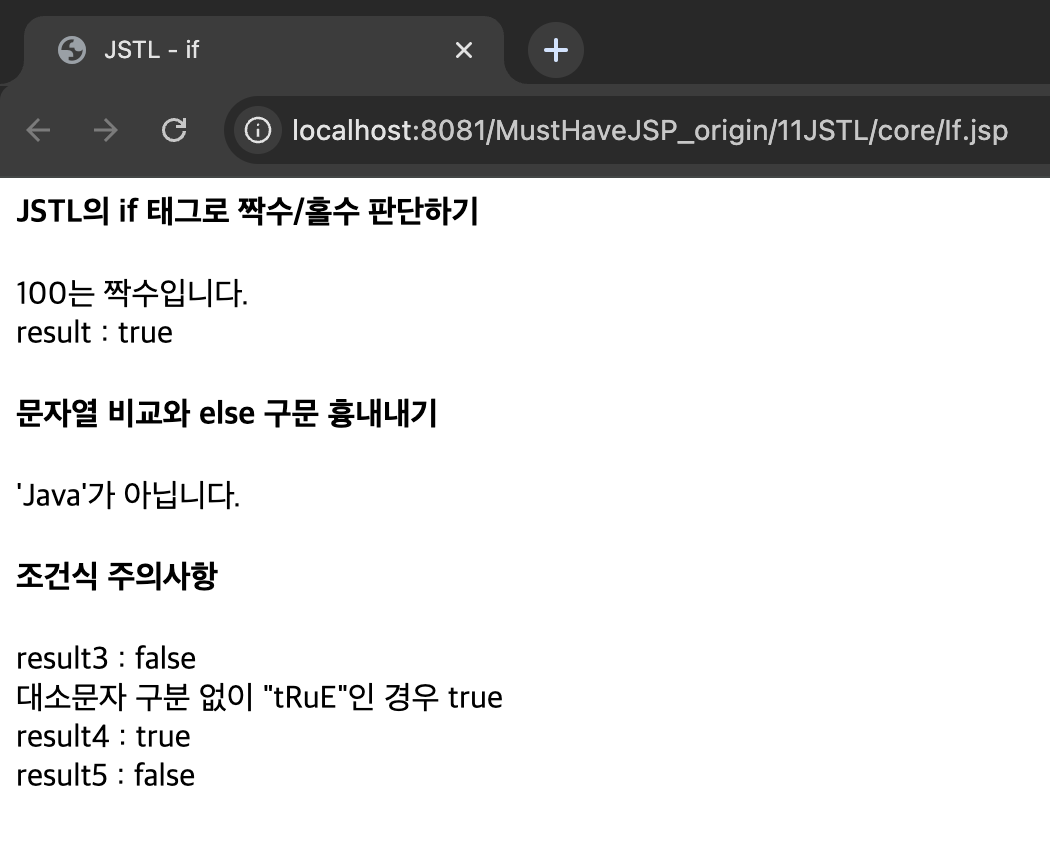
11.3.4 <c:choose>, <c:when>, <c:otherwise>
<c:choose>
<c:when test="조건1">조건1을 만족하는 경우</c:when>
<c:when test="조건2">조건2을 만족하는 경우</c:when>
<c:otherwise>아무 조건도 만족하지 않는 경우</c:otherwise>
</c:choose>
Choose.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head><title>JSTL - choose/when/otherwise</title></head>
<body>
<c:set var="number" value="100" />
<h4>choose 태그로 홀짝 판단하기</h4>
<c:choose>
<c:when test="${ number mod 2 eq 0 }">
${ number }는 짝수입니다.
</c:when>
<c:otherwise>
${ number }는 홀수입니다.
</c:otherwise>
</c:choose>
<h4>국,영,수 점수를 입력하면 평균을 내어 학점 출력</h4>
<form>
국어 : <input type="text" name="kor" /> <br />
영어 : <input type="text" name="eng" /> <br />
수학 : <input type="text" name="math" /> <br />
<input type="submit" value="학점 구하기" />
</form>
<c:if test="${ not (empty param.kor or empty param.eng or empty param.math) }">
<c:set var="avg" value="${ (param.kor + param.eng + param.math) / 3}" />
평균 점수는 ${avg }으로
<c:choose>
<c:when test="${ avg >= 90 }">A 학점</c:when>
<c:when test="${ avg >= 80 }">B 학점</c:when>
<c:when test="${ avg ge 70 }">C 학점</c:when>
<c:when test="${ avg ge 60 }">D 학점</c:when>
<c:otherwise>F 학점</c:otherwise>
</c:choose>
입니다.
</c:if>
</body>
</html>
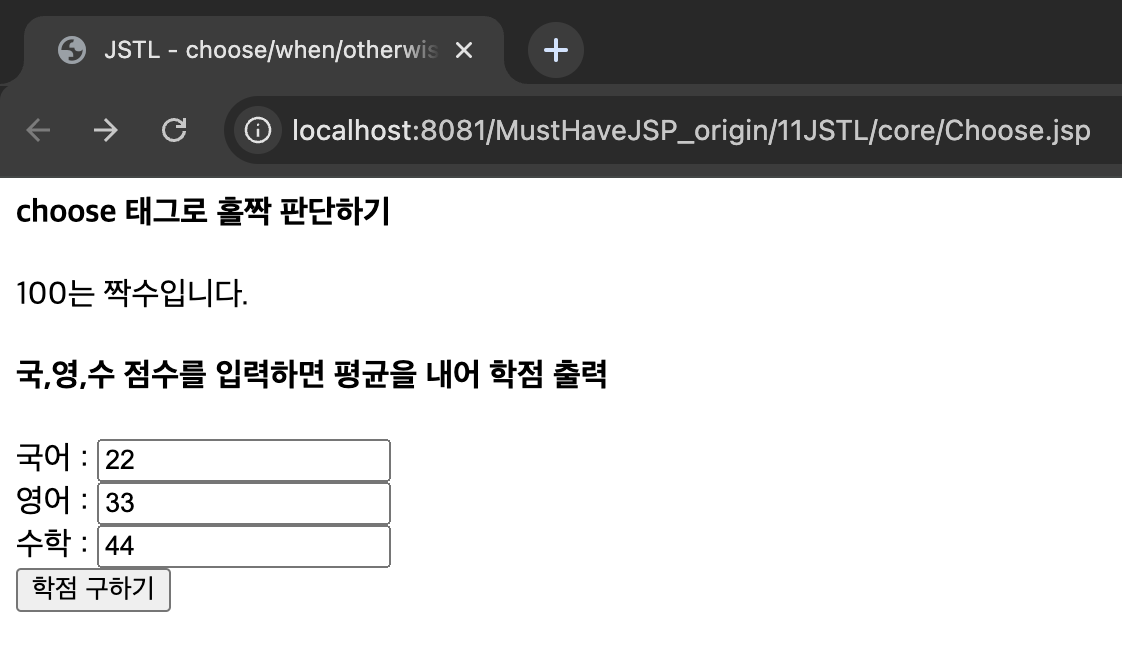
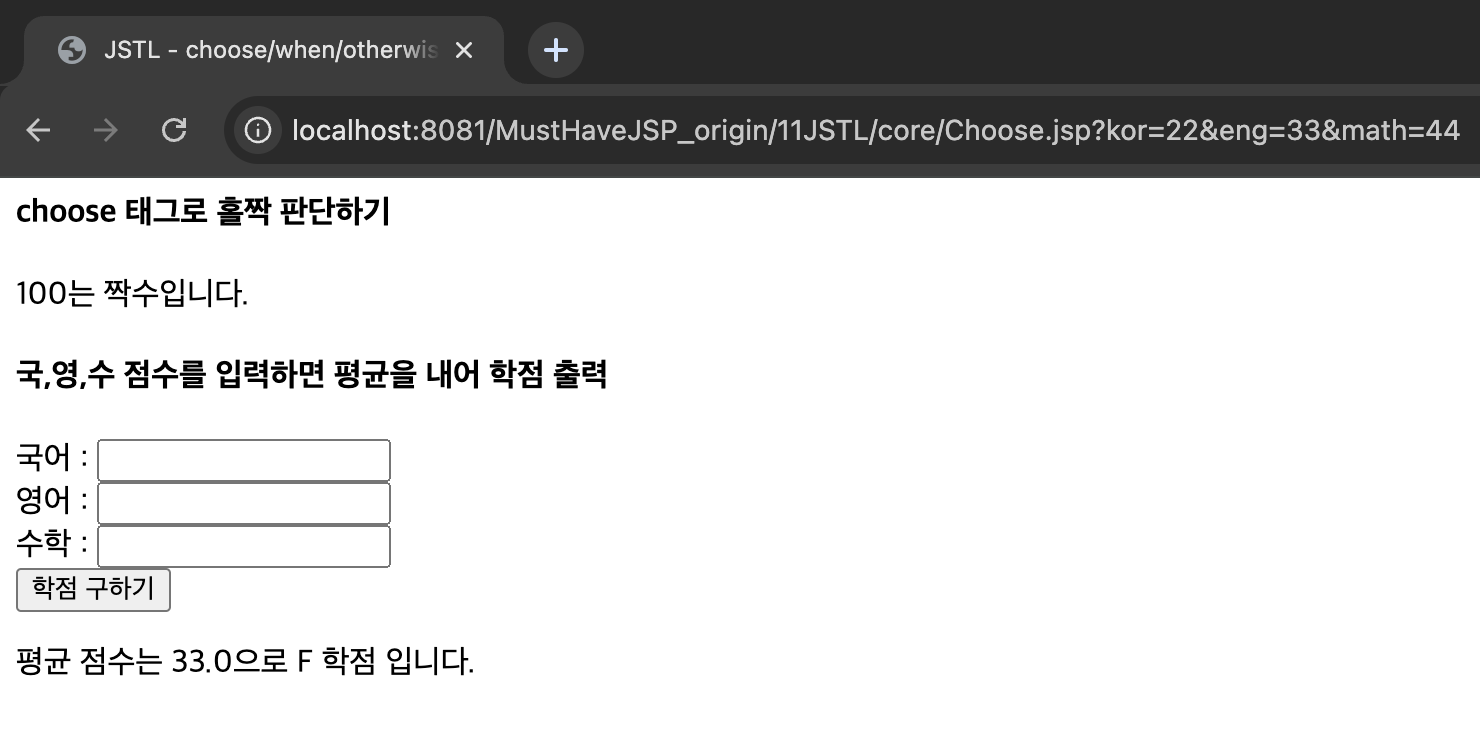
11.3.5 <c:forEach> 태그
<c:forEach var="변수명" begin="시작값" end="마지막값" step="증가값" />
<c:forEach var="변수명" items="컬렉션 혹은 배열" />
ForEachNormal.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head><title>JSTL - forEach 1</title></head>
<body>
<h4>일반 for문 형태의 forEach 태그</h4>
<c:forEach begin="1" end="3" step="1" var="i">
<p>반복 ${ i }입니다</p>
</c:forEach>
<h4>varStatus 속성 살펴보기</h4>
<table border="1">
<c:forEach begin="3" end="5" var="i" varStatus="loop">
<tr>
<td>count : ${ loop.count }</td>
<td>index : ${ loop.index }</td>
<td>current : ${ loop.current }</td>
<td>first : ${ loop.first }</td>
<td>last : ${ loop.last }</td>
</tr>
</c:forEach>
</table>
<h4>1에서 100까지 정수 중 홀수의 합</h4>
<c:forEach begin="1" end="100" var="j">
<c:if test="${ j mod 2 ne 0}">
<c:set var="sum" value="${ sum + j }" />
</c:if>
</c:forEach>
1~100 사이의 정수 중 홀수의 합은? ${ sum }
</body>
</html>
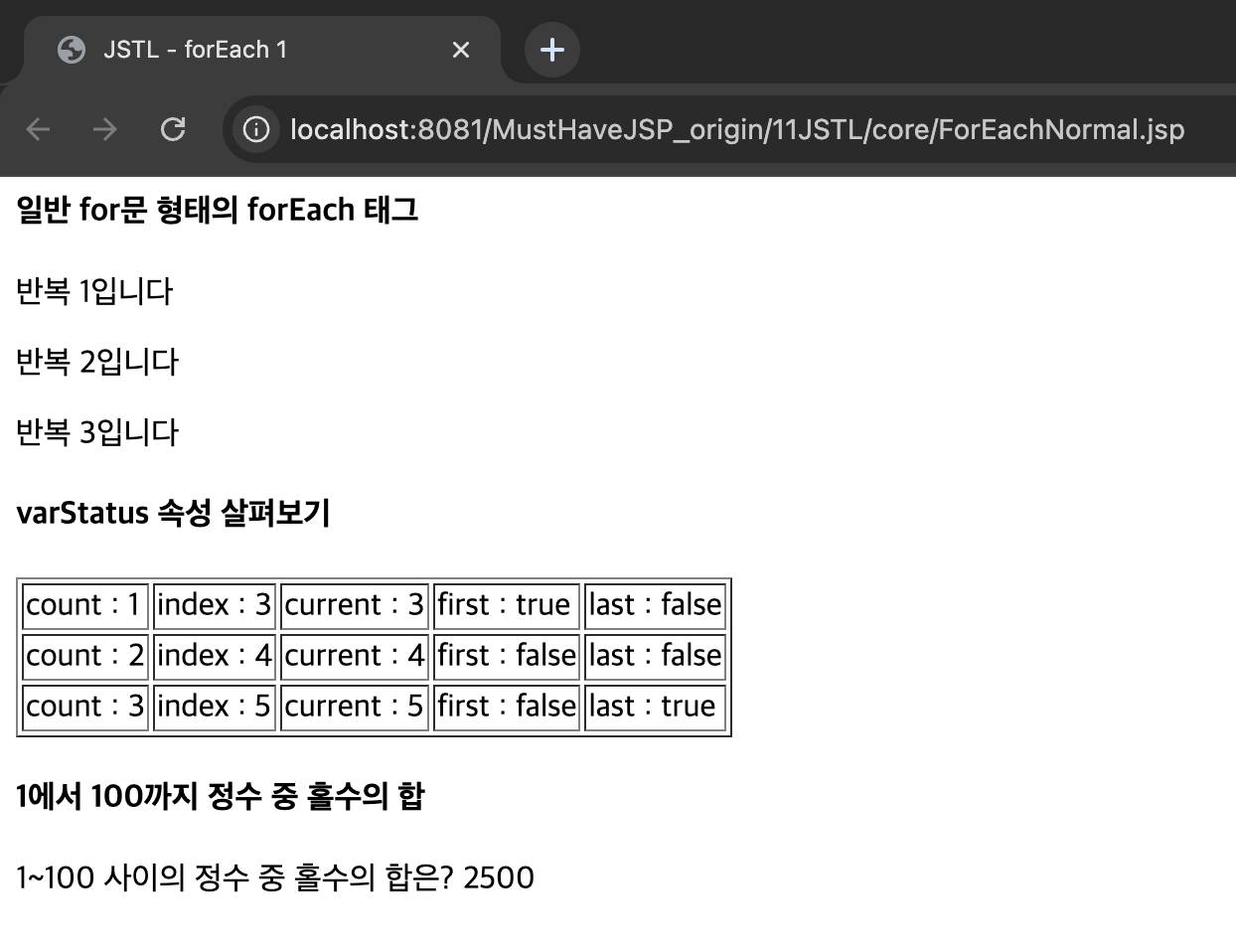
ForEachExtend1.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head><title>JSTL - forEach2</title></head>
<body>
<h4>향상된 for문 형태의 forEach 태그</h4>
<%
String[] rgba = {"Red", "Green", "Blue", "Black"};
%>
<c:forEach items="<%= rgba %>" var="c">
<span style="color:${ c };">${ c }</span>
</c:forEach>
<h4>varStatus 속성 살펴보기</h4>
<table border="1">
<c:forEach items="<%= rgba %>" var="c" varStatus="loop">
<tr>
<td>count : ${ loop.count }</td>
<td>index : ${ loop.index }</td>
<td>current : ${ loop.current }</td>
<td>first : ${ loop.first }</td>
<td>last : ${ loop.last }</td>
</tr>
</c:forEach>
</table>
</body>
</html>
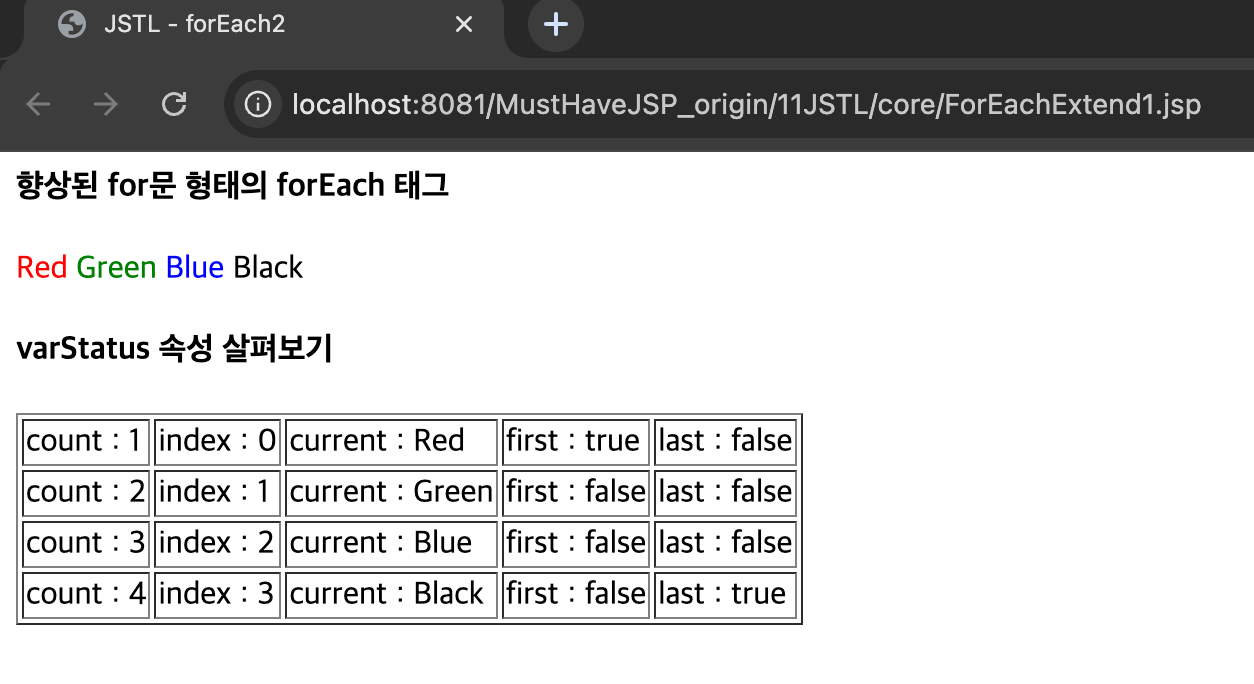
ForEachExtend2.jsp
<%@ page import="java.util.HashMap"%>
<%@ page import="java.util.Map"%>
<%@ page import="common.Person"%>
<%@ page import="java.util.LinkedList"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head><title>JSTL - forEach 2</title></head>
<body>
<h4>List 컬렉션 사용하기</h4>
<%
LinkedList<Person> lists = new LinkedList<Person>();
lists.add(new Person("맹사성", 34));
lists.add(new Person("장영실", 44));
lists.add(new Person("신숙주", 54));
%>
<c:set var="lists" value="<%= lists %>"/>
<c:forEach items="${ lists }" var="list">
<li>
이름 : ${ list.name }, 나이 : ${ list.age }
</li>
</c:forEach>
<h4>Map 컬렉션 사용하기</h4>
<%
Map<String,Person> maps = new HashMap<String,Person>();
maps.put("1st", new Person("맹사성", 34));
maps.put("2nd", new Person("장영실", 44));
maps.put("3rd", new Person("신숙주", 54));
%>
<c:set var="maps" value="<%= maps %>" />
<c:forEach items="${ maps }" var="map">
<li>Key => ${ map.key } <br />
Value => 이름 : ${ map.value.name }, 나이 : ${ map.value.age }</li>
</c:forEach>
</body>
</html>
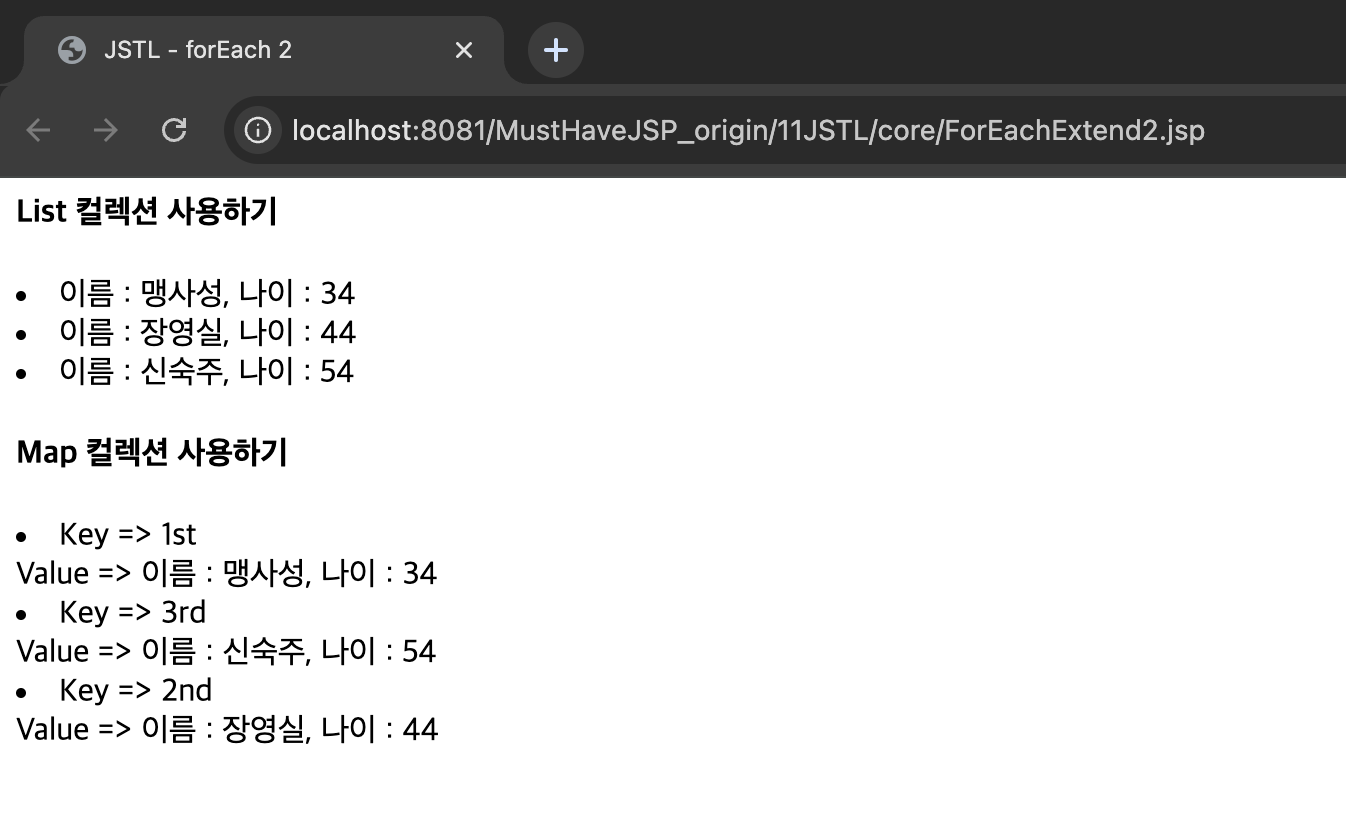
11.3.6 <c:forTokens> 태그
<c:forTokens items="문자열" delims="구분자" var="변수명" />
ForTokens.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<meta charset="UTF-8">
<head><title>JSTL - forTokens</title></head>
<body>
<%
String rgba = "Red,Green,Blue,Black";
%>
<h4>JSTL의 forTokens 태그 사용</h4>
<c:forTokens items="<%= rgba %>" delims="," var="color">
<span style="color:${ color };">${ color }</span> <br />
</c:forTokens>
</body>
</html>
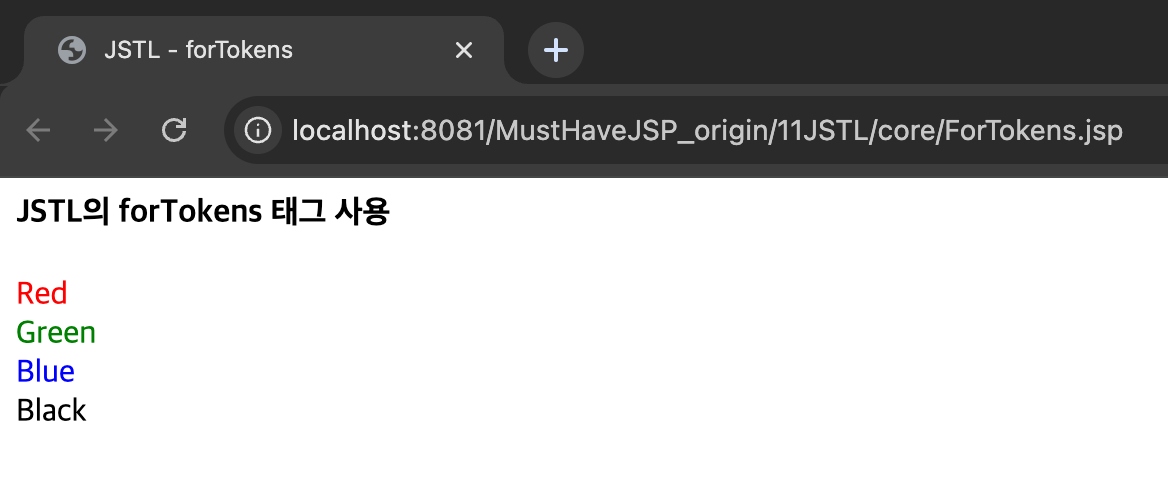
11.3.7 <c:import> 태그
<c:import url="페이지 경로 혹은 URL" scope="영역" />
<c:import url="페이지 경로 혹은 URL" var="변수명" scope="영역" />
${ 변수명 }
<c:import url="페이지 경로 혹은 URL?매개변수1=값1" >
<c:param name="매개변수2" value="값2" />
</c:import>
OtherPage.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<h4>OtherPage.jsp</h4>
<ul>
<li>저장된 값 : ${ requestVar }</li>
<li>매개변수 1 : ${ param.user_param1 }</li>
<li>매개변수 2 : ${ param.user_param2 }</li>
</ul>
GoldPage.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<c:import url="https://goldenrabbit.co.kr/" />
Import.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head><title>JSTL - import</title></head>
<body>
<c:set var="requestVar" value="MustHave" scope="request" />
<c:import url="/11JSTL/inc/OtherPage.jsp" var="contents">
<c:param name="user_param1" value="JSP" />
<c:param name="user_param2" value="기본서" />
</c:import>
<h4>다른 문서 삽입하기</h4>
${contents }
<h4>외부 자원 삽입하기</h4>
<iframe src="../inc/GoldPage.jsp" style="width:100%;height:600px;"></iframe>
</body>
</html>
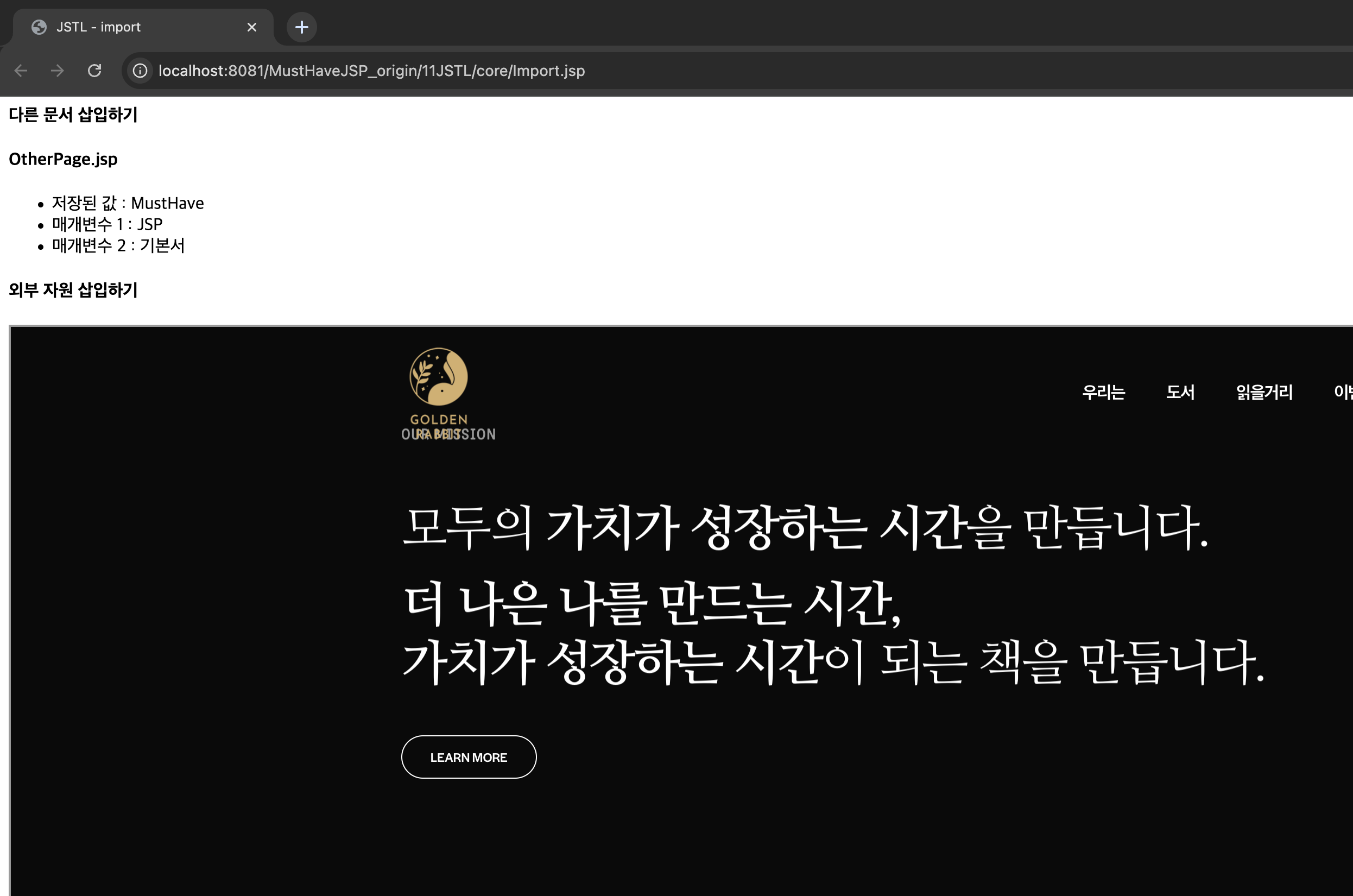
11.3.8 <c:redirect> 태그
<c:redirect url="이동할 경로 및 URL" />
Redirect.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head><title>JSTL - redirect</title></head>
<body>
<c:set var="requestVar" value="MustHave" scope="request" />
<c:redirect url="/11JSTL/inc/OtherPage.jsp">
<c:param name="user_param1" value="출판사" />
<c:param name="user_param2" value="골든래빗" />
</c:redirect>
</body>
</html>
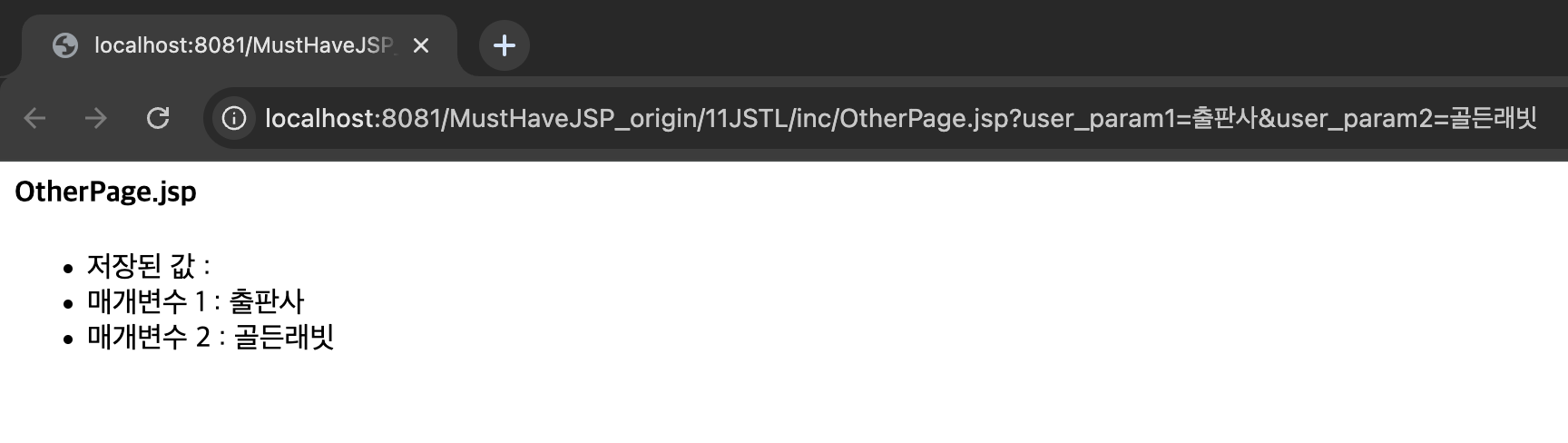
11.3.9 <c:url> 태그
<c:url value="설정한 경로" scope="영역" />
<c:url value="설정한 경로" scope="영역" var="변수명" />
${ 변수명 }
Url.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head><title>JSTL - url</title></head>
<body>
<h4>url 태그로 링크 걸기</h4>
<c:url value="/11JSTL/inc/OtherPage.jsp" var="url">
<c:param name="user_param1" value="Must" />
<c:param name="user_param2">Have</c:param>
</c:url>
<a href="${url }">OtherPage.jsp 바로가기</a>
</body>
</html>
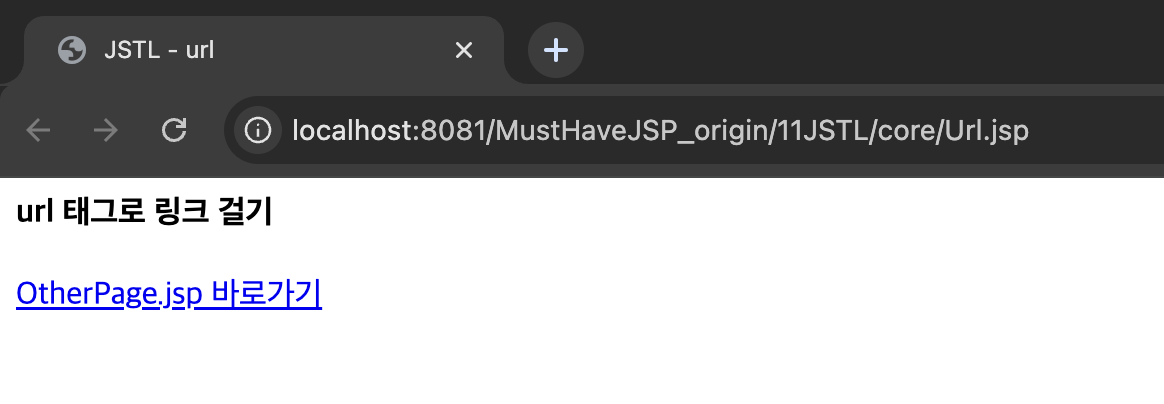
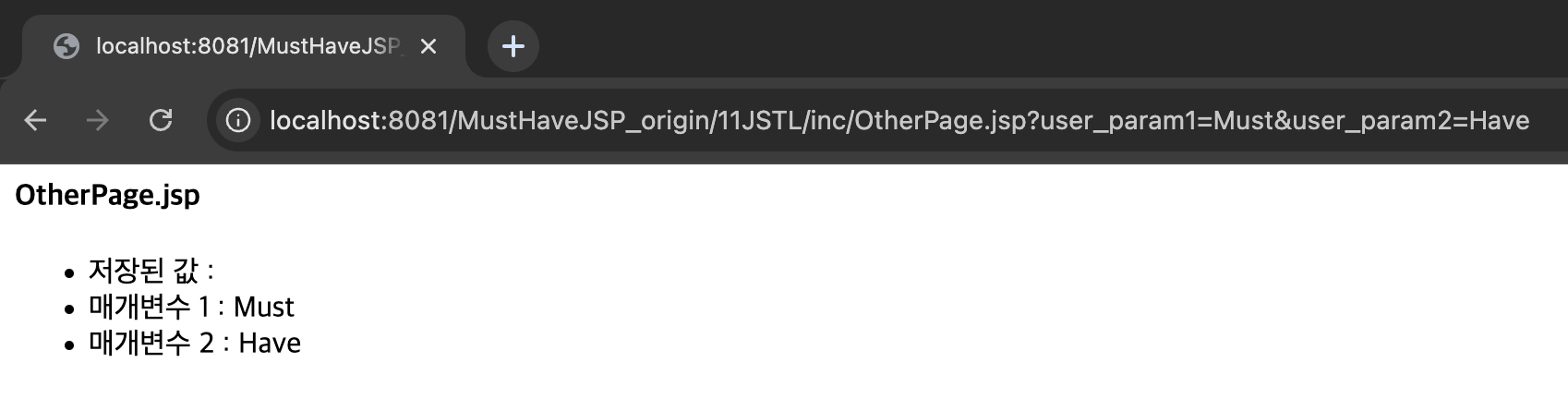
11.3.10 <c:out> 태그
<c:out value="출력할 변수" default="기본값" escapeXml="특수문자 처리 유무" />
Out.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head><title>JSTL - out</title></head>
<body>
<c:set var="iTag">
i 태그는 <i>기울임</i>을 표현합니다.
</c:set>
<h4>기본 사용</h4>
<c:out value="${ iTag }" />
<h4>escapeXml 속성</h4>
<c:out value="${ iTag }" escapeXml="false" />
<h4>default 속성</h4>
<c:out value="${ param.name }" default="이름 없음" />
<c:out value="" default="빈 문자열도 값입니다." />
</body>
</html>
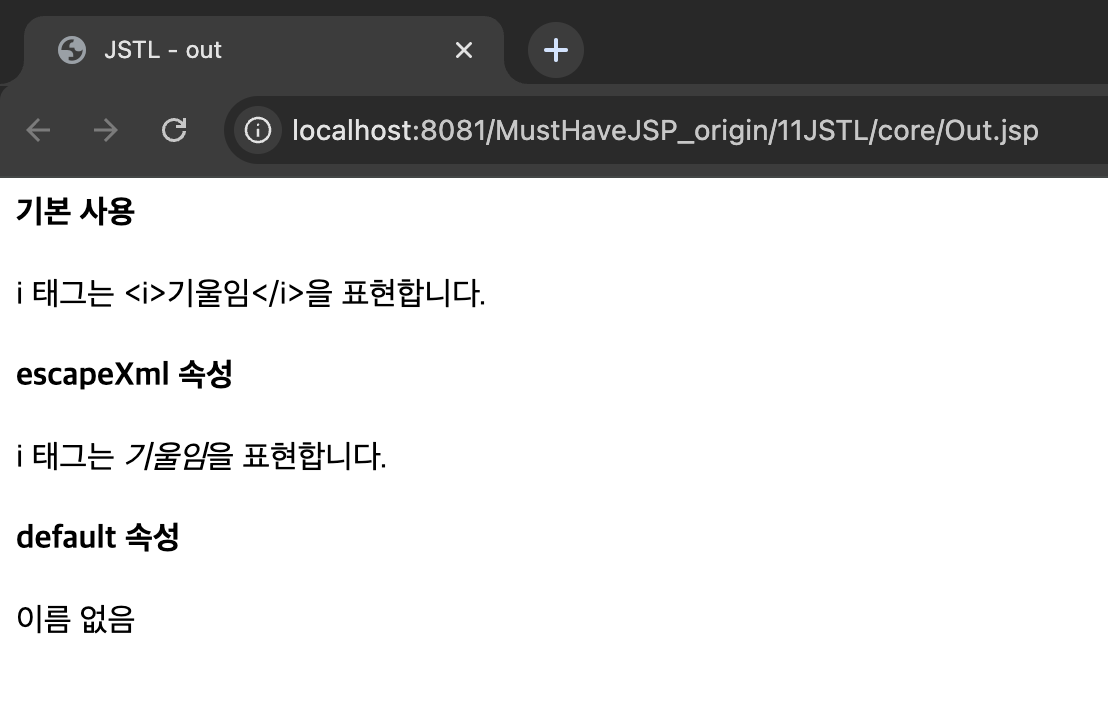
11.3.11 <c:catch> 태그
<c:catch var="변수명">
실행 코드
</c:catch>
Catch.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head><title>JSTL - catch</title></head>
<body>
<h4>자바 코드에서의 예외</h4>
<%
int num1 = 100;
%>
<c:catch var="eMessage">
<%
int result = num1 / 0;
%>
</c:catch>
예외 내용 : ${ eMessage }
<h4>EL에서의 예외</h4>
<c:set var="num2" value="200" />
<c:catch var="eMessage">
${"일" + num2 }
</c:catch>
예외 내용 : ${ eMessage }
</body>
</html>
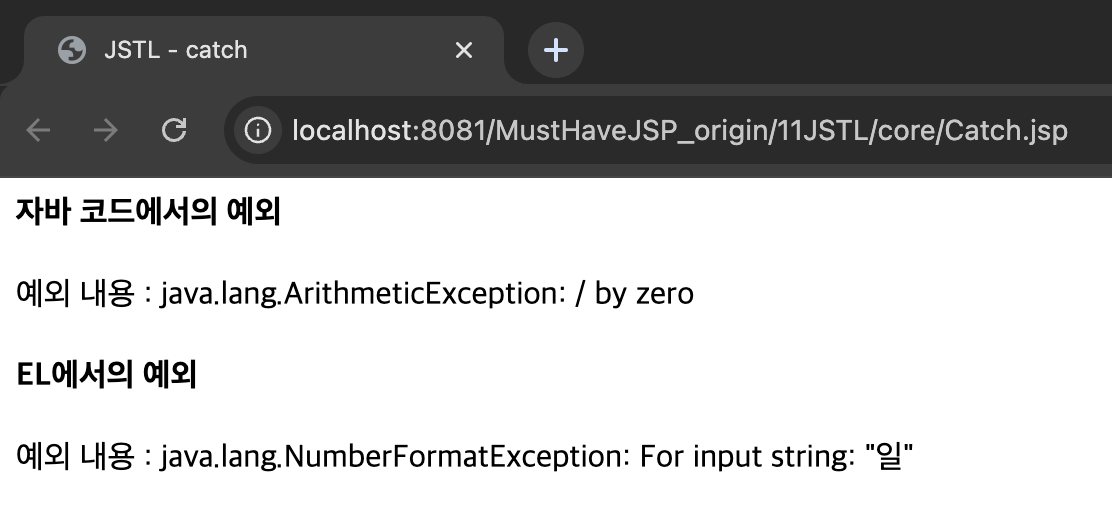
국가별로 다양한 언어, 날짜, 시간, 숫자 형식을 설정 할 때 사용.
분류 |
태그명 |
기능 |
숫자 포맷 |
formatNumber |
숫자 포맷을 설정 |
parseNumber |
문자열을 숫자 포맷으로 변환 |
날짜 포맷 |
formaDate |
날짜나 시간의 포맷을 설정 |
parseDate |
문자열을 날짜 포맷으로 변환 |
타임존 설정 |
setTimeZone |
시간대 설정 정보를 변수에 저장 |
timeZone |
시간대를 설정 |
로케일 설정 |
setLocale |
통화 기호나 시간대를 설정한 지역에 맞게 표시 |
requestEncoding |
요청 매개변수의 문자셋을 설정 |
11.4.1 숫자 포맷팅 및 파싱
<fmt:formatNumber value="출력할 숫자" type="문자열 양식 패턴" var="변수 설정"
groupingUsed="구분 기호 사용 여부" pattern="숫자 패턴" scope="영역" />
속성명 | 기능 |
---|
value | 출력할 숫자 |
type | 출력 양식. percent(퍼센트), currency(통화), number(일반 숫자, 기본값) |
var | 출력할 숫자를 변수에 저장. 사용시 즉시 출력되지 않고, 원하는 위치에 출력 |
groupingUsed | 세 자리마다 콤마를 출력할지 |
pattern | 출력할 숫자의 양식을 패턴으로 지정 |
scope | 변수를 저장할 영역을 지정 |
<fmt:parseNumber value="파싱할 문자열" type="출력 양식" var="변수 설정"
integerOnly="정수만 파싱" pattern="패턴" scope="영역" />
속성명 | 기능 |
---|
value | 변환할 문자열 |
type | 문자열의 타입을 설정. 기본값은 number |
var | 출력할 값을 변수에 저장 |
pattern | 문자열의 양식을 패턴으로 지정 |
scope | 변수를 저장할 영역을 지정 |
integerOnly | 정수 부분만 표시할지 결정. 기본값은 false |
Fmt1.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<html>
<head><title>JSTL - fmt 1</title></head>
<body>
<h4>숫자 포맷 설정</h4>
<c:set var="number1" value="12345" />
콤마 O : <fmt:formatNumber value="${ number1 }" /><br />
콤마 X : <fmt:formatNumber value="${ number1 }" groupingUsed="false" /><br />
<fmt:formatNumber value="${number1 }" type="currency" var="printNum1" />
통화기호 : ${ printNum1 } <br />
<fmt:formatNumber value="0.03" type="percent" var="printNum2" />
퍼센트 : ${ printNum2 }
<h4>문자열을 숫자로 변경</h4>
<c:set var="number2" value="6,789.01" />
<fmt:parseNumber value="${ number2 }" pattern="00,000.00" var="printNum3" />
소수점까지 : ${ printNum3 } <br />
<fmt:parseNumber value="${ number2 }" integerOnly="true" var="printNum4" />
정수 부분만 : ${ printNum4 }
</body>
</html>
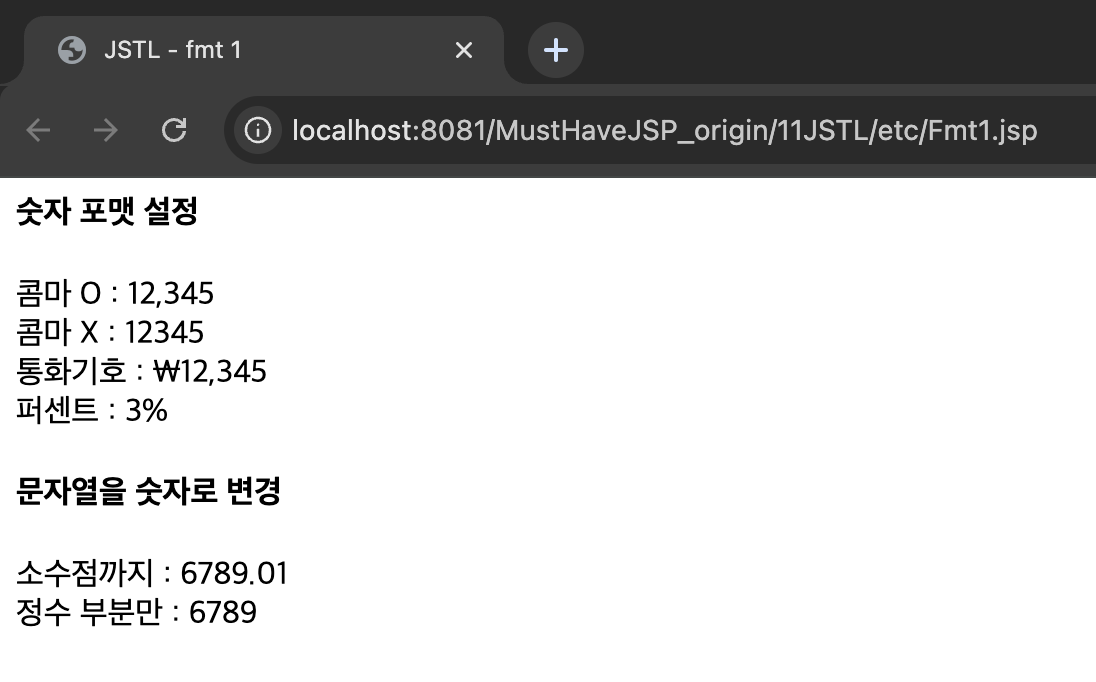
11.4.2 날짜 포맷 및 타임존
<fmt:formatDate value="출력할 날짜" type="출력 양식" var="변수 설정"
dateStyle="날짜 스타일" timeStyle="시간 스타일" pattern="날짜 패턴" scope="영역"
/>
속성명 | 기능 |
---|
value | 출력할 값 |
type | 출력시 날짜(date), 시간(time), 날짜 및 시간(both) 세가지 중 선택 |
var | 출력할 숫자를 변수에 저장 |
dateStyle | 날짜 스타일을 지정. default, short, medium, long, full |
timeStyle | 시간 스타일을 지정. default, short, medium, long, full |
pattern | 출력할 날짜 및 시간의 양식을 패턴으로 지정 |
scope | 변수를 저장할 영역을 지정 |
Fmt2.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<html>
<head><title>JSTL - fmt 2</title></head>
<body>
<c:set var="today" value="<%= new java.util.Date() %>" />
<h4>날짜 포맷</h4>
full : <fmt:formatDate value="${ today }" type="date" dateStyle="full"/> <br />
short : <fmt:formatDate value="${ today }" type="date" dateStyle="short"/> <br />
long : <fmt:formatDate value="${ today }" type="date" dateStyle="long"/> <br />
default : <fmt:formatDate value="${ today }" type="date" dateStyle="default"/>
<h4>시간 포맷</h4>
full : <fmt:formatDate value="${ today }" type="time" timeStyle="full"/> <br />
short : <fmt:formatDate value="${ today }" type="time" timeStyle="short"/> <br />
long : <fmt:formatDate value="${ today }" type="time" timeStyle="long"/> <br />
default : <fmt:formatDate value="${ today }" type="time" timeStyle="default"/>
<h4>날짜/시간 표시</h4>
<fmt:formatDate value="${ today }" type="both" dateStyle="full" timeStyle="full"/>
<br />
<fmt:formatDate value="${ today }" type="both" pattern="yyyy-MM-dd hh:mm:ss"/>
<h4>타임존 설정</h4>
<fmt:timeZone value="GMT">
<fmt:formatDate value="${ today }" type="both" dateStyle="full" timeStyle="full"/>
<br />
</fmt:timeZone>
<fmt:timeZone value="America/Chicago">
<fmt:formatDate value="${ today }" type="both" dateStyle="full" timeStyle="full"/>
</fmt:timeZone>
</body>
</html>
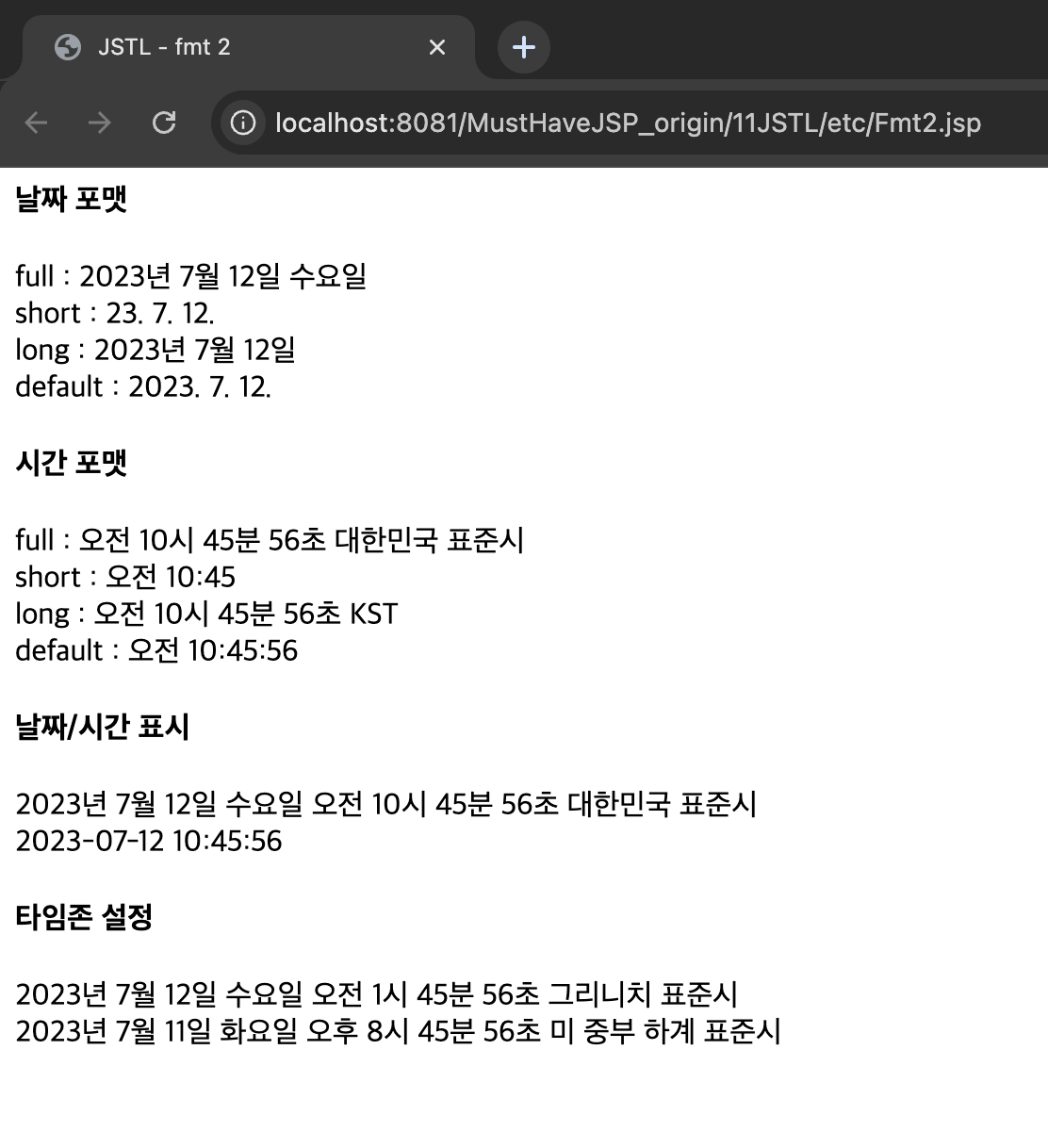
11.4.3 로케일 설정
Fmt3.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<html>
<head><title>JSTL - fmt 3</title></head>
<body>
<h4>로케일 설정</h4>
<c:set var="today" value="<%= new java.util.Date() %>"/>
한글로 설정 : <fmt:setLocale value="ko_kr" />
<fmt:formatNumber value="10000" type="currency" /> /
<fmt:formatDate value="${ today }" /><br />
일어로 설정 : <fmt:setLocale value="ja_JP" />
<fmt:formatNumber value="10000" type="currency" /> /
<fmt:formatDate value="${ today }" /><br />
영어로 설정 : <fmt:setLocale value="en_US" />
<fmt:formatNumber value="10000" type="currency" /> /
<fmt:formatDate value="${ today }" /><br />
</body>
</html>
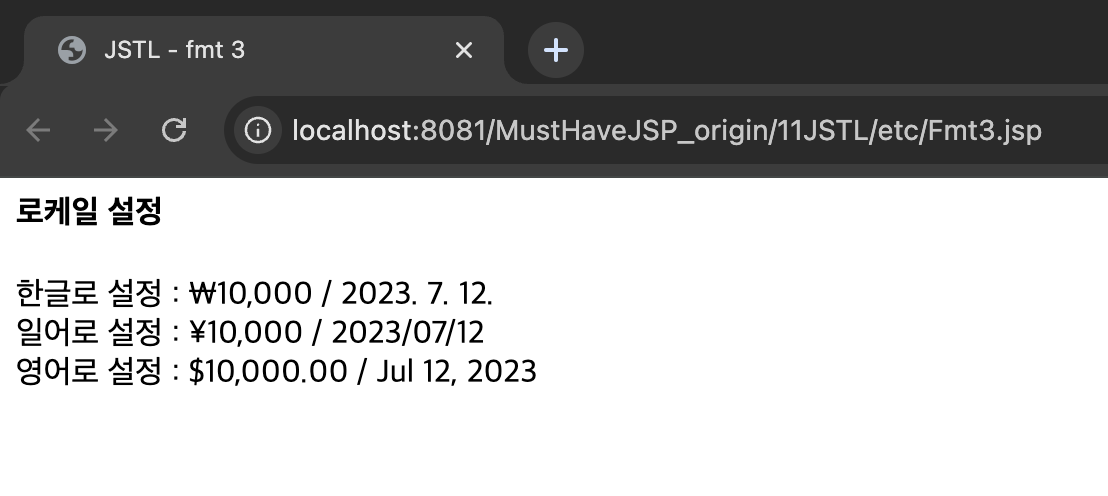
11.5 XML 태그
태그명 | 기능 |
---|
out | select 속성에 지정한 XPath 표현식의 결과를 출력 |
parse | XML을 파싱할 때 사용 |
forEach | select 속성에 지정한 반복되는 노드를 파싱 |
if | select 속성에 지정한 xPath 표현식의 값을 하나의 조건으로 결정 |
choose | select 속성에 지정한 xPath 표현식의 값을 다중 조건으로 결정 |
BookList.xml
<?xml version="1.0" encoding="UTF-8"?>
<booklist>
<book>
<name>사피엔스</name>
<author>유발 하라리</author>
<price>19800</price>
</book>
<book>
<name>총,균,쇠</name>
<author>제러드 다이아몬드</author>
<price>25200</price>
</book>
</booklist>
Xml.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<%@ taglib prefix="x" uri="http://java.sun.com/jsp/jstl/xml"%>
<html>
<head><title>JSTL - xml</title></head>
<body>
<c:import url="../inc/BookList.xml" var="booklist" charEncoding="UTF-8"/>
<x:parse xml="${booklist}" var="blist" />
<h4>파싱 1</h4>
제목 : <x:out select="$blist/booklist/book[1]/name" /> <br />
저자 : <x:out select="$blist/booklist/book[1]/author" /> <br />
가격 : <x:out select="$blist/booklist/book[1]/price" /> <br />
<h4>파싱 2</h4>
<table border="1">
<tr>
<th>제목</th><th>저자</th><th>가격</th>
</tr>
<x:forEach select="$blist/booklist/book" var="item">
<tr>
<td><x:out select="$item/name" /></td>
<td><x:out select="$item/author" /></td>
<td>
<x:choose>
<x:when select="$item/price >= 20000">
2만 원 이상 <br />
</x:when>
<x:otherwise>
2만 원 미만 <br />
</x:otherwise>
</x:choose>
</td>
</tr>
</x:forEach>
</table>
<h4>파싱 3</h4>
<table border="1">
<x:forEach select="$blist/booklist/book" var="item">
<tr>
<td><x:out select="$item/name" /></td>
<td><x:out select="$item/author" /></td>
<td><x:out select="$item/price" /></td>
<td><x:if select="$item/name = '총,균,쇠'">구매함</x:if></td>
</tr>
</x:forEach>
</table>
</body>
</html>
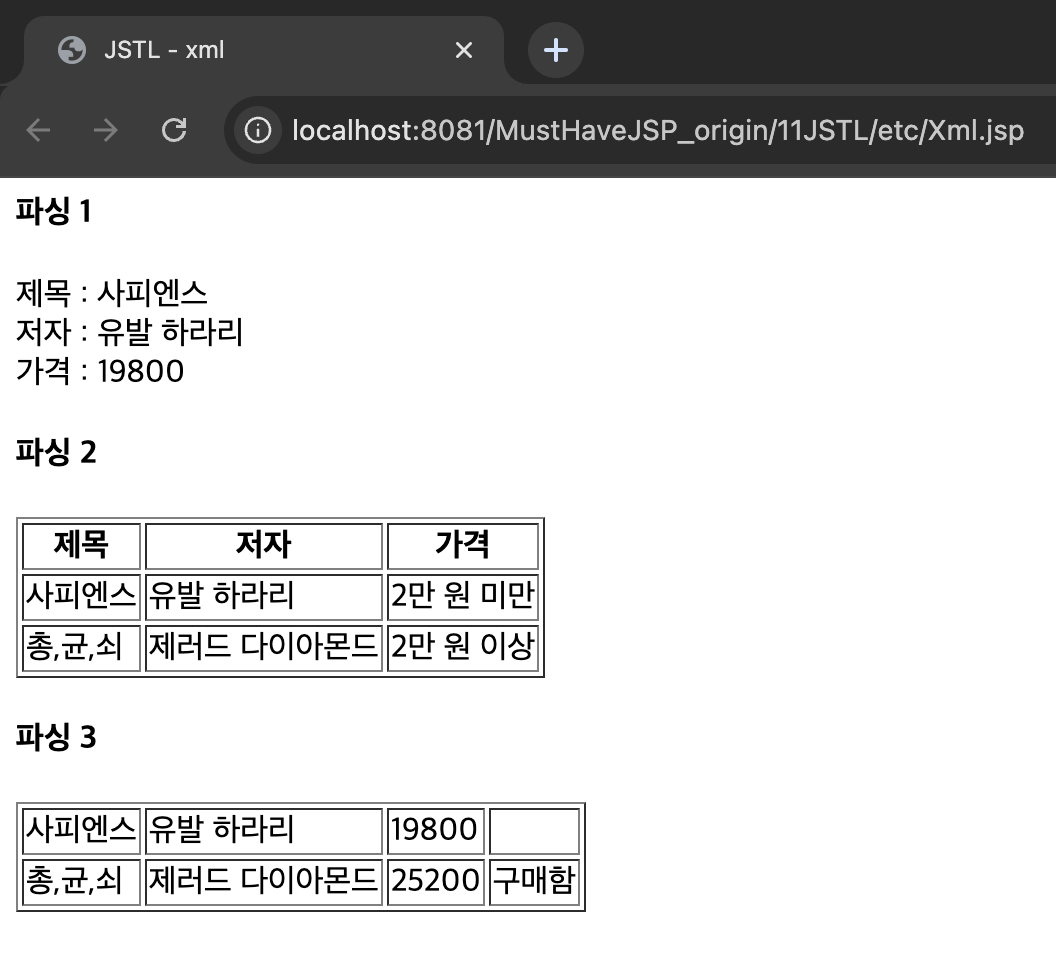