1. 로그인 창 UI 제작
- DB는 회사거를 일단 사용해서 만들지 뭘 사용할지 권고사직 통보받은 날에는 머리가 안돌아가서 일단 로그인 UI랑 대략적인 기능만 만들어보자!
01. Login
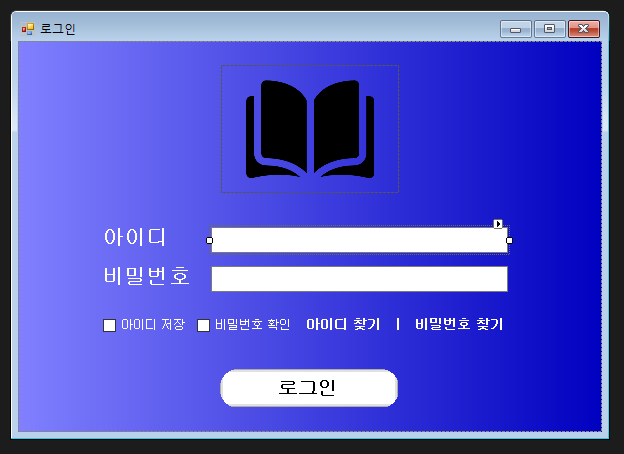
-1. Controls
- PictureBox
- 책 모양 아이콘은 무료 아이콘 받아왔습니다.
- 배경은 transparant로 투명하게 해주었구요!
- Label
- 아이디, 비밀번호 Text, 아이디 찾기, 비밀번호 찾기 Label
- 클릭하면 해당 textbox에 focusing
- 아이디 찾기와 비밀번호 찾기는 cursor을 pointer로 해주고 클릭 시 FindById Form과 FindByPw Form을 보여준다
- TextBox
- maxLength를 20까지로 제한했다 그런데 생각해보니 안해도 될것 같다
- CheckBox
- 아이디 저장은 따로 이벤트가 없다 로그인 버튼 시에 체크가 되어있는지 확인하는 버튼 이벤트에 종속된다
- 비밀번호 확인은 체크가 될 시 입력한 글이 보이고 체크 해제시 '*'로 보인다
- Button
- 로그인 버튼으로 TextBox에 값이 입력되어 있는지 아이디 저장을 해야되는지 등을 체크하고 Pass하면 Main Form을 보여준다
-2. Code
using System;
using System.Windows.Forms;
namespace BookManagementProgram.LOGIN
{
public partial class Login : Form
{
public Login()
{
InitializeComponent();
this.StartPosition = FormStartPosition.CenterScreen;
if(Properties.Settings.Default.isLoginIdSave)
{
id_txt.Text = Properties.Settings.Default.LoginId;
idSaveCheck_chk.Checked = Properties.Settings.Default.isLoginIdSave;
}
}
// TextBox Focussing
private void label1_Click(object sender, EventArgs e)
{
id_txt.Focus();
}
private void label2_Click(object sender, EventArgs e)
{
pw_txt.Focus();
}
// 비밀번호 보이기 체크박스
private void showPassword_chk_CheckedChanged(object sender, EventArgs e)
{
if (showPassword_chk.Checked)
pw_txt.PasswordChar = default(char);
else
pw_txt.PasswordChar = '*';
}
// 아이디 저장 체크박스
private static void NotSaveID()
{
Properties.Settings.Default.isLoginIdSave = false;
Properties.Settings.Default.Save();
}
private void SaveID()
{
Properties.Settings.Default.LoginId = id_txt.Text;
Properties.Settings.Default.isLoginIdSave = true;
Properties.Settings.Default.Save();
}
// 아이디 찾기 라벨
private void findId_lbl_Click(object sender, EventArgs e)
{
(new FindById()).ShowDialog();
}
// 비밀번호 찾기 라벨
private void findPassword_lbl_Click(object sender, EventArgs e)
{
(new FindByPassword()).ShowDialog();
}
// 로그인 버튼
private void login_btn_Click(object sender, EventArgs e)
{
if (string.IsNullOrEmpty(id_txt.Text))
{
MessageBox.Show("아이디를 입력해주세요.");
id_txt.Focus();
return;
}
if (string.IsNullOrEmpty(pw_txt.Text))
{
MessageBox.Show("비밀번호를 입력해주세요.");
pw_txt.Focus();
return;
}
if (idSaveCheck_chk.Checked)
{
SaveID();
}
else
{
NotSaveID();
}
Main main = new Main();
main.Show();
main.BringToFront();
this.Hide();
}
}
}
02. FindById
- UI가 확연히 차이가 나는데 어쩔 수 없다, 나는 그렇게까지 디자인 감각이 있는 편이 아니니...
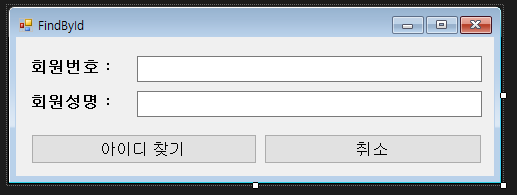
-1. Controls
- Label
- 회원번호, 회원 성명 Label이며 클릭시 해당 TextBox에 Focusing 된다
- TextBox
- Button
- 아이디 찾기를 누르면 TextBox에 값이 있는지 확인하고 아이디를 조회할 예정이다
- 취소는 창을 닫아준다
-2. Code
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace BookManagementProgram.LOGIN
{
public partial class FindById : Form
{
public FindById()
{
InitializeComponent();
this.StartPosition = FormStartPosition.CenterParent;
}
private void cancel_btn_Click(object sender, EventArgs e)
{
this.Close();
}
private void find_btn_Click(object sender, EventArgs e)
{
string memberNo = memberNumber_txt.Text;
string memberName = memberName_txt.Text;
if(string.IsNullOrEmpty(memberNo))
{
MessageBox.Show("회원 번호를 입력해주세요.");
memberNumber_txt.Focus();
return;
}
if(string.IsNullOrEmpty(memberName))
{
MessageBox.Show("회원 성명를 입력해주세요.");
memberName_txt.Focus();
return;
}
}
private void label1_Click(object sender, EventArgs e)
{
memberNumber_txt.Focus();
}
private void label2_Click(object sender, EventArgs e)
{
memberName_txt.Focus();
}
}
}
03. FindByPassword
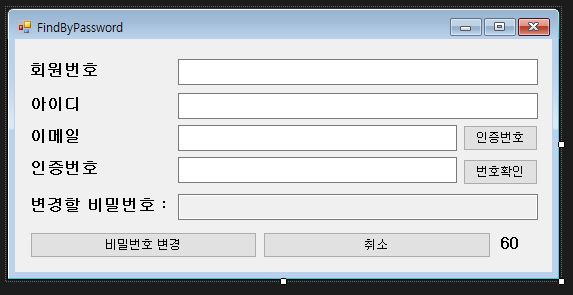
-1. Controls
- Label
- 지금까지와 동일하게 선택시 해당 TextBox로 Focusing
- TextBox
- 변경할비밀번호에 해당하는 TextBox는 read-only를 true로 초기화해주고 번호확인 버튼을 눌러서 인증번호 확인이 되면 false로 바꿔준다
- Button
- 인증번호 버튼
- 회원 번호, 아이디, 이메일에 값이 들어있는지 확인
- 이메일이 이메일 형식에 적합한지 정규표현식으로 체크
- 전부 Pass하면 Gmail Api를 통해서 해당 이메일에 인증번호 발생
- Timer Control을 초기화하고 시작하여 하단 우측에 있는 숫자 Label에 카운트 다운을 해준다
- 번호확인
- 인증번호를 보낼 때 생성된 인증번호와 입력한 인증번호가 맞는지 확인 맞으면 변경할 비밀번호 textbox read-only를 false로 바꿔준다
- 비밀번호 변경
- 전체 데이터 확인 후 비밀번호 변경 프로시저 사용 예정
- 취소
-2. Code
using System;
using System.Data;
using System.Linq;
using System.Net.Mail;
using System.Text.RegularExpressions;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace BookManagementProgram.LOGIN
{
public partial class FindByPassword : Form
{
private int _duration = 0;
private string _auth_code = "";
public FindByPassword()
{
InitializeComponent();
this.StartPosition = FormStartPosition.CenterParent;
}
// 인증번호 요청 버튼
private void authenticationRequest_btn_Click(object sender, EventArgs e)
{
if(CheckTextBox())
{
string email = memberEmail_txt.Text;
if(CheckEmailPattern(email))
{
GmailRun(email);
}
}
}
// 인증번호 확인 버튼
private void checkAuthentication_btn_Click(object sender, EventArgs e)
{
if (authenticationNumber_txt.Text.Trim().Equals(_auth_code))
{
MessageBox.Show("인증 되었습니다");
ResetTimerAndCode();
newPassword_txt.ReadOnly = false;
newPassword_txt.Focus();
}
else
{
MessageBox.Show("다시 확인해주세요");
}
}
// 비밀번호 변경 버튼
private void changePassword_btn_Click(object sender, EventArgs e)
{
if(CheckTextBox())
{
if(newPassword_txt.Text.Length > 0)
{
MessageBox.Show("변경 완료");
}
}
}
// 취소
private void cancel_btn_Click(object sender, EventArgs e)
{
this.Close();
}
private bool CheckTextBox()
{
if(string.IsNullOrEmpty(memberNumber_txt.Text))
{
MessageBox.Show("회원의 성함을 입력해주세요.");
memberNumber_txt.Focus();
return false;
}
if (string.IsNullOrEmpty(memberID_txt.Text))
{
MessageBox.Show("회원의 아이디를 입력해주세요.");
memberID_txt.Focus();
return false;
}
if (string.IsNullOrEmpty(memberEmail_txt.Text))
{
MessageBox.Show("회원의 이메일을 입력해주세요.");
memberEmail_txt.Focus();
return false;
}
return true;
}
private bool CheckEmailPattern(string email)
{
string pattern = @"[a-zA-Z0-9!#$%&'*+/=?^_`{|}~-]+(?:\.[a-zA-Z0-9!#$%&'*+/=?^_`{|}~-]+)*@(?:[a-zA-Z0-9](?:[a-zA-Z0-9-]*[a-zA-Z0-9])?\.)+[a-zA-Z0-9](?:[a-zA-Z0-9-]*[a-zA-Z0-9])?";
if(Regex.IsMatch(email, pattern))
{
return true;
}
else
{
MessageBox.Show("이메일을 확인해주세요.");
memberEmail_txt.Focus();
return false;
}
}
// 랜덤 코드 생성
private string getRandomCode()
{
Random rand = new Random();
string input = "abcdefghijklmnopqrstuvxyz0123456789";
var chars = Enumerable.Range(0, 6).Select(x => input[rand.Next(0, input.Length)]);
return new string(chars.ToArray());
}
// 타이머 카운트 다운
private void CountDown(object sender, EventArgs e)
{
if (_duration == 0 || _duration < 0)
{
ResetTimerAndCode();
MessageBox.Show("인증 시간이 초과되었습니다.");
return;
}
_duration--;
timer_lbl.Text = _duration.ToString();
}
// 타이머 및 코드 초기화
private void ResetTimerAndCode()
{
timer1.Stop();
timer1.Dispose();
_auth_code = "";
}
// gmail
private async void GmailRun(string email)
{
// 타이머 초기화 및 인증번호 생성
_duration = 60;
_auth_code = getRandomCode();
timer1.Stop();
timer1 = new Timer();
timer1.Tick += new EventHandler(CountDown);
timer1.Interval = 1000;
timer1.Start();
await Task.Run(() => sendGmailAsync(email));
//auth_btn.Enabled = true;
}
private void sendGmailAsync(string email)
{
MailMessage mailMessage = new MailMessage();
mailMessage.From = new MailAddress(Properties.Settings.Default.GmailID);
mailMessage.To.Add(email);
mailMessage.Subject = "인증코드";
mailMessage.Body = $"인증 코드 : {_auth_code}";
SmtpClient SmtpServer = new SmtpClient("smtp.gmail.com");
SmtpServer.Port = 587;
SmtpServer.EnableSsl = true;
SmtpServer.UseDefaultCredentials = false;
SmtpServer.DeliveryMethod = System.Net.Mail.SmtpDeliveryMethod.Network;
SmtpServer.Credentials = new System.Net.NetworkCredential(Properties.Settings.Default.GmailID, Properties.Settings.Default.GmailPW);
SmtpServer.Send(mailMessage);
}
private void label1_Click(object sender, EventArgs e)
{
memberNumber_txt.Focus();
}
private void label2_Click(object sender, EventArgs e)
{
memberID_txt.Focus();
}
private void label3_Click(object sender, EventArgs e)
{
memberEmail_txt.Focus();
}
private void label4_Click(object sender, EventArgs e)
{
authenticationNumber_txt.Focus();
}
private void label5_Click(object sender, EventArgs e)
{
newPassword_txt.Focus();
}
}
}