1. Project Test
- spring initalizr / swagger / web, lombok / swagger은 maven repository에서 따로 확인하여 설치
01. Swagger 설치
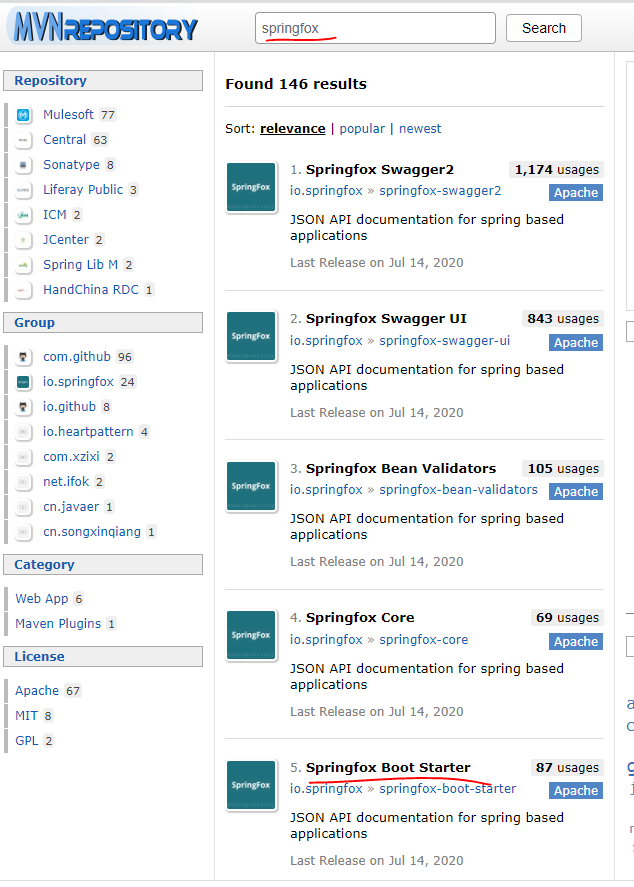
// https://mvnrepository.com/artifact/io.springfox/springfox-boot-starter
implementation group: 'io.springfox', name: 'springfox-boot-starter', version: '3.0.0'
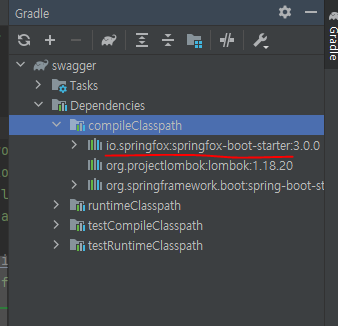
02. Talent API 대신 Swagger UI 사용
-1. 일단 간단 테스트
- Package : controller
- Class : ApiController
- controller / ApiController.java
package com.example.swagger.controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/api")
public class ApiController {
@GetMapping("/hello")
public String hello() {
return "hello";
}
}
-2. Swagger UI의 api controller 수정해보기
_1. Class의 리소스 변경
- ApiController.java
- @Api(tags = {"API 정보를 제공하는 Controller"})
- @Api, 클래스를 스웨거의 리소스로 표시
- tags를 통해서 리소스 명 지정
package com.example.swagger.controller;
import io.swagger.annotations.Api;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
// @Api, 클래스를 스웨거의 리소스로 표시
@Api(tags = {"API 정보를 제공하는 Controller"})
@RestController
@RequestMapping("/api")
public class ApiController {
@GetMapping("/hello")
public String hello() {
return "hello";
}
}
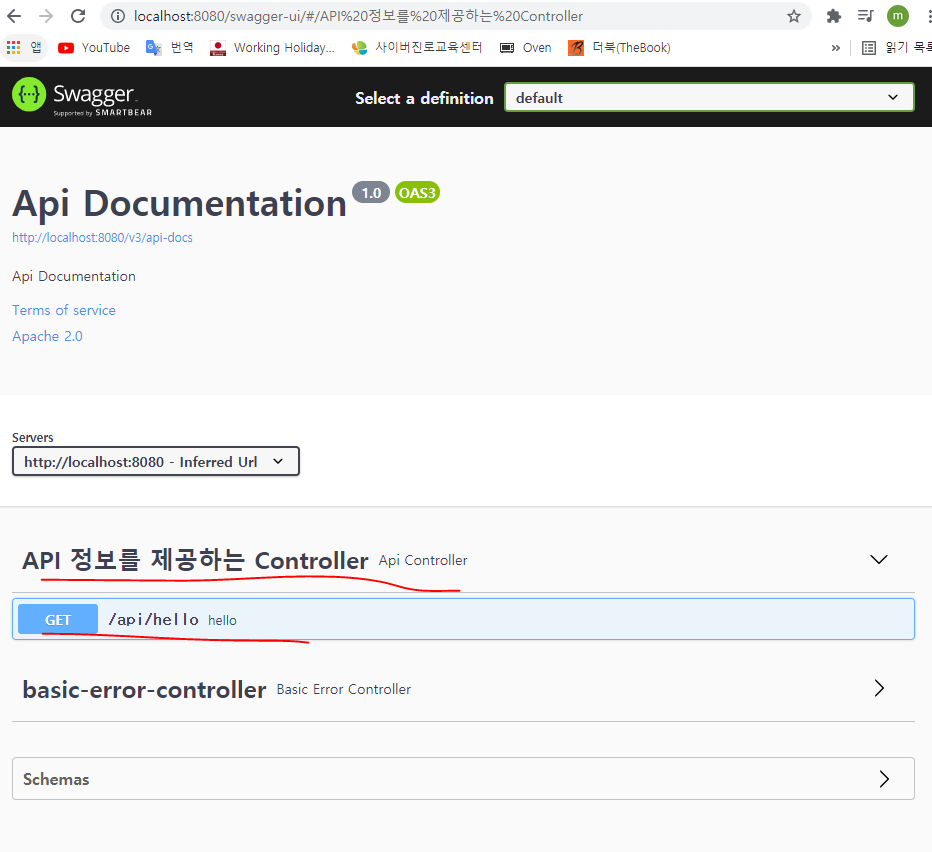
_2. 오퍼레이션(Method) Parameter 메타 데이터 설정
- ApiController.java
- @ApiParam(value = "x값")
- @ApiParam은 오퍼레이션 파라미터의 메타 데이터 지정
- value로 지정
package com.example.swagger.controller;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiParam;
import org.springframework.web.bind.annotation.*;
@Api(tags = {"API 정보를 제공하는 Controller"})
@RestController
@RequestMapping("/api")
public class ApiController {
@GetMapping("/hello")
public String hello() {
return "hello";
}
@GetMapping("/plus/{x}")
public int plus(
@ApiParam(value = "x값")
@PathVariable int x,
@ApiParam(value = "y값")
@RequestParam int y
) {
return x + y;
}
}
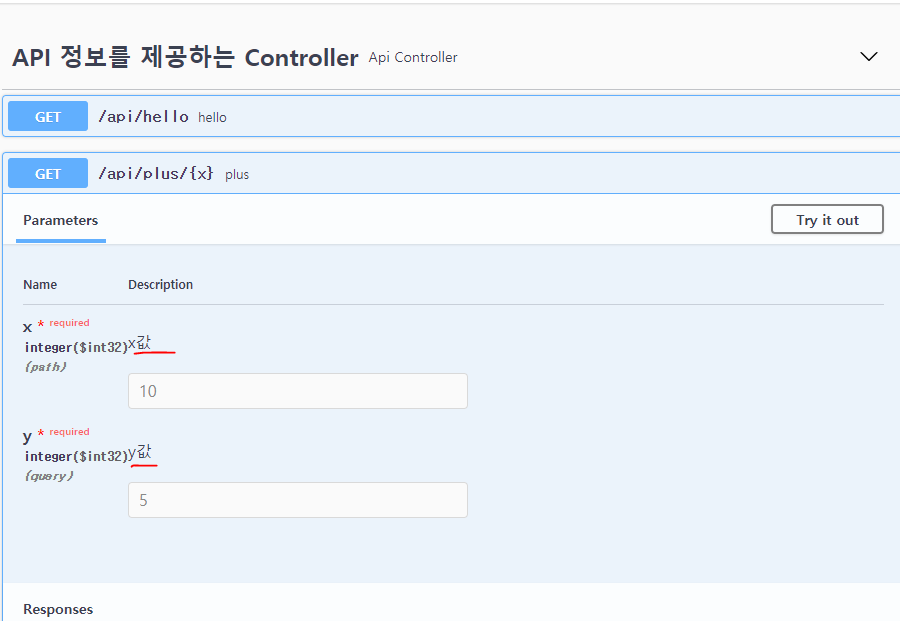
_3. Model의 속성 데이터 설정
- Package : dto
- Class : UserReq, UserRes
- dto / UserReq.java
- @ApiModelProperty(value = "사용자의 이름", example = "steve", required = true)
package com.example.swagger.dto;
import io.swagger.annotations.ApiModelProperty;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
@Data
@NoArgsConstructor
@AllArgsConstructor
public class UserReq {
@ApiModelProperty(value = "사용자의 이름", example = "steve", required = true)
private String name;
@ApiModelProperty(value = "사용자의 나이", example = "20", required = true)
private int age;
}
package com.example.swagger.dto;
import io.swagger.annotations.ApiModelProperty;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
@Data
@NoArgsConstructor
@AllArgsConstructor
public class UserRes {
@ApiModelProperty(value = "사용자의 이름", example = "steve", required = true)
private String name;
@ApiModelProperty(value = "사용자의 나이", example = "20", required = true)
private int age;
}
- controller / ApiController.java
package com.example.swagger.controller;
import com.example.swagger.dto.UserReq;
import com.example.swagger.dto.UserRes;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiParam;
import org.springframework.web.bind.annotation.*;
@Api(tags = {"API 정보를 제공하는 Controller"})
@RestController
@RequestMapping("/api")
public class ApiController {
@GetMapping("/hello")
public String hello() {
return "hello";
}
@GetMapping("/plus/{x}")
public int plus(
@ApiParam(value = "x값")
@PathVariable int x,
@ApiParam(value = "y값")
@RequestParam int y
) {
return x + y;
}
@GetMapping("/user")
public UserRes user(UserReq userReq) {
return new UserRes(userReq.getName(), userReq.getAge());
}
}
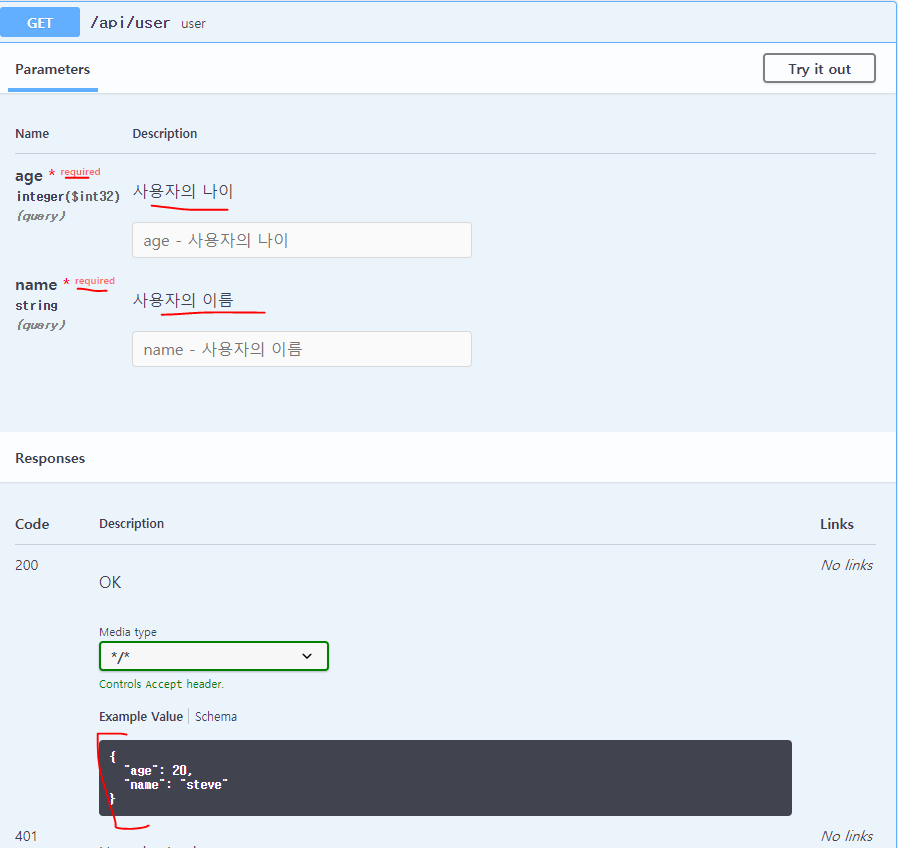
_4. 특정 경로의 오퍼레이션 HTTP 메소드 설명 추가
- controller / ApiController.java
- @ApiOperation(value = "사용자의 이름과 나이를 리턴하는 메소드")
- 특정 경로의 오퍼레이션 HTTP 메소드 설명 추가
package com.example.swagger.controller;
import com.example.swagger.dto.UserReq;
import com.example.swagger.dto.UserRes;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiParam;
import org.springframework.web.bind.annotation.*;
@Api(tags = {"API 정보를 제공하는 Controller"})
@RestController
@RequestMapping("/api")
public class ApiController {
@GetMapping("/hello")
public String hello() {
return "hello";
}
@GetMapping("/plus/{x}")
public int plus(
@ApiParam(value = "x값")
@PathVariable int x,
@ApiParam(value = "y값")
@RequestParam int y
) {
return x + y;
}
@ApiOperation(value = "사용자의 이름과 나이를 리턴하는 메소드")
@GetMapping("/user")
public UserRes user(UserReq userReq) {
return new UserRes(userReq.getName(), userReq.getAge());
}
}
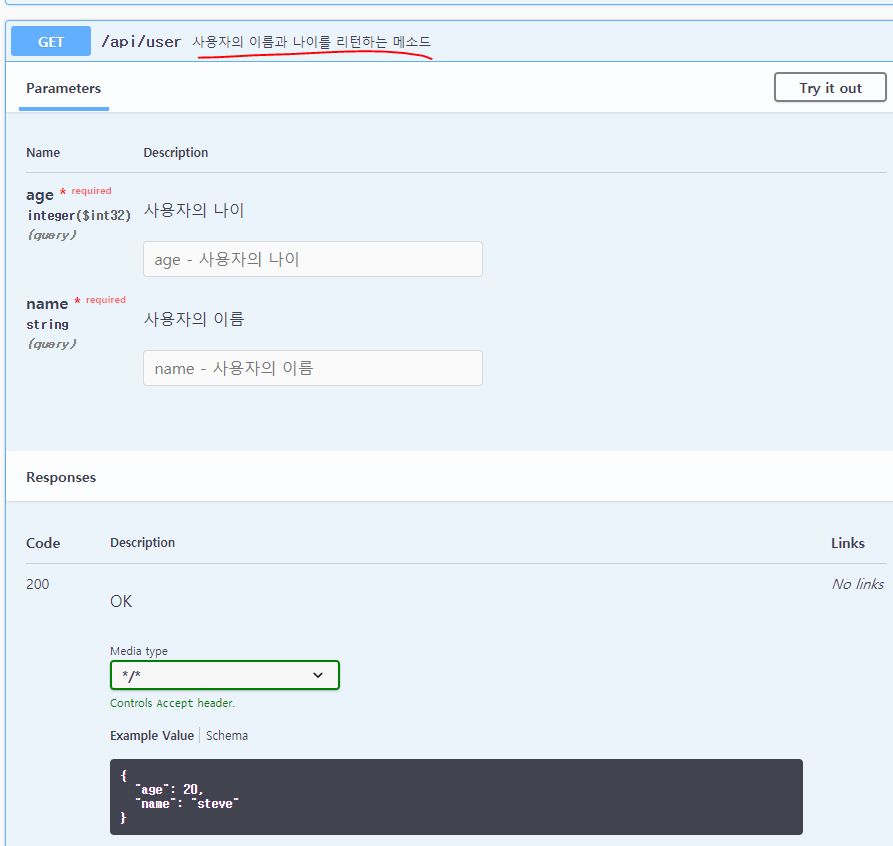
_5. 오퍼레이션 응답지정(code, error 등)
- controller / ApiController.java
- @ApiResponse(code = 502, message = "사용자의 나이가 10살 이하일때")
- 오퍼레이션 응답지정(code, error 등)
package com.example.swagger.controller;
import com.example.swagger.dto.UserReq;
import com.example.swagger.dto.UserRes;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiParam;
import io.swagger.annotations.ApiResponse;
import org.springframework.web.bind.annotation.*;
@Api(tags = {"API 정보를 제공하는 Controller"})
@RestController
@RequestMapping("/api")
public class ApiController {
@GetMapping("/hello")
public String hello() {
return "hello";
}
@GetMapping("/plus/{x}")
public int plus(
@ApiParam(value = "x값")
@PathVariable int x,
@ApiParam(value = "y값")
@RequestParam int y
) {
return x + y;
}
@ApiResponse(code = 502, message = "사용자의 나이가 10살 이하일때")
@ApiOperation(value = "사용자의 이름과 나이를 리턴하는 메소드")
@GetMapping("/user")
public UserRes user(UserReq userReq) {
return new UserRes(userReq.getName(), userReq.getAge());
}
}
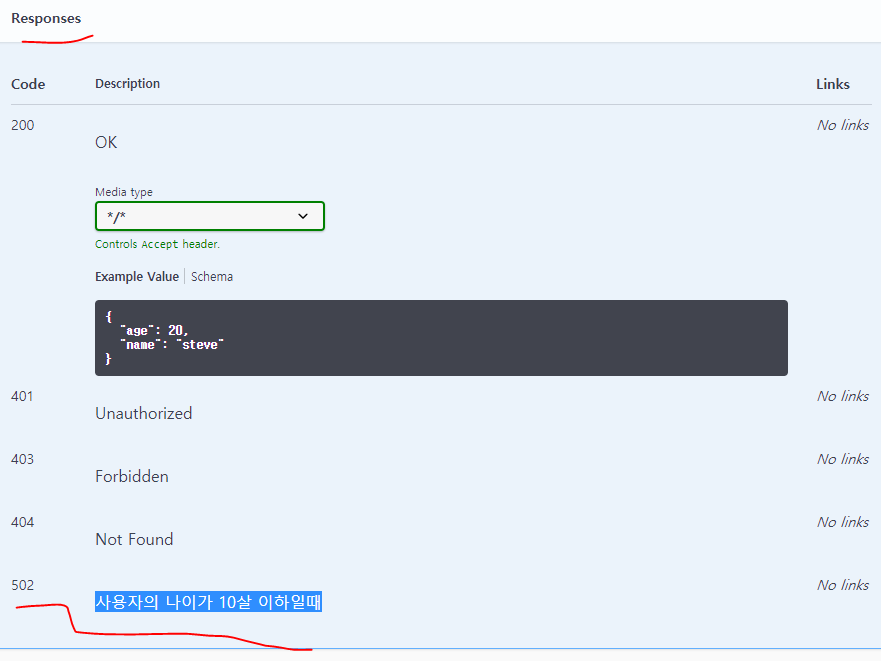
_6. POST 비교
- controller / ApiController.java
- 모델 설정을 그대로 갖고 오는 점을 보와 위와 동일하게 사용
package com.example.swagger.controller;
import com.example.swagger.dto.UserReq;
import com.example.swagger.dto.UserRes;
import io.swagger.annotations.*;
import org.springframework.web.bind.annotation.*;
@Api(tags = {"API 정보를 제공하는 Controller"})
@RestController
@RequestMapping("/api")
public class ApiController {
@GetMapping("/hello")
public String hello() {
return "hello";
}
@ApiImplicitParams({
@ApiImplicitParam(name = "x", value = "x 값", required = true, dataType = "int", paramType = "path"),
@ApiImplicitParam(name = "y", value = "y 값", required = true, dataType = "int", paramType = "query")
})
@GetMapping("/plus/{x}")
public int plus(@PathVariable int x,@RequestParam int y) {
return x + y;
}
@ApiResponse(code = 502, message = "사용자의 나이가 10살 이하일때")
@ApiOperation(value = "사용자의 이름과 나이를 리턴하는 메소드")
@GetMapping("/user")
public UserRes user(UserReq userReq) {
return new UserRes(userReq.getName(), userReq.getAge());
}
@PostMapping("/user")
public UserRes userPost(@RequestBody UserReq userReq) {
return new UserRes(userReq.getName(), userReq.getAge());
}
}
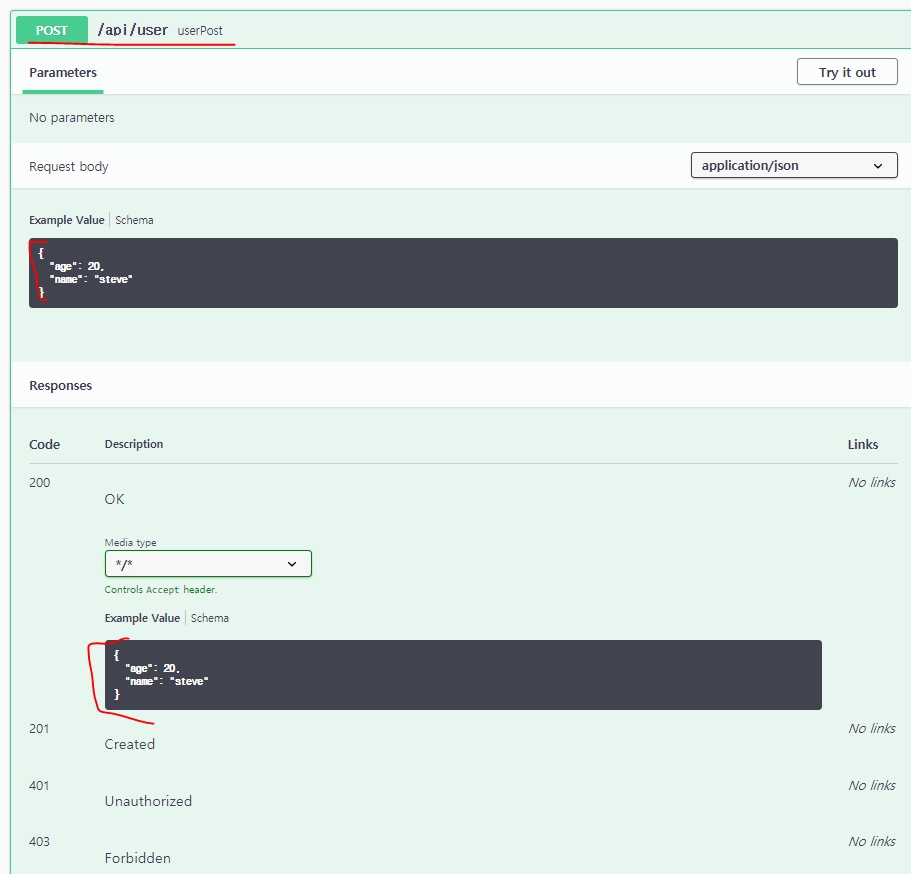
_7. @ApiParam을 @ApiImplicitParams으로 변경
- controller / ApiController.java
- @ApiImplicitParams & @ApiImplicitParam
package com.example.swagger.controller;
import com.example.swagger.dto.UserReq;
import com.example.swagger.dto.UserRes;
import io.swagger.annotations.*;
import org.springframework.web.bind.annotation.*;
@Api(tags = {"API 정보를 제공하는 Controller"})
@RestController
@RequestMapping("/api")
public class ApiController {
@GetMapping("/hello")
public String hello() {
return "hello";
}
@ApiImplicitParams({
@ApiImplicitParam(name = "x", value = "x 값", required = true, dataType = "int", paramType = "path"),
@ApiImplicitParam(name = "y", value = "y 값", required = true, dataType = "int", paramType = "query")
})
@GetMapping("/plus/{x}")
public int plus(@PathVariable int x,@RequestParam int y) {
return x + y;
}
@ApiResponse(code = 502, message = "사용자의 나이가 10살 이하일때")
@ApiOperation(value = "사용자의 이름과 나이를 리턴하는 메소드")
@GetMapping("/user")
public UserRes user(UserReq userReq) {
return new UserRes(userReq.getName(), userReq.getAge());
}
@PostMapping("/user")
public UserRes userPost(@RequestBody UserReq userReq) {
return new UserRes(userReq.getName(), userReq.getAge());
}
}