sort()
메서드는 배열을 알파벳 순으로 정렬한다.
reverse()
메서드는 배열의 요소 순서를 뒤집는다. 내림차순으로 배열을 정렬하는데 사용할 수 있다.
기본적으로 sort()
함수는 값을 문자열로 정렬한다. 'Apple', 'Banana'등과 같이 문자열에 대해서는 잘 작동한다. 하지만 숫자를 문자열로 정렬하면 '2'가 '1'보다 크므로 '25'는 '100'보다 크다고 인식한다.
이 문제로 sort()
메서드는 숫자를 정렬할 때 잘못된 결과를 생성한다.
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Array Sort</h2>
<p>Sort the array in ascending order:</p>
<p id="demo1"></p>
<p id="demo2"></p>
<script>
const points = [40, 100, 1, 5, 25, 10];
document.getElementById("demo1").innerHTML = points;
points.sort(function(a, b){return a - b});
document.getElementById("demo2").innerHTML = points;
</script>
</body>
</html>
동일한 트릭을 사용하여 배열을 내림차순으로 정렬한다.
비교 기능의 목적은 정렬 순서를 정의하는 것이다.
비교 함수는 인수에 따라 음수, 0 또는 양수 값을 반환해야 한다.
function(a, b){return a - b}
sort()
함수는 두 값을 비교할 때 값을 비교 함수로 보내고 반환된 값에 따라 값을 정렬한다.
음수
이면 a가 b앞에 정렬된다. 고로 if문으로 작성하여 return값을 -1
로 해도 된다.양수
이면 b가 a앞에 정렬된다. 고로 if문으로 작성하여 return값을 +1
로 해도 된다.0
이면 두값의 정렬 순서가 변경되지 않는다.비교 함수는 배열의 모든값을 한번에 두 값(a,b)으로 비교한다.
40과 100을 비교할 때 sort()
메서드는 비교 함수 (40,100)을 호출한다.
함수는 40-100 (a-b)
을 계산하고 결과가 -60
이므로 정렬 함수는 40을 100보다 작은 값으로 정렬한다. 이 코드를 사용하여 숫자 및 알파벳순서를 정렬시킬 수 있다.
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Array Sort</h2>
<p>Click the buttons to sort the array alphabetically or numerically.</p>
<button onclick="myFunction1()">Sort Alphabetically</button>
<button onclick="myFunction2()">Sort Numerically</button>
<p id="demo"></p>
<script>
const points = [40, 100, 1, 5, 25, 10];
document.getElementById("demo").innerHTML = points;
function myFunction1() {
points.sort();
document.getElementById("demo").innerHTML = points;
}
function myFunction2() {
points.sort(function(a, b){return a - b});
document.getElementById("demo").innerHTML = points;
}
</script>
</body>
</html>
버튼을 누를 때마다 정렬 순서가 랜덤으로 된다.
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Array Sort</h2>
<p>Click the button (again and again) to sort the array in random order.</p>
<button onclick="myFunction()">Try it</button>
<p id="demo"></p>
<script>
const points = [40, 100, 1, 5, 25, 10];
document.getElementById("demo").innerHTML = points;
function myFunction() {
points.sort(function(a, b){return 0.5 - Math.random()});
document.getElementById("demo").innerHTML = points;
}
</script>
</body>
</html>
위의 예시의 Array.sort()
는 정확하지 않으며 일부 숫자를 다른 숫자보다 선호한다.
가장 인기 있는 올바른 방법은 Fisher Yates
셔풀이 라고 하며 1938년에 데이터 과학데 도입되었다.
JS에서 메서드는 다음과 같이 번역될 수 있다.
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Array Sort</h2>
<h3>The Fisher Yates Method</h3>
<p>Click the button (again and again) to sort the array in random order.</p>
<button onclick="myFunction()">Try it</button>
<p id="demo"></p>
<script>
const points = [40, 100, 1, 5, 25, 10];
document.getElementById("demo").innerHTML = points;
function myFunction() {
for (let i = points.length -1; i > 0; i--) {
let j = Math.floor(Math.random() * i)
let k = points[i]
points[i] = points[j]
points[j] = k
}
document.getElementById("demo").innerHTML = points;
}
</script>
</body>
</html>
배열에서 최대값 또는 최소값을 찾기 위한 내장 함수는 없다.
그러나 배열을 정렬한 후에는 인덱스를 사용하여 가장 높은 값과 가낭 낮은 값을 얻을 수 있따.
올름차순 정렬 :
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Array Sort</h2>
<p>The lowest number is <span id="demo"></span>.</p>
<script>
const points = [40, 100, 1, 5, 25, 10];
points.sort(function(a, b){return a-b});
document.getElementById("demo").innerHTML = points[0];
</script>
</body>
</html>
내림차순 정렬 :
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Array Sort</h2>
<p>The highest number is <span id="demo"></span>.</p>
<script>
const points = [40, 100, 1, 5, 25, 10];
points.sort(function(a, b){return b-a});
document.getElementById("demo").innerHTML = points[0];
</script>
</body>
</html>
가장 높은값 혹은 낮은값을 찾으려는 경우 전체 배열을 정렬하는 것은 상당히 비효율 적인 방법이다.
Math.max.apply
를 사용하여 배열에서 가장 높은 숫자를 찾을 수 있다.
왜 myArrayMax()
함수를 따로 선언했을까? 테스트 해보면 바로 Math.max.apply()
를 사용해도 된다.
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Array Sort</h2>
<p>The highest number is <span id="demo"></span>.</p>
<script>
const points = [40, 100, 1, 5, 25, 10];
document.getElementById("demo").innerHTML = myArrayMax(points);
function myArrayMax(arr) {
return Math.max.apply(null, arr);
}
</script>
</body>
</html>
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Array Sort</h2>
<p>The highest number is <span id="demo"></span>.</p>
<script>
const points = [40, 100, 1, 5, 25, 10];
document.getElementById("demo").innerHTML = Math.max.apply(null, points)
</script>
</body>
</html>
Math.max.apply(null,[1,2,3])
은Math.max(1,2,3)
과 동일하다.
Math.min.apply
를 사용하여 배열에서 가장 낮은 숫자를 찾을 수 있다.
Math.mix.apply(null,[1,2,3])
은Math.mix(1,2,3)
과 동일하다.My Min / Max JavaScript Methods
가장 빠른 방법은
home made
를 사용하는 것이다. 즉, 이미 만들어진 함수를 호출 하는 것이아닌 함수를 새로 정의 하는 것이다.
아래 함수는 각 값을 비교하며 가장 높은 값을 찾는 과정을 반복한다.<!DOCTYPE html> <html> <body>
The highest number is .
``` 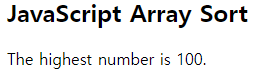 아래 함수는 각 값을 비교하며 가장 낮은 값을 찾는 과정을 반복한다. ```The lowest number is .
``` 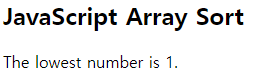 ## Sorting Object Arrays JS 배열에는 종종 다음과 같은 객체가 포함 된다. 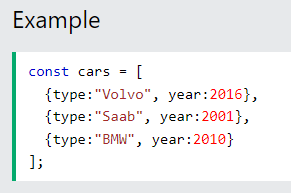 객체안에 다른 데이터 유형의 속성이 있더라도 `sort()` 메서드를 사용하여 배열을 정리할 수 있다. 방법은 속성 값을 비교하는 비교 함수를 정의 하는 것이다 : `car[]`라는 배열안의 data(item)는 `object`형식이다. ```Sort car objects on age:
``` 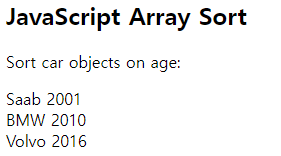문자열 속성을 비교하는 것은 더 복잡하다 :
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Array Sort</h2>
<p>Click the buttons to sort car objects on type.</p>
<button onclick="myFunction()">Sort</button>
<p id="demo"></p>
<script>
const cars = [
{type:"Volvo", year:2016},
{type:"Saab", year:2001},
{type:"BMW", year:2010}
];
displayCars();
function myFunction() {
cars.sort(function(a, b){
let x = a.type.toLowerCase();
let y = b.type.toLowerCase();
if (x < y) {return -1;}
if (x > y) {return 1;}
return 0;
});
displayCars();
}
function displayCars() {
document.getElementById("demo").innerHTML =
cars[0].type + " " + cars[0].year + "<br>" +
cars[1].type + " " + cars[1].year + "<br>" +
cars[2].type + " " + cars[2].year;
}
</script>
</body>
</html>
전체 Array에 대해 참고 하려면 아래 주소를 참조하자
(참조 : https://www.w3schools.com/jsref/jsref_obj_array.asp)