`const' 키워드는 아래 주소에서 소개하고있다.
let
으로 정의한 변수는 재 선언이 불가하다.
let
으로 정의한 변수는 재 할당이 불가하다.
let
으로 정의한 변수는 Block Scope 성질이다.
<html>
<body>
<h2>JavaScript const</h2>
<p id="demo"></p>
<script>
try {
const PI = 3.141592653589793;
PI = 3.14;
}
catch (err) {
document.getElementById("demo").innerHTML = err;
}
</script>
</body>
</html>
const
로 선언된 변수는 재 할당이 불가하다.const PI = 3.14159265359;
위와같이 선언과 동시에 값을 할당 시켜야 한다.
PI = 3.14159265359;
위와 같이 선언과 할당을 따로하여 사용 할 수 없다.
일반적으로 const
값이 변경될 것이라는 것을 모르는 경우에는 항상 const
로 선언하면 된다.
※아래와 같은 상황에서const
선언한다 :
상수 값
을 정의 하지 않는다, 다만 값에 대한 상수 참조
를 정의한다.
※위와 같은 이유로 아래와 같은 내용을 할 수 없다 :
※하지만 아래 내용은 가능하다 :
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript const</h2>
<p>Declaring a constant array does NOT make the elements unchangeble:</p>
<p id="demo"></p>
<script>
// Create an Array:
const cars = ["Saab", "Volvo", "BMW"];
// Change an element:
cars[0] = "Toyota";
// Add an element:
cars.push("Audi");
// Display the Array:
document.getElementById("demo").innerHTML = cars;
</script>
</body>
</html>
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript const</h2>
<p>You can NOT reassign a constant array:</p>
<p id="demo"></p>
<script>
try {
const cars = ["Saab", "Volvo", "BMW"];
cars = ["Toyota", "Volvo", "Audi"];
}
catch (err) {
document.getElementById("demo").innerHTML = err;
}
</script>
</body>
</html>
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript const</h2>
<p>Declaring a constant object does NOT make the objects properties unchangeable:</p>
<p id="demo"></p>
<script>
// Create an object:
const car = {type:"Fiat", model:"500", color:"white"};
// Change a property:
car.color = "red";
// Add a property:
car.owner = "Johnson";
// Display the property:
document.getElementById("demo").innerHTML = "Car owner is " + car.owner;
</script>
</body>
</html>
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript const</h2>
<p>You can NOT reassign a constant object:</p>
<p id="demo"></p>
<script>
try {
const car = {type:"Fiat", model:"500", color:"white"};
car = {type:"Volvo", model:"EX60", color:"red"};
}
catch (err) {
document.getElementById("demo").innerHTML = err;
}
</script>
</body>
</html>
const
키워드는 인터넷 익스플로러 11 또는 이전 버전에서 지원되지 않는다.
다음 표는 const
키워드 를 완벽하게 지원하는 첫 번째 브라우저 버전을 정의한다.
<!DOCTYPE html>
<html>
<body>
<h2>JavaScropt const variables has block scope</h2>
<p id="demo"></p>
<script>
const x = 10;
// Here x is 10
{
const x = 2;
// Here x is 2
}
// Here x is 10
document.getElementById("demo").innerHTML = "x is " + x;
</script>
</body>
</html>
const
로 변수를 선언하는 것은 블록 범위와 관련한 내용은 let
과 유사하다.
위의 예시에서 블록 선언된 x
는 블록 외부에서 선언된 x
와 동일하지 않다.
JS Scope에서 자세하게 확인 가능하다.
(참조 : https://www.w3schools.com/js/js_scope.asp)
var
변수를 다시 선언하는 것은 프로그램 어디에서나 허용된다: var x = 2; // Allowed
var x = 3; // Allowed
x = 4; // Allowed
var
또는 let
변수를 const
로 재 선언하는 것은 허용 되지 않는다: var x = 2; // Allowed
const x = 2; // Not allowed
{
let x = 2; // Allowed
const x = 2; // Not allowed
}
{
const x = 2; // Allowed
const x = 2; // Not allowed
}
const
변수를 재할당하는 것은 허용되지 않는다: const x = 2; // Allowed
x = 2; // Not allowed
var x = 2; // Not allowed
let x = 2; // Not allowed
const x = 2; // Not allowed
{
const x = 2; // Allowed
x = 2; // Not allowed
var x = 2; // Not allowed
let x = 2; // Not allowed
const x = 2; // Not allowed
}
const
를 사용하여 변수를 다시 선언하는 것은 허용된다: const x = 2; // Allowed
{
const x = 3; // Allowed
}
{
const x = 4; // Allowed
}
var
로 정의된 변수는 맨위로 올려지며 언제든지 초기화 가능하다. 즉, 선언하기 전에 변수를 사용할 수 있다.
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Hoisting</h2>
<p>With <b>var</b>, you can use a variable before it is declared:</p>
<p id="demo"></p>
<script>
carName = "Volvo";
document.getElementById("demo").innerHTML = carName;
var carName;
</script>
</body>
</html>
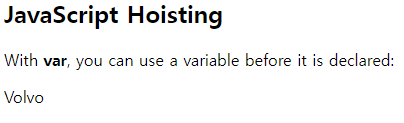
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Hoisting</h2>
<p>With <b>const</b>, you cannot use a variable before it is declared:</p>
<p id="demo"></p>
<script>
try {
alert(carName);
const carName = "Volvo";
}
catch (err) {
document.getElementById("demo").innerHTML = err;
}
</script>
</body>
</html>
※const
로 정의된 변수도 맨위로 올려지지만 초기화는 되지 않는다. 즉, const
변수를 선언 하기전에 사용하면ReferenceError
발생한다.
Hoisting에 대해 더 알고 싶다면 'JavaScript Hoisting'을 공부하자