플로이드 워셜 주의할 점
- 배열을 사용할 때 초기화를 잘 해주자. (INF 값 및 0값 그리고 거리 간의 비용)
- 3중 for문을 돌때 가장 위에 경유지를 설정해야 한다.
import java.util.Arrays;
class Solution {
public int solution(int nodeNum, int startNode, int aHome, int bHome, int[][] fares) {
int[][] graph = new int[nodeNum + 1][nodeNum + 1];
for (int[] ints : graph) {
Arrays.fill(ints, (int) Math.pow(10, 8));
}
for (int i = 0; i <= nodeNum; i++) {
graph[i][i] = 0;
}
for (int[] fare : fares) {
int node1 = fare[0];
int node2 = fare[1];
int cost = fare[2];
graph[node1][node2] = cost;
graph[node2][node1] = cost;
}
floydWarshall(nodeNum, graph);
int minCost = Integer.MAX_VALUE;
for (int shareNode = 1; shareNode <= nodeNum; shareNode++) {
int shareCost = graph[startNode][shareNode];
int aCost = graph[shareNode][aHome];
int bCost = graph[shareNode][bHome];
int totalCost = shareCost + aCost + bCost;
minCost = Math.min(minCost, totalCost);
}
return minCost;
}
private void floydWarshall(int nodeNum, int[][] graph) {
for (int mid = 1; mid <= nodeNum; mid++) {
for (int start = 1; start <= nodeNum; start++) {
for (int end = 1; end <= nodeNum; end++) {
int originCost = graph[start][end];
int newCost = graph[start][mid] + graph[mid][end];
if (originCost <= newCost) continue;
graph[start][end] = newCost;
}
}
}
}
}
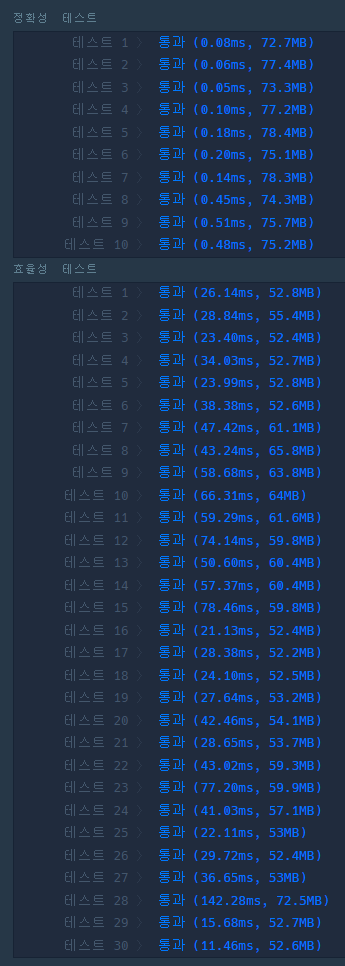