package com.company;
import java.util.*;
class Solution {
List<List<DeliverNode>> graph;
int[] roadLengthArrFromStartNode;
public int solution(int villageNum, int[][] roadInfoArr, int targetTime) {
int answer = 0;
graph = new ArrayList<>();
roadLengthArrFromStartNode = new int[villageNum + 1];
final int INF = Integer.MAX_VALUE;
Arrays.fill(roadLengthArrFromStartNode, INF);
for (int i = 0; i < villageNum + 1; i++) {
graph.add(new ArrayList<>());
}
for (int[] roadInfo : roadInfoArr) {
int start = roadInfo[0];
int dest = roadInfo[1];
int roadLength = roadInfo[2];
graph.get(start).add(new DeliverNode(dest, roadLength));
graph.get(dest).add(new DeliverNode(start, roadLength));
}
dijkstra(1);
for (int deliverTime : roadLengthArrFromStartNode) {
if (deliverTime <= targetTime)
answer++;
}
return answer;
}
private void dijkstra(int startNodeNum) {
PriorityQueue<DeliverNode> pq = new PriorityQueue<>();
pq.add(new DeliverNode(startNodeNum, 0));
roadLengthArrFromStartNode[startNodeNum] = 0;
while (!pq.isEmpty()) {
DeliverNode tempNode= pq.poll();
int nodeDestination = tempNode.getDestination();
int existDistance = tempNode.getDistance();
if(existDistance > roadLengthArrFromStartNode[nodeDestination]) continue;
for (int i = 0; i < graph.get(nodeDestination).size(); i++){
int distanceFromCurToNextDestination = graph.get(nodeDestination).get(i).getDistance();
int nextDestination = graph.get(nodeDestination).get(i).getDestination();
if(existDistance + distanceFromCurToNextDestination < roadLengthArrFromStartNode[nextDestination]){
roadLengthArrFromStartNode[nextDestination] = existDistance + distanceFromCurToNextDestination;
pq.add(new DeliverNode(nextDestination, existDistance + distanceFromCurToNextDestination));
}
}
}
}
}
class DeliverNode implements Comparable<DeliverNode>{
int destination;
int distance;
public DeliverNode(int destination, int distance) {
this.destination = destination;
this.distance = distance;
}
public int getDestination() {
return destination;
}
public int getDistance() {
return distance;
}
@Override
public int compareTo(DeliverNode o) {
return this.getDistance() - o.getDistance();
}
}
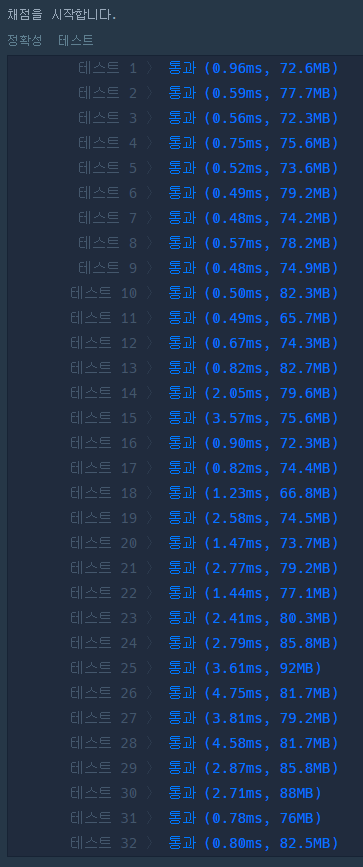