1차 풀이
import java.util.Comparator;
import java.util.PriorityQueue;
class Solution {
public int solution(int scoreMax, int appleNum, int[] scores) {
int answer = 0;
PriorityQueue<Integer> pq = new PriorityQueue<>(Comparator.reverseOrder());
for (int j : scores) {
pq.offer(j);
}
while (pq.size() >= appleNum) {
int min = Integer.MAX_VALUE;
for (int i = 0; i < appleNum; i++) {
int appleScore = pq.poll();
min = Math.min(min, appleScore);
}
answer += (min * appleNum);
}
return answer;
}
}
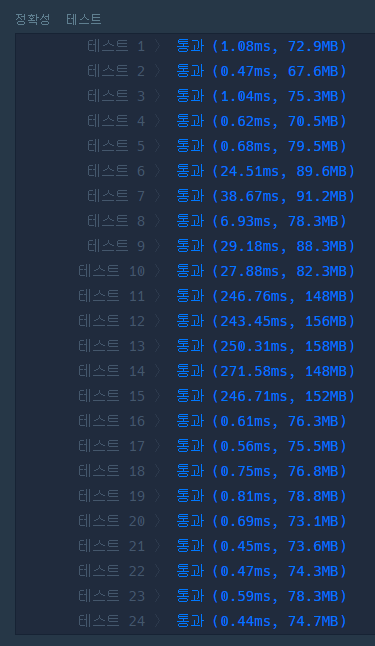
2차 풀이
import java.util.Comparator;
import java.util.PriorityQueue;
class Solution {
public int solution(int scoreMax, int appleNum, int[] scores) {
int answer = 0;
PriorityQueue<Integer> pq = new PriorityQueue<>(Comparator.reverseOrder());
for (int j : scores) {
pq.offer(j);
}
while (pq.size() >= appleNum) {
int min = Integer.MAX_VALUE;
for (int i = 0; i < appleNum; i++) {
min = pq.poll();
}
answer += (min * appleNum);
}
return answer;
}
}
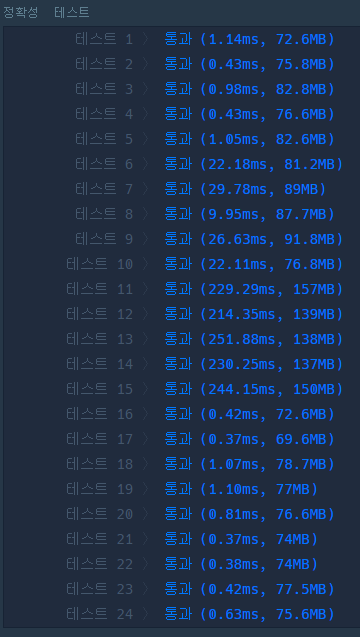
3차 풀이 (정답 참고)
import java.util.Arrays;
class Solution {
public int solution(int scoreMax, int appleNum, int[] scores) {
int answer = 0;
Arrays.sort(scores);
int idx = scores.length;
while (idx >= appleNum) {
answer += (scores[idx - appleNum] * appleNum);
idx -= appleNum;
}
return answer;
}
}
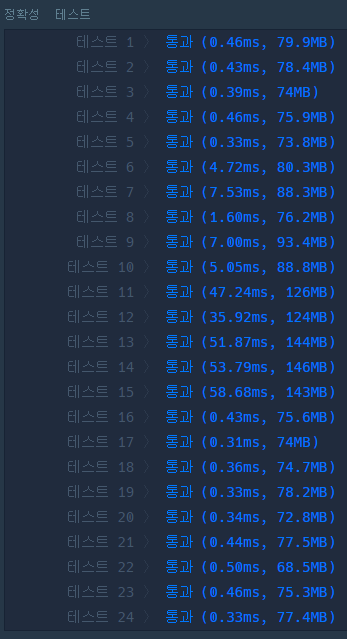