package com.company;
import java.util.Arrays;
import java.util.Collections;
import java.util.LinkedList;
import java.util.Queue;
public class Solution {
static public void main(String[] args) {
Solution testSolution = new Solution();
int[] quizArr1 = {2, 1, 3, 2};
int quizInt1 = 2;
int[] quizArr2 = {1, 1, 9, 1, 1, 1};
int quizInt2 = 0;
System.out.println(testSolution.solution(quizArr1, quizInt1));
System.out.println(testSolution.solution(quizArr2, quizInt2));
}
public int solution(int[] priorities, int target) {
int answer = 0;
Queue<Paper> queue = new LinkedList<Paper>();
for (int i = 0; i < priorities.length; i++) {
queue.add(new Paper(priorities[i], i));
}
Integer[] sortedDescPriorities = new Integer[priorities.length];
Arrays.setAll(sortedDescPriorities, index -> priorities[index]);
Arrays.sort(sortedDescPriorities, Collections.reverseOrder());
int priorIndex = 0;
while(true){
Paper headValue = null;
if(!queue.isEmpty())
headValue = queue.poll();
if(headValue.getPriority() != sortedDescPriorities[priorIndex] ){
queue.add(headValue);
}
else{
priorIndex++;
answer++;
if(headValue.getIndex() == target)
break;
}
}
return answer;
}
}
class Paper{
int priority;
int index;
public Paper(int priority, int index) {
this.priority = priority;
this.index = index;
}
public int getPriority() {
return priority;
}
public int getIndex() {
return index;
}
}
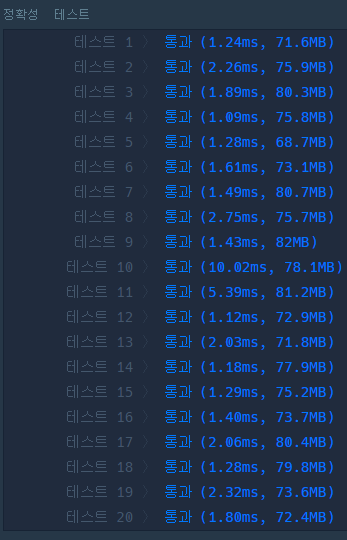