문제
- 문장이 하나 주어지면, 해당 문장 속에서 길이가 가장 긴 단어를 출력한다. 문장 속의 단어는 공백으로 구분되고, 가장 길이가 긴 단어가 여러 개인 경우 문장 속에서 가장 앞쪽에 위치한 단어를 답으로 한다.
입출력
it is time to study
study
풀이 1 : split() 이용
public String solution(String sentence) {
String[] wordArray = sentence.split(" ");
int maxLength = 0;
String longestWord = "";
for (String word : wordArray) {
if (word.length() > maxLength) {
maxLength = word.length();
longestWord = word;
}
}
return longestWord;
}
- 구분자를 기준으로 문자열을 자르고 배열로 리턴하는
spilt()
을 이용한다. 이 때, sentence.split(" ")
처럼 구분자를 “ “ 으로 주어 공백을 기준으로 문자열을 자른다.
- 이 후 잘려진 문자열이 담긴
wordArray
배열을 향상된 for문을 이용하여 순회하며 가장 긴 단어를 찾는데, 이 때 중요한 것은 maxLength
(현재까지 가장 긴 단어) 보다 클 때만 단어와 길이를 갱신 해주는 것이다. 즉 ‘≥’ 가 아닌 ‘>’를 사용해야 한다.
- ‘≥’ 를 사용할 경우 뒷 순서에서 같은 길이의 단어를 찾았을 때도 갱신이 되기 때문에, ‘긴 단어가 여러 개인 경우 문장 속에서 가장 앞쪽에 위치한 단어‘가 정답이 되어야한다는 문제의 조건을 위배하게 된다.
풀이 2 : indexOf(), substring() 활용
public String solution(String sentence) {
String longestWord = "";
int maxLength = Integer.MIN_VALUE;
int pos;
while ((pos = sentence.indexOf(" ")) != -1) {
String tmp = sentence.substring(0, pos);
if (tmp.length() > maxLength) {
maxLength = tmp.length();
longestWord = tmp;
}
sentence = sentence.substring(pos + 1);
}
if (sentence.length() > maxLength) {
maxLength = sentence.length();
longestWord = sentence;
}
return longestWord;
}
indexOf
를 사용하여 문자열에서 공백이 어디 위치하는지 인덱스를 찾는다.
- indexOf 는 찾는 문자열이 없는 경우 ‘-1’을 리턴하기 때문에, while문에서는 문장 마지막 단어 직전 단어까지만 확인할 수 있다.
substring
을 이용해서 주어진 문장에서 이미 확인이 끝난 단어와 공백하나를 제거한다. 이를 그림으로 표현하면 아래와 같다.
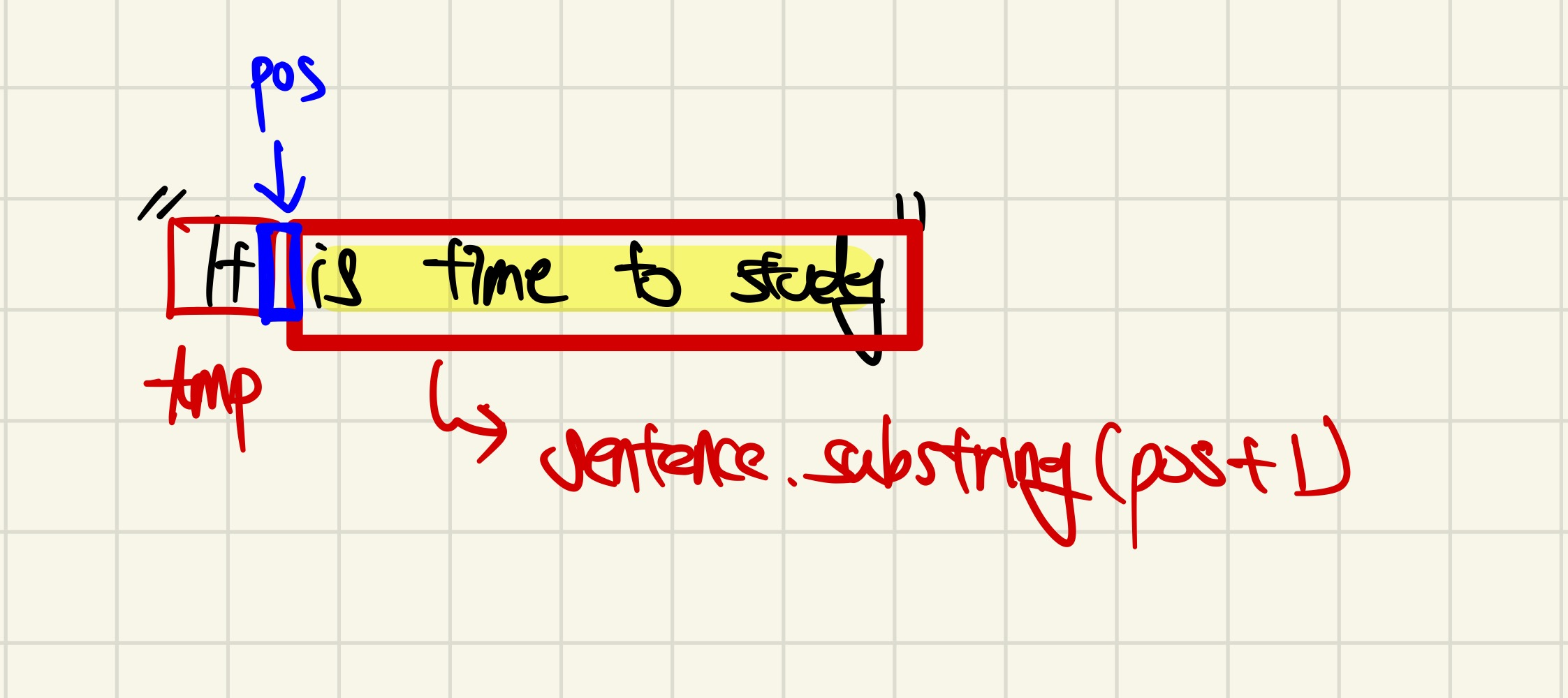
- 마지막으로 문장을 한 번 더 검사한다. while문 이후 처음 문장의 마지막 단어가 남게 되므로, 마지막에 한 번 더 검사하여 해당 단어까지 문자열 길이를 비교한다.
전체 코드
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Main {
public String solution(String sentence) {
String[] wordArray = sentence.split(" ");
int maxLength = 0;
String longestWord = "";
for (String word : wordArray) {
if (word.length() > maxLength) {
maxLength = word.length();
longestWord = word;
}
}
return longestWord;
}
public static void main(String[] args) throws IOException {
Main sol = new Main();
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String sentence = br.readLine();
System.out.println(sol.solution(sentence));
}
}
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Main {
public String solution(String sentence) {
String longestWord = "";
int maxLength = Integer.MIN_VALUE;
int pos;
while ((pos = sentence.indexOf(" ")) != -1) {
String tmp = sentence.substring(0, pos);
if (tmp.length() > maxLength) {
maxLength = tmp.length();
longestWord = tmp;
}
sentence = sentence.substring(pos + 1);
}
if (sentence.length() > maxLength) {
maxLength = sentence.length();
longestWord = sentence;
}
return longestWord;
}
public static void main(String[] args) throws IOException {
Main sol = new Main();
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String sentence = br.readLine();
System.out.println(sol.solution(sentence));
}
}