resource controller
php artisan make:controller PostController --resource
PostController 'CRUD' 구조
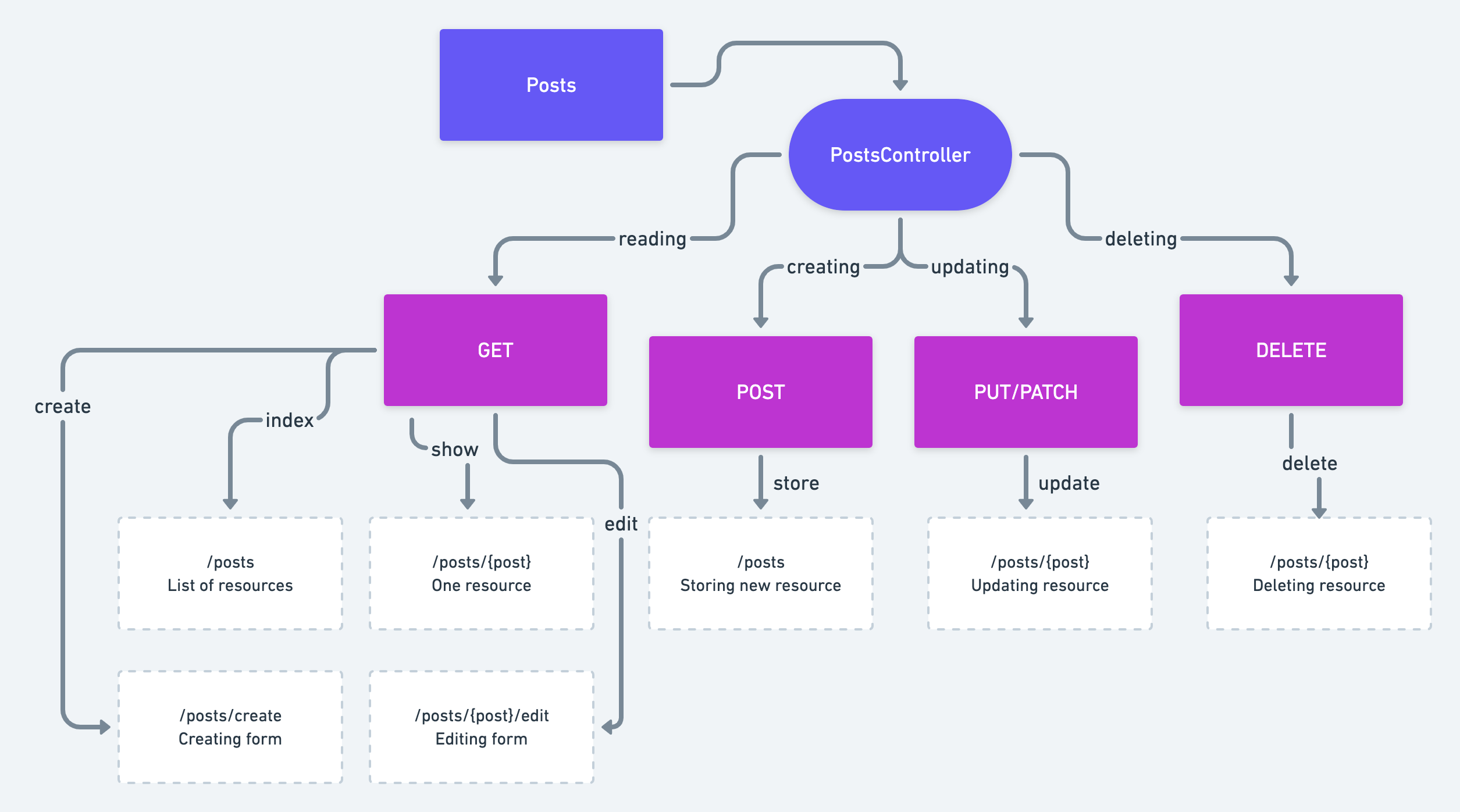
실습 코드
app/Http/Controller/PostController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class PostController extends Controller
{
private $posts = [
1 => [
'title' => 'Intro to Laravel',
'content' => 'This is a short intro to Laravel',
'is_new' => true,
'has_comments' => true
],
2 => [
'title' => 'Intro to PHP',
'content' => 'This is a short intro to PHP',
'is_new' => false,
],
3 => [
'title' => 'Intro to Golang',
'content' => 'This is a short intro to Golang',
'is_new' => false,
]
];
public function index()
{
return view('posts.index', ['posts' => $this -> posts]);
}
public function create()
{
}
public function store(Request $request)
{
}
public function show($id)
{
abort_if(!isset($this->posts[$id]), 404);
return view('posts.show', ['post' => $this -> posts[$id]]);
}
public function edit($id)
{
}
public function update(Request $request, $id)
{
}
public function destroy($id)
{
}
}
리소스풀 컨트롤러에 의해서 구성된 액션들
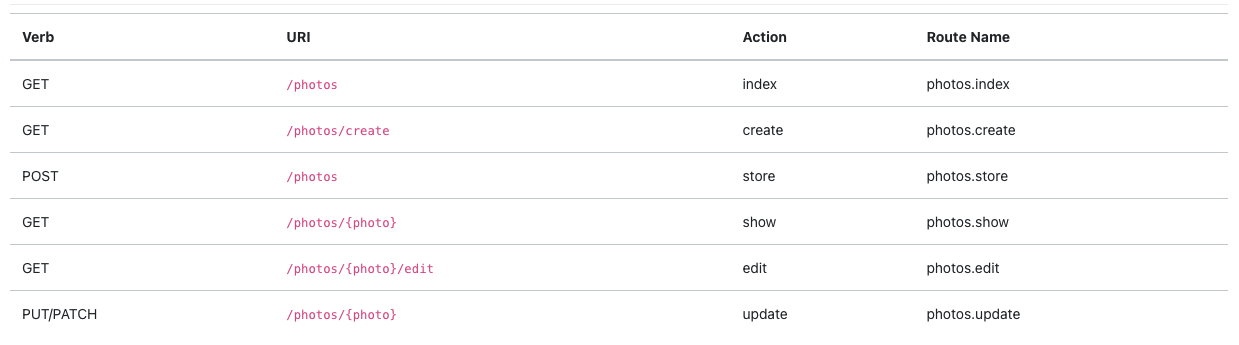
실제 리소스풀 컨트롤러에 의해서 구성된 액션들
php artisan route:list

routes/web.php
- 위의 코드로 제가 사용할 메소드는 index, show만 사용 하려 합니다.
그러기 위해서는 only()를 사용하여 주면 지정한 것만 사용하게 됩니다.
- 반대로 except()를 사용하게 된다면 원하는 메소드를 사용하지 않게 합니다.
Route::resource('posts', \App\Http\Controllers\PostController::class)->only(['index','show']);
결과
- 이 전에 했던 실습과 똑같이 나오는 것을 확인 할 수 있습니다. 조금 복잡하다고 생각은 하고 있습니다. 아직 계속 해봐야 알 것 같은 구조입니다. ㅠㅠ
posts/index.blade.php
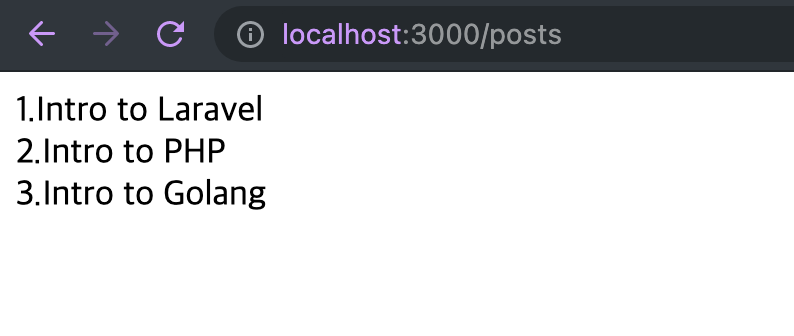
posts/show.blade.php
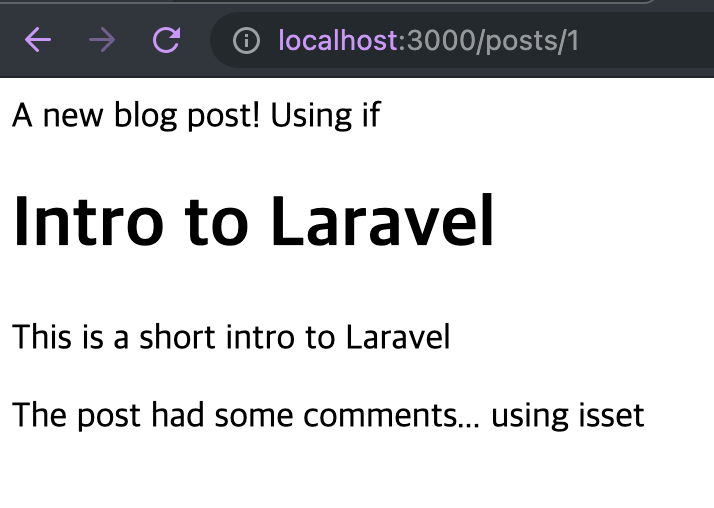