문제
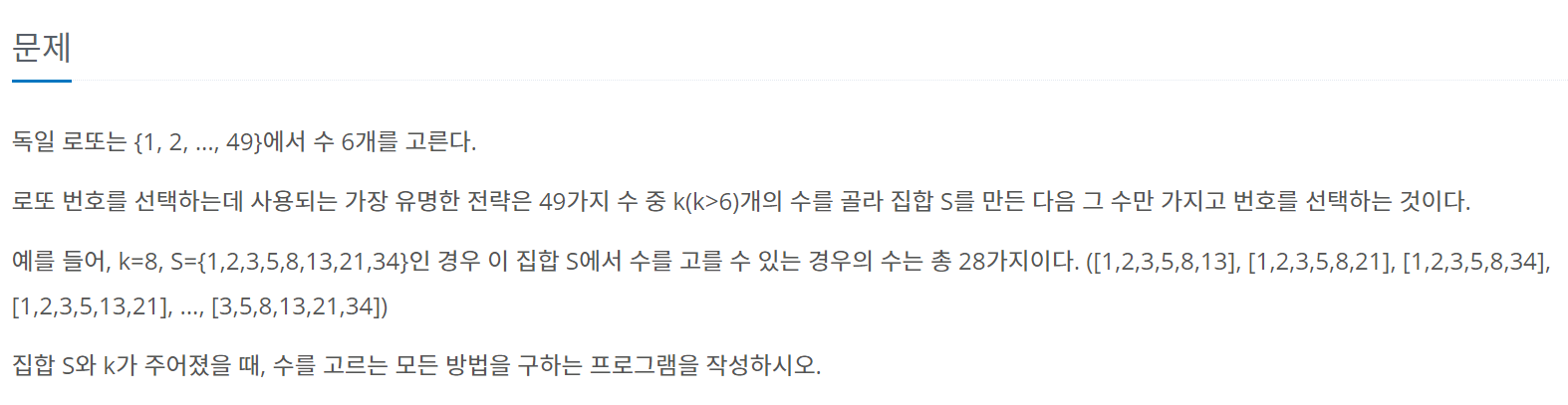
제한사항
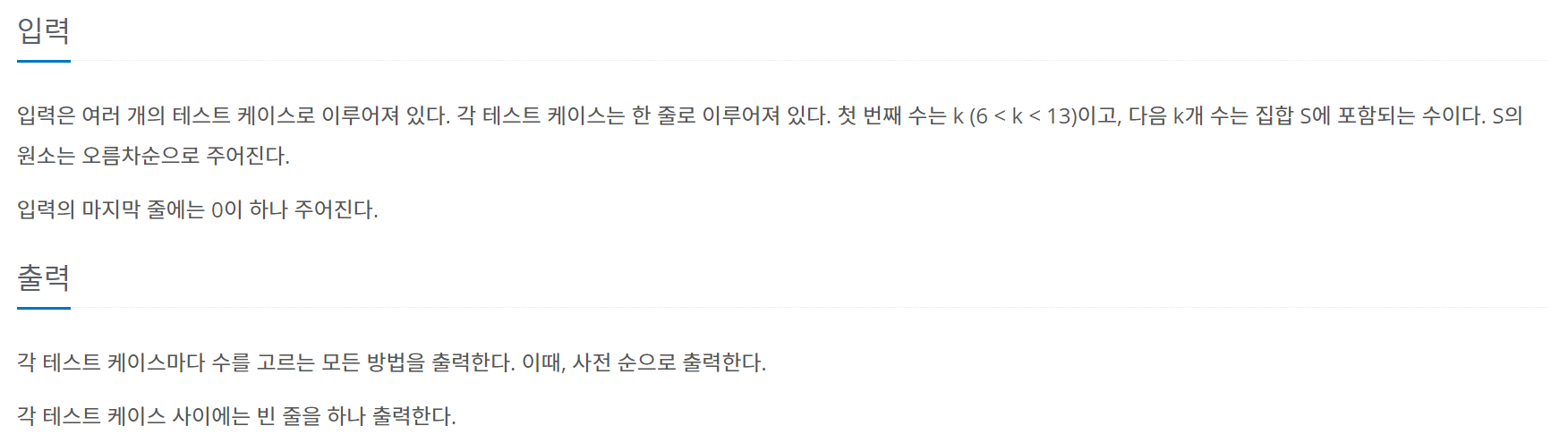
입출력 예
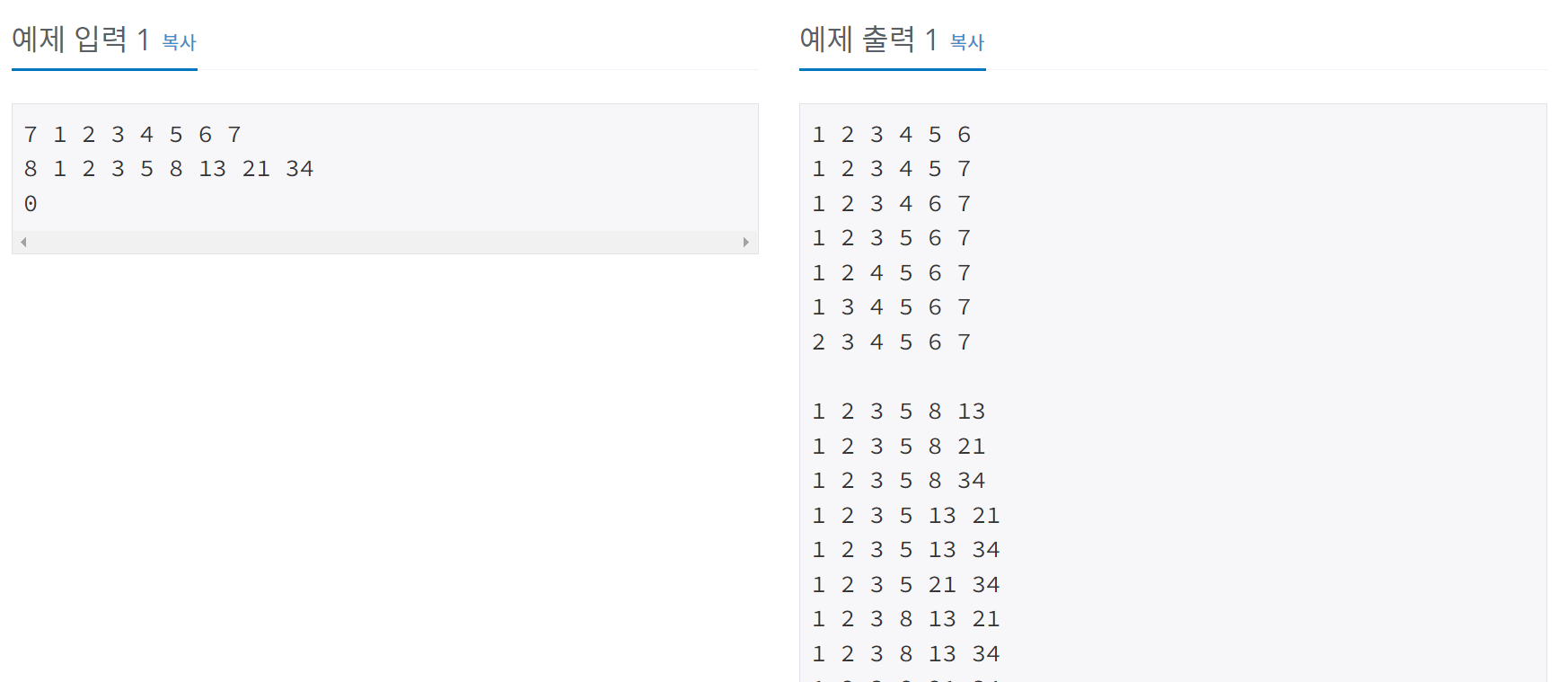
풀이
let input = require('fs').readFileSync('/dev/stdin').toString().trim().split("\n");
const solution = (n, arr) => {
let answer = [];
let visited = new Array(n).fill(0);
let tmp = [];
const dfs = (index, cnt) => {
if (cnt === 6) {
answer.push(tmp.join(" "));
} else {
for (let i = index; i < n; i++) {
if (!visited[i]) {
visited[i] = 1;
tmp.push(arr[i]);
dfs(i, tmp.length);
tmp.pop();
visited[i] = 0;
}
}
}
};
dfs(0);
return answer.join("\n");
};
for(let x of input){
let arr = x.split(" ").map(e => +e);
let n = arr.shift();
console.log(solution(n, arr));
console.log("");
}
- 조합문제이다.
- 입력, 출력을 착각해서 괜한 시간을 잡아먹었다. 잘 읽자!
const combinations = function* (elements, selectNumber) {
for (let i = 0; i < elements.length; i++) {
if (selectNumber === 1) {
yield [elements[i]];
} else {
const fixed = elements[i];
const rest = combinations(elements.slice(i + 1), selectNumber - 1);
for (const a of rest) {
yield [fixed, ...a];
}
}
}
};
const input = ["7 1 2 3 4 5 6 7", "8 1 2 3 5 8 13 21 34", "0"];
const sol = (str) => {
const [n, ...arr] = str.split(" ").map(Number);
const answer = [];
for (let number of combinations(arr, 6)) {
answer.push(number.join(" "));
}
return answer.join("\n");
};
for (let x of input) {
console.log(sol(x));
console.log("");
}