HATEOAS
- Hypermedia As The Engine Of Application State의 약자
- REST 아키텍쳐의 한 구성요소로 Level 3에 해당
- 리소스에 대한 추가 작업을 제공하는 방식
- 구현이 복잡해지는 단점이 존재
HATEOAS 적용하기
dependencies
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-hateoas'
}
Entity를 반환할 때
@GetMapping("/{id}")
public EntityModel<TeamDTO> getTeam(@PathVariable long id) {
TeamDTO team = teamService.getTeamById(id);
if(team == null) {
throw new TeamNotFoundException("Team not found with id: " + id);
}
return EntityModel.of(team,
linkTo(methodOn(TeamController.class).getTeam(id)).withSelfRel(),
linkTo(methodOn(TeamController.class).getAllTeams()).withRel("teams"),
linkTo(methodOn(TeamController.class).deleteTeam(id)).withRel("delete"),
linkTo(methodOn(TeamController.class).updateTeam(id, team)).withRel("update")
);
}
- EntityModel<?>을 이용하여 반환한다.
- linkTo를 이용하여 링크를 생성할 수 있다.
- methodOn은 클래스를 선택하고 함수를 선택한다.
- withSelfRel(): self로 반환
- withRel(): 구분을 지정하는 것
Entity List를 반환할 때
@GetMapping("")
public CollectionModel<EntityModel<TeamDTO>> getAllTeams() {
List<TeamDTO> teams = teamService.getAllTeams();
if(teams.isEmpty()) {
throw new TeamNotFoundException("No teams found");
}
List<EntityModel<TeamDTO>> teamEntityModels = teams.stream()
.map(team -> EntityModel.of(team,
linkTo(methodOn(TeamController.class).getTeam(team.getId())).withSelfRel(),
linkTo(methodOn(TeamController.class).getAllTeams()).withRel("teams"),
linkTo(methodOn(TeamController.class).deleteTeam(team.getId())).withRel("delete"),
linkTo(methodOn(TeamController.class).updateTeam(team.getId(), team)).withRel("update"))
)
.toList();
return CollectionModel.of(teamEntityModels,
linkTo(methodOn(TeamController.class).getAllTeams()).withSelfRel());
}
- CollectionModel<EntityModel<?>>: 다음과 같이 CollectionModel을 사용해서 EntityModel의 리스트를 반환할 수 있다.
ResponseEntity와 함께 사용하기
@PostMapping("")
public ResponseEntity<CollectionModel<EntityModel<TeamDTO>>> createTeams(@Valid @RequestBody TeamDTOListWrapper teamDTOList) {
List<TeamDTO> savedTeams = teamService.createTeams(teamDTOList.getTeamDTOList());
URI location = ServletUriComponentsBuilder
.fromCurrentRequest()
.path("")
.build()
.toUri();
List<EntityModel<TeamDTO>> teamEntityModels = savedTeams.stream()
.map(team -> EntityModel.of(team,
linkTo(methodOn(TeamController.class).getTeam(team.getId())).withSelfRel(),
linkTo(methodOn(TeamController.class).getAllTeams()).withRel("teams")))
.toList();
return ResponseEntity.created(location).body(CollectionModel.of(teamEntityModels,
linkTo(methodOn(TeamController.class).getAllTeams()).withSelfRel()));
}
- 다음과 같이 ResponseEntity.created(location).body()에 담아서 반환할 수 있다.
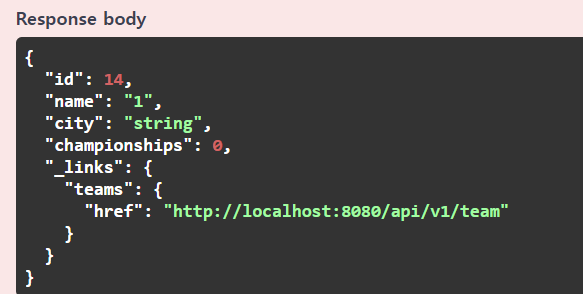
다음과 같다면 잘 수행한 것이다.