Http 요청이란?
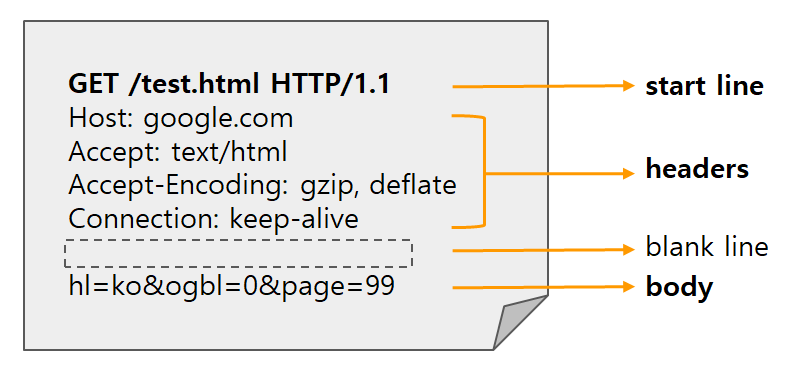
- 다음과 같이 POST, GET등의 요청으로 구성됨!
사용자의 요청
POST와 GET의 차이
- GET은 데이터의 요청을 위한 것으로 URL 주소 끝에 파라미터를 포함
- POST는 생성/업데이트를 위한 데이터를 보내기 위해 사용함, body에 데이터를 포함
- POST는 캐시되지 않음
- POST도 개발자 도구를 사용하면 확인할 수 있기 때문에 암호화를 해서 데이터를 전송해야함!
사용자 요청 받기
- HttpServletRequest
- @PathVariable
- @RequestParam
- @ModelAttribute
- @RequestBody
HttpServletRequest
- 헤더정보, 파라미터, 쿠키, URI, URL등의 정보등을 가져옴
- 모든 정보를 가져올 수 있다.
@GetMapping("/hsr")
public String hsr(HttpServletRequest request) {
String result = "";
result += "Request URI: " + request.getRequestURI() + "<br>";
result += "Request URL: " + request.getRequestURL() + "<br>";
result += "Request Method: " + request.getMethod() + "<br>";
result += "Request Protocol: " + request.getProtocol() + "<br>";
return result;
}
@PathVariable
- "/{id}" 다음과 같은 URL로 부터 데이터를 가져옴
- "/{id}/path/{name}"과 같이 2개의 데이터도 가져올 수 있음
@GetMapping("/{id}")
public String pathVariable(@PathVariable long id) {
return id + " request received!";
}
@GetMapping("/{id}/path/{name}")
public String pathVariables(@PathVariable long id, @PathVariable(value = "name") String str) {
return id + " " + str + " request received!";
}
- @PathVariable: 변수명과 위에서 설정한 이름이 같아야함, 다르게 하고 싶다면 (value = "name")과 같이 설정해야함
@RequestParam
- GET, POST로 부터 보낸 파라미터를 가져옴
- 배열 정보도 가져올 수 있음
- @PathVariable와 같이 사용할 수 있음
- String, int 등 단순 타입은 생략 가능
- required: 필수여부 지정 가능, true/false
- value: key값 지정 가능
- defaultValue: 기본 값 지정
- 객체는 받아올 수 없음!
@GetMapping("/get-str")
public String getString(@RequestParam String str) {
return str;
}
@PostMapping("/post-strs")
public String postStrings(@RequestParam String[] strs) {
String result = "";
for(String str : strs) {
result += str + " ";
}
return result;
}
@ModelAttribute
- HTTP 파라미터를 Object에 바인딩하는 일종의 커멘드 함수
- 생성자 또는 setter가 필요하다.
- 생략이 가능하다.
- @Valid을 사용할 수 있음
- MyRequest.java
package com.chan.ssb;
public class MyRequest {
private long id;
private String name;
private String city;
public MyRequest() {
}
public MyRequest(long id, String name, String city) {
this.id = id;
this.name = name;
this.city = city;
}
public long getId() {
return id;
}
public String getName() {
return name;
}
public String getCity() {
return city;
}
public void setId(long id) {
this.id = id;
}
public void setName(String name) {
this.name = name;
}
public void setCity(String city) {
this.city = city;
}
}
@GetMapping("/get-obj")
public MyRequest getObj(@ModelAttribute MyRequest myRequest) {
return myRequest;
}
- 객체 배열을 받아올 수 있다고 하지만 아직 방법을 찾지 못했다....
@RequestBody
- 클라이언트가 보내는 HTTP 요청 Body (JSON, XML)을 받아옴
- HttpMessageConverter를 이용해서 자바객체로 변환함
- Jackson을 이용해서 파싱
- 객체 배열을 가져올수있다.
- @Vaild 사용가능
- POST요청에 대하여 사용가능!!
@PostMapping("/post-objs")
public MyRequest[] getObjsByRb(@RequestBody MyRequest[] myRequests) {
return myRequests;
}