문제
https://leetcode.com/problems/majority-element/description/?envType=study-plan-v2&envId=top-interview-150
해결 방법 1
- HashMap을 사용하여 해결
- HashMap에서 nums/[i]를 Key로 가진 Value 조회 후 Value 증가
- 이 때, Value값이 최대인 nums[i]를 저장 후 return
코드
class Solution {
public int majorityElement(int[] nums) {
Map<Integer, Integer> map = new HashMap<>();
int maxCnt = 1;
int maxNum = nums[0];
for (int num : nums) {
int numCnt = map.getOrDefault(num, 0) + 1;
map.put(num, numCnt);
if (numCnt > maxCnt) {
maxCnt = numCnt;
maxNum = num;
}
}
return maxNum;
}
}
결과
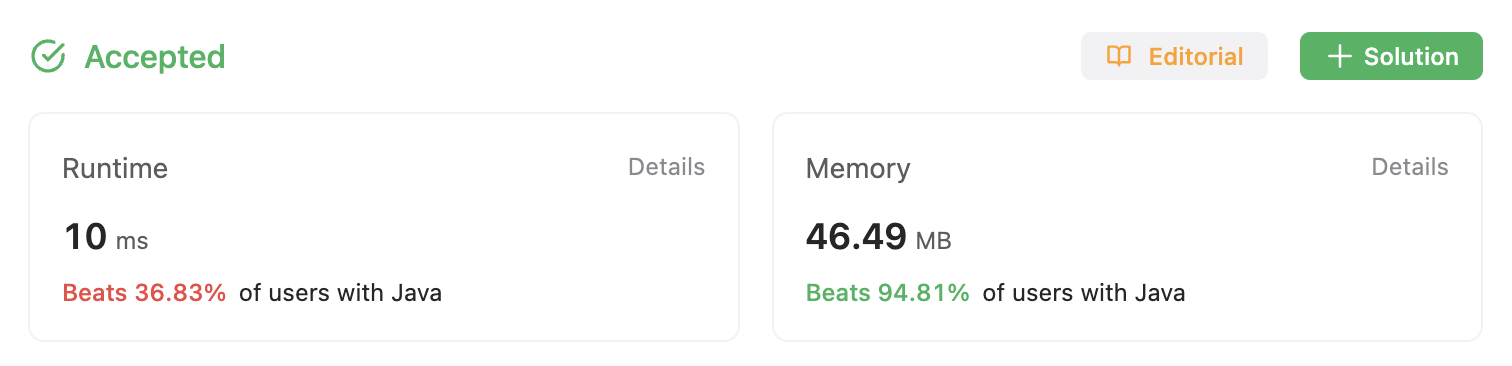
해결 방법 2
- nums 배열 정렬
- Majority Element는 무조건 n/2보다 많이 존재한다고 했으므로, nums의 가운데에는 무조건 Majority Element가 있음
코드
class Solution {
public int majorityElement(int[] nums) {
Arrays.sort(nums);
return nums[nums.length / 2];
}
}
결과
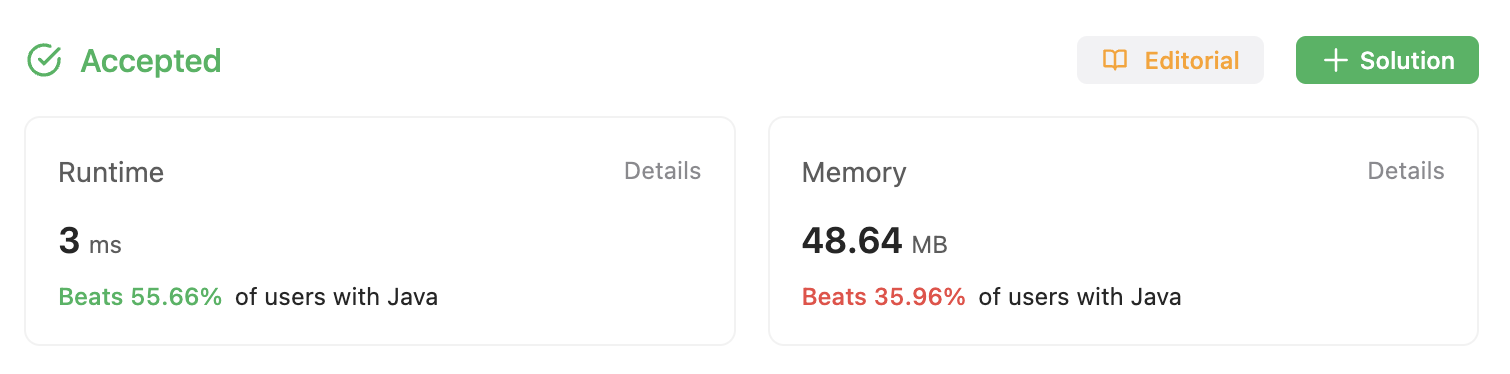