1. 프린터 큐
- 문제 이해 잘못해서 거의 5시간 걸린 문제 ★★★★★★★★★★★★
package problem_solving.queue;
import java.util.Collections;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.PriorityQueue;
import java.util.Queue;
import java.util.Scanner;
public class BaekJoon_1966 {
static Queue<HashMap<Integer, Integer>> q = new LinkedList();
static PriorityQueue<Integer> pq = new PriorityQueue<>(Collections.reverseOrder());
static int cnt ;
static boolean flag ;
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int T = Integer.parseInt(sc.next());
int checkNum = 0;
while(T-- > 0){
int n = Integer.parseInt(sc.next());
int m = Integer.parseInt(sc.next());
for(int i= 0 ; i < n ; i++) {
HashMap<Integer, Integer> hm = new HashMap<>();
if( i == m ) {
int a = Integer.parseInt(sc.next());
hm.put(a,1);
pq.offer(a);
checkNum = a ;
}else {
int a = Integer.parseInt(sc.next());
pq.offer(a);
hm.put(a, 0);
}
q.offer(hm);
}
maxCheck(checkNum);
if( n == 1 ) {
System.out.println(1);
cnt= 0 ;
q.clear();
pq.clear();
flag = false;
continue;
}
System.out.println(cnt);
cnt= 0 ;
flag = false;
q.clear();
pq.clear();
}
}
public static void maxCheck(int checkNum) {
HashMap<Integer,Integer> hm = q.peek();
for(int num : hm.keySet()) {
if( flag ) {
return ;
}
int max = pq.peek();
int val = hm.get(num);
if( max == checkNum && num == checkNum && val == 1) {
cnt++;
flag= true;
return ;
} else if( max == checkNum && num == checkNum) {
cnt++;
q.poll();
maxCheck(checkNum);
} else if( max == num && checkNum!=num) {
pq.poll();
q.poll();
cnt++;
maxCheck(checkNum);
} else {
q.offer(q.poll());
maxCheck(checkNum);
}
}
}
}
2. 큐2
package problem_solving.queue;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.LinkedList;
import java.util.Queue;
import java.util.Scanner;
public class BaekJoon_18258 {
public static void main(String[] args) throws NumberFormatException, IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int n = Integer.parseInt(br.readLine());
Queue<Integer> q = new LinkedList();
int lastNum = 0 ;
StringBuilder sb = new StringBuilder();
for(int i= 0 ; i < n ; i++) {
String s = br.readLine();
String [] arr = s.split(" ");
if ( arr[0].equals("push")) {
q.offer(Integer.parseInt(arr[1]));
lastNum = Integer.parseInt(arr[1]);
} else if (arr[0].equals("pop")) {
if( q.isEmpty()) {
sb.append(-1+"\n");
}else {
sb.append(q.poll()+"\n");
}
} else if (arr[0].equals("size")) {
sb.append(q.size()+"\n");
} else if (arr[0].equals("empty")) {
if(q.isEmpty()) {
sb.append(1+"\n");
}else {
sb.append(0+"\n");
}
} else if (arr[0].equals("front")) {
if( q.peek()!=null) {
sb.append(q.peek()+"\n");
}else {
sb.append(-1+"\n");
}
} else if (arr[0].equals("back")) {
if( q.isEmpty()) {
sb.append(-1+"\n");
}else {
sb.append(lastNum+"\n");
}
}
}
System.out.println(sb.toString());
}
}
3. 균형잡힌 세상
package problem_solving.stack;
import java.util.LinkedList;
import java.util.Queue;
import java.util.Scanner;
import java.util.Stack;
public class BaekJoon_4949 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in) ;
while(true) {
Stack<Character> st = new Stack<>();
String startOrEnd = sc.nextLine();
if( startOrEnd.equals(".")) {
break;
}
for(int i= 0 ; i <startOrEnd.length();i++) {
if( startOrEnd.charAt(i) == '(' || startOrEnd.charAt(i) == '[' ) {
st.push(startOrEnd.charAt(i));
} else if ( startOrEnd.charAt(i) == ')' ||startOrEnd.charAt(i) == ']' ) {
if( st.isEmpty()|| (st.peek() == '(' && startOrEnd.charAt(i)==']') ||
(st.peek()=='[' && startOrEnd.charAt(i)==')')) {
st.push(startOrEnd.charAt(i));
break;
}
st.pop();
}
}
if( !st.isEmpty()) {
System.out.println("no");
}else {
System.out.println("yes");
}
}
}
}
4. 수 정렬하기 2
package problem_solving.sort;
import java.util.Arrays;
import java.util.Scanner;
public class BaekJoon_2751 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = Integer.parseInt(sc.next());
int [] arr = new int[n];
StringBuilder sb = new StringBuilder();
for(int i= 0 ; i < n ; i++) {
arr[i] = Integer.parseInt(sc.next());
}
Arrays.sort(arr);
for(int i= 0 ; i < n ; i++) {
sb.append(arr[i]).append('\n');
}
System.out.println(sb);
}
}
5. 카드
package problem_solving.sort;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Scanner;
import java.util.TreeMap;
public class BaekJoon_11652 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
TreeMap<Long,Integer> tm = new TreeMap<>();
int n = Integer.parseInt(sc.next());
for(int i = 0 ; i < n ; i++) {
Long key = Long.parseLong(sc.next());
if( !tm.containsKey(key)) {
tm.put(key, 1);
}else {
int cnt = tm.get(key);
tm.put(key, cnt+1);
}
}
int [] arr = new int[tm.size()];
int index = 0 ;
for(Long num : tm.keySet()) {
int cnt = tm.get(num);
arr[index++] = cnt;
}
Arrays.sort(arr);
int maxCnt = arr[arr.length-1];
for(Long num : tm.keySet()) {
if( tm.get(num)==maxCnt) {
System.out.println(num);
return ;
}
}
}
}
드디어 골드다
Silver 1 92% -> Gold 5 3%
Queue - 2문제
Stack - 1문제
sort - 1문제
Hash - 1문제
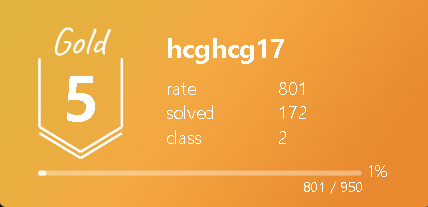