🧩 @postConstruct
- 객체의 초기화 부분
- 객체가 생성된 후 별도의 초기화 작업을 위해 실행하는 메소드를 선언한다.
- WAS가 띄워질 때 실행된다.
🧩 @PreDestroy
🧩 경로 이동
- context path를 추가해서 이동
- 프로젝트이름/index.html
프로젝트이름/user/userMain.jsp
- /
🧩 @PathVariable
- (@PathVariable("name")) 값을 String name 변수로 받아옴
- RequestParam
http://192.168.0.1:8080?aaa=bbb&ccc=ddd
- PathVariable
http://192.168.0.1:8080/bbb/ddd
@GetMapping("/member/{name}")
public String memberFind(@PathVariable("name") String name){
}
생략가능
public String memberFind(@PathVariable String name){
}
@GetMapping("/member/{id}/{name}")
public String memberFind(@PathVariable("id") String id,
@PathVariable("name") String name){
}
🧩 @ModelAttribute
- 요청 파라미터 처리
-> Item
객체를 생성하고, 요청 파라미터의 값을 프로퍼티 접근법
(set..) 으로 입력해준다.
-> model.addAttribute("item",item); 대신
-> @ModelAttribute("이름 설정")
🧩 RedirectAttributes
- redirect 할 때 사용될 수 있는 여러 속성값을 컨트롤
- 리다이렉트를 통해 페이지를 이동하는 것은 좋은데, 이 경우 내가 수행한 로직(상품 등록, 상품 수정 등) 이 정상적으로 완료되었는지를 알 수 없다. 그래서 리다이렉트 된 페이지에 이런 결과를 노출하고싶을 때
RedirectAttributes
를 이용하면 된다.
- URL 인코딩 뿐아니라
PathVariable
, 쿼리파라미터
처리까지 해준다.
🧩 RequiredArgsConstructor
- @RequiredArgsConstructor
-> final이 붙은 멤버변수만 사용해서 생성자를 자동으로 만들어준다
🧩상품 페이지 실습
item
@Getter @Setter
public class Item {
private Long id;
private String itemName;
private Integer price;
private Integer quantity;
public Item() {}
public Item( String itemName, Integer price, Integer quantity) {
super();
this.itemName = itemName;
this.price = price;
this.quantity = quantity;
}
}
ItemRepository
@Repository
public class ItemRepository {
private static final Map<Long, Item> store = new HashMap<Long, Item>();
private static long sequence = 0L;
public Item save(Item item) {
item.setId(++sequence);
store.put(item.getId(), item);
return item;
}
public Item findById(Long id) {
return store.get(id);
}
public List<Item> findAll(){
return new ArrayList<Item>(store.values());
}
public void update(Long itemId, Item updateParam) {
Item findItem = findById(itemId);
findItem.setItemName(updateParam.getItemName());
findItem.setPrice(updateParam.getPrice());
findItem.setQuantity(updateParam.getQuantity());
}
}
ItemController
@Controller
@RequestMapping("/basic/items")
@RequiredArgsConstructor
public class ItemController {
private final ItemRepository itemRepository;
@GetMapping
public String items(Model model) {
List<Item> items = itemRepository.findAll();
model.addAttribute("items", items);
return "basic/items";
}
@GetMapping("/{itemId}")
public String item(@PathVariable long itemId, Model model) {
Item item = itemRepository.findById(itemId);
model.addAttribute("item",item);
return "basic/item";
}
@GetMapping("/add")
public String addForm() {
return "basic/addForm";
}
public String save( @RequestParam String itemName,
@RequestParam int price,
@RequestParam Integer quantity,
Model model) {
Item item = new Item();
item.setItemName(itemName);
item.setPrice(price);
item.setQuantity(quantity);
itemRepository.save(item);
model.addAttribute("item",item);
return "basic/item";
}
public String saveV2( @ModelAttribute("item")Item item){
itemRepository.save(item);
return "basic/item";
}
public String saveV3( @ModelAttribute Item item){
itemRepository.save(item);
return "basic/item";
}
public String saveV4(Item item){
itemRepository.save(item);
return "basic/item";
}
@PostMapping("/add")
public String saveV5(Item item){
itemRepository.save(item);
return "redirect:/basic/items/" + item.getId();
}
public String saveV6(Item item, RedirectAttributes redirectAttributes){
Item saveItem = itemRepository.save(item);
redirectAttributes.addAttribute("itemId", saveItem.getId());
redirectAttributes.addAttribute("status", true);
return "redirect:/basic/items/{itemId}";
}
@GetMapping("/{itemId}/edit")
public String editForm(@PathVariable Long itemId, Model model) {
Item item = itemRepository.findById(itemId);
model.addAttribute("item",item);
return "basic/editForm";
}
@PostMapping("/{itemId}/edit")
public String edit(@PathVariable Long itemId, @ModelAttribute Item item) {
itemRepository.update(itemId, item);
return "redirect:/basic/items/{itemId}";
}
@PostConstruct
public void init() {
System.out.println("초기화 메서드");
itemRepository.save(new Item("testA",10000,10));
itemRepository.save(new Item("testB", 20000, 20));
}
@PreDestroy
public void destroy() {
System.out.println("종료 메서드");
}
}
items
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="utf-8">
<link href="./css/bootstrap.min.css"
th:href="@{/css/bootstrap.min.css}"
rel="stylesheet">
</head>
<body>
<div class="container" style="max-width: 600px">
<div class="py-5 text-center">
<h2>상품 목록</h2>
</div>
<div class="row">
<div class="col">
<button class="btn btn-primary float-end"
onclick="location.href='addForm.html'"
th:onclick="|location.href='@{/basic/items/add}'|" type="button">
상품 등록
</button>
</div>
</div>
<hr class="my-4">
<div>
<table class="table">
<thead>
<tr>
<th>ID</th>
<th>상품명</th>
<th>가격</th>
<th>수량</th>
</tr>
</thead>
<tbody>
<tr th:each="item : ${items}">
<td>
<a href="item.html"
th:href="@{/basic/items/{itemId}(itemId=${item.id})}"
th:text="${item.id}">회원id</a>
</td>
<td>
<a href="item.html"
th:href="@{|/basic/items/${item.id}|}"
th:text="${item.itemName}">상품명</a>
</td>
<td th:text="${item.price}"></td>
<td th:text="${item.quantity}"></td>
</tr>
</tbody>
</table>
</div>
</div>
</body>
</html>
item
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="utf-8">
<link href="./css/bootstrap.min.css"
th:href="@{/css/bootstrap.min.css}"
rel="stylesheet">
<style>
.container {
max-width: 560px;
}
</style>
</head>
<body>
<div class="container">
<div class="py-5 text-center">
<h2>상품 상세</h2>
</div>
<h2 th:if="${param.status}" th:text="'저장완료!'"></h2>
<div>
<label for="itemId">상품 ID</label>
<input type="text" id="itemId" name="itemId" class="form-control"
value="1" th:value="${item.Id}"readonly>
</div>
<div>
<label for="itemName">상품명</label>
<input type="text" id="itemName" name="itemName" class="form-control"
value="상품A" th:value="${item.itemName}" readonly>
</div>
<div>
<label for="price">가격</label>
<input type="text" id="price" name="price" class="form-control"
value="10000" th:value="${item.price}" readonly>
</div>
<div>
<label for="quantity">수량</label>
<input type="text" id="quantity" name="quantity" class="form-control"
value="10" th:value="${item.quantity}" readonly>
</div>
<hr class="my-4">
<div class="row">
<div class="col">
<button class="w-100 btn btn-primary btn-lg"
th:onclick="|location.href='@{/basic/items/{itemId}/edit(itemId=${item.Id})}'|"
onclick="location.href='editForm.html'" type="button">
상품수정
</button>
</div>
<div class="col">
<button class="w-100 btn btn-secondary btn-lg"
th:onclick="|location.href='@{/basic/items}'|"
onclick="location.href='items.html'" type="button">
목록으로
</button>
</div>
</div>
</div>
<script th:inline="javascript">
if([[${param.status}]]){
alert("저장완료");
}
</script>
</body>
</html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="utf-8">
<link href="./css/bootstrap.min.css"
th:href="@{/css/bootstrap.min.css}"
rel="stylesheet">
<style>
.container {
max-width: 560px;
}
</style>
</head>
<body>
<div class="container">
<div class="py-5 text-center">
<h2>상품 등록 폼</h2>
</div>
<h4 class="mb-3">상품 입력</h4>
<form action="item.html" th:action="@{/basic/items/add}" method="post">
<div>
<label for="itemName">상품명</label>
<input type="text" id="itemName" name="itemName" class="form-control"
placeholder="이름을 입력하세요">
</div>
<div>
<label for="price">가격</label>
<input type="text" id="price" name="price" class="form-control" placeholder="가격을 입력하세요">
</div>
<div>
<label for="quantity">수량</label>
<input type="text" id="quantity" name="quantity" class="form-control" placeholder="수량을 입력하세요">
</div>
<hr class="my-4">
<div class="row">
<div class="col">
<button class="w-100 btn btn-primary btn-lg" type="submit">
상품등록
</button>
</div>
<div class="col">
<button class="w-100 btn btn-secondary btn-lg"
onclick="location.href='items.html'"
th:onclick="|location.href='@{/basic/items}'|"
type="button">
취소
</button>
</div>
</div>
</form>
</div>
</body>
</html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="utf-8">
<link href="./css/bootstrap.min.css"
th:href="@{/css/bootstrap.min.css}"
rel="stylesheet">
<style>
.container {
max-width: 560px;
}
</style>
</head>
<body>
<div class="container">
<div class="py-5 text-center">
<h2>상품 수정 폼</h2>
</div>
<form action="item.html" th:action method="post">
<div>
<label for="id">상품 ID</label>
<input type="text" id="id" name="id" class="form-control"
value="1" th:value="${item.Id}" readonly>
</div>
<div>
<label for="itemName">상품명</label>
<input type="text" id="itemName" name="itemName" class="form-control"
value="상품A" th:value="${item.itemName}">
</div>
<div>
<label for="price">가격</label>
<input type="text" id="price" name="price" class="form-control"
value="10000" th:value="${item.price}">
</div>
<div>
<label for="quantity">수량</label>
<input type="text" id="quantity" name="quantity" class="form-control"
value="10" th:value="${item.quantity}">
</div>
<hr class="my-4">
<div class="row">
<div class="col">
<button class="w-100 btn btn-primary btn-lg" type="submit">
저장
</button>
</div>
<div class="col">
<button class="w-100 btn btn-secondary btn-lg"
onclick="location.href='item.html'"
th:onclick="|location.href='@{/basic/items/{itemId}(itemId=${item.id})}'|"
type="button">
취소
</button>
</div>
</div>
</form>
</div>
</body>
</html>
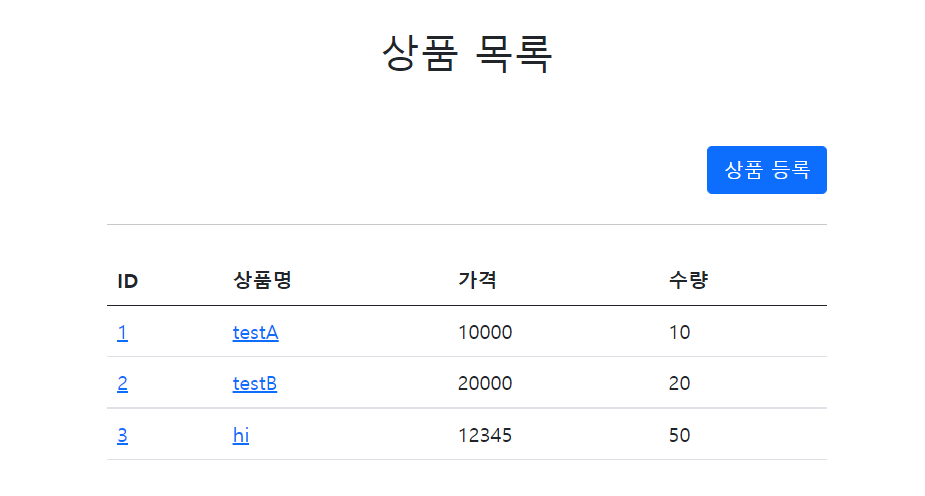