🧩index.jsp
<body>
<a href="">[글 쓰기]</a><br>
<a href="${pageContext.request.contextPath }/board/BoardList.bo">
[글 목록]</a><br>
</body>
🧩boardlist.jsp
- /board/BoardList.bo : 프론트컨트롤러로 요청을 보내고
page=${i}: 파라미터 값으로 내가원하는 페이지를 담아서 보낸다
프론트컨트롤러 거쳐서 보드리스트액션으로 간다
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/functions" prefix="fn" %>
<body>
//넘겨받음
<c:set var="boardList" value="${requestScope.boardList }" />
<c:set var="totalCnt" value="${requestScope.totalCnt }" />
<c:set var="nowPage" value="${requestScope.nowPage }"/>
<c:set var="totalPage" value="${requestScope.totalPage }"/>
<c:set var="startPage" value="${requestScope.startPage }"/>
<c:set var="endPage" value="${requestScope.endPage }"/>
<div>
<table style="width: 900px;border: 1px;">
<tr align="center" valign="middle">
<td><h3>MVC 게시판</h3></td>
</tr>
<tr align="right" valign="middle">
<td>글 개수 : ${totalCnt} 개</td>
</tr>
</table>
<table border="1" style="border-collapse: collapse;border-spacing: 0;width: 900px;" >
<tr align="center" valign="middle">
<th width="8%">번호</th>
<th width="50%">제목</th>
<th width="15%">작성자</th>
<th width="17%">날짜</th>
<th width="10%">조회수</th>
</tr>
<%-- 게시글 작성 --%>
<c:choose>
<%-- 게시글이 있는경우 --%>
<c:when test="${boardList != null and fn:length(boardList) > 0 }">
<c:forEach var="board" items="${boardList }">
<tr align="center" valign="middle"
onmouseover="this.style.background='#bbdefb'"
onmouseout="this.style.background=''" >
<td height="23px;">${board.boardnum }</td>
<td height="23px;">
<a href="${pageContext.request.contextPath }/board/BoardView.bo?boardnum="${board.boardnum }>
${board.boardtitle }
</a>
</td>
<td height="23px;">${board.username }</td>
<td height="23px;">${board.boarddate }</td>
<td height="23px;">${board.boardreadcount }</td>
</tr>
</c:forEach>
</c:when>
<%-- 게시글이 없는경우 --%>
<c:otherwise>
<tr style="height: 50px;">
<td colspan="5" style="text-align: center;">등록된 게시물이 없습니다.</td>
</tr>
</c:otherwise>
</c:choose>
</table>
<br>
<%-- 페이징 처리 --%>
<table style="border: 0px; width: 900px;">
<tr align="center" valign="middle">
<td>
<c:if test="${nowPage > 1 }">
<a href="${pageContext.request.contextPath }/board/BoardList.bo?page=${nowPage - 1}">[<]</a>
</c:if>
<c:forEach var="i" begin="${startPage }" end="${endPage }">
<c:choose>
<%--현재 보고있는 페이지는 a tag 제거 --%>
<c:when test="${i == nowPage }"> [${i }] </c:when>
<c:otherwise>
<a href="${pageContext.request.contextPath }/board/BoardList.bo?page=${i}">[${i }]</a>
</c:otherwise>
</c:choose>
</c:forEach>
<c:if test="${nowPage < totalPage }">
<a href="${pageContext.request.contextPath }/board/BoardList.bo?page=${nowPage + 1}">[>]</a>
</c:if>
</td>
</tr>
</table>
<table style="border: 0px;width: 900px; ">
<tr align="right" valign="middle">
<td><a href="">[글쓰기]</a></td>
</tr>
</table>
</div>
</body>
🧩BoardFrontController.java
protected void doProcess(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String requestURI = req.getRequestURI();
ActionForward forward = null;
switch (requestURI) {
case "/board/BoardList.bo":
forward = new BoardListAction().execute(req, resp);
break;
case "/board/BoardView.bo":
forward = new ActionForward(false,"/board/boardview.jsp");
break;
}
🧩BoardListAction.java
- boardlist : /board/BoardList.bo page=${i}
String temp = req.getParameter("page"); :
최초에는 null 값 그 다음에는 요청한 파라미터값이 담긴다
요청한 페이지값에 따라서 페이징 처리가 된다
public class BoardListAction implements Action {
@Override
public ActionForward execute(HttpServletRequest req, HttpServletResponse resp) {
ActionForward forward = new ActionForward();
BoardDAO bdao = new BoardDAO();
int totalCnt = bdao.getBoardCnt();
String temp = req.getParameter("page");
int page = 0;
page = temp == null ? 1 : Integer.parseInt(temp);
int pageSize = 10;
int endRow = page * 10;
int startRow = endRow - 9;
int startPage = (page -1) / pageSize * pageSize + 1;
int endPage = startPage + pageSize -1;
int totalPage = (totalCnt -1)/pageSize + 1;
endPage = endPage > totalPage? totalPage : endPage;
req.setAttribute("totalPage", totalPage);
req.setAttribute("nowPage", page);
req.setAttribute("startPage", startPage);
req.setAttribute("endPage", endPage);
req.setAttribute("boardList", bdao.getBoardList(startRow, endRow));
req.setAttribute("totalCnt", totalCnt);
forward.setRedirect(false);
forward.setPath("/board/boardlist.jsp" );
return forward;
}
}
🧩BoardDAO
public class BoardDAO {
SqlSessionFactory factory = SqlMapConfig.getFactory();
SqlSession sqlsession;
public BoardDAO() {
sqlsession = factory.openSession(true);
}
public List<BoardDTO> getBoardList(int startRow, int endRow) {
HashMap<String, Integer> datas = new HashMap<>();
datas.put("startRow", startRow);
datas.put("endRow", endRow);
List<BoardDTO> boardList = sqlsession.selectList("Board.getBoardList", datas);
return boardList;
}
public int getBoardCnt() {
return sqlsession.selectOne("Board.getBoardCnt");
}
}
🧩board.xml
<mapper namespace="Board">
<select id="getBoardList" parameterType="hashmap" resultType="boarddto">
SELECT * from( SELECT rownum R, D.*
FROM (SELECT * FROM TBL_BOARD tb ORDER BY BOARDNUM DESC) D) B
WHERE B.R BETWEEN #{startRow} AND
#{endRow}
</select>
<select id="getBoardCnt" resultType="_int">
SELECT count(*) FROM TBL_BOARD
</select>
</mapper>
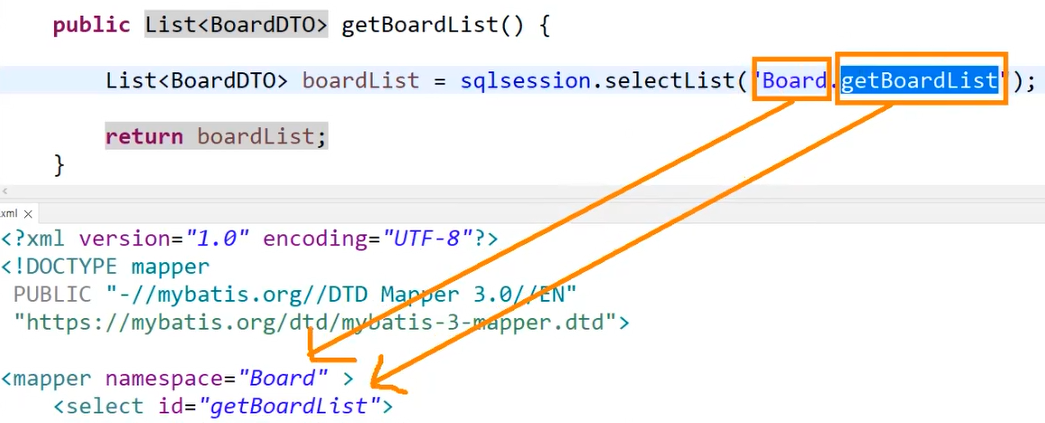
🧩DB
CREATE TABLE tbl_board(
boardnum number(10) PRIMARY KEY,
boardtitle varchar2(300),
boardcontents varchar2(4000),
username varchar2(300),
boarddate DATE,
boardreadcount number(10)
);
SELECT * FROM tbl_board;
CREATE SEQUENCE board_seq
START WITH 1
INCREMENT BY 1;
INSERT INTO TBL_BOARD
VALUES (board_seq.nextval, '첫 번째글', '첫번째 내용', 'student', sysdate, 0)
;
INSERT INTO TBL_BOARD
VALUES (board_seq.nextval, '두 번째글', '두번째 내용', 'student', sysdate, 0)
;
INSERT INTO TBL_BOARD
VALUES (board_seq.nextval, '세 번째글', '세번째 내용', 'student', sysdate, 0)
;
SELECT count(*) FROM TBL_BOARD tb ;
COMMIT ;
🧩결과
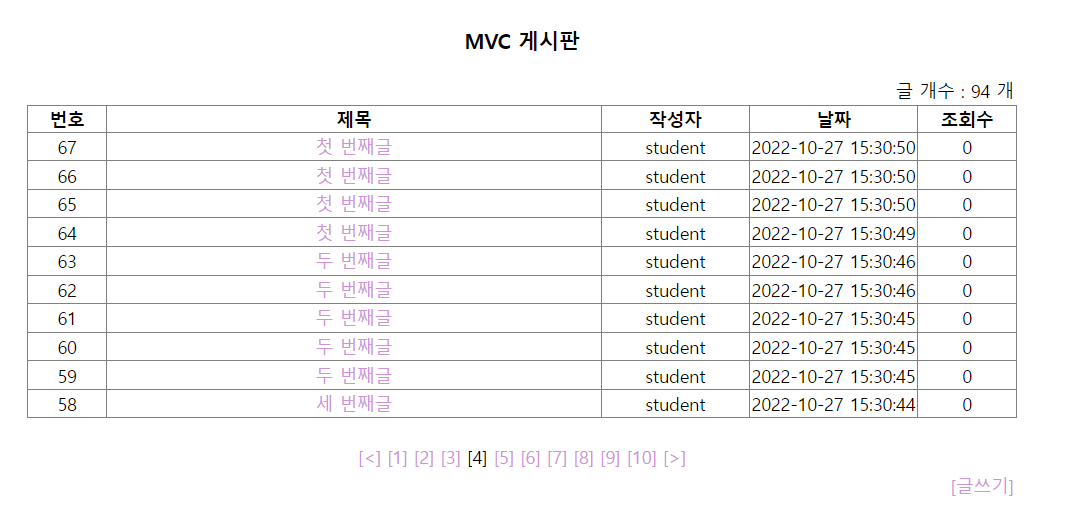