1. enums, types
export enum Status {
Initialized = "Initialized",
Pending = "Pending",
Complete = "Complete",
}
enum Color {
Red,
Blue,
Black,
}
enum Car {
Sedan,
Truck,
Coupe,
}
enum ProgrammingLanguage {
TypeScript = "TypeScript",
JavaScript = "JavaScript",
Python = "Python",
Golang = "Golang",
}
type Customer = {
firstName: string;
lastName: string;
};
2. 문제 and 정답
type Inventory = {
[key in keyof typeof Car]? : keyof typeof Color
};
const inventory: Inventory = {
Sedan: "Red",
Truck: "Black",
};
type TColor = {
[key in keyof typeof Color] : string[]
};
const colors : TColor = {
Red: ["red"],
Blue: [],
Black: ["obsidian", "ink"],
};
type ColorKey = keyof typeof Color;
const someRose: ColorKey = "Red";
const someSky: ColorKey = "Blue";
const someTerminal: ColorKey = "Black";
export function getSum(number1: number, number2: number): number {
return number1 + number2
}
export function isStatusPending(status: Status): boolean {
if(status === Status.Pending) return true
else return false
}
export function isStatusComplete(status: Status): boolean {
if(status === Status.Complete) return true
else return false
}
type StatusObject = {
[key in Status] : string
};
export function getStatusObject(): StatusObject {
const values = Object.values(Status)
const myObject = {} as StatusObject
values.forEach((key) => {
myObject[key] = key
})
return myObject
}
export function getCars(): Car[] {
return [Car.Sedan, Car.Truck, Car.Coupe]
}
type TProgrammingLanguages = {
[key in ProgrammingLanguage as number]: key
}[]
export function getProgrammingLanguages(): TProgrammingLanguages {
const entries = Object.entries(ProgrammingLanguage)
const arr = entries.map(([key, value]) => {
return {[key.length]: value}
})
return arr
}
type TOrder = {
firstCar: {
status: Status,
color: Color,
availableColors: Color[],
orderedBy: Customer
},
secondCar: {
status: Status,
color: Color,
availableColors: Color[],
orderedBy: Customer
}
};
const orders: TOrder = {
firstCar: {
status: Status.Initialized,
color: Color.Black,
availableColors: [Color.Red],
orderedBy: {
firstName: "jane",
lastName: "doe",
},
},
secondCar: {
status: Status.Complete,
color: Color.Blue,
availableColors: [Color.Black],
orderedBy: {
firstName: "john",
lastName: "doe",
},
},
};
type TCustomerCar = {
1: {
customerLastName: Customer['lastName'],
car: Car,
carColor: keyof typeof Color
},
2: {
customerLastName: Customer['lastName'],
car: Car,
carColor: keyof typeof Color
}
};
const customerCars: TCustomerCar = {
1: {
customerLastName: "skywalker",
car: Car.Coupe,
carColor: "Red",
},
2: {
customerLastName: "jedi",
car: Car.Sedan,
carColor: "Blue",
},
};
3. fork한 파일 pull request하기
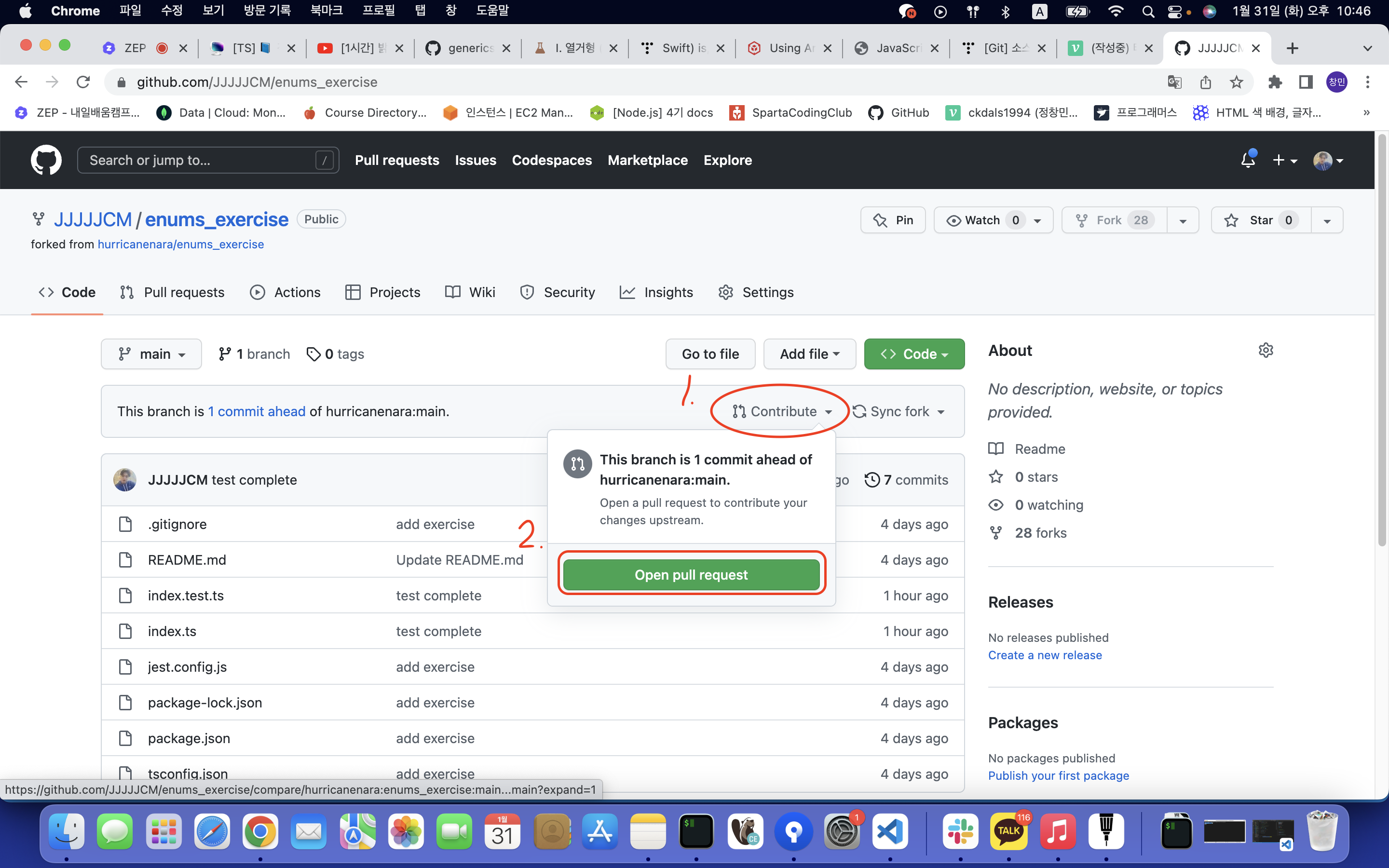