값 타입 vs. 참조 타입
- 이 두 가지 타입은 데이터를 저장하고 전달하는 방식에서 차이가 있음.
값 타입(value type)
- 원시 값에 해당.
- 데이터를 복사해서 저장하고 변수에 값이 직접 저장 됨.
let x = 10;
let y = x;
x = 20;
console.log(x);
console.log(y);
참조 타입(reference type)
- 객체에 해당.
- 데이터를 메모리에 저장하고, 변수에는 해당 데이터의 참조(메모리 주소)가 저장.
- 얕은 복사(shallow copy): 객체를 가진 변수를 다른 변수에 할당하면, 원본의 참조 값(주소)이 복사되어 전달 => 여러 변수가 동일한 데이터를 공유.
- 따라서, 참조 타입은 유일한 값을 가진다 라고 함.
let obj1 = { name: "Alice" };
let obj2 = obj1;
obj1.name = "Bob";
console.log(obj1.name);
console.log(obj2.name);
Deep Copy(깊은 복사)
- 참조 타입을 값 타입 처럼 복사 하는 방법.
- 객체를 Deep copy하여 새로운 객체를 만드는 것은 불변성을 부여하는데 효과적인 방법 중 하나.
- 새 객체는 원본 객체와 완전히 분리되어 있으므로 원본 객체의 수정이 새 객체에 영향을 미치지 않음.
확산연산자(spread)
const test = { a: { b: 1 } };
const testCopy = { ...test };
console.log(test === testCopy);
console.log(test.a === testCopy.a);
const testCopy2 = { ...test, a: 3 };
console.log(testCopy2);
console.log(testCopy2 === test);
console.log(testCopy2 === testCopy);
const test = { a: { b: 1 }, b: 3, c: 5 };
const testCopy = { ...test, a: { ...test.a } };
console.log(test === testCopy);
console.log(test.a === testCopy.a);
Object.assign()
Object.assign(target, ...sources)
target
: 복사 받을 목표 객체.
sources
: 복사 할 소스 객체.
- 복사 받은 목표 객체를 반환.
- 1depth.
const test = { a: { b: 1 } };
const testCopy = Object.assign({}, test);
console.log(test === testCopy);
console.log(test.a === testCopy.a);
const testCopy2 = Object.assign({}, test, { a: 3 });
console.log(testCopy2);
console.log(testCopy2 === test);
console.log(testCopy2 === testCopy);
const test = { a: { b: 1 }, b: 3, c: 5 };
const testCopy = Object.assign({}, test, { a: { ...test.a } });
console.log(test === testCopy);
console.log(test.a === testCopy.a);
V8 엔진에서 작동 방식
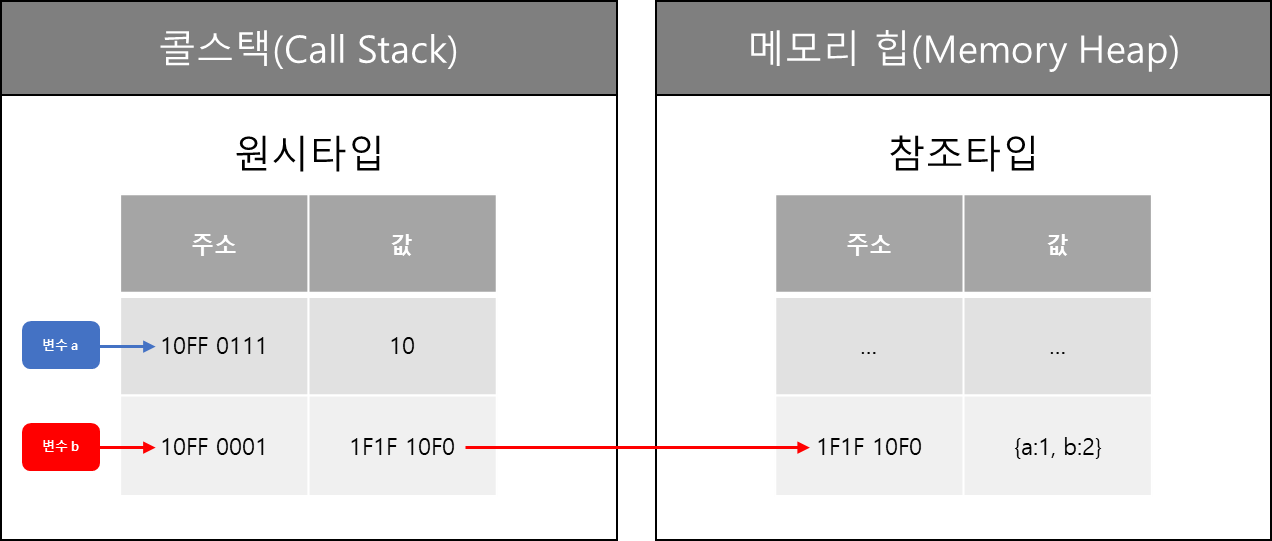