백준
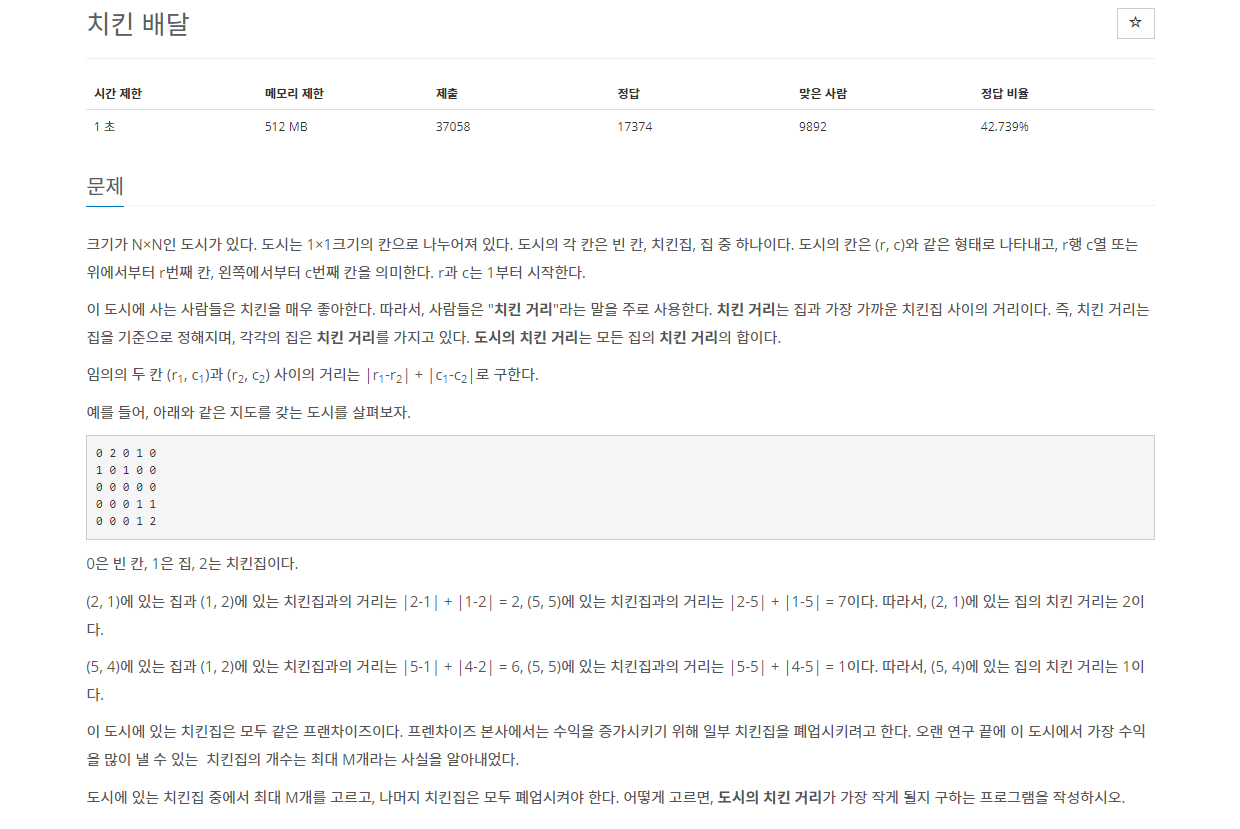
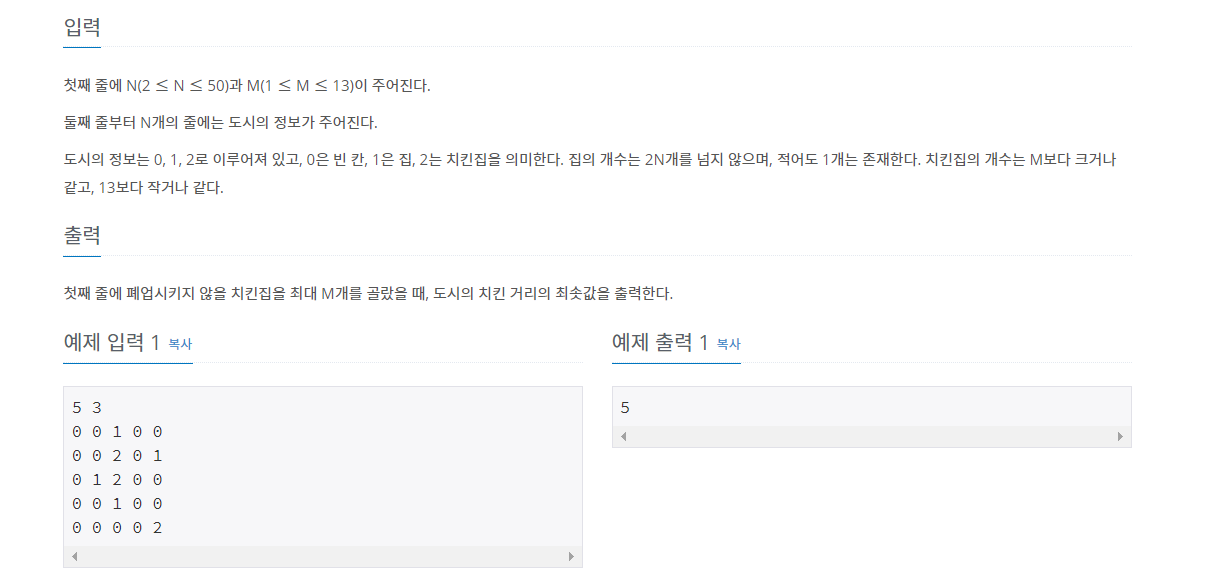
from itertools import combinations
n, m = map(int, input().split())
board = [list(map(int, input().split())) for _ in range(n)]
house = []
chicken = []
for i in range(n):
for j in range(n):
if board[i][j] == 1: house.append((i, j))
elif board[i][j] == 2: chicken.append((i, j))
value = float('inf')
for ch in combinations(chicken, m):
plus = 0
for home in house:
plus += min([abs(home[0]-i[0])+abs(home[1]-i[1]) for i in ch])
if value <= plus: break
if plus < value: value = plus
print(value)
from itertools import combinations
n, m = map(int, input().split())
chicken, house = [], []
for r in range(n):
data = list(map(int, input().split()))
for c in range(n):
if data[c] == 1:
house.append((r, c))
elif data[c] == 2:
chicken.append((r, c))
candidates = list(combinations(chicken, m))
def get_sum(candidate):
result = 0
for hx, hy in house:
temp = 1e9
for cx, cy in candidate:
temp = min(temp, abs(hx - cx) + abs(hy - cy))
result += temp
return result
result = 1e9
for candidate in candidates:
result = min(result, get_sum(candidate))
print(result)
2. C++
#include <bits/stdc++.h>
using namespace std;
int n, m;
int arr[50][50];
vector<pair<int, int> > chicken;
vector<pair<int, int> > house;
int getSum(vector<pair<int, int> > candidates) {
int result = 0;
for (int i = 0; i < house.size(); i++) {
int hx = house[i].first;
int hy = house[i].second;
int temp = 1e9;
for (int j = 0; j < candidates.size(); j++) {
int cx = candidates[j].first;
int cy = candidates[j].second;
temp = min(temp, abs(hx - cx) + abs(hy - cy));
}
result += temp;
}
return result;
}
int main(void) {
cin >> n >> m;
for (int r = 0; r < n; r++) {
for (int c = 0; c < n; c++) {
cin >> arr[r][c];
if (arr[r][c] == 1) house.push_back({r, c});
else if (arr[r][c] == 2) chicken.push_back({r, c});
}
}
vector<bool> binary(chicken.size());
fill(binary.end() - m, binary.end(), true);
int result = 1e9;
do {
vector<pair<int, int> > now;
for (int i = 0; i < chicken.size(); i++) {
if (binary[i]) {
int cx = chicken[i].first;
int cy = chicken[i].second;
now.push_back({cx, cy});
}
}
result = min(result, getSum(now));
} while(next_permutation(binary.begin(), binary.end()));
cout << result << '\n';
}