프로그래머스
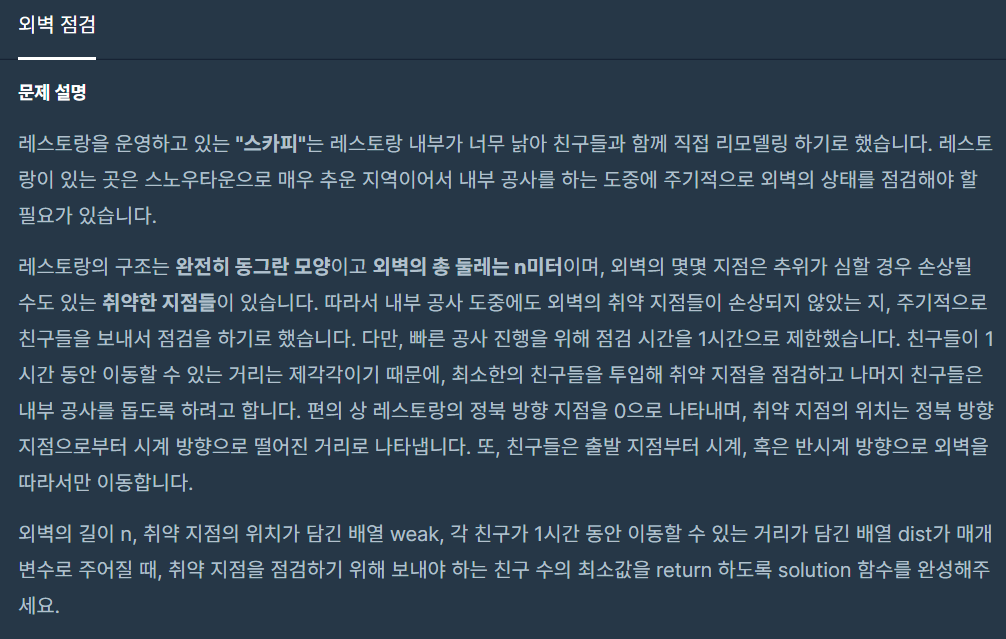
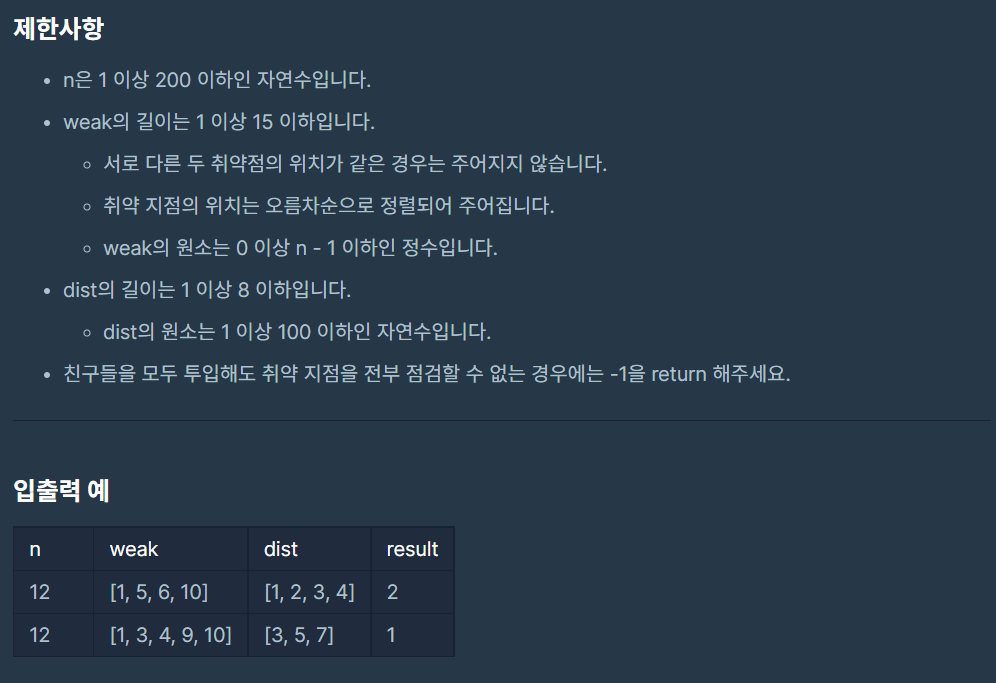
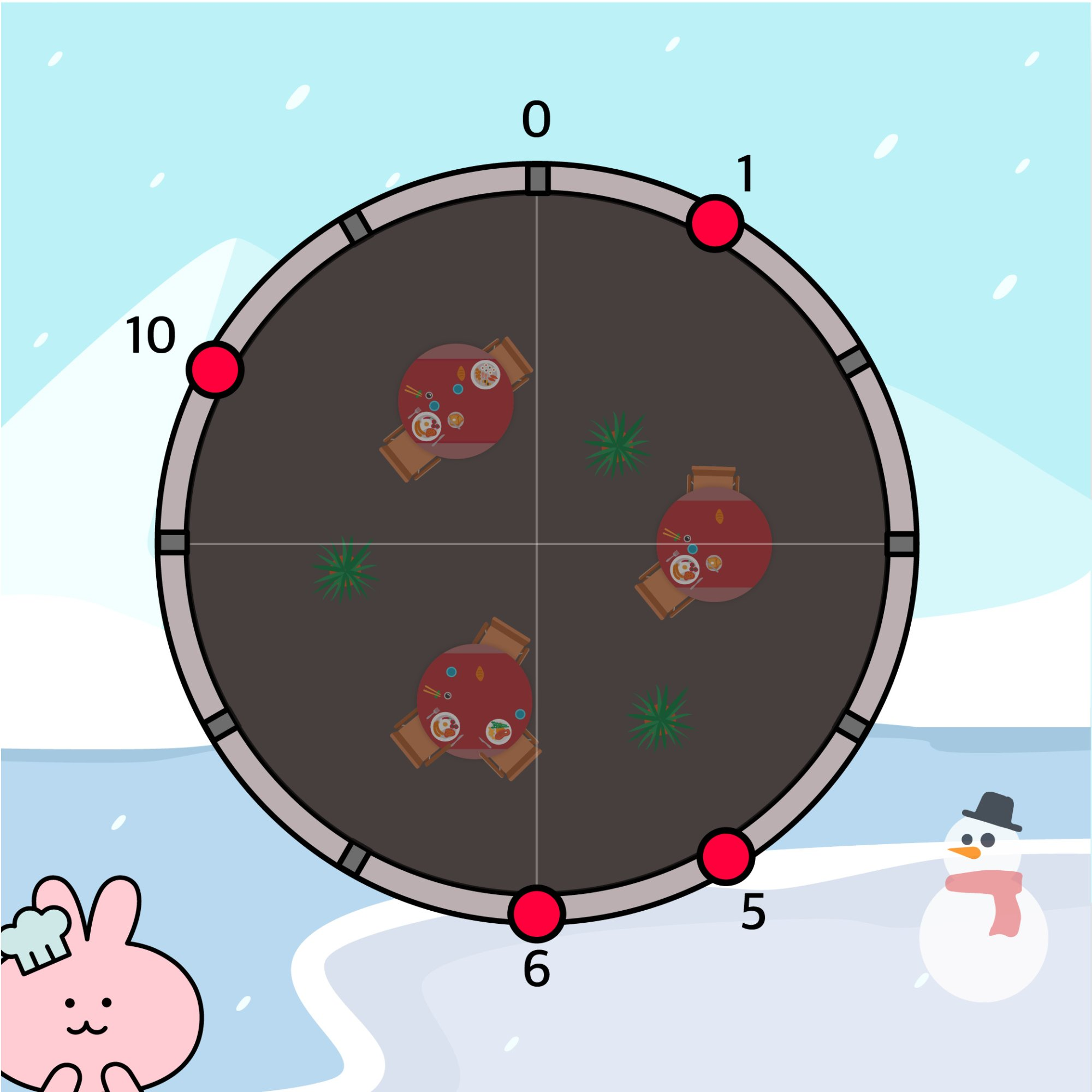
1. Python
from itertools import permutations
def solution(n, weak, dist):
length = len(weak)
for i in range(length):
weak.append(weak[i] + n)
answer = len(dist) + 1
for start in range(length):
for friends in list(permutations(dist, len(dist))):
count = 1
position = weak[start] + friends[count - 1]
for index in range(start, start + length):
if position < weak[index]:
count += 1
if count > len(dist):
break
position = weak[index] + friends[count - 1]
answer = min(answer, count)
if answer >len(dist):
return -1
else:
return answer
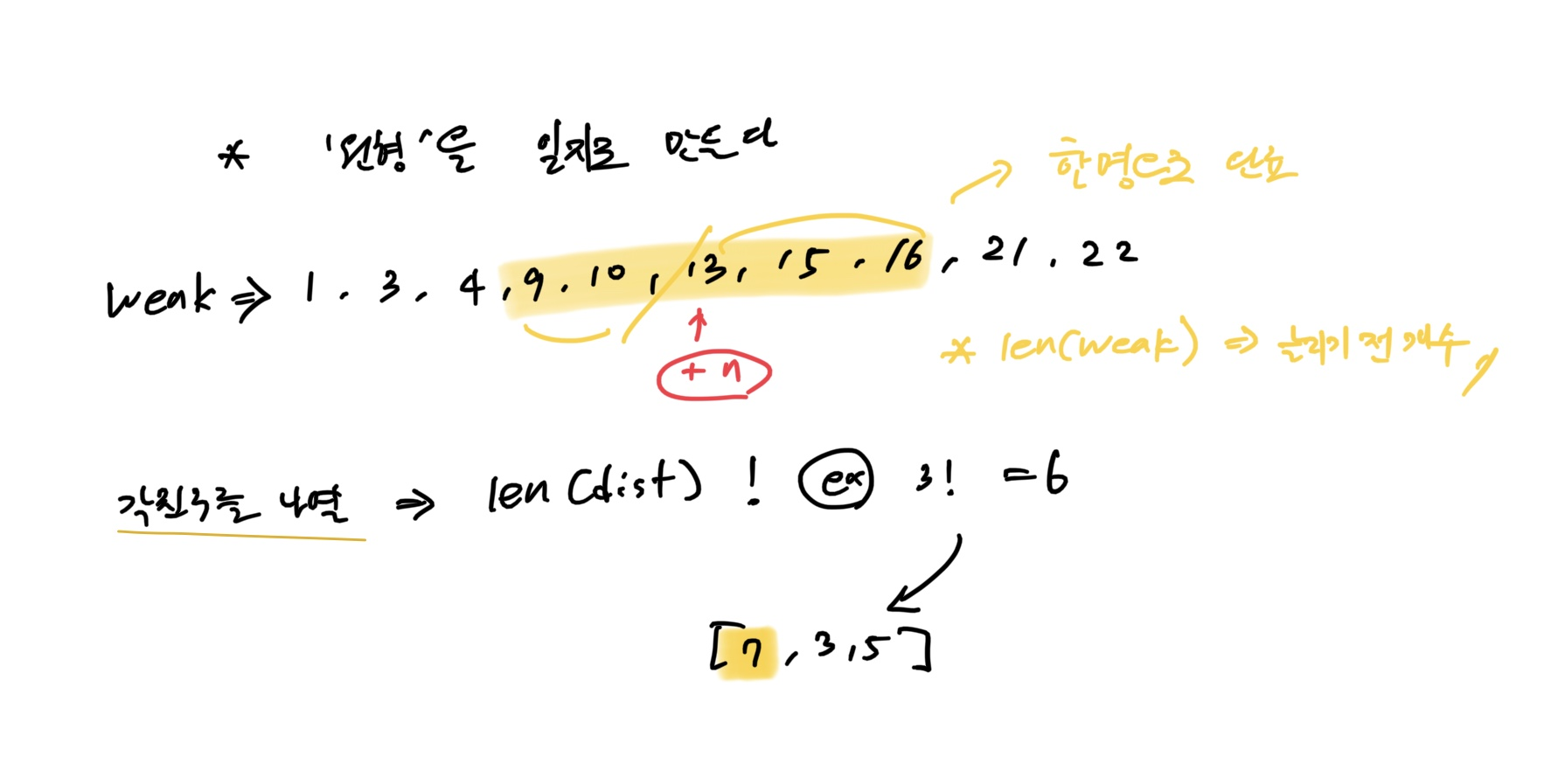
2. C++
#include <bits/stdc++.h>
using namespace std;
int solution(int n, vector<int> weak, vector<int> dist) {
int length = weak.size();
for (int i = 0; i < length; i++) {
weak.push_back(weak[i] + n);
}
int answer = dist.size() + 1;
for (int start = 0; start < length; start++) {
do {
int cnt = 1;
int position = weak[start] + dist[cnt - 1];
for (int index = start; index < start + length; index++) {
if (position < weak[index]) {
cnt += 1;
if (cnt > dist.size()) {
break;
}
position = weak[index] + dist[cnt - 1];
}
}
answer = min(answer, cnt);
} while(next_permutation(dist.begin(), dist.end()));
}
if (answer > dist.size()) {
return -1;
}
return answer;
}