프로그래머스
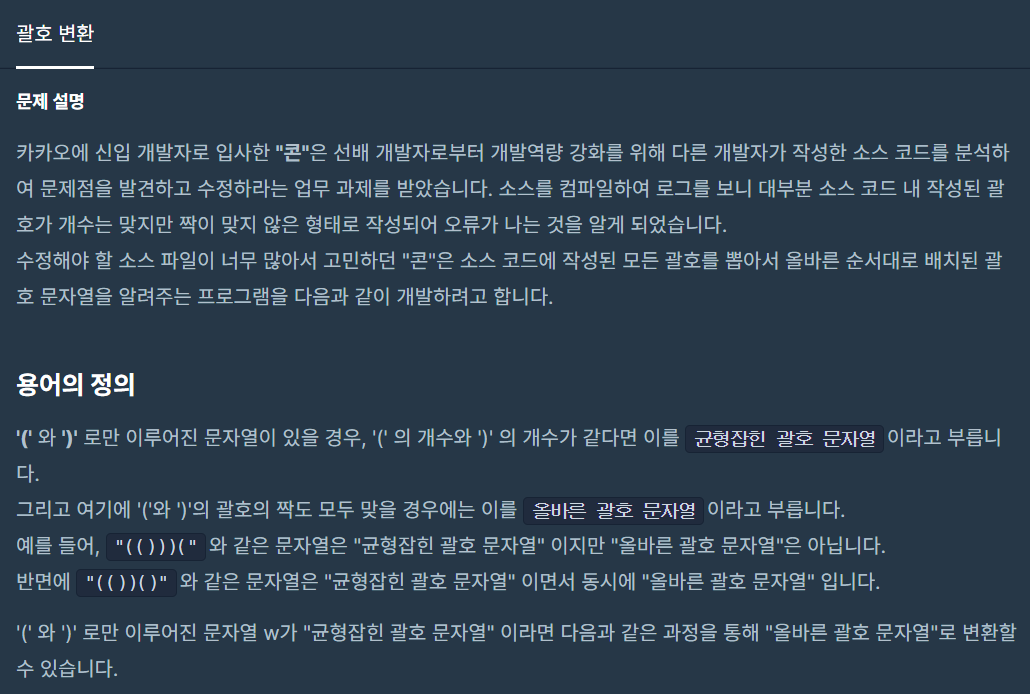
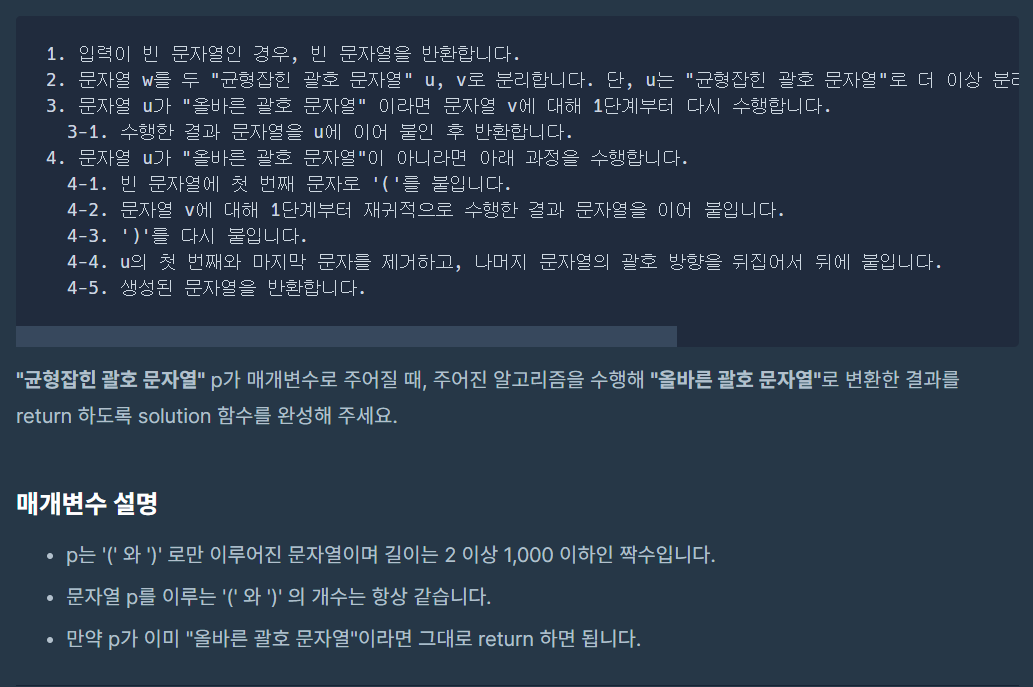
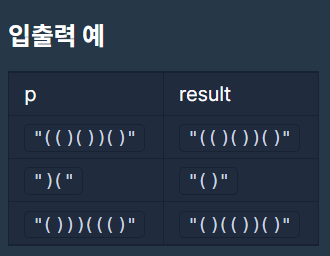
1. 구현
def balanced_index(p):
count = 0
for i in range(len(p)):
if p[i] == '(':
count += 1
else:
count -= 1
if count == 0:
return i
def check_proper(p):
count = 0
for i in p:
if i == '(':
count += 1
else:
if count == 0:
return False
count -= 1
return True
def solution(p):
answer = ''
if p == '':
return answer
index = balanced_index(p)
u = p[:index + 1]
v = p[index + 1:]
if check_proper(u):
answer = u + solution(v)
else:
answer = '('
answer += solution(v)
answer += ')'
u = list(u[1:-1])
for i in range(len(u)):
if u[i] == '(':
u[i] = ')'
else:
u[i] = '('
answer += ''.join(u)
return answer
2. C++
#include <bits/stdc++.h>
using namespace std;
int balancedIndex(string p) {
int count = 0;
for (int i = 0; i < p.size(); i++) {
if (p[i] == '(') count += 1;
else count -= 1;
if (count == 0) return i;
}
return -1;
}
bool checkProper(string p) {
int count = 0;
for (int i = 0; i < p.size(); i++) {
if (p[i] == '(') count += 1;
else {
if (count == 0) {
return false;
}
count -= 1;
}
}
return true;
}
string solution(string p) {
string answer = "";
if (p == "") return answer;
int index = balancedIndex(p);
string u = p.substr(0, index + 1);
string v = p.substr(index + 1);
if (checkProper(u)) {
answer = u + solution(v);
}
else {
answer = "(";
answer += solution(v);
answer += ")";
u = u.substr(1, u.size() - 2);
for (int i = 0; i < u.size(); i++) {
if (u[i] == '(') u[i] = ')';
else u[i] = '(';
}
answer += u;
}
return answer;
}