프로그래머스
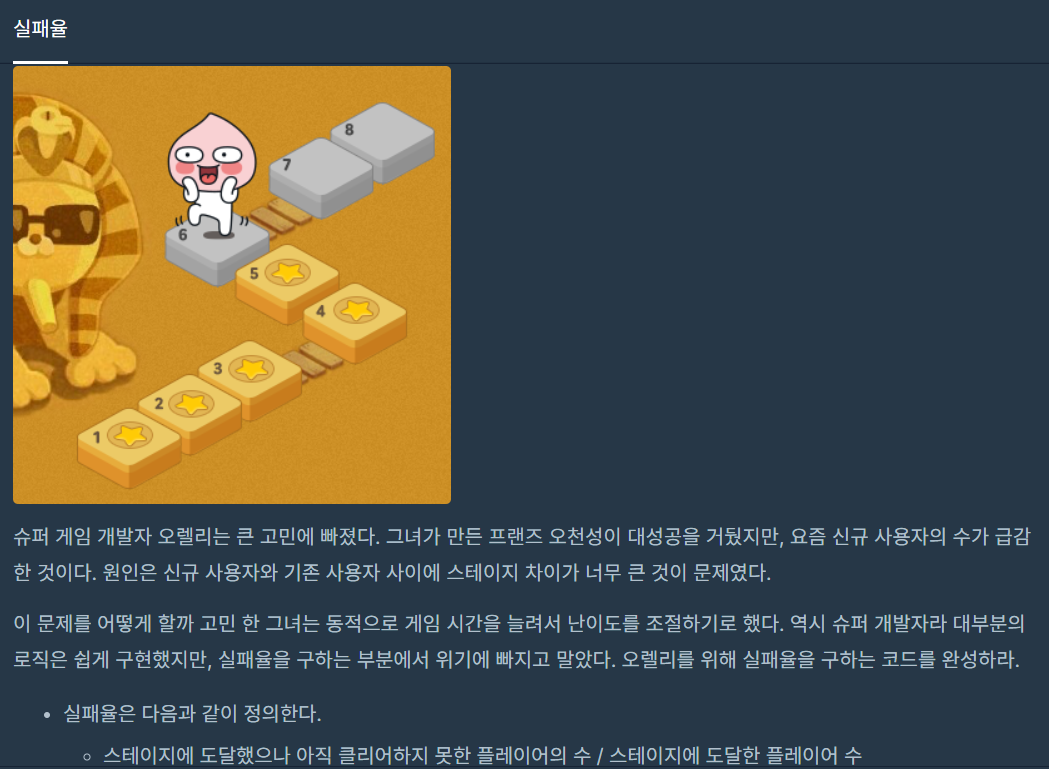
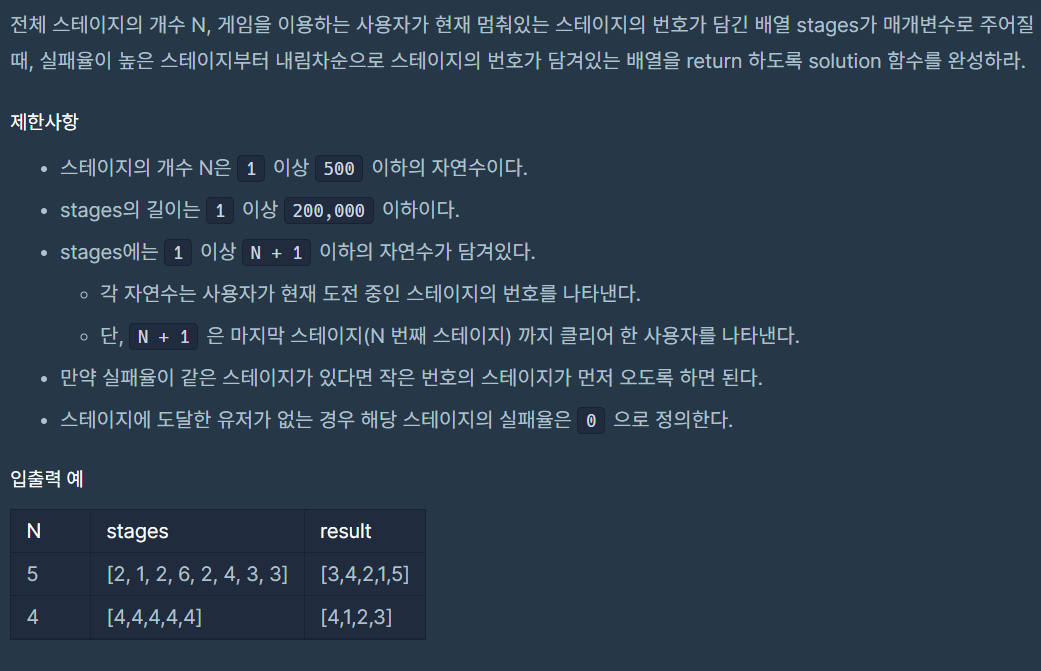
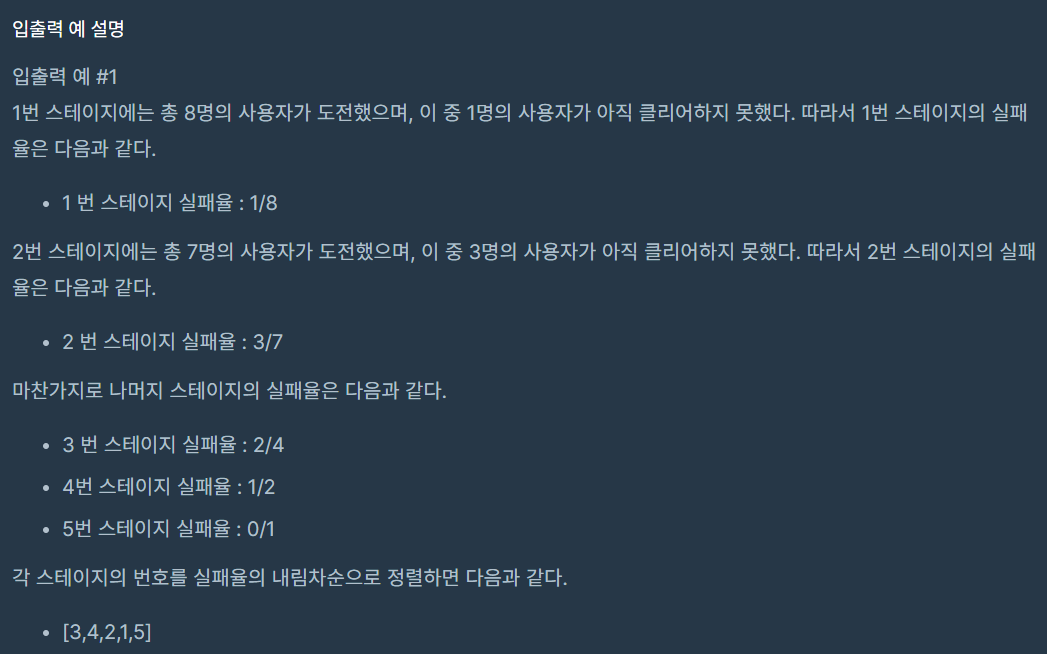
1. Python
런타임 에러
def solution(N, stages):
count = [0] * (N + 2)
rate = []
result = []
for stage in stages:
count[stage] += 1
for i in range(1, N + 1):
r = count[i]/sum(count[i:])
rate.append((r, i) )
rate.sort(key = lambda x: (-(x[0])))
for i in range(0, N):
result.append(rate[i][1])
return result
정답
def solution(N, stages):
answer = []
length = len(stages)
for i in range(1, N + 1):
count = stages.count(i)
if length == 0:
fail = 0
else:
fail = count / length
answer.append((i, fail))
length -= count
answer = sorted(answer, key=lambda t: t[1], reverse=True)
answer = [i[0] for i in answer]
return answer
2. C++
#include <bits/stdc++.h>
using namespace std;
bool compare(pair<int, double> a, pair<int, double> b) {
if (a.second == b.second) return a.first < b.first;
return a.second > b.second;
}
vector<int> solution(int N, vector<int> stages) {
vector<pair<int, double> > v;
vector<int> answer;
int length = stages.size();
for (int i = 1; i <= N; i++) {
int cnt = count(stages.begin(), stages.end(), i);
double fail = 0;
if (length >= 1) {
fail = (double) cnt / length;
}
v.push_back({i, fail});
length -= cnt;
}
sort(v.begin(), v.end(), compare);
for (int i = 0; i < N; i++) {
answer.push_back(v[i].first);
}
return answer;
}