백준
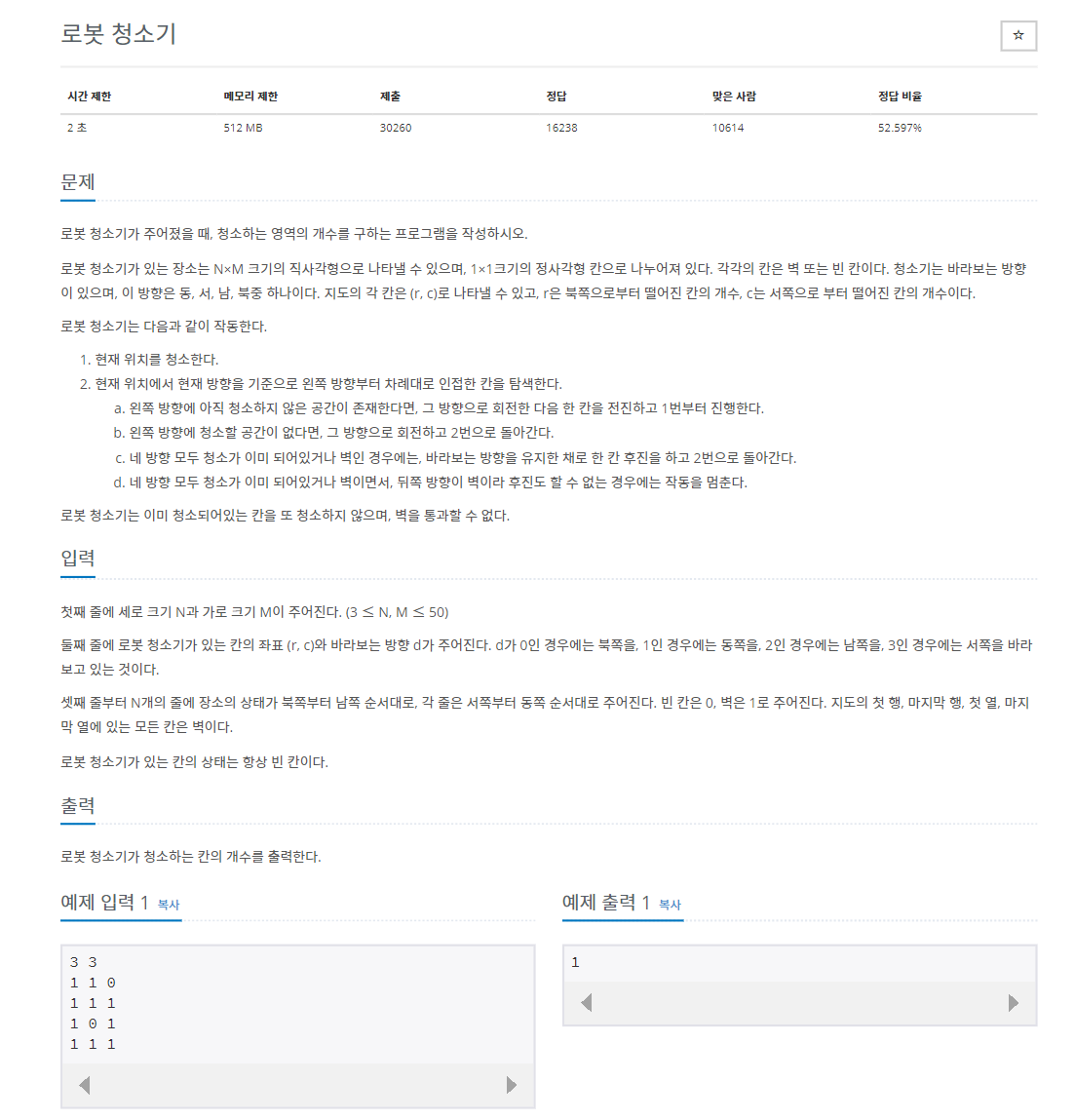
1. Python
import sys
from collections import deque
input = sys.stdin.readline
n, m = map(int, input().split())
r, c, d = map(int, input().split())
data = [list(map(int, input().split())) for _ in range(n)]
dx = [-1, 0, 1, 0]
dy = [0, 1, 0, -1]
def bfs(x, y, d):
count = 1
q = deque()
q.append((x, y, d))
data[x][y] = 2
while q:
x, y, d = q.popleft()
cur = d
for i in range(4):
cur = (cur - 1) % 4
nx = x + dx[cur]
ny = y + dy[cur]
if 0 <= nx < n and 0 <= ny < m and data[nx][ny] == 0:
count+= 1
data[nx][ny] = 2
q.append((nx, ny, cur))
break
elif i == 3:
cur = (d + 2) % 4
nx = x + dx[cur]
ny = y + dy[cur]
if data[nx][ny] == 1:
return count
else:
q.append((nx, ny, d))
return count
print(bfs(r, c, d))