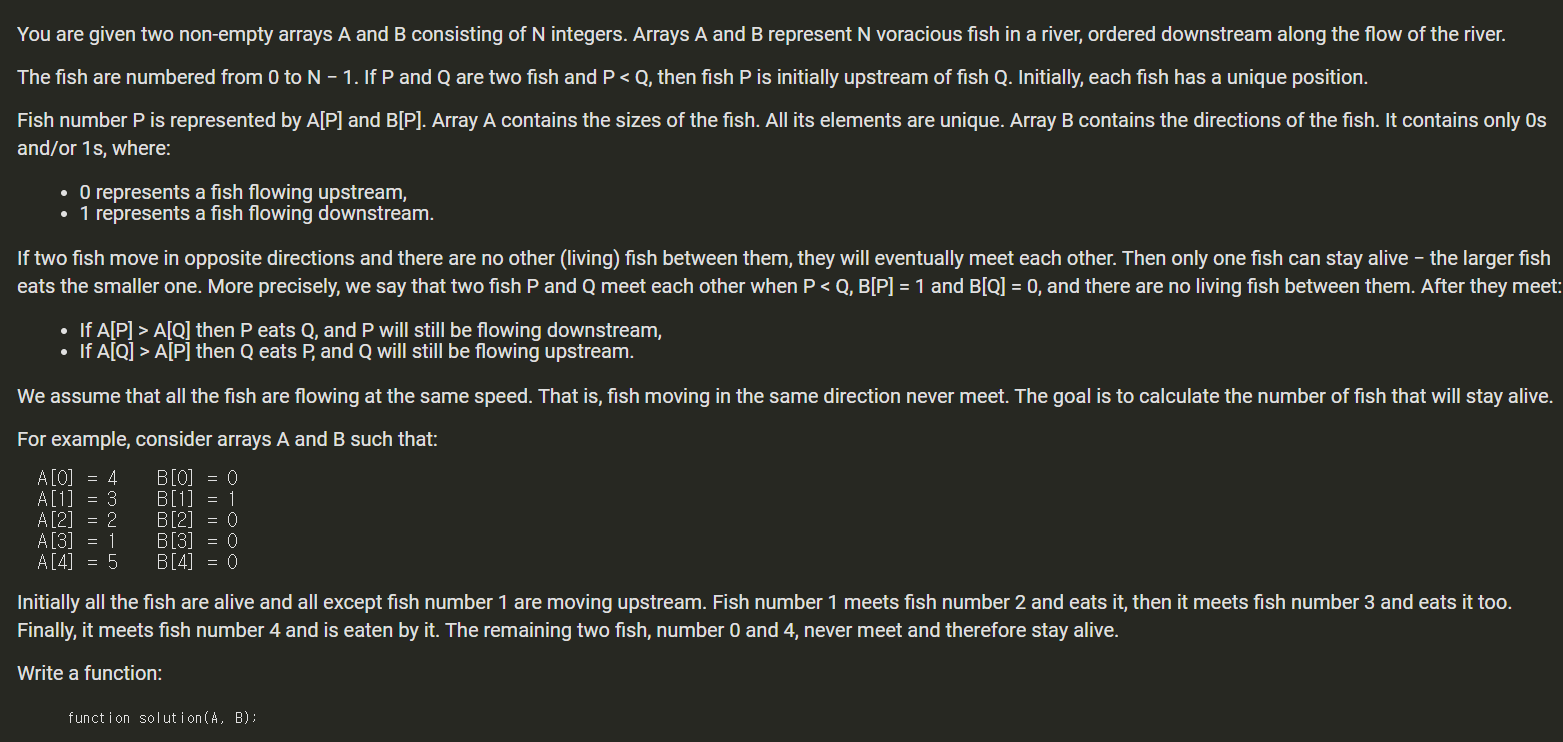
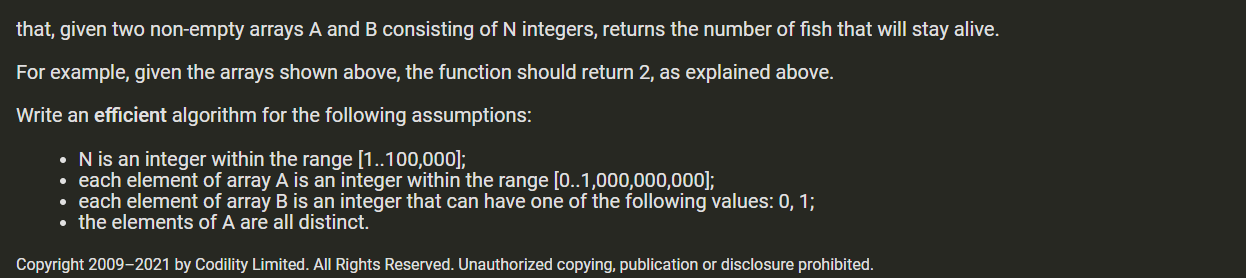
1. JavaScript
function solution(A, B) {
let downFish = [];
let survived= 0;
for(let i = 0; i < A.length; i++) {
if(B[i] === 1) {
downFish.push(i);
} else {
while(downFish.length !== 0) {
const downFishIdx = downFish.pop();
if(A[downFishIdx] > A[i]) {
downFish.push(downFishIdx);
break;
}
}
if(downFish.length === 0) survived++;
}
}
survived += downFish.length;
return survived;
}
2. Python
def solution(A, B):
stack = []
cnt = 0
for i in range(len(A)):
if B[i] == 1:
stack.append(A[i])
else:
while stack:
f = stack[-1]
if f > A[i]:
break
else:
stack.pop()
if not stack:
cnt += 1
return cnt + len(stack)