프로그래머스
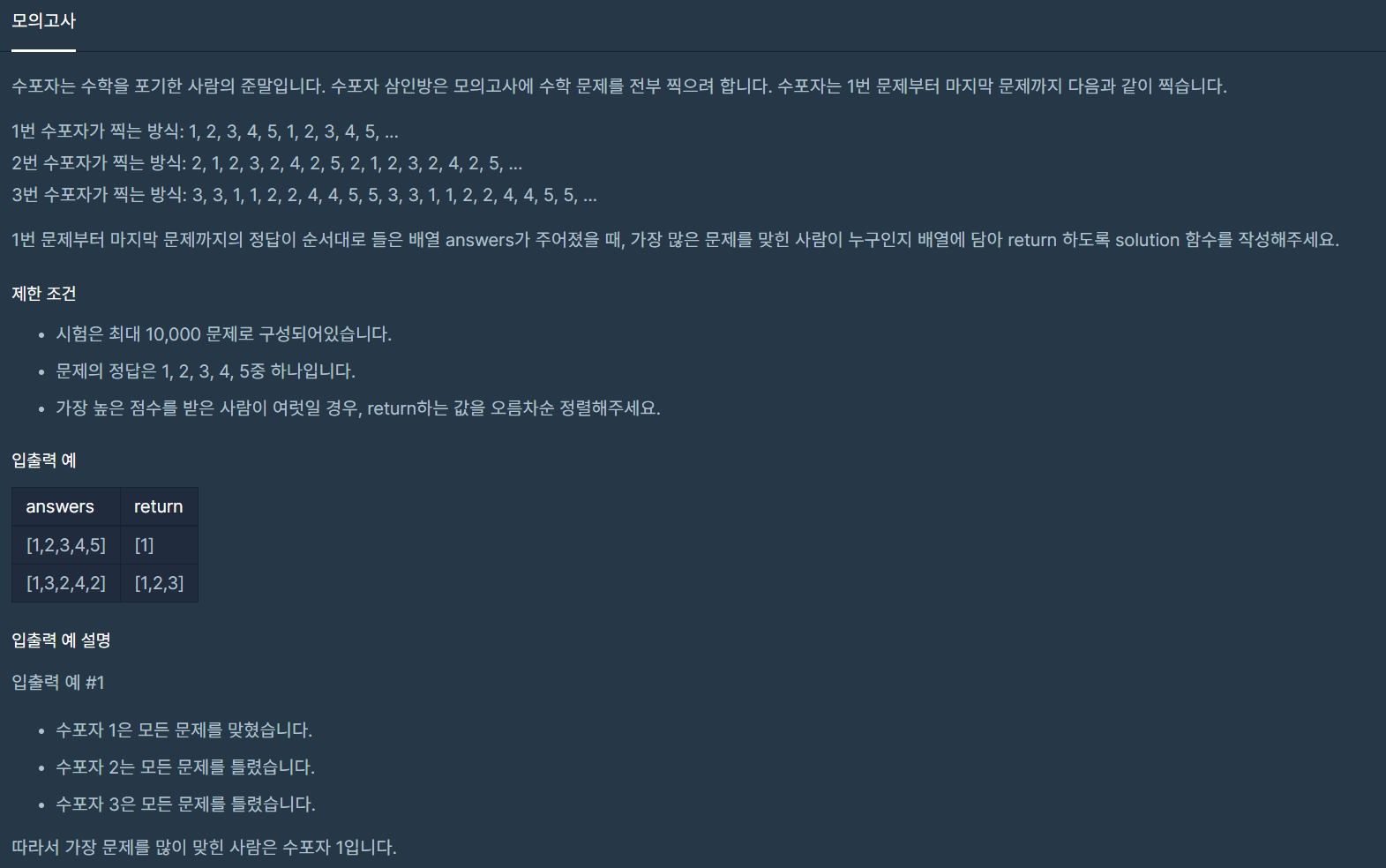
1. Python
def solution(answers):
dict = {
"one": [1, 2, 3, 4, 5],
"two": [2, 1, 2, 3, 2, 4, 2, 5],
"three":[3, 3, 1, 1, 2, 2, 4, 4, 5, 5]
}
count = [0, 0, 0]
for i in range(len(answers)):
if dict["one"][i%5] == answers[i]:
count[0] += 1
if dict["two"][i%8] == answers[i]:
count[1] += 1
if dict["three"][i%10] == answers[i]:
count[2] += 1
answer = []
m = max(count)
for i in range(len(count)):
if count[i] == m:
answer.append(i+1)
return answer
2. C++
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
vector<int> solution(vector<int> answers) {
vector<int> answer;
int one[5] = {1, 2, 3, 4, 5};
int two[8] = {2, 1, 2, 3, 2, 4, 2, 5};
int three[10] = {3, 3, 1, 1, 2, 2, 4, 4, 5, 5};
int count [3] = {0, 0, 0};
int i;
for(int i = 0; i < answers.size(); i++){
if (one[i % 5] == answers[i]){
count[0]++;
}
if(two[i % 8] == answers[i]){
count[1]++;
}
if(three[i % 10] == answers[i]){
count[2]++;
}
}
int m = *max_element(count, count+3);
for(i = 0; i < 3; i++){
if(count[i] == m)
answer.push_back(i+1);
}
return answer;
}
3. JavaScript
function solution(answers) {
let one = [1, 2, 3, 4, 5];
let two = [2, 1, 2, 3, 2, 4, 2, 5];
let three = [3, 3, 1, 1, 2, 2, 4, 4, 5, 5];
let count = [0, 0, 0];
for (let i = 0; i < answers.length; i++){
if (one[i % 5] == answers[i]){
count[0]++;
}
if(two[i % 8] == answers[i]){
count[1]++;
}
if(three[i % 10] == answers[i]){
count[2]++;
}
}
let m = Math.max.apply(null,count);
return count.map((x, i) => m == x ? i+1 : null ).filter(
(element, i) => element != null);
}