프로그래머스
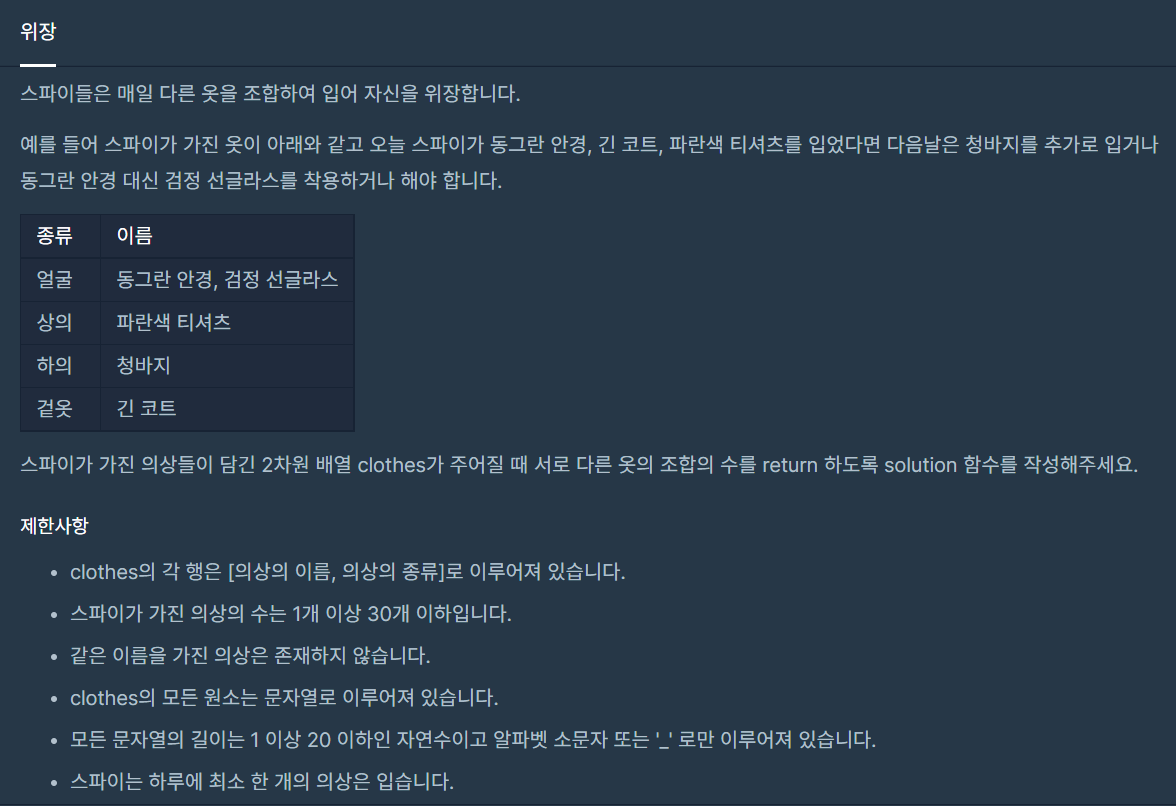
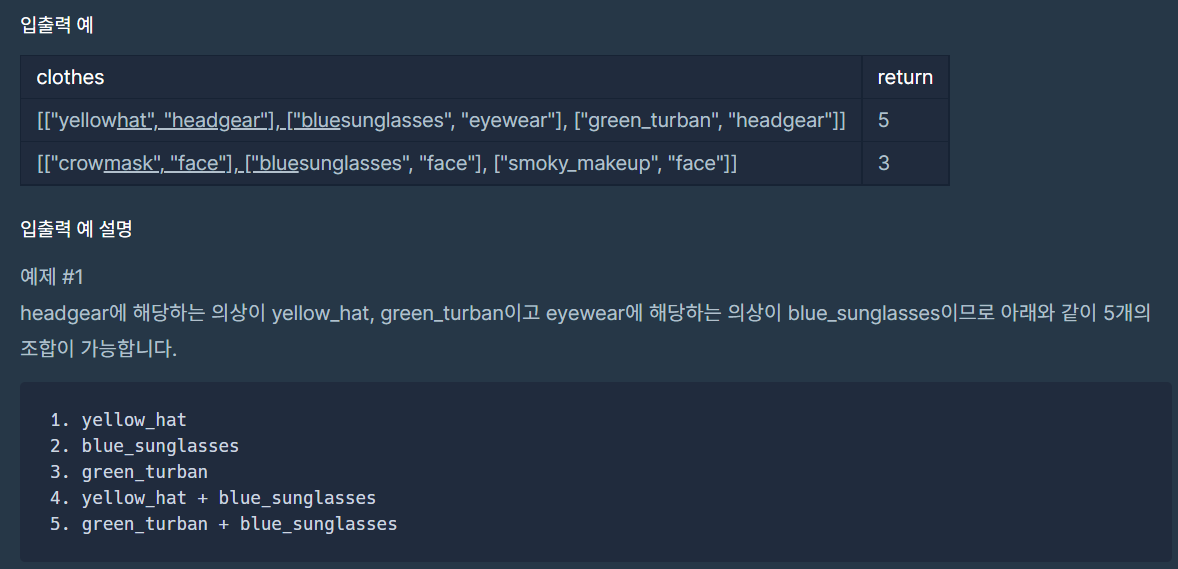
1. Python
from collections import Counter
def solution(clothes):
answer = 1
dict = Counter()
for a, b in clothes:
dict[b] += 1
for k, v in dict.items():
answer *= (v + 1)
return answer - 1
2. C++
#include <string>
#include <vector>
#include <unordered_map>
using namespace std;
int solution(vector<vector<string>> clothes) {
int answer = 1;
unordered_map <string, int> dict;
for(int i = 0; i < clothes.size(); i++)
dict[clothes[i][1]]++;
for(auto it = dict.begin(); it != dict.end(); it++)
answer *= (it->second+1);
return answer - 1;
}
3. JavaScript
function solution(clothes) {
var answer = 1;
let dict = {};
for(let [a, b] of clothes){
if (!dict.hasOwnProperty(b)){
dict[b] = 1;
}else{
dict[b]++;
}
}
for(let a in dict){
answer *= (dict[a] + 1)
}
return answer - 1;
}