import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a = sc.nextInt();
int b = sc.nextInt();
System.out.println(a + b);
}
}
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a = sc.nextInt();
int b = sc.nextInt();
int c = sc.nextInt();
System.out.println((a+b)%c);
System.out.println(((a%c) + (b%c))%c);
System.out.println((a*b)%c);
System.out.println(((a%c) * (b%c))%c);
}
}
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a = sc.nextInt();
int b = sc.nextInt();
System.out.println(a * (b % 10));
System.out.println(a * ((b % 100) / 10));
System.out.println(a * (b / 100));
System.out.println(a * b);
}
}
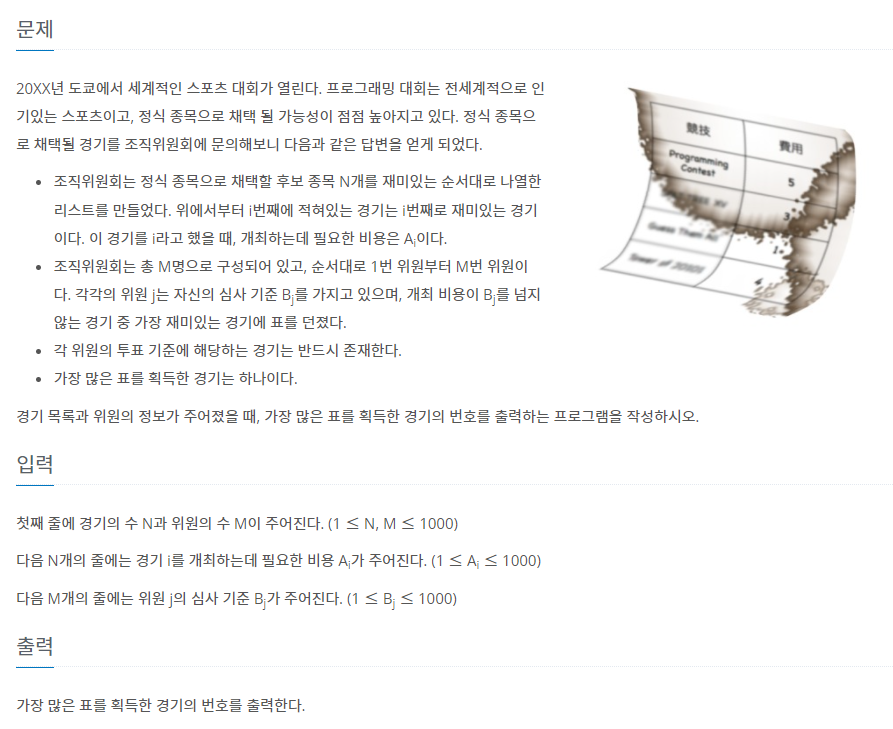
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int m = sc.nextInt();
int [] match =new int [n];
int [] person =new int [m];
int [] result =new int[n];
int max=0; int cnt=0;
for(int i = 0; i < n; i++) {
match[i] = sc.nextInt();
result[i] = 0;
}
for(int i = 0; i < m; i++) {
person[i] = sc.nextInt();
}
for(int i = 0; i < m; i++) {
out: for(int j = 0; j < n; j++) {
if(match[j] <= person[i]) {
result[j]++;
break out;
}
}
}
for(int i = 0; i < n; i++) {
if(result[i] > max) {
max = result[i];
cnt = i + 1;
}
}
System.out.println(cnt);
}
}