문제
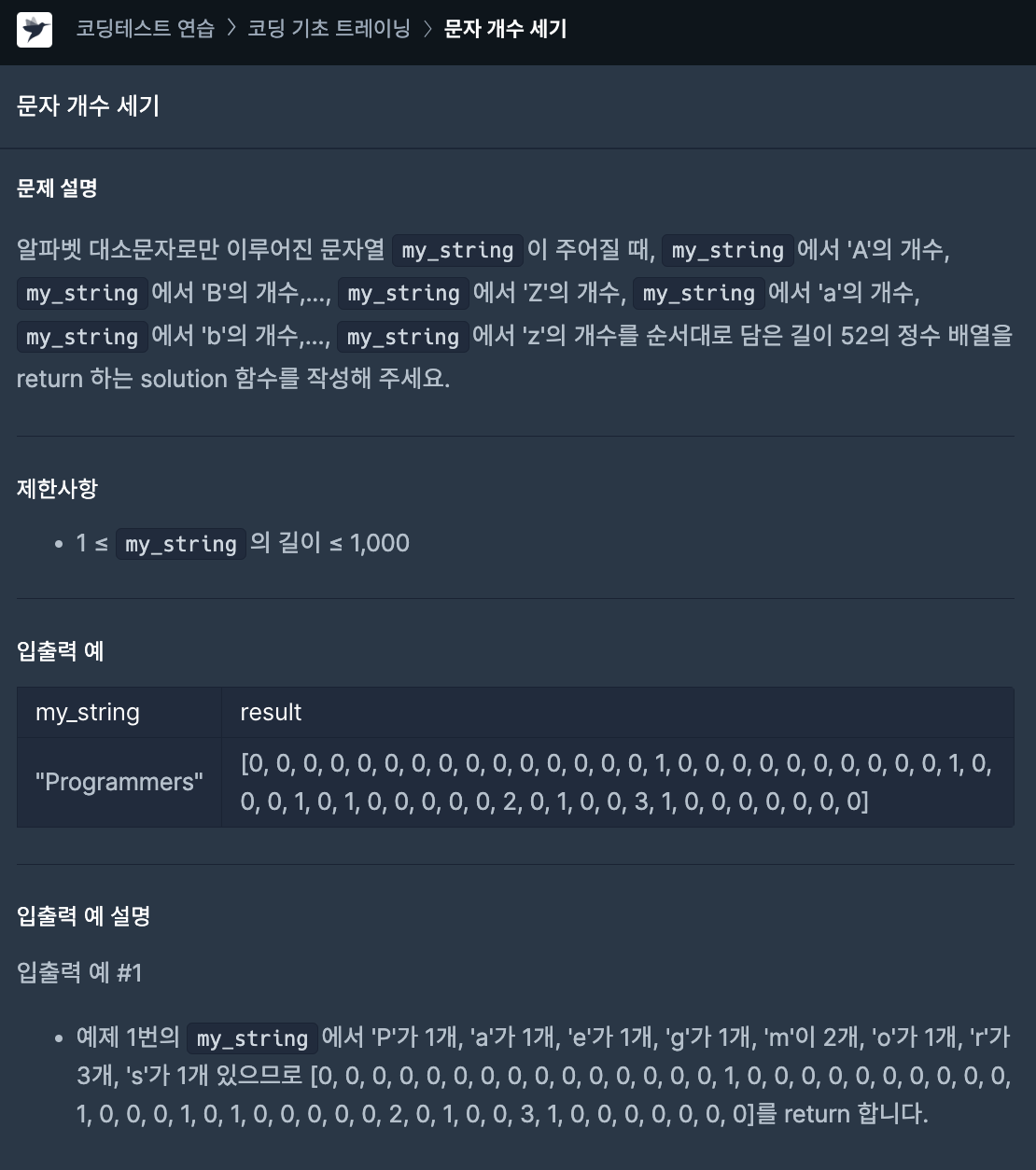
Python Code
from typing import List
def solution(my_string: str) -> List[str]:
alpha_dict = {chr(x): 0 for x in range(ord('A'), ord('Z')+1)}
alpha_dict.update({chr(x): 0 for x in range(ord('a'), ord('z')+1)})
for string in my_string:
alpha_dict[string] += 1
return list(alpha_dict.values())
Go Code
func solution(myString string) []int {
counts := make([]int, 52)
for _, char := range myString {
if char >= 'A' && char <= 'Z' {
counts[char-'A']++
} else if char >= 'a' && char <= 'z' {
counts[char-'a'+26]++
}
}
return counts
}
Java Code
import java.util.Arrays;
class Solution {
public int[] solution(String my_string) {
int[] counts = new int[52];
for (char c : my_string.toCharArray()) {
if (c >= 'A' && c <= 'Z') {
int index = c - 'A';
counts[index]++;
} else if (c >= 'a' && c <= 'z') {
int index = c - 'a' + 26;
counts[index]++;
}
}
return counts;
}
}
결과
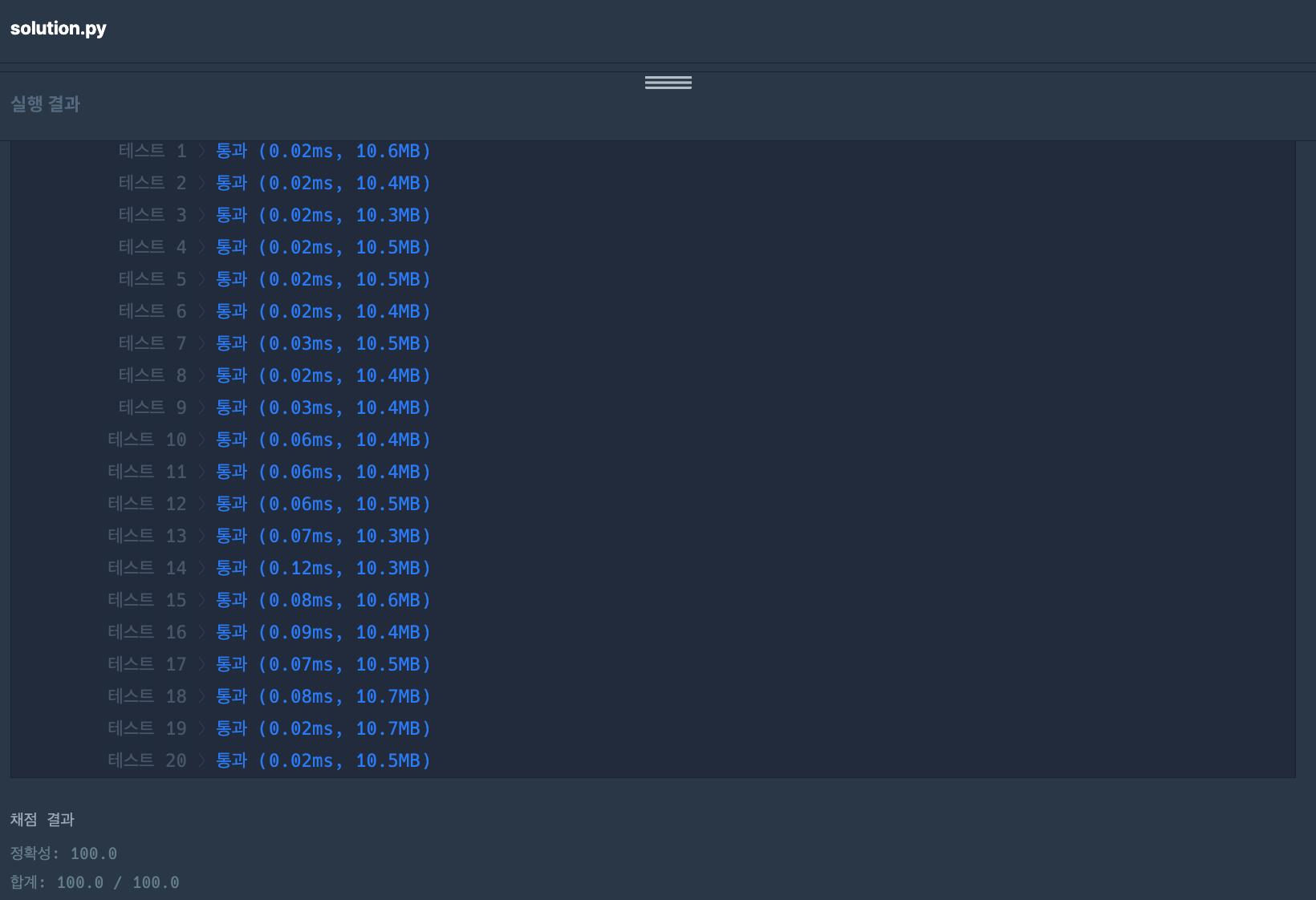
문제출처 & 깃허브
Programmers
Github