문제
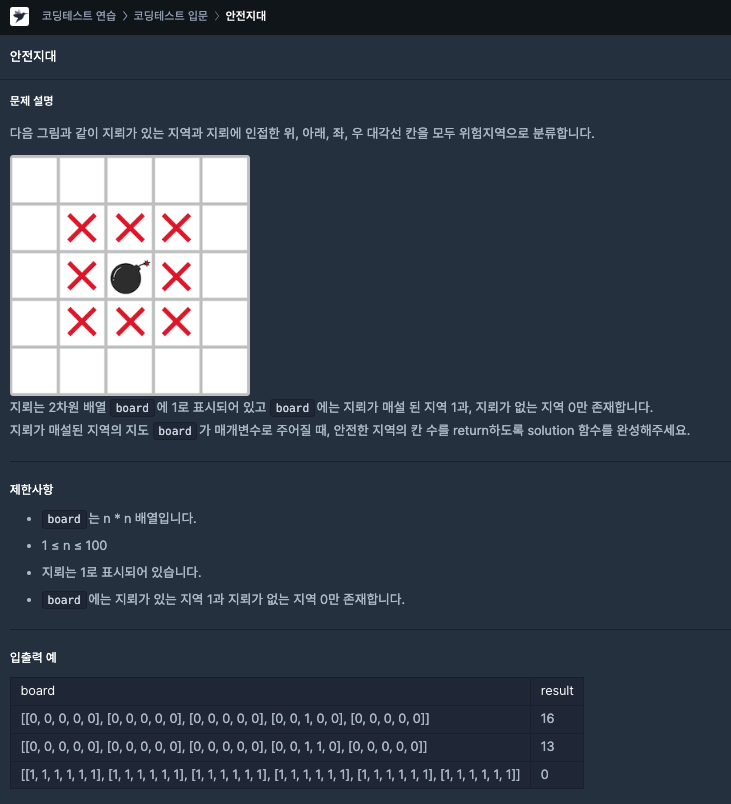
코드
from collections import deque
dx = [-1, 1, 0 , 0, 1, 1, -1, -1]
dy = [0, 0, -1, 1, -1, 1, 1, -1]
def bfs(x: int, y: int, visited: list, board: list):
queue = deque()
queue.append([x, y])
visited[x][y] = True
while queue:
x, y = queue.popleft()
for i in range(8):
nx = x + dx[i]
ny = y + dy[i]
if 0 <= nx < len(board) and 0 <= ny < len(board) and visited[nx][ny] == False:
visited[nx][ny] = True
if board[nx][ny] == 1:
queue.append([nx, ny])
else:
board[nx][ny] = -1
def solution(board: list):
answer = 0
visited = [[False] * len(board) for _ in range(len(board))]
for x in range(len(board)):
for y in range(len(board)):
if board[x][y] == 1 and visited[x][y] == False:
bfs(x, y, visited, board)
for x in range(len(board)):
for y in range(len(board)):
if board[x][y] == 0:
answer += 1
return answer
if __name__ == '__main__':
print(solution(
[[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 1, 0, 0], [0, 0, 0, 0, 0]]
))
print(solution(
[[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 1, 1, 0], [0, 0, 0, 0, 0]]
))
print(solution(
[[1, 1, 1, 1, 1, 1], [1, 1, 1, 1, 1, 1], [1, 1, 1, 1, 1, 1], [1, 1, 1, 1, 1, 1], [1, 1, 1, 1, 1, 1], [1, 1, 1, 1, 1, 1]]
))
결과
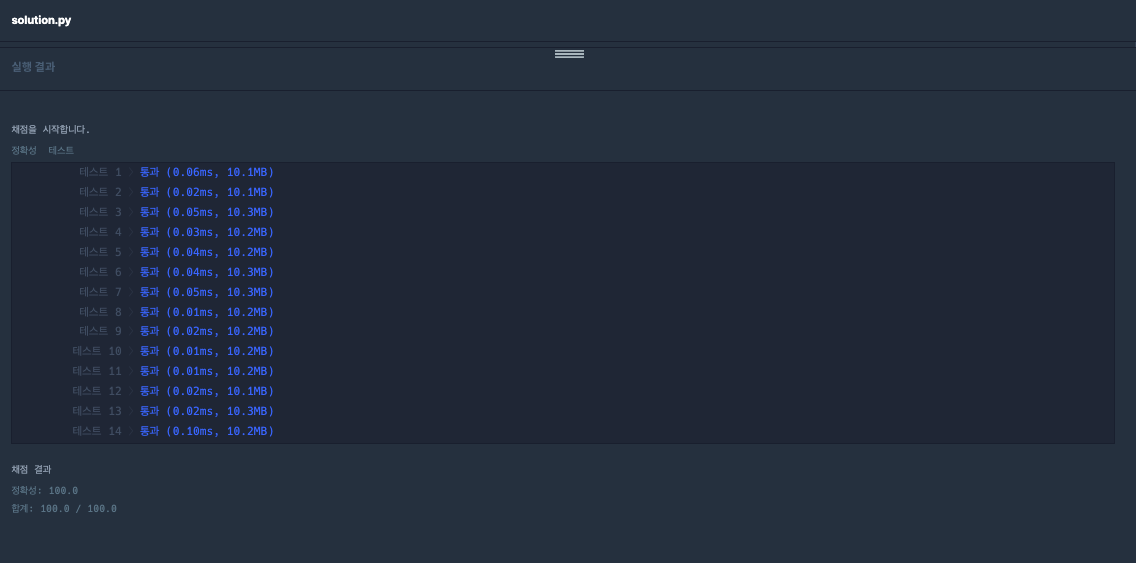
출처 & 깃허브
프로그래머스
깃허브