JSON
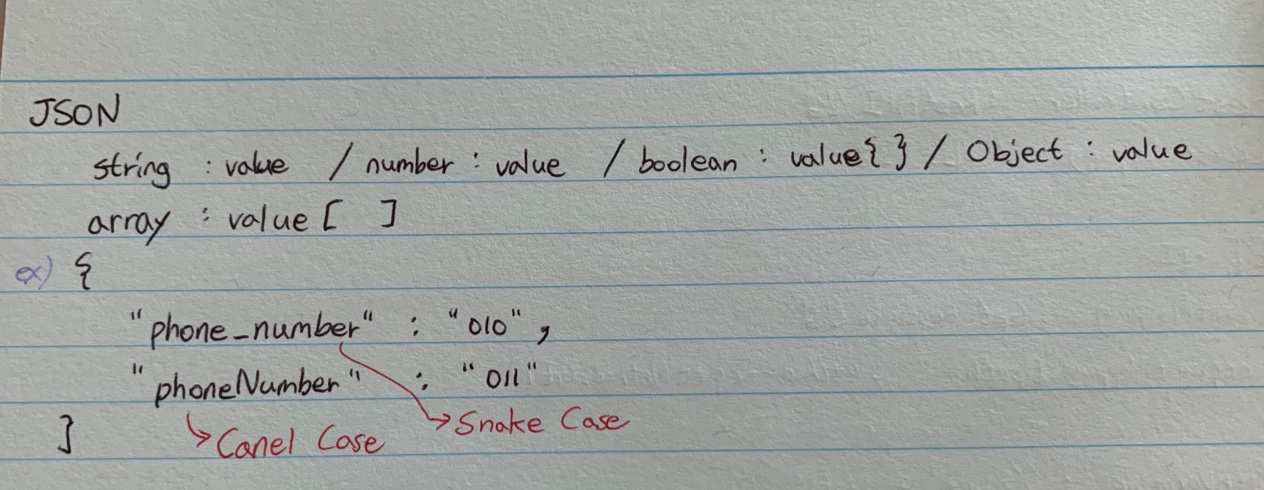
JSON 예시
{
"name" : "ming",
"age" : 20,
"familyList" :
[
{
"name" : "milk",
"relation" : "dog",
"age" : 10
},
{
"name" : "cookie",
"relation" : "dog",
"age" : 5
}
]
}
REST API
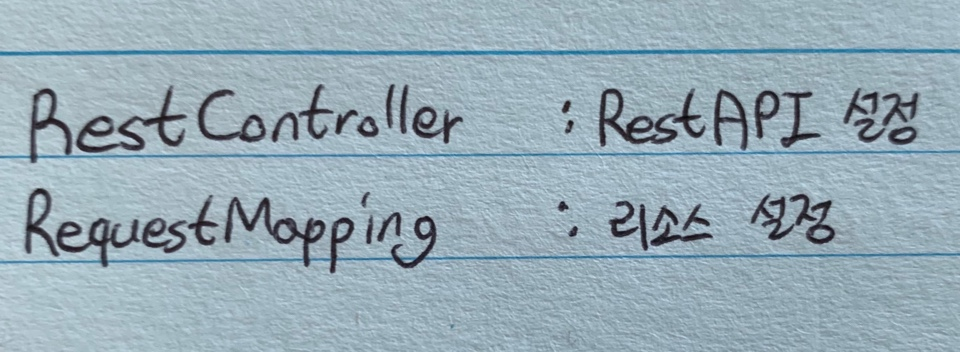
GET API
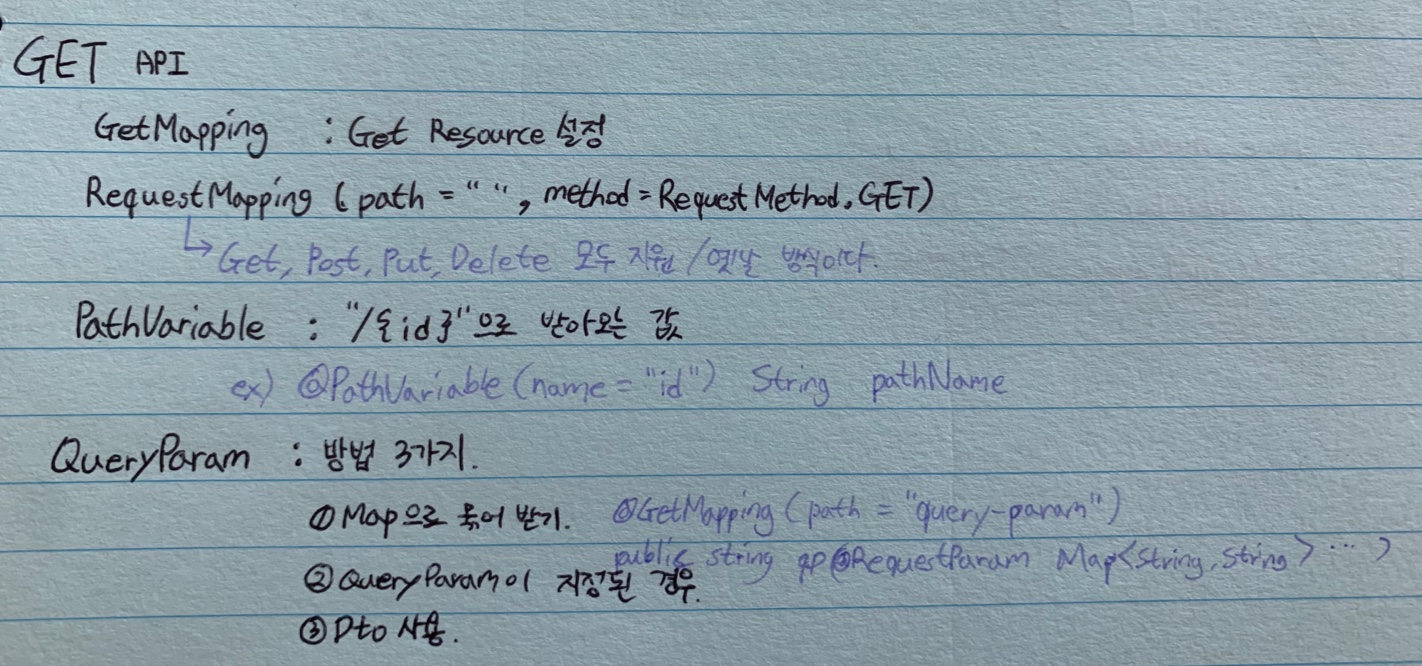
@GetMapping(path = "/hello")
public String getHello(){
return "get Hello";
}
//예전 버전으로, 아래 두가지를 합쳐서 getmapping 이 되었다.
//requestMapping은 get, post, delete, put 다 지원한다.
@RequestMapping(path = "hi", method = RequestMethod.GET)
public String getHi(){
return "hi";
}
@GetMapping("/path-variable/{id}")
public String pathVariable(@PathVariable(name = "id") String Pathname){
System.out.println("Path-Variable : "+Pathname);
return "Hello "+ Pathname;
}
//01. queryParam받기
//http://localhost:9090/api/get/query-param?user=milk&email=milk@gmail.com&age=13
@GetMapping(path = "query-param")
public String queryParam(@RequestParam Map<String,String> queryParam){
StringBuilder sb = new StringBuilder();
//01에서는 queryParam.get("name")처럼 하나씩 받아야함
queryParam.entrySet().forEach(entry ->{
System.out.println(entry.getKey());
System.out.println(entry.getValue());
System.out.println("\n");
sb.append(entry.getKey() + " = "+entry.getValue() + "\n");
});
return sb.toString();
}
//02. query param이 지정된 경우. ex) user,email,age만 넣어라.
@GetMapping(path = "query-param02")
public String queryParam02(@RequestParam String user,
@RequestParam String email,
@RequestParam int age){
System.out.println(user);
System.out.println(email);
System.out.println(age);
//반면에 01에서는 queryParam.get("name")처럼 하나씩 받아야함
return user +" " + email +" "+ age;
}
//03. 매번 queryParam을 추가하고 수정하기 힘드니 Dto를 사용한다.
//가장 많이 사용하는 방법이다. (들어오는 종류가 적다면 1,2 사용도 가능)
//여기서는 RequestParam 어노테이션을 사용하지 않아야 한다.
//객체가 들어오면 스프링 부트가 알아서 매칭을 시켜준다. 따라서 어노테이션 필요 없음.
@GetMapping(path = "query-param03")
public String queryParam03(UserRequest userRequest){
System.out.println(userRequest.getUser());
System.out.println(userRequest.getEmail());
System.out.println(userRequest.getAge());
return userRequest.toString();
}
}
POST API
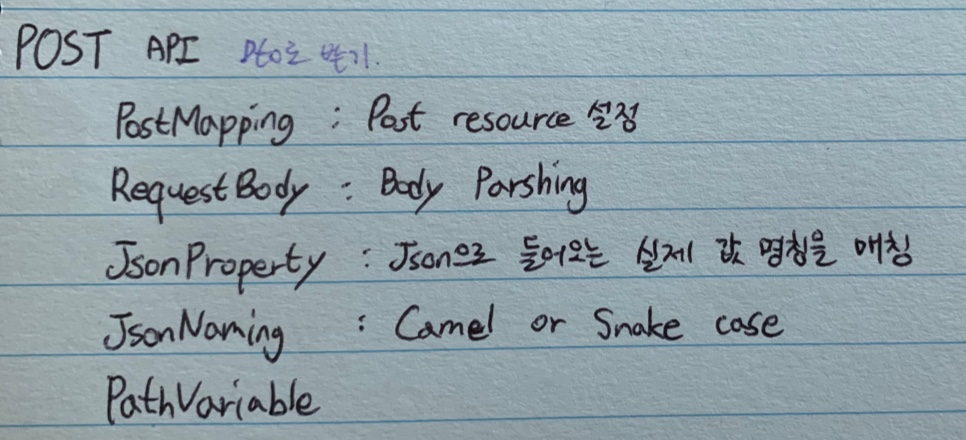
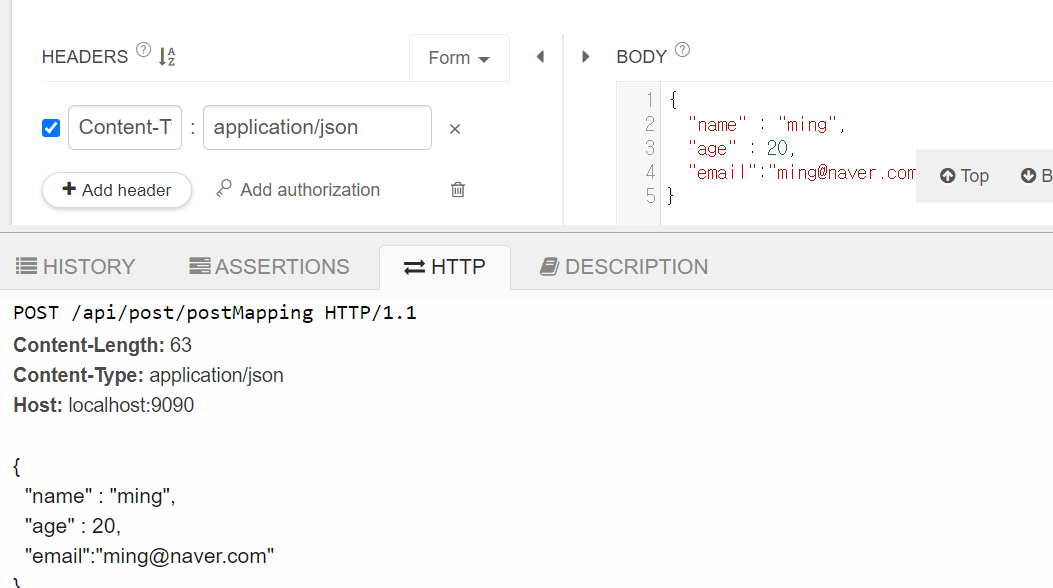
// @PostMapping("/postMapping")
// public void post(@RequestBody Map<String, Object> requestData){
// requestData.entrySet().forEach(stringObjectEntry -> {
// System.out.println("RequestData KEY : " + stringObjectEntry.getKey());
// System.out.println("RequestData VALUE : " + stringObjectEntry.getValue());
// }
// );
// }
//그런데 어떤 값인지 미리 알아야하는데 map으로만 받으면 코딩하는데 어려울 것이다. > DTO 사용해서 해결
@PostMapping("/postMapping")
public void post(@RequestBody PostRequestDto requestData){
System.out.println(requestData);
}
PUT API
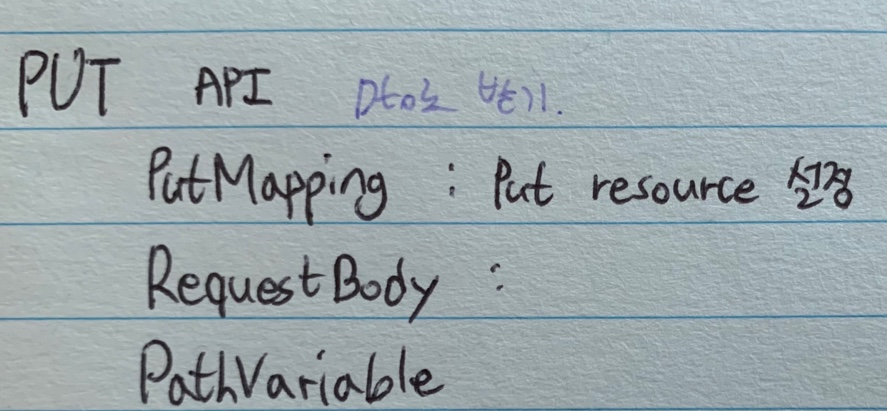
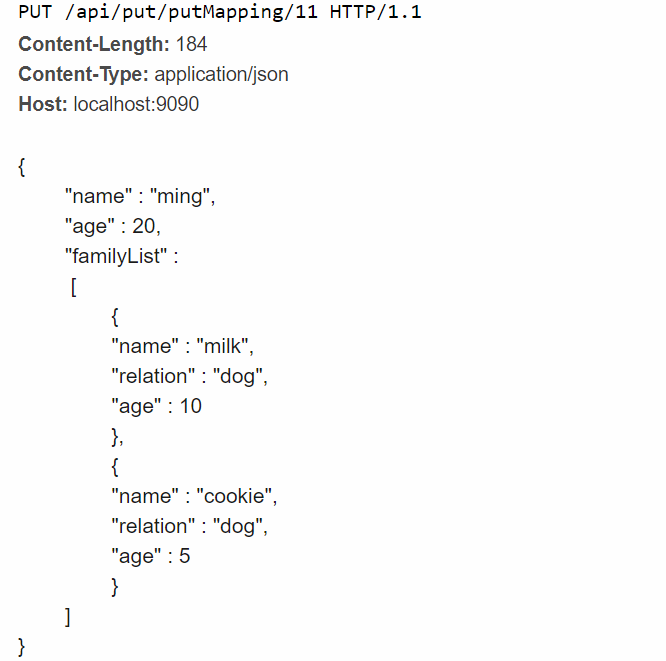
@PutMapping("/putMapping/{userId}")
public PutRequestDto put(
@RequestBody PutRequestDto requestDto,
@PathVariable(name = "userId") Long id){
System.out.println(requestDto);
System.out.println(id);
return requestDto;
}