DinoRun 마무리(PyGame)
import pygame
import os
import random
pygame.init()
ASSETS='./PyGame/Assets/'
SCREEN_WIDTH=1100
SCREEN_HEIGHT=600
SCREEN=pygame.display.set_mode((SCREEN_WIDTH,SCREEN_HEIGHT))
pygame.display.set_caption('다이노 런')
icon = pygame.image.load('./PyGame/dinoRun.png')
pygame.display.set_icon(icon)
bg=pygame.image.load(os.path.join(f'{ASSETS}Other','Track.png'))
RUNNING = [pygame.image.load(f'{ASSETS}Dino/DinoRun1.png'),
pygame.image.load(f'{ASSETS}Dino/DinoRun2.png')]
DUCKING = [pygame.image.load(f'{ASSETS}Dino/DinoDuck1.png'),
pygame.image.load(f'{ASSETS}Dino/DinoDuck1.png')]
JUMPING = pygame.image.load(f'{ASSETS}Dino/DinoJump.png')
START=pygame.image.load(f'{ASSETS}Dino/DinoStart.png')
DEAD=pygame.image.load(f'{ASSETS}Dino/DinoDead.png')
CLOUD = pygame.image.load(f'{ASSETS}Other/Cloud.png')
BIRD = [pygame.image.load(f'{ASSETS}Bird/Bird1.png'),
pygame.image.load(f'{ASSETS}Bird/Bird2.png')]
LARGE_CACTUS = [pygame.image.load(f'{ASSETS}Cactus/LargeCactus1.png'),
pygame.image.load(f'{ASSETS}Cactus/LargeCactus2.png'),
pygame.image.load(f'{ASSETS}Cactus/LargeCactus3.png')]
SMALL_CACTUS = [pygame.image.load(f'{ASSETS}Cactus/SmallCactus1.png'),
pygame.image.load(f'{ASSETS}Cactus/SmallCactus2.png'),
pygame.image.load(f'{ASSETS}Cactus/SmallCactus3.png')]
class Dino:
X_POS=80 ; Y_POS = 310 ; Y_POS_DUCK = 340 ; JUMP_VEL = 9.0
def __init__(self) -> None:
self.run_img=RUNNING
self.duck_img=DUCKING
self.jump_img=JUMPING
self.dino_run=True
self.dino_duck=False
self.dino_jump=False
self.step_index=0
self.jump_vel=self.JUMP_VEL
self.image=self.run_img[0]
self.dino_rect=self.image.get_rect()
self.dino_rect.x=self.X_POS
self.dino_rect.y=self.Y_POS
def update(self,userInput) -> None:
if self.dino_run:
self.run()
elif self.dino_duck:
self.duck()
elif self.dino_jump:
self.jump()
if self.step_index>=10 : self.step_index=0
if userInput[pygame.K_UP] and not self.dino_jump :
self.dino_run=False
self.dino_duck=False
self.dino_jump=True
self.dino_rect.y=self.Y_POS
elif userInput[pygame.K_DOWN] and not self.dino_jump:
self.dino_run=False
self.dino_duck=True
self.dino_jump=False
elif not (self.dino_jump or userInput[pygame.K_DOWN]):
self.dino_run=True
self.dino_duck=False
self.dino_jump=False
def run(self):
self.image=self.run_img[self.step_index//5]
self.dino_rect=self.image.get_rect()
self.dino_rect.x=self.X_POS
self.dino_rect.y=self.Y_POS
self.step_index+=1
def duck(self):
self.image=self.duck_img[self.step_index//5]
self.dino_rect=self.image.get_rect()
self.dino_rect.x=self.X_POS
self.dino_rect.y=self.Y_POS_DUCK
self.step_index+=1
def jump(self):
self.image=self.jump_img
if self.dino_jump:
self.dino_rect.y-=self.jump_vel * 4
self.jump_vel -= 0.8
if self.jump_vel<-self.JUMP_VEL:
self.dino_jump=False
self.jump_vel=self.JUMP_VEL
def draw(self,SCREEN) -> None:
SCREEN.blit(self.image,(self.dino_rect.x,self.dino_rect.y))
class Cloud:
def __init__(self) -> None:
self.x = SCREEN_WIDTH + random.randint(300,500)
self.y = random.randint(50,100)
self.image=CLOUD
self.width=self.image.get_width()
def update(self) -> None:
self.x-=game_speed
if self.x < -self.width:
self.x=SCREEN_WIDTH + random.randint(1300,2000)
self.y=random.randint(50,100)
def draw(self,SCREEN) -> None:
SCREEN.blit(self.image, (self.x,self.y))
class Obstacle:
def __init__(self,image,type) -> None:
self.image=image
self.type=type
self.rect=self.image[self.type].get_rect()
self.rect.x=SCREEN_WIDTH
def update(self)->None:
self.rect.x-=game_speed
if self.rect.x<=-self.rect.width:
obstacles.pop()
def draw(self,SCREEN)->None:
SCREEN.blit(self.image[self.type],self.rect)
class Bird(Obstacle):
def __init__(self, image) -> None:
self.type=0
super().__init__(image,self.type)
self.rect.y=250
self.index=0
def draw(self,SCREEN)->None:
if self.index >= 9:
self.index=0
SCREEN.blit(self.image[self.index//5],self.rect)
self.index+=1
class LargeCactus(Obstacle):
def __init__(self, image) -> None:
self.type=random.randint(0,2)
super().__init__(image, self.type)
self.rect.y=300
class SmallCactus(Obstacle):
def __init__(self, image) -> None:
self.type=random.randint(0,2)
super().__init__(image, self.type)
self.rect.y=325
def main():
global game_speed, x_pos_bg , y_pos_bg, points , obstacles , font
x_pos_bg = 0
y_pos_bg = 380
points=0
run=True
clock=pygame.time.Clock()
dino=Dino()
cloud = Cloud()
game_speed = 14
obstacles=[]
death_count = 0
font=pygame.font.Font(f'{ASSETS}NanumGothicBold.ttf',size=20)
def background():
global x_pos_bg, y_pos_bg
image_width=bg.get_width()
SCREEN.blit(bg,(x_pos_bg,y_pos_bg))
SCREEN.blit(bg,(image_width+x_pos_bg,y_pos_bg))
if x_pos_bg<=-image_width:
x_pos_bg=0
x_pos_bg-=game_speed
def score():
global points, game_speed
points+=1
if points % 100==0:
game_speed +=1
txtScore=font.render(f'SCORE : {points}', True , (83,83,83))
txtRect=txtScore.get_rect()
txtRect.center = (1000,40)
SCREEN.blit(txtScore,txtRect)
while run:
for event in pygame.event.get():
if event.type==pygame.QUIT:
run=False
SCREEN.fill((255,255,255))
userInput=pygame.key.get_pressed()
background()
score()
cloud.draw(SCREEN)
cloud.update()
dino.draw(SCREEN)
dino.update(userInput)
if len(obstacles)==0:
if random.randint(0,2)==0:
obstacles.append(SmallCactus(SMALL_CACTUS))
elif random.randint(0,2)==1:
obstacles.append(LargeCactus(LARGE_CACTUS))
elif random.randint(0,2)==2:
obstacles.append(Bird(BIRD))
for obs in obstacles:
obs.draw(SCREEN)
obs.update()
if dino.dino_rect.colliderect(obs.rect):
pygame.time.delay(300)
death_count +=1
menu(death_count)
clock.tick(30)
pygame.display.update()
def menu(death_count):
global points, font
run = True
font=pygame.font.Font(f'{ASSETS}NanumGothicBold.ttf',size=20)
while run:
SCREEN.fill((255,255,255))
if death_count == 0 :
text=font.render('시작하려면 아무 키나 누르세요',True,(83,83,83))
SCREEN.blit(START,(SCREEN_WIDTH//2-20,SCREEN_HEIGHT//2-140))
elif death_count >0:
text=font.render('재시작하려면 아무키나 누르세요',True,(83,83,83))
score=font.render(f'SCORE : {points}',True,(83,83,83))
scoreRect=score.get_rect()
scoreRect.center=(SCREEN_WIDTH//2,SCREEN_HEIGHT//2+50)
SCREEN.blit(score,scoreRect)
SCREEN.blit(DEAD,(SCREEN_WIDTH//2-20,SCREEN_HEIGHT//2-140))
textRect=text.get_rect()
textRect.center = (SCREEN_WIDTH//2,SCREEN_HEIGHT//2)
SCREEN.blit(text,textRect)
pygame.display.update()
for event in pygame.event.get():
if event.type==pygame.QUIT:
run=False
pygame.quit()
if event.type==pygame.KEYDOWN:
main()
if __name__=='__main__':
menu(death_count=0)
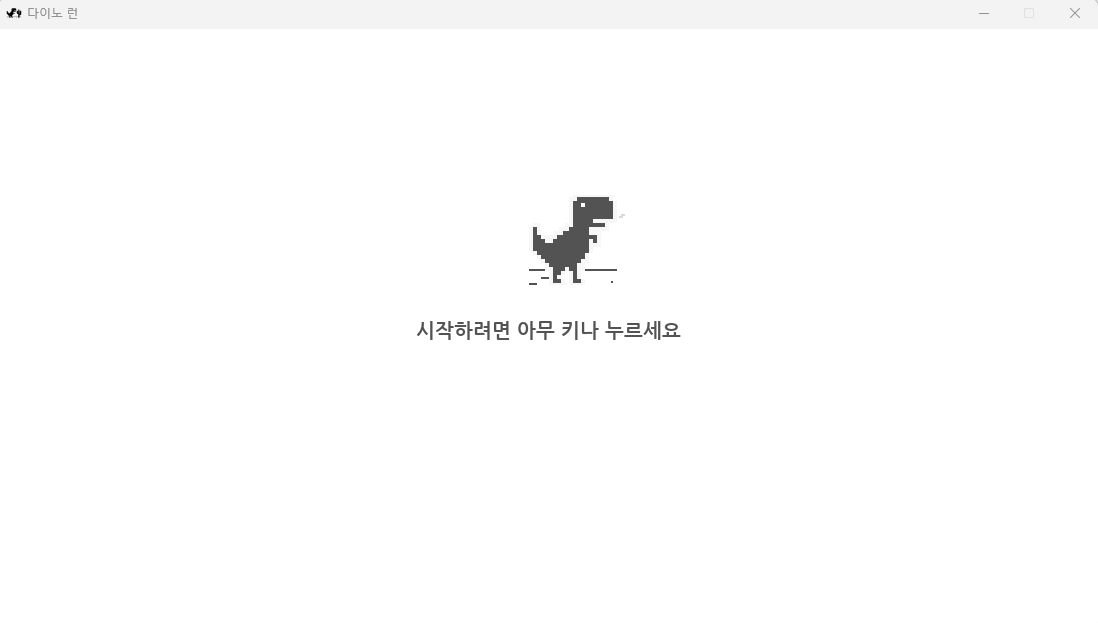
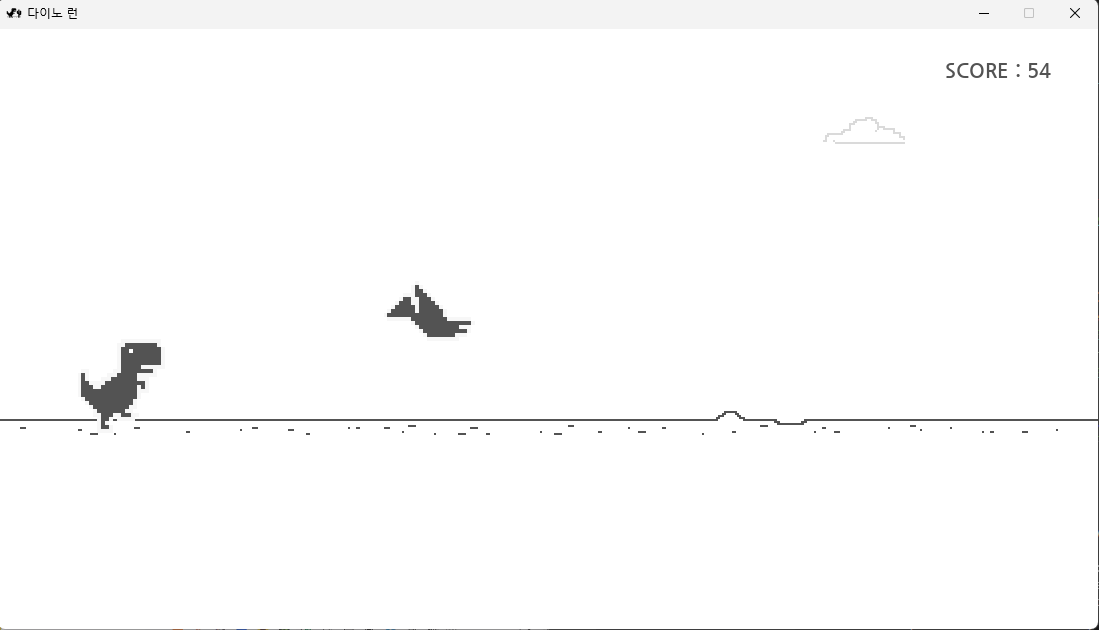
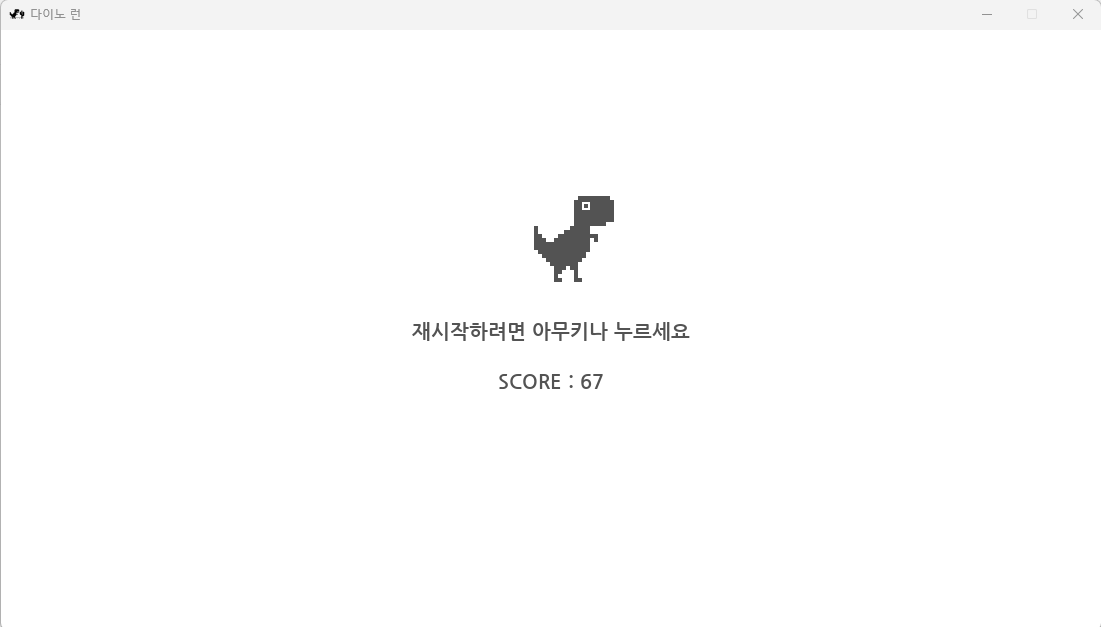
전국 대학교를 지도에 표시(Folium)
import sys
import folium
import io
import pandas as pd
from PyQt5 import uic
from PyQt5.QtWidgets import *
from PyQt5.QtGui import *
from PyQt5.QtCore import *
from PyQt5.QtWebEngineWidgets import QWebEngineView
class qtApp(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle('전국대학교 위치')
self.width,self.height=1000,800
self.setMinimumSize(self.width,self.height)
layout=QVBoxLayout()
self.setLayout(layout)
filePath='./Python_practice/university_locations.xlsx'
df_excel=pd.read_excel(filePath,engine='openpyxl',header=None)
df_excel.columns=['학교명','주소','lng','lat']
name_list=df_excel['학교명'].to_list()
addr_list=df_excel['주소'].to_list()
lng_list=df_excel['lng'].to_list()
lat_list=df_excel['lat'].to_list()
m=folium.Map(location=[37.553175,126.989326],zoom_start=10)
for i in range(len(name_list)):
if lng_list[i] !=0:
marker=folium.Marker([lat_list[i],lng_list[i]],popup=name_list[i],
icon=folium.Icon(color='blue'))
marker.add_to(m)
data=io.BytesIO()
m.save(data,close_file=False)
webView=QWebEngineView()
webView.setHtml(data.getvalue().decode())
layout.addWidget(webView)
if __name__=='__main__':
app=QApplication(sys.argv)
ex=qtApp()
ex.show()
sys.exit(app.exec_())
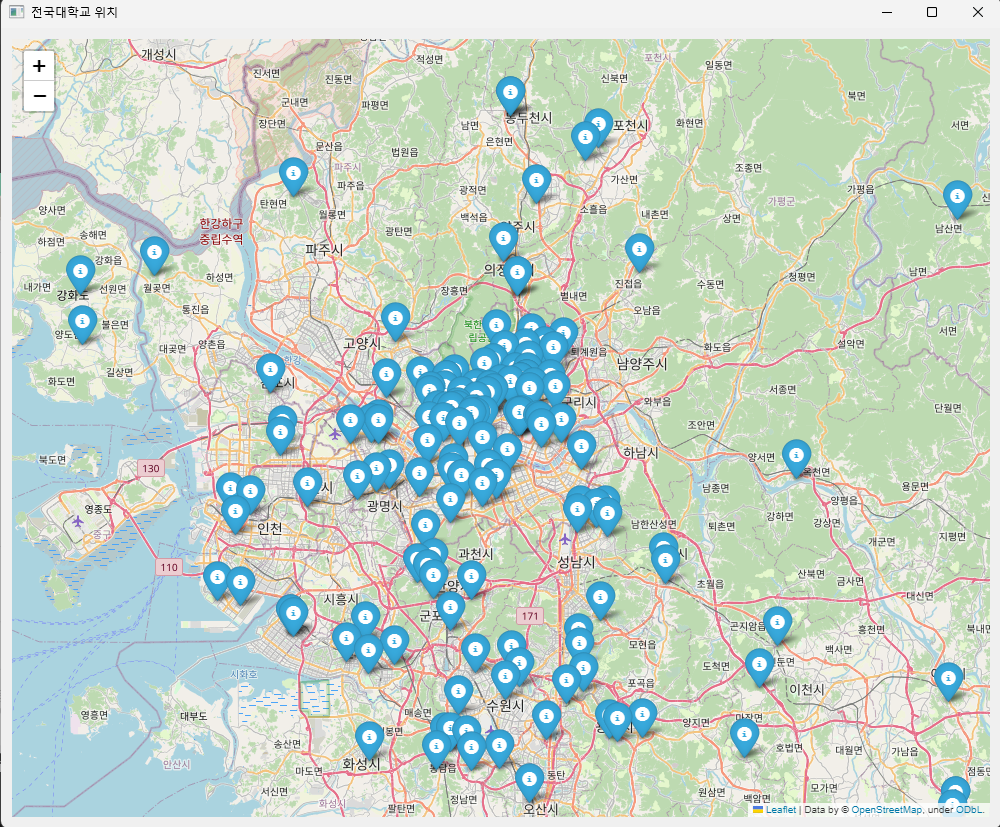