1. Thread
- 프로세스의 실행 단위
- 하나의 프로세스는 여러개의 스레드 가질 수 있음
- 프로세스
- 현재 실행중인 프로그램
- 운영체제로부터 자원을 할당받는 작업의 단위
2. Thread 생성 방법
1) Runnable 인터페이스 구현
public class ThreadCreateTest implements Runnable {
@Override
public void run() {
try {
while (true) {
Thread.sleep(1000);
System.out.println("Thread start");
}
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
}
public class ThreadCreateTestMain {
public static void main(String[] args) {
ThreadCreateTest th = new ThreadCreateTest();
Thread th2 = new Thread(th);
th2.start();
}
}
2) Thread 클래스 상속 받기
public class ThreadCreateTest extends Thread{
public void run(){
try {
while(true){
Thread.sleep(1000);
System.out.println("Thread start");
}
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
}
public class ThreadCreateTestMain {
public static void main(String args[]){
ThreadCreateTest th = new ThreadCreateTest();
th.start();
}
}
3. Thread 생명 주기
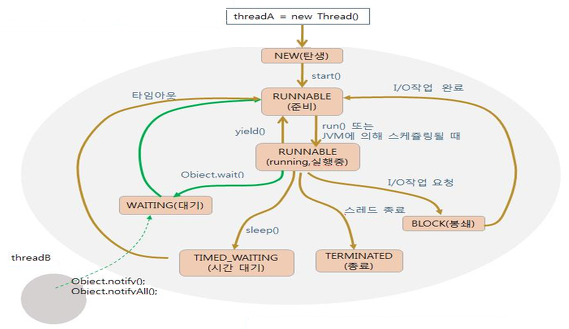
1) New
- 스레드가 생성 된 상태
- 아직 start() 되지 않음
2) Runnable 상태
3) Running 상태
4) Waiting
5) Timed_Waiting
6) Block
- 일시정지 상태
- 사용하려는 객체의 lock이 풀릴 때까지 대기 등..
7) Terminated
4. Thread와 프로세스의 차이점?
- 프로세스는 운영체제로부터 독립된 시간, 공간 자원을 할당받아 실행 됨
- 스레드는 한 프로세스 내에서 많은 자원을 공유하며 병렬적으로 실행 됨