GOAL
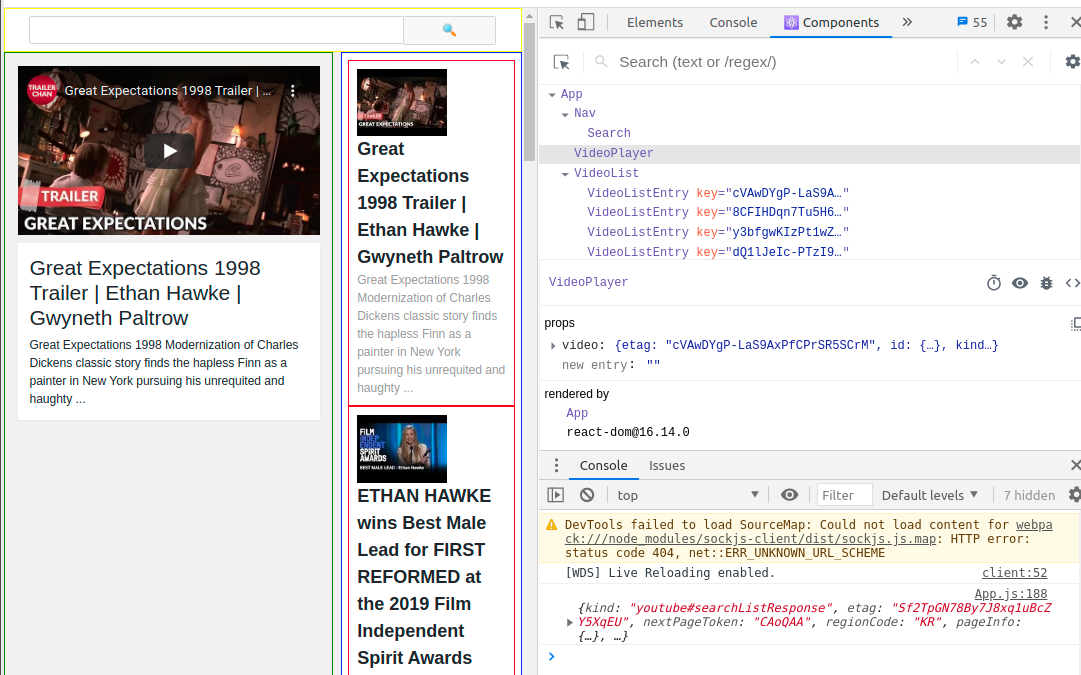
import React from "react";
import Nav from "./Nav";
import VideoPlayer from "./VideoPlayer";
import VideoList from "./VideoList";
import LoadingIndicator from "./LoadingIndicator";
class App extends React.Component {
constructor(props) {
super(props)
this.state = {
videos: [],
currentVideo: null,
isLoading: true
}
this.setCurrentVideo = this.setCurrentVideo.bind(this)
this.searchVideo = this.searchVideo.bind(this)
}
componentDidMount() {
this.searchVideo('ethan howke')
}
setCurrentVideo(video) {
this.setState({
currentVideo: video
})
}
searchVideo(queryString) {
let key = "------"
let url = `https://www.googleapis.com/youtube/v3/search?part=snippet&key=${key}&q=${queryString}&type=video&videoEmbeddable=true&maxResults=10`
fetch(url)
.then(res => res.json())
.then(result => {
console.log(result)
return this.setState({
videos: result.items,
currentVideo: result.items[0],
isLoading: false
})
})
}
render() {
return (
<div>
<Nav handleButtonClick = {this.searchVideo}/>
{this.state.isLoading
?
<LoadingIndicator />
:
<div className="parent">
<VideoPlayer video={this.state.currentVideo} />
<VideoList videos={this.state.videos} handleClickEntry={this.setCurrentVideo} />
</div>
}
</div>
)
}
}
export default App;
----------------------------------------------------------
import React from 'react';
import Search from './Search';
const Nav = (props) => (
<nav className="navbar">
<div className="col-md-6 col-md-offset-3">
<Search handleButtonClick = {props.handleButtonClick} />
</div>
</nav>
);
export default Nav;
----------------------------------------------------------
import React, { Component } from 'react';
class Search extends Component {
constructor(props) {
super(props)
this.state = {
queryString : ""
}
}
render() {
return (
<div className="search-bar form-inline">
<input className="form-control" type="text" onChange = {(e) => {
console.log(`e.target.value 로 ${e.target.value} 를 입력합니다`)
return this.setState({queryString : e.target.value})
}} />
<button className="btn hidden-sm-down" onClick = {() => {this.props.handleButtonClick(this.state.queryString)}} >
🔍️
</button>
</div>
);
}
}
export default Search;
----------------------------------------------------------
import React from 'react';
const VideoPlayer = ({ video }) => {
if (!video) {
return <div>비디오를 선택하세요</div>
}
return (
<div className="video-player">
<div className="embed-responsive embed-responsive-16by9">
<iframe className="embed-responsive-item"
src={`https://www.youtube.com/embed/${video.id.videoId}`} allowFullScreen></iframe>
</div>
<div className="video-player-details">
<h3>{video.snippet.title}</h3>
<div>{video.snippet.description}</div>
</div>
</div>
)
}
export default VideoPlayer;
----------------------------------------------------------
import React from 'react';
import VideoListEntry from './VideoListEntry';
const VideoList = ({ videos, handleClickEntry }) => {
if (videos.length === 0) {
return '비디오 목록이 없습니다'
}
return (
<div className="video-list media">
{videos.map(video =>
<VideoListEntry video={video} key={video.etag} handleClick={handleClickEntry} />
)}
</div>
)
};
export default VideoList;
----------------------------------------------------------
import React from 'react';
const VideoListEntry = ({ video, handleClick }) => (
<div className="video-list-entry">
<div className="media-left media-middle">
<img className="media-object" src={video.snippet.thumbnails.default.url} alt="" />
</div>
<div className="media-body">
<div className="video-list-entry-title" onClick={() => handleClick(video)}>{video.snippet.title}</div>
<div className="video-list-entry-detail">{video.snippet.description}</div>
</div>
</div>
)
export default VideoListEntry;