Skeleton Loader
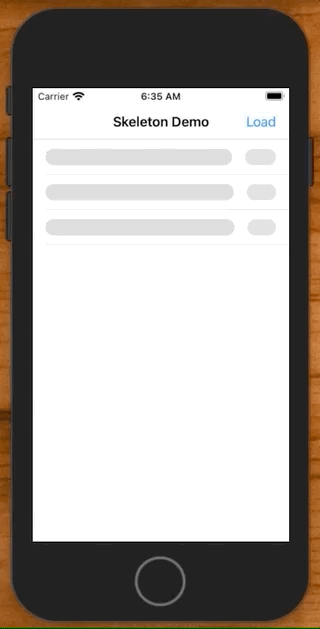
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let label = UILabel()
label.text = "Shimmer"
label.font = UIFont.systemFont(ofSize: 80)
label.frame = CGRect(x: 0, y: 80, width: view.frame.width, height: 100)
label.textAlignment = .center
let gradient = CAGradientLayer()
gradient.startPoint = CGPoint(x: 0, y: 0.5)
gradient.endPoint = CGPoint(x: 1, y: 0.5)
label.layer.addSublayer(gradient)
let animationGroup = makeAnimationGroup()
animationGroup.beginTime = 0.0
gradient.add(animationGroup, forKey: "backgroundColor")
gradient.frame = label.bounds
view.addSubview(label)
}
func makeAnimationGroup(previousGroup: CAAnimationGroup? = nil) -> CAAnimationGroup {
let animDuration: CFTimeInterval = 1.5
let anim1 = CABasicAnimation(keyPath: #keyPath(CAGradientLayer.backgroundColor))
anim1.fromValue = UIColor.gradientLightGrey.cgColor
anim1.toValue = UIColor.gradientDarkGrey.cgColor
anim1.duration = animDuration
anim1.beginTime = 0.0
let anim2 = CABasicAnimation(keyPath: #keyPath(CAGradientLayer.backgroundColor))
anim2.fromValue = UIColor.gradientDarkGrey.cgColor
anim2.toValue = UIColor.gradientLightGrey.cgColor
anim2.duration = animDuration
anim2.beginTime = anim1.beginTime + anim1.duration
let group = CAAnimationGroup()
group.animations = [anim1, anim2]
group.repeatCount = .greatestFiniteMagnitude
group.duration = anim2.beginTime + anim2.duration
group.isRemovedOnCompletion = false
if let previousGroup = previousGroup {
group.beginTime = previousGroup.beginTime + 0.33
}
return group
}
}
extension UIColor {
static var gradientDarkGrey: UIColor {
return UIColor(red: 239 / 255.0, green: 241 / 255.0, blue: 241 / 255.0, alpha: 1)
}
static var gradientLightGrey: UIColor {
return UIColor(red: 201 / 255.0, green: 201 / 255.0, blue: 201 / 255.0, alpha: 1)
}
}