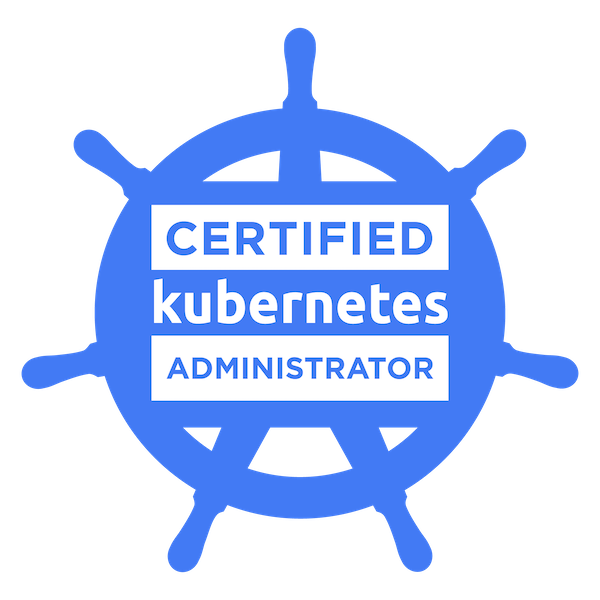
Rolling Updates and Rollbacks
1
Inspect the deployment and identify the number of PODs deployed by it
$ k describe deploy frontend | grep -i desire
2
What container image is used to deploy the applications?
$ k describe deploy frontend | grep -i image
3
Inspect the deployment and identify the current strategy
$ k describe deploy frontend | grep -i strategy
4
Let us try that. Upgrade the application by setting the image on the deployment to kodekloud/webapp-color:v2
Do not delete and re-create the deployment. Only set the new image name for the existing deployment.
$ k edit deploy frontend
image: kodekloud/webapp-color:v2
5
Run the script curl-test.sh. Notice the requests now hit both the old and newer versions. However none of them fail.
Execute the script at /root/curl-test.sh.
$ sh curl-test.sh
6
Change the deployment strategy to Recreate
Delete and re-create the deployment if necessary. Only update the strategy type for the existing deployment.
$ k edit deploy frontend
strategy:
type: Recreate
7
Upgrade the application by setting the image on the deployment to kodekloud/webapp-color:v3
Do not delete and re-create the deployment. Only set the new image name for the existing deployment.
$ k edit deploy frontend
image: kodekloud/webapp-color:v3
Commands and Arguments
1
How many PODs exist on the system?
In the current(default) namespace
$ k get pods
2
What is the command used to run the pod ubuntu-sleeper?
$ k describe pod ubuntu-sleeper | grep -iA 3 command
3
Create a pod with the ubuntu image to run a container to sleep for 5000 seconds. Modify the file ubuntu-sleeper-2.yaml.
Note: Only make the necessary changes. Do not modify the name.
ubuntu-sleeper-2.yaml
apiVersion: v1
kind: Pod
metadata:
name: ubuntu-sleeper-2
spec:
containers:
- name: ubuntu
image: ubuntu
command: ["sleep","5000"]
$ k apply -f ubuntu-sleeper-2.yaml
4
Create a pod using the file named ubuntu-sleeper-3.yaml. There is something wrong with it. Try to fix it!
Note: Only make the necessary changes. Do not modify the name.
ubuntu-sleeper-3.yaml
apiVersion: v1
kind: Pod
metadata:
name: ubuntu-sleeper-3
spec:
containers:
- name: ubuntu
image: ubuntu
command:
- "sleep"
- "1200"
$ k apply -f ubuntu-sleeper-3.yaml
5
Update pod ubuntu-sleeper-3 to sleep for 2000 seconds.
$ k edit pod ubuntu-sleeper-3
containers:
- command:
- sleep
- "2000"
$ k delete pod ubuntu-sleeper-3
$ k apply -f /tmp/kubectl-edit-3512171132.yaml
6
Inspect the file Dockerfile given at /root/webapp-color directory. What command is run at container startup?
$ cd webapp-color
$ cat Dockerfile
ENTRYPOINT ["python", "app.py"]
7
Inspect the file Dockerfile2 given at /root/webapp-color directory. What command is run at container startup?
$ cat Dockerfile2
ENTRYPOINT ["python", "app.py"]
CMD ["--color", "red"]
8
Inspect the two files under directory webapp-color-2. What command is run at container startup?
Assume the image was created from the Dockerfile in this directory.
$ cd ~/webapp-color-2
$ cat cat webapp-color-pod.yaml
command: ["--color","green"]
9
Inspect the two files under directory webapp-color-3. What command is run at container startup?
Assume the image was created from the Dockerfile in this directory.
$ cd ~/webapp-color-3
$ cat cat webapp-color-pod-2.yaml
command: ["python", "app.py"]
args: ["--color", "pink"]
10
Create a pod with the given specifications. By default it displays a blue background. Set the given command line arguments to change it to green.
$ cd ~/webapp-color-2
$ vi webapp-color-pod.yaml
apiVersion: v1
kind: Pod
metadata:
name: webapp-green
labels:
name: webapp-green
spec:
containers:
- name: simple-webapp
image: kodekloud/webapp-color
args: ["--color", "green"]
$ k apply -f webapp-color-pod.yaml
Env Variables
1
How many PODs exist on the system?
in the current(default) namespace
$ k get pods
2
What is the environment variable name set on the container in the pod?
$ k describe pod webapp-color | grep -iA 5 env
3
What is the value set on the environment variable APP_COLOR on the container in the pod?
$ k describe pod webapp-color | grep -iA 5 env
4
Update the environment variable on the POD to display a green background.
Note: Delete and recreate the POD. Only make the necessary changes. Do not modify the name of the Pod.
$ k edit pod webapp-color
spec:
containers:
- env:
- name: APP_COLOR
value: green
$ k delete pod webapp-color
$ k apply -f /tmp/kubectl-edit-4270112926.yaml
5
How many ConfigMaps exists in the default namespace?
$ k get cm
6
Identify the database host from the config map db-config.
$ k describe cm db-config
7
Create a new ConfigMap for the webapp-color POD. Use the spec given below.
$ k create cm webapp-config-map --from-literal=APP_COLOR=darkblue --from-literal=APP_OTHER=disregard
8
Update the environment variable on the POD to use only the APP_COLOR key from the newly created ConfigMap.
Note: Delete and recreate the POD. Only make the necessary changes. Do not modify the name of the Pod.
$ k edit pod webapp-color
spec:
containers:
- env:
- name: APP_COLOR
valueFrom:
configMapKeyRef:
key: APP_COLOR
name: webapp-config-map
$ k delete pod webapp-color
$ k apply -f /tmp/kubectl-edit-2833310666.yaml
Secret
1
How many Secrets exist on the system?
In the current(default) namespace.
$ k get secret
2
How many secrets are defined in the dashboard-token secret?
$ k describe secret dashboard-token
3
What is the type of the dashboard-token secret?
$ k describe secret dashboard-token | grep -i type
4
The reason the application is failed is because we have not created the secrets yet. Create a new secret named db-secret with the data given below.
You may follow any one of the methods discussed in lecture to create the secret.
$ k create secret generic db-secret --from-literal=DB_Host=sql01 --from-literal=DB_User=root --from-literal=DB_Password=password123
5
Configure webapp-pod to load environment variables from the newly created secret.
Delete and recreate the pod if required.
$ k edit pod webapp-pod
envFrom:
- secretRef:
name: db-secret
Multi Container PODs
1
Identify the number of containers created in the red pod.
$ k get pod red
2
Identify the name of the containers running in the blue pod.
$ k describe pod blue | grep -i container
3
Create a multi-container pod with 2 containers.
Use the spec given below:
If the pod goes into the crashloopbackoff then add the command sleep 1000 in the lemon container.
Name: yellow
Container 1 Name: lemon
Container 1 Image: busybox
Container 2 Name: gold
Container 2 Image: redis
yellow.yaml
apiVersion: v1
kind: Pod
metadata:
name: yellow
spec:
containers:
- name: lemon
image: busybox
command:
- sleep
- "1000"
- name: gold
image: redis
$ k apply -f yellow.yaml
4
Inspect the app pod and identify the number of containers in it.
It is deployed in the elastic-stack namespace.
$ k get pod app -n elastic-stack
5
The application outputs logs to the file /log/app.log. View the logs and try to identify the user having issues with Login.
Inspect the log file inside the pod.
$ k -n elastic-stack exec -it app -- cat /log/app.log
6
Edit the pod to add a sidecar container to send logs to Elastic Search. Mount the log volume to the sidecar container.
Only add a new container. Do not modify anything else. Use the spec provided below.
$ k edit pod -n elastic-stack
apiVersion: v1
kind: Pod
metadata:
name: app
namespace: elastic-stack
labels:
name: app
spec:
containers:
- name: app
image: kodekloud/event-simulator
volumeMounts:
- mountPath: /log
name: log-volume
- name: sidecar
image: kodekloud/filebeat-configured
volumeMounts:
- mountPath: /var/log/event-simulator/
name: log-volume
volumes:
- name: log-volume
hostPath:
# directory location on host
path: /var/log/webapp
# this field is optional
type: DirectoryOrCreate
Init Containers
1
Identify the pod that has an initContainer configured.
$ k describe pod green | grep -i init
$ k describe pod red | grep -i init
$ k describe pod blue | grep -i init
2
What is the image used by the initContainer on the blue pod?
$ k describe pod blue
3
We just created a new app named purple. How many initContainers does it have?
$ k describe pod purple
4
Update the pod red to use an initContainer that uses the busybox image and sleeps for 20 seconds
Delete and re-create the pod if necessary. But make sure no other configurations change.
$ k edit pod red
initContainers:
- image: busybox
name: red-initcontainer
command: ["sleep","20"]
$ k delete pod red
$ k apply -f /tmp/kubectl-edit-410690827.yaml