1. JSON
- JSON(JavaScript Object Notation)은 클라이언트와 서버 간의 HTTP 통신을 위한 텍스트 데이터 포멧이다.
- 자바스크립트에 종속되지 않는 언어 독립형 데이터 포멧으로, 대부분의 프로그래밍 언어에서 사용할 수 있다.
1) JSON 표기 방식
- JSON은 자바스크립트의 객체 리터럴과 유사하게 키와 값으로 구성된 순수한 텍스트다.
- JSON은 키-값 쌍 사이 공백은 사용할 수 없다.
- JSON의 key는 반드시 큰따옴표(
""
, 작은 따옴표 사용 불가)로 감싸야 한다.
- JSON의 value는 객체 리터럴과 같은 표기법을 그대로 사용할 수 있지만, 문자열은 큰따옴표(
""
, 작은 따옴표 사용 불가)로 감싸야 한다.
2) JSON.stringfy
JSON.stringfy
메서드는 객체를 JSON으로 변환한다.
- JSON으로 변환된 객체의 타입은 문자열(string)이다.
- 클라이언트가 서버로 객체를 전송하려면 객체를 문자열화해야 하는데 이를 직렬화(serilalize)라고 한다.
const message = {
sender: "김코딩",
receiver: "박해커",
message: "해커야 오늘 저녁 같이 먹을래?",
createdAt: "2021-01-12 10:10:10"
}
let transferableMessage = JSON.stringify(message)
console.log(typeof(transferableMessage))
console.log(transferableMessage)
const transferableMessage = JSON.stringify(message, null, 2)
console.log(typeof(transferableMessage))
console.log(transferableMessage)
JSON.stringfy
메서드는 객체뿐만 아니라 배열도 JSON 포맷 문자열로 변환한다.
const todos = [
{ id: 1, content: 'HTML', completed: false },
{ id: 2, content: 'CSS', completed: true },
{ id: 3, content: 'JavaScript', completed: false }
];
const json = JSON.stringify(todos, null, 2);
console.log(json);
3) JSON.parse
JSON.parse
메서드는 JSON을 객체로 변환한다.
- 서버로부터 클라이언트에게 전송된 JSON 데이터는 문자열(string)이다. 이 문자열을 객체로 사용하려면 JSON 포맷의 문자열을 객체화해야 하는데 이를 역직렬화 역직렬화(deserilalize)라고 한다.
- 배열의 요소가 객체인 경우, 배열의 요소까지 객체로 변환된다.
let packet =
let obj = JSON.parse(packet)
console.log(obj)
console.log(typeof(obj))
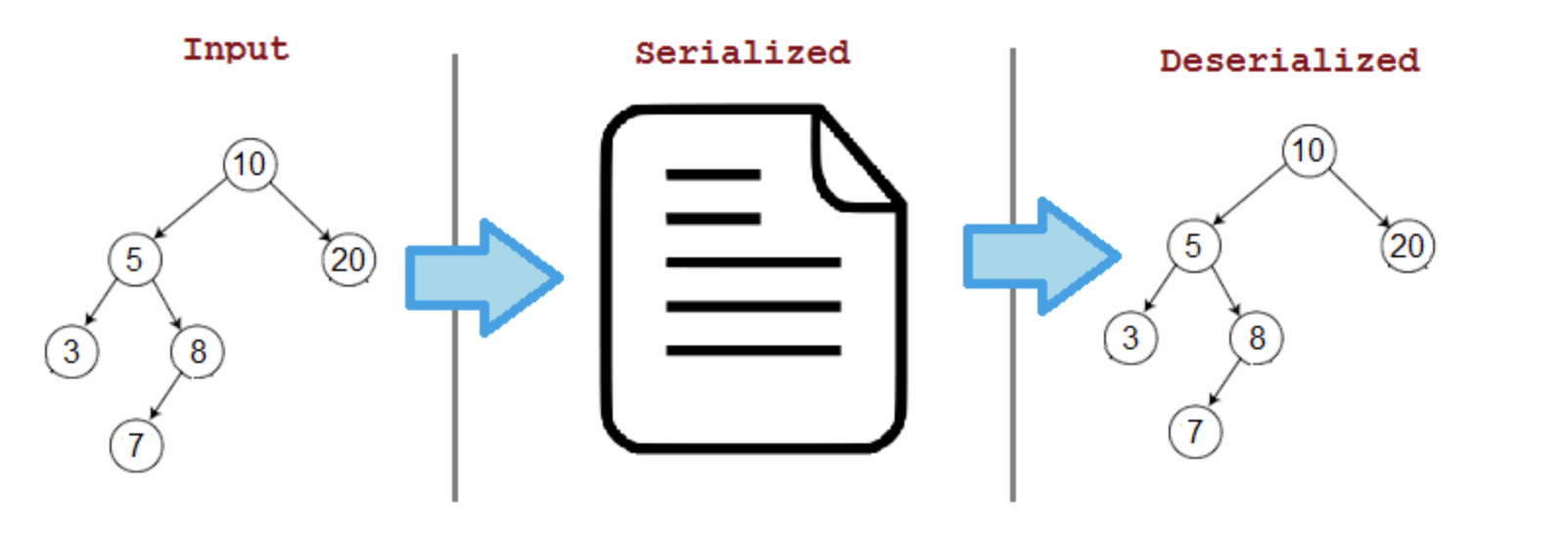
4) JSON의 기본 규칙
- JSON은 자바스크립트의 객체와 비슷해 보이지만, 다른 규칙이 있다.
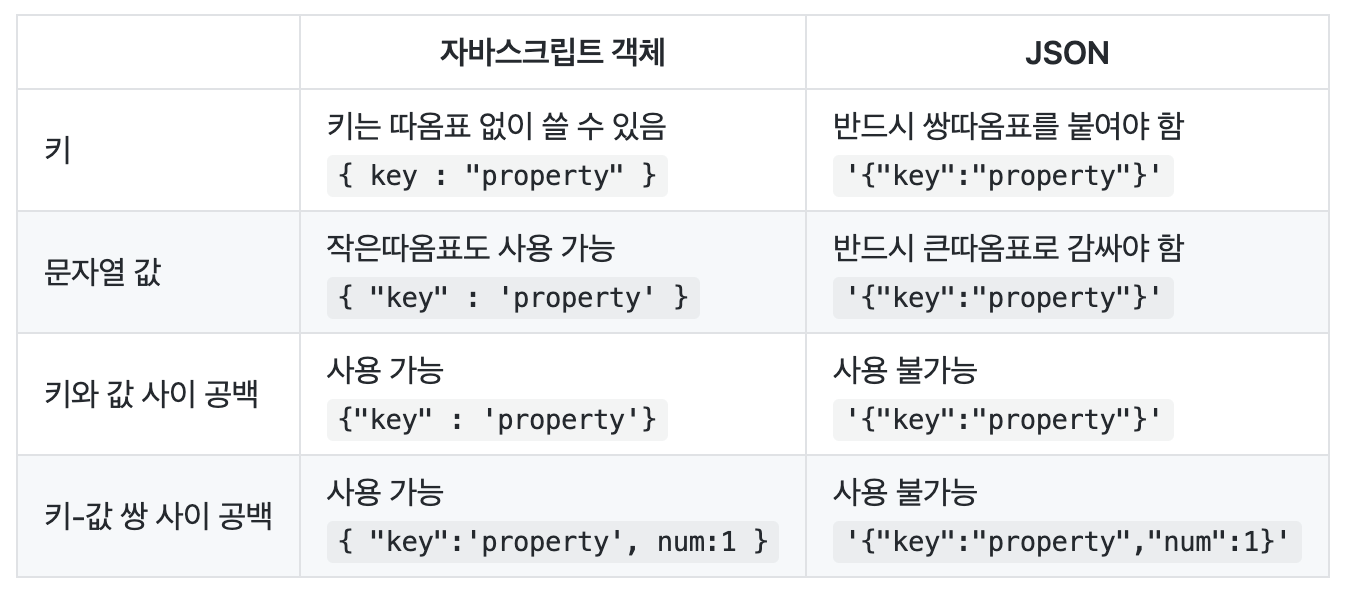