Bcrypt
설치
pip install bcrypt
import bcrypt
암호화
- bcrypt는
str
데이터가 아닌 bytes
데이터를 암호화하기 때문에 암호화를 하기 위해서는 데이터를 bytes
화 해야한다.
- python에서는
str
을 encode 하면 bytes(이진화)
되고, bytes
를 decode 하면 str
화 한다.
- encode, decode시에는 우리가 인식할 수 있는 형태로 변환하기 위해 'UTF-8' 유니코드 문자 규격을 사용
password = '1234'
hashed_password = bcrypt.hashpw(password.encode('utf-8'), bcrypt.gensalt())
print(hashed_password)
b'$2b$12$YFs9rh.1LgJwZuf9ibyjpuLvBoCaGX0MzedFWF2Jo0zU3lMZurZ4a'
복호화
new_password = '1234'
bcrypt.checkpw(new_password.encode('utf-8'),hashed_password)
True
- bcrypt.checkpw()
입력받은 패스워드, 저장된 암호화된 패스워드 둘 다 데이터 타입이 bytes
여야 함
JWT(JSON Web Token)
설치
pip install pyjwt
python token 발행
import jwt
SECRET = 'secret'
access_token = jwt.encode({'id' : 1}, SECRET, algorithm = 'HS256')
print(access_token)
'eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJpZCI6MX0.-xXA0iKB4mVNvWLYFtt2xNiYkFpObF54J9lj2RwduAI'
- jwt의 결과물은 pyjwt의 버전에 따라
bytes
(ver. 1.7)타입 또는 str
(ver. 2.0 이상)타입
token 확인
header = jwt.decode(access_token, SECRET, algorithm = 'HS256')
print(header)
{'id': 1}
- decode 결과는 바로 우리가 encode 할 때 넘겼던 header값인 { 'id' : 1}
- 인증하는 코드는 엔드포인트에 데코레이터를 구현해야 한다.
- 데코레이터 구현은 보통 user app에 utils.py를 만들어서 작성
Django에 적용하기

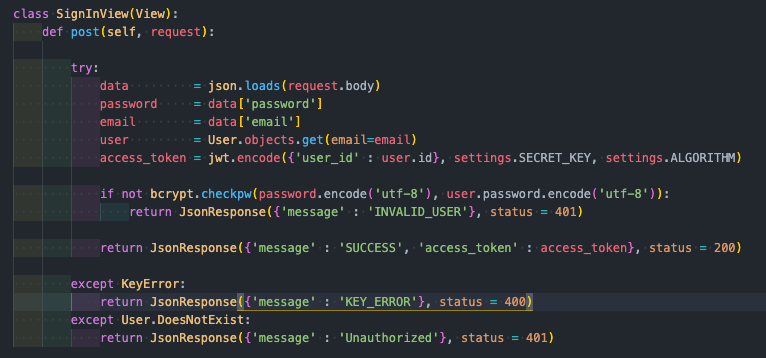
hashed_password = bcrypt.hashpw(password.encode('utf-8'), bcrypt.gensalt()).decode('utf-8')