과제 풀이
interface
Anonymous inner class (익명 중첩 클래스)
Button menu = new Button() {
@Override
public void push() {
System.out.println("메뉴 표시");
}
};
- 상속을 일회용으로 받는다. (변수에 저장하지 않는 class 구현)
- 그 자체를 상속받아 사용하려 할 때
Lambda(추론식)
- 추론을 할 수 있도록 안써도 되는 코드 축약한 식
- Lambda식 사용조건
- interface일 것 (추상 class X, static X)
- method를 1개만 가지고 있을 것 (함수형 interface)
- 함수형 programming을 위해 java에서 제공하는 방법
- 속도가 약간 느려지지만 편하니까 쓰자
- 등장 시기: Java 8+
Library
Java Standard API
> API(Application Programming Interface)
- API: OS를 효과적으로 이용하는 도구
- Scanner는 OS의 input stream
- System은 OS의 각종 정보와 자원을 제공
- API = document + Class, interface, package
- API -> (module) -> package -> class/interface -> field/method/constructor
- Java 8-
- Java 9+
> Library
- 자주 사용되고 유용한 기능 class들을 관련된 기능별로 분류하여 패키지단위로 묶어서 관리
> Framework
- library를 포함한 완성된 시스템(분식집)
lang.object
- Object is the root of the class hierarchy (모든 data type을 저장할 수 있는 배열이나 ArrayList에 활용할 수 있음)
- Constructor
- 기본 생성자
Object a = new Object()
- method
a.equals(b)
각 subclass마다 override해서 기준을 정해줄 수 있음
(VScode기준 우클릭 - Source Action - Generate hashCode() and equals() - field 선택)
- hashCode()
a.hashCode()
객체의 일련번호 출력
- toString()
a.toString()
객체의 요약정보 확인
It is recommended that all subclasses override this method.
print의 parameter가 object일 때, .toString()의 결과 출력
lang.string
- String 비교(==)
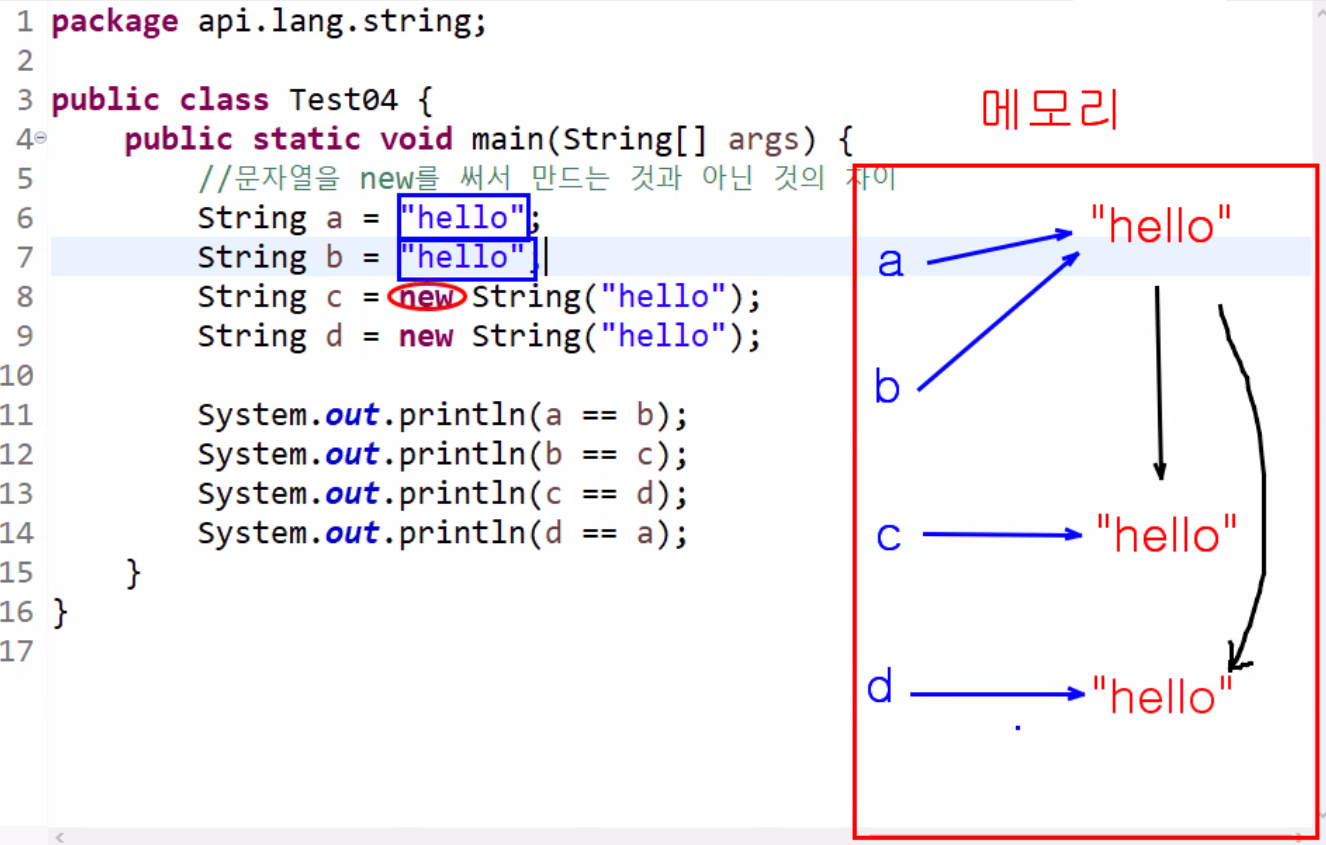
> method
return int
- .length()
- The length is equal to the number of Unicode code units in the string
- .indexOf(int ch, int fromIndex), indexOf(String str, int fromIndex)
- .compareTo(String compareStr)
return boolean
- g.equals(String compareStr)
- the argument / the same sequence of characters
- .equalsIgnoreCase(String compareStr)
- equalsIgnoreCase method보다 case를 통일해서 비교하는게 속도가 더 빠르다.
- .match(regex)
- Tells whether or not this string matches the given regular expression.
- .contains(CharSequence s)
- .startsWith(), endsWith()
return String
- .substring(int startIndex, int end)
- startIndex < 0 || startIndex > string.length()
runtime error 발생
- startIndex == string.length()
return "";
- .replace(CharSequence target, CharSequence replacement)
- .replaceAll(String regex, String replacement), replaceFirst
- .concat(String str)
- .repeat()
- String.valueof()
- String.join(CharSequence delimiter, CharSequence... elements)
- .toLowerCase(), toUpperCase()
- .trim()
return String[]
- .split(String regex, int limit)
- ex) "boo:and:foo".split(":") => { "boo", "and", "foo" }
"boo:and:foo".split("o") => { "b", "", ":and:f" }
return char
- .charAt(int index)
- !( 0 <= index < String.length() ) -> runtime error!
return char[]
byte[] .getBytes(?String charsetName)
File I/O에서 사용
regex (정규 표현식)
> regex 시각화 사이트
https://regexper.com/
reference: https://codechacha.com/ko/java-regex/
- .: 어떤 문자 1개
- ^regex: regex가 line의 처음인지
- regex$: regex가 line의 마지막인지
- [abc]: abc중 하나
- [^abc]: abc를 제외한 문자 1개
- X|Y: X또는 Y
- \d: [0-9]
- \D: [^0-9]
- \w: [a-zA-Z_0-9]
> Quantifiers
- *: 0회 이상
- +: 1회 이상
- ?: 0또는 1회만
- {X}: X회 이상
- {X, Y}: X~Y 사이의 수만큼
> Grouping
@Test
public void ex8() {
String pattern = "(\\w)(\\s+)([\\w])";
System.out.println("Hello World".replaceAll(pattern, "-"));
pattern = "(\\w)(\\s+)([\\w])";
System.out.println("Hello World".replaceAll(pattern, "$1-$3"));
}