Servlet
MIME(Multipurpose Internet Mail Extensions) type
- 클라이언트와 서버간에 주고받는 컨텐츠의 타입을 나타내는 것
- 구조
type/subType으로 구성되어 있다.
- 예시
text/plain
text/html
text/xml
image/png
image/jpg
audio/mp3
video/mp4
- 대표적인 타입
text
- 인간이 읽을 수 있는 데이터 혹은 문서
application
- 모든 종류의 이진(바이너리) 데이터
- 사람이 직접 읽을 수 없는 데이터 혹은 문서
- 전용의 애플리케이션이 필요해
- 대표적인 MIME타입
text/plain
- 일반 텍스트 데이터
text/html
- HTML 컨텐츠
text/xml
- XML 컨텐츠
application/json
- json 형식의 데이터
application/octet-stream
- 이진(바이너리) 데이터의 기본값
- 타입이 알려지지 않은 이진(바이너리) 데이터를 의미함
multipart/form-data
- 브라우저에서 서버로 폼 입력값을 전송할 때 사용되는 변환방법 중 하나다.
- 첨부파일이 있을 때 사용
- [서버로 전송되는 형식]
----WebKitFormBoundaryQtk5bV9PVWAMOkEI
Content-Disposition: form-data; name="title"
휴가신청서
application/x-www-form-urlencoded
- 브라우저에서 서버로 폼 입력값을 전송할 때 사용되는 변환방법 중 하나다.
- 첨부파일이 없을 때 사용
- [서버로 전송되는 형식]
- name=value&name=value&name=value
File Upload
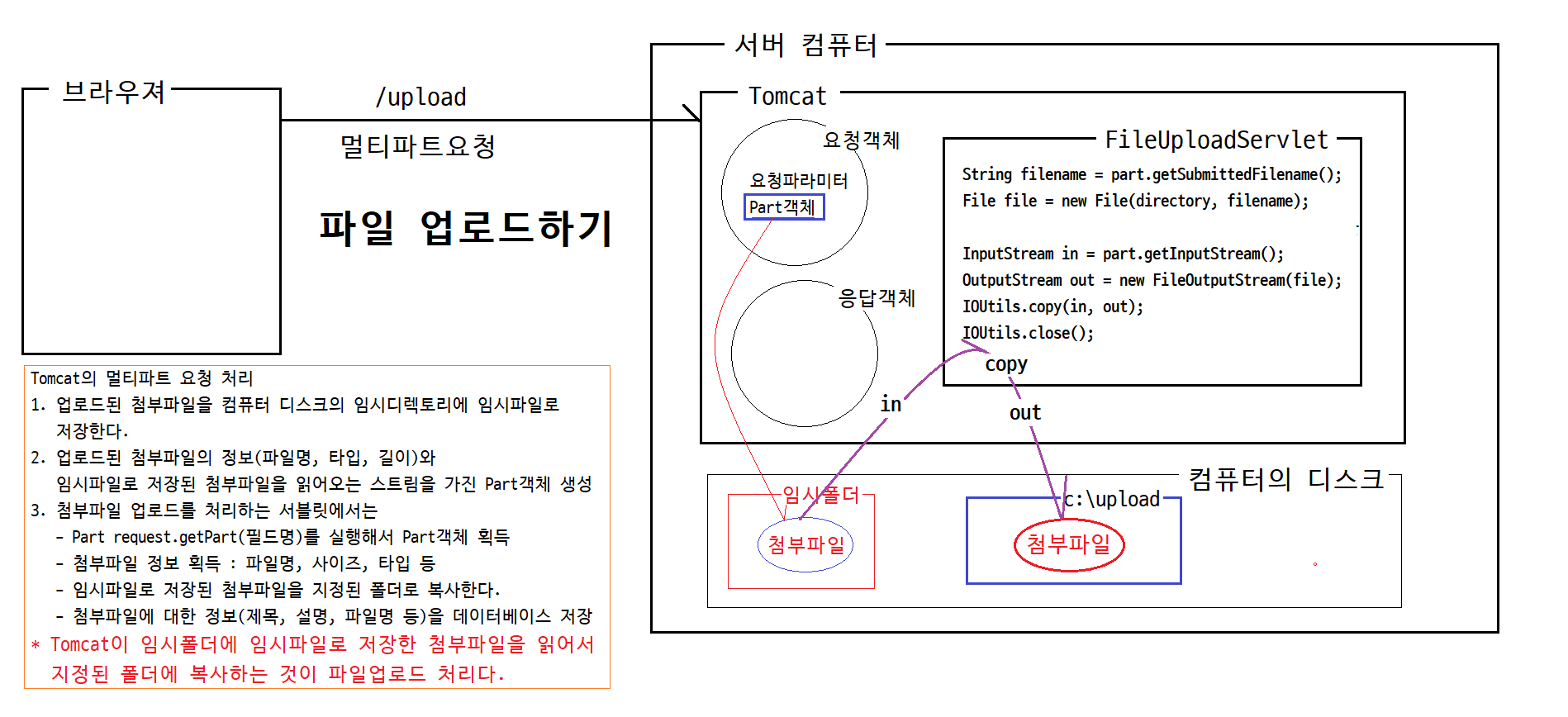
<%@ page contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" %>
<!DOCTYPE html>
<html lang="ko">
<head>
<title>Bootstrap 4 Example</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.16.0/umd/popper.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container">
<div class="row mt-3">
<div class="col-12">
<h3>파일 업로드 폼</h3>
<form method="post" action="/upload" enctype="multipart/form-data">
<div class="form-group">
<label>제목</label>
<input type="text" class="form-control" name="title"/>
</div>
<div class="form-group">
<label>설명</label>
<textarea rows="3" class="form-control" name="description"></textarea>
</div>
<div class="form-group">
<label>첨부파일</label>
<input type="file" class="form-control" name="upfile" />
</div>
<div class="text-right">
<a href="../index.jsp" class="btn btn-secondary">취소</a>
<button type="submit" class="btn btn-primary">등록</button>
</div>
</form>
</div>
</div>
</div>
</body>
</html>
@WebServlet("/upload")
@MultipartConfig
public class FileUploadServlet extends HttpServlet {
private static final String saveDirectory = "C:\\workspace\\Central_HTA\\java-web\\upload";
@Override
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String title = request.getParameter("title");
String description = request.getParameter("description");
System.out.println("제목 : " + title);
System.out.println("설명 : " + description);
Part part = request.getPart("upfile");
String filename = System.currentTimeMillis() + part.getSubmittedFileName();
OutputStream out = new FileOutputStream(new File(saveDirectory, filename));
InputStream in = part.getInputStream();
IOUtils.copy(in, out);
out.close();
FileItem fileItem = new FileItem();
fileItem.setTitle(title);
fileItem.setDescription(description);
fileItem.setFilename(filename);
FileItemDao fileItemDao = FileItemDao.getInstance();
fileItemDao.insertFileItem(fileItem);
response.sendRedirect("/index.jsp");
}
}
File Download
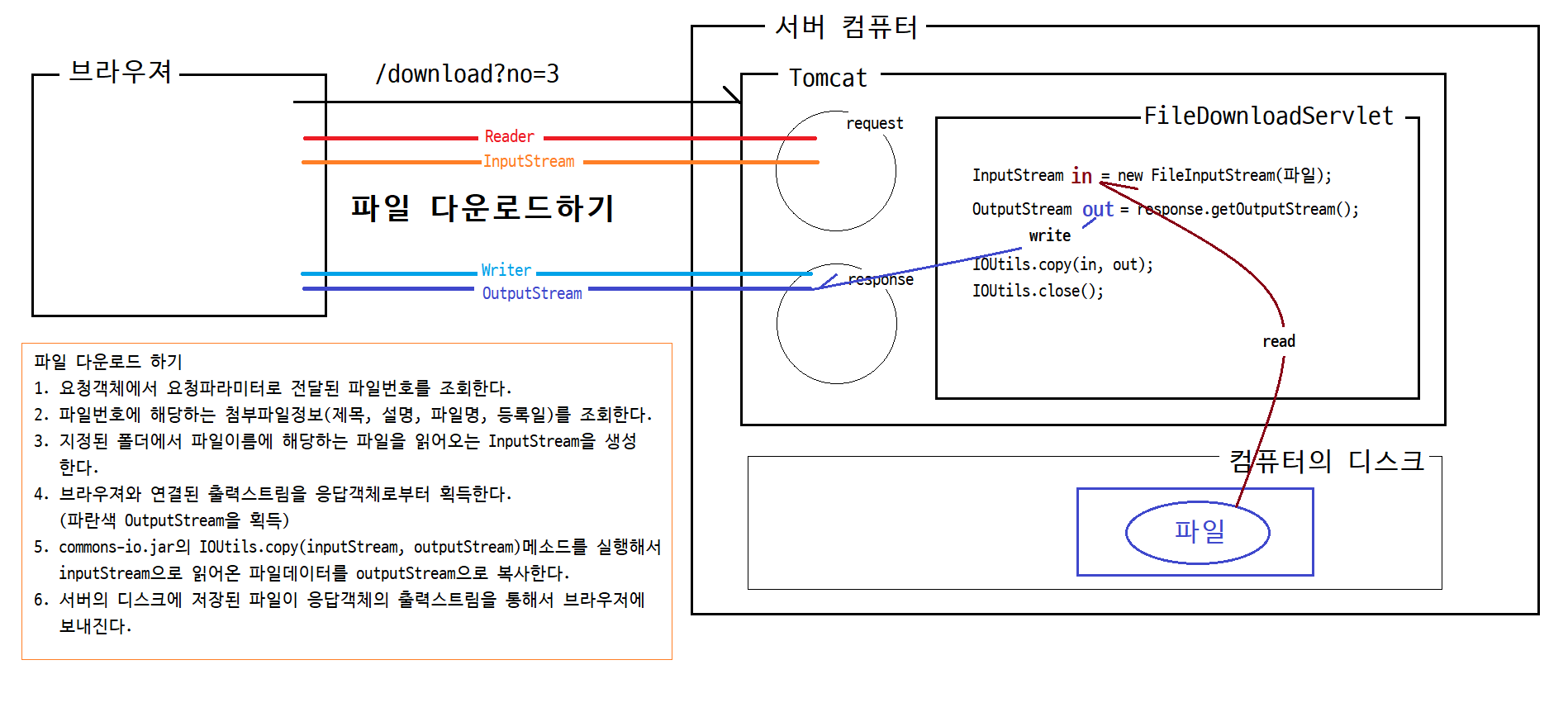
@WebServlet("/download")
public class FileDownloadServlet extends HttpServlet {
private static final String saveDirectory = "C:\\workspace\\Central_HTA\\java-web\\upload";
@Override
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int fileNo = Integer.parseInt(request.getParameter("no"));
FileItemDao fileItemDao = FileItemDao.getInstance();
FileItem fileItem = fileItemDao.getFileItem(fileNo);
String filename = fileItem.getFilename();
response.setContentType("application/octet-stream");
String shortFilename = fileItem.getShortFilename();
shortFilename = URLEncoder.encode(shortFilename, "utf-8");
response.setHeader("Content-Disposition", "attachment; filename=" + shortFilename);
InputStream in = new FileInputStream(new File(saveDirectory, filename));
OutputStream out = response.getOutputStream();
IOUtils.copy(in, out);
IOUtils.close();
}
}