애플 공식 문서를 바탕으로 정리합니다!
Comparing Structures and Classes
- Define properties to store values
- Define methods to provide functionality
- Define subscripts to provide access to their values using subscript syntax
- Define initializers to set up their initial state
- Be extended to expand their functionality beyond a default implementation
- Conform to protocols to provide standard functionality of a certain kind
Classes have additional capabilities that structures don’t have:
- Inheritance enables one class to inherit the characteristics of another.
- Type casting enables you to check and interpret the type of a class instance at runtime.
- Deinitializers enable an instance of a class to free up any resources it has assigned.
- Reference counting allows more than one reference to a class instance.
Structures and Enumerations Are Value Types
A value type is a type whose value is copied when it’s assigned to a variable or constant, or when it’s passed to a function.
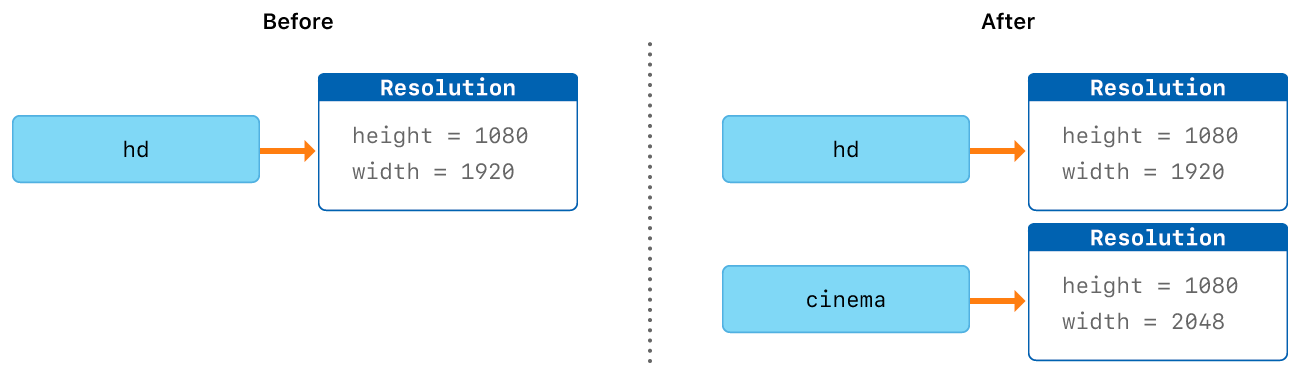
Classes Are Reference Types
Unlike value types, reference types are not copied when they’re assigned to a variable or constant, or when they’re passed to a function. Rather than a copy, a reference to the same existing instance is used.
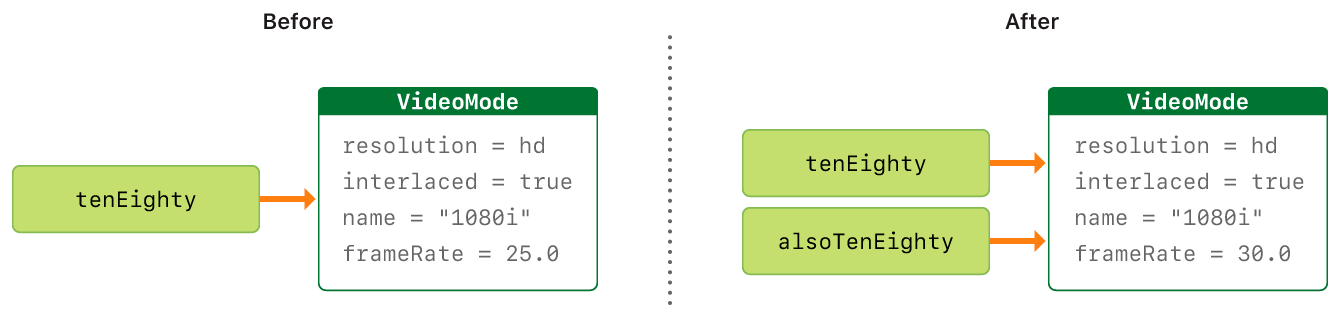
Identity Operators
- Identical to (===)
- Not identical to (!==)
if tenEighty === alsoTenEighty {
print("tenEighty and alsoTenEighty refer to the same VideoMode instance.")
}
Pointers
A Swift constant or variable that refers to an instance of some reference type is similar to a pointer in C, but isn’t a direct pointer to an address in memory, and doesn’t require you to write an asterisk (*) to indicate that you are creating a reference.
When we have to create the object in Swift, what kind of object do we have to use?
We have to consider it by the purpose of the object.
Use 'Struct',
- When you have to compare the objects with data
- When you want the copied object that has independent status
- When the data of the object will be used in several threads in the code
Use 'Class',
- When you have to compare the objects without data. Compare only the type of the instance.
- When you need only one object that can be shared and edited in many different ways.
If you don't know which one is better, create the object with struct. If you think 'Class' is better afterward, change it to 'Class'. It is not difficult! Btw Swift loves struct more than the class!