bcrypt
pip instlal bcrypt
>>> import bcrypt
>>> password = '1234'
>>> encoded_password = password.encode('utf'8')
Here, utf-8 converts String to Byte.
So, password.decode('utf-8')
will be String.
>>> hashed_password = bcrypt.hashpw( password.encode('utf-8'), bcrypt.gensalt() )
>>> hashed_password
b'$2b$12$9thPwDyIL0RMHnDn8MJyJurrlgJGNvWWE0hA.6i41DvT7RrQePHz6'
- First parameter is encoded password. There will be an error, if we don't encode with the byte.
- Second parameter generates salt.
- Salt adds random data to the actual password and calculates its hash value.
>>> salt=bcrypt.gensalt()
>>> salt
b'$2b$12$BAFxBp19.ct1NDBDKzrhO'
>>> hahsed_password = bcrypt.hashpw( password.encode('utf-8'), salt )
>>> hahsed_password
b'$2b$12$BAFxBp19.ct1NDBDKzrhOJ4tITcrsDDPS7NyM7i7WkUg.SZDUabq'
b'2b$12BAFxBp19, This value is the salt value in which hashed password was added. 이
>>> bcrypt.checkpw('1234'.encode('utf-8'), hashed_password )
True
>>> bcrypt.checkpw('123'.encode('utf-8'), hashed_password)
False
- check function checks if two passwords are identical. If yes, True, Otherwise, False.
pyjwt
- If user logs in, it will send the request that contains access tocken(has encoded user information). Then, the surver will receive the certain user's information by decoding that access token.
- There are several ways to generate access token. The most widely used way is JWT(Json Web Tokens).
- JWT encodes Json Data(that contains the user information) and lets the client and the server communicate.
- Frontend and Backend will use JWT to deliver encoded data.
pip install pyjwt
>>> import jwt
>>>
>>> encoded_jwt = jwt.encode( {'user-id' :5 }, 'secret', algorithm='HS256' )
>>> encoded_jwt
b'eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJ1c2VyLWlkIjo1fQ.tBQu0HfnOYK7lL3tH5ImgsI-y4Jz1RKscJWbV3U2QMI'
>>> type(encoded_jwt)
<class 'bytes'>
>>> jwt.decode( encoded_jwt, 'secret', algorithm='HS256' )
{'user-id': 5}
encoded_jwt = jwt.encode( {'user-id' :5 }, 'secret', algorithms=['HS256'] )
- Decoded jwt will always be a dictionary.
- Here, first parameter is the information for user_id (Here, it's 5)
- Second parameter is a secret key. If we upload on github, we can encode the data with this secret key and the third parameter (algorithm we're trying to encode with).
- This way, the information of user with the user_id of 5 will be encoded.
- REMARKS:
- In payload, you should not write user's personal information. User ID is relatively safe because it's just a number.
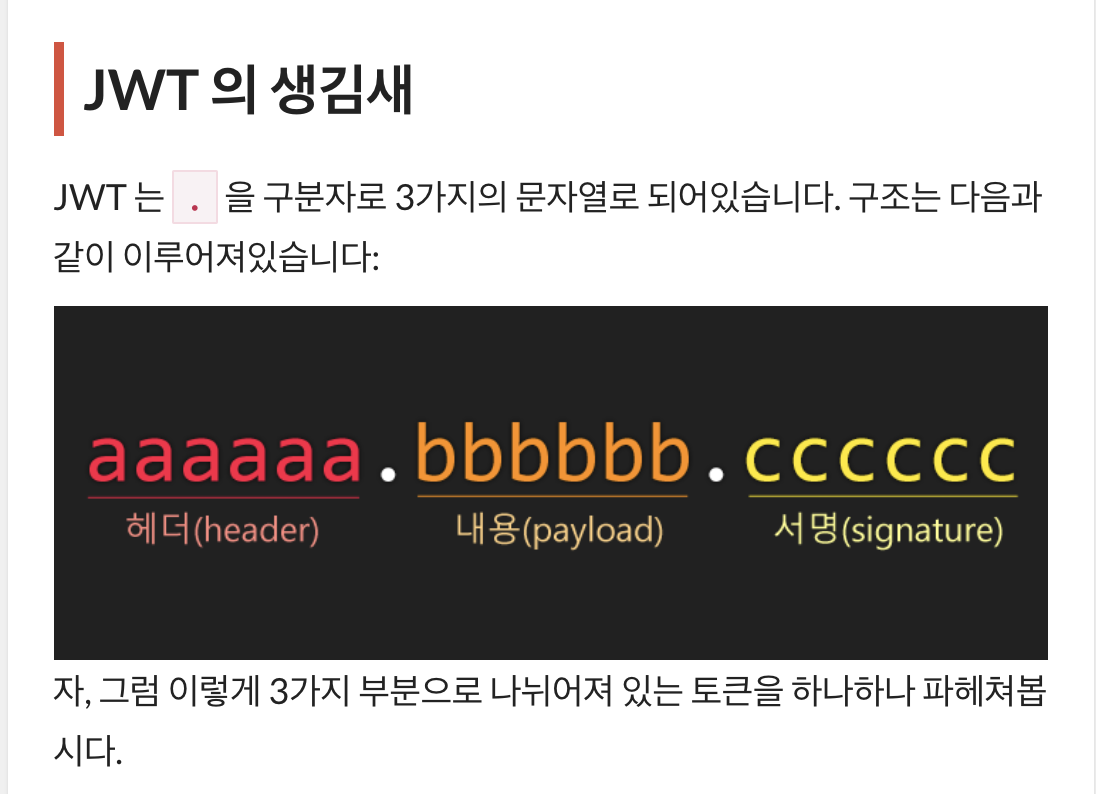
- Header contains two kinds of information.
- typ: assigns the type of token (JWT).
- alg: assigns the hashing algorith (HS256).
- Payload contains the information for token.