서버
app.py
from flask import Flask, render_template, request, jsonify
app = Flask(__name__)
from pymongo import MongoClient
client = MongoClient('mongodb+srv://test:sparta@cluster0.jmelm.mongodb.net/Cluster0?retryWrites=true&w=majority')
db = client.dbsparta
@app.route('/')
def home():
return render_template('index.html')
@app.route('/mypage')
def mypage():
return render_template('mypage.html')
@app.route('/record')
def record():
return render_template('record.html')
@app.route("/record", methods=["POST"])
def record_post():
Name_receive = request.form['Name_give']
Introduce_receive = request.form['Introduce_give']
Goal_receive = request.form['Goal_give']
Favorite_thing_receive = request.form['Favorite_thing_give']
Good_thing_receive = request.form['Good_thing_give']
First_values_receive = request.form['First_values_give']
World_needs_receive = request.form['World_needs_give']
doc = {
'Name': Name_receive,
'Introduce': Introduce_receive,
'Goal': Goal_receive,
'Favorite_thing': Favorite_thing_receive,
'Good_thing': Good_thing_receive,
'First_values': First_values_receive,
'World_needs': World_needs_receive
}
db.record.insert_one(doc)
return jsonify({'msg': '기록 완료!'})
@app.route("/", methods=["GET"])
def record_get():
record_list = list(db.record.find({}, {'_id': False}))
return jsonify({'records': record_list})
if __name__ == '__main__':
app.run('0.0.0.0', port=5000, debug=True)
HTML
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>홈페이지</title>
</head>
<body>
<a href="/mypage">my page</a>
<a href="/record">기록하기</a>
</body>
</html>
mypage.html
- 해야할 것
- 기록 페이지에서 기록한 거 마이 페이지에 붙이기
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js"
integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM"
crossorigin="anonymous"></script>
<title>마이 페이지</title>
<style>
@font-face {
font-family: 'ChosunGu';
src: url('https://cdn.jsdelivr.net/gh/projectnoonnu/noonfonts_20-04@1.0/ChosunGu.woff') format('woff');
font-weight: normal;
font-style: normal;
}
@font-face {
font-family: 'S-CoreDream-3Light';
src: url('https://cdn.jsdelivr.net/gh/projectnoonnu/noonfonts_six@1.2/S-CoreDream-3Light.woff') format('woff');
font-weight: normal;
font-style: normal;
}
@font-face {
font-family: 'GangwonEdu_OTFLightA';
src: url('https://cdn.jsdelivr.net/gh/projectnoonnu/noonfonts_2201-2@1.0/GangwonEdu_OTFLightA.woff') format('woff');
font-weight: normal;
font-style: normal;
}
@font-face {
font-family: 'S-CoreDream-5Medium';
src: url('https://cdn.jsdelivr.net/gh/projectnoonnu/noonfonts_six@1.2/S-CoreDream-5Medium.woff') format('woff');
font-weight: normal;
font-style: normal;
}
.box {
display: flex;
flex-direction: row;
justify-content: center;
align-items: flex-start;
padding: 20px;
font-family: 'S-CoreDream-3Light';
}
.profile {
margin: 10px 50px auto auto;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
th, td {
border-bottom: 1px solid #b7b7b7;
padding: 10px;
}
.personal {
font-family: 'ChosunGu';
margin: 10px auto auto 50px;
}
.personal > h1 {
font-family: 'GangwonEdu_OTFLightA';
text-align: center;
}
.goal {
display: flex;
flex-direction: row;
margin: 10px auto 10px auto;
}
.goal > .card {
width: 800px;
margin: 10px auto 10px auto;
}
.name {
font-family: 'S-CoreDream-5Medium';
margin: 10px;
padding: 3px;
width: 200px;
background-color: gray;
color: white;
text-align: center;
}
h5 {
color: #b7b7b7;
font-size: 15px;
}
</style>
</head>
<body>
<div class="box">
<div class="profile">
<img src="" width="200" height="200">
<p class="name">이름</p>
<a href="/record">기록하기</a>
</div>
<div class="personal">
<h1 class="title">"나를 표현하는 한 문장"</h1>
<div class="goal">
<div class="card">
<div class="card-body">
<h5 class="card-title">목표</h5>
<p class="goaltext">내용</p>
</div>
</div>
</div>
<div class="row row-cols-1 row-cols-md-2 g-4">
<div class="col">
<div class="card">
<div class="card-body">
<h5 class="card-title">좋아하는 것</h5>
<p class="favoritethingtext">내용</p>
</div>
</div>
</div>
<div class="col">
<div class="card">
<div class="card-body">
<h5 class="card-title">잘 하는 것</h5>
<p class="goodthingtext">내용</p>
</div>
</div>
</div>
<div class="col">
<div class="card">
<div class="card-body">
<h5 class="card-title">우선 가치관</h5>
<p class="firstvaluestext">내용</p>
</div>
</div>
</div>
<div class="col">
<div class="card">
<div class="card-body">
<h5 class="card-title">세상이 필요로 하는 것</h5>
<p class="worldneedstext">내용</p>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
record.html
- 해야할 것
- 이미지 파일 url db에 저장하기
- CSS - 깔끔하게 하기
<!DOCTYPE html>
<html lang="en">
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js"
integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM"
crossorigin="anonymous"></script>
<title>마이 페이지 - 기록하기</title>
<style>
th, td {
border-bottom: 1px solid #b7b7b7;
padding: 10px;
}
</style>
<script>
function readURL(input) {
if (input.files && input.files[0]) {
const reader = new FileReader();
reader.onload = function (e) {
document.getElementById('preview').src = e.target.result;
};
reader.readAsDataURL(input.files[0]);
} else {
document.getElementById('preview').src = "";
}
}
$(document).ready(function () {
recorded();
});
function recorded() {
$.ajax({
type: 'GET',
url: '/record',
data: {},
success: function (response) {
let rows = response['records']
for (let i = 0; i < rows.length; i++) {
let Name = rows[i]['Name']
let Introduce = rows[i]['Introduce']
let Goal = rows[i]['Goal']
let Favorite_thing = rows[i]['Favorite_thing']
let Good_thing = rows[i]['Good_thing']
let First_values = rows[i]['First_values']
let World_needs = rows[i]['World_needs']
console.log(Name,Introduce, Goal, Favorite_thing, Good_thing, First_values, World_needs)
}
}
});
}
function recording() {
let Name = $('#Name').val()
let Introduce = $('#Introduce').val()
let Goal = $('#Goal').val()
let Favorite_thing = $('#Favorite_thing').val()
let Good_thing = $('#Good_thing').val()
let First_values = $('#First_values').val()
let World_needs = $('#World_needs').val()
$.ajax({
type: 'POST',
url: '/record',
data: {
Name_give: Name,
Introduce_give: Introduce,
Goal_give: Goal,
Favorite_thing_give: Favorite_thing,
Good_thing_give: Good_thing,
First_values_give: First_values,
World_needs_give: World_needs
},
success: function (response) {
alert(response['msg'])
window.location.reload()
}
});
}
</script>
</head>
<body>
<a href="/mypage">my page</a>
<box id="recording-box" class="recording-box">
<table>
<tbody>
<tr class="tr1">
<td>
<label for="Name">이름</label>
</td>
<td>
<p><input type="text" id="Name" class="input-name" value=""/></p>
</td>
</tr>
<tr class="tr1">
<td>프로필 이미지</td>
<td>
<p><input type="file" class="input-image" onchange="readURL(this);"></p>
<p><img id="preview" width="200px" height="200px"/></p>
</td>
</tr>
<tr class="tr1">
<td>
<label for="Introduce">나를 표현하는 한 문장</label>
</td>
<td>
<p><textarea id="Introduce" class="input-introduce" value=""></textarea></p>
</td>
</tr>
<tr class="tr1">
<td>
<label for="Goal">목표</label>
</td>
<td>
<p><textarea id="Goal" class="input-goal" value=""></textarea></p>
</td>
</tr>
<tr class="tr1">
<td>
<label for="Favorite_thing">좋아하는 것</label>
</td>
<td>
<p><textarea id="Favorite_thing" class="input-favorite-thing" value=""></textarea></p>
</td>
</tr>
<tr class="tr1">
<td>
<label for="Good_thing">잘 하는 것</label>
</td>
<td>
<p><textarea id="Good_thing" class="input-good-thing" value=""></textarea></p>
</td>
</tr>
<tr class="tr1">
<td>
<label for="First_values">우선 가치관</label>
</td>
<td>
<p><textarea id="First_values" class="input-first-values" value=""></textarea></p>
</td>
</tr>
<tr class="tr1">
<td>
<label for="World_needs">세상이 필요로 하는 것</label>
</td>
<td>
<p><textarea id="World_needs" class="input-world-needs" value=""></textarea></p>
</td>
</tr>
</tbody>
</table>
<button onclick="recording()" type="button">기록하기</button>
</box>
</body>
</html>
페이지
홈페이지('/')

마이페이지('/mypage')
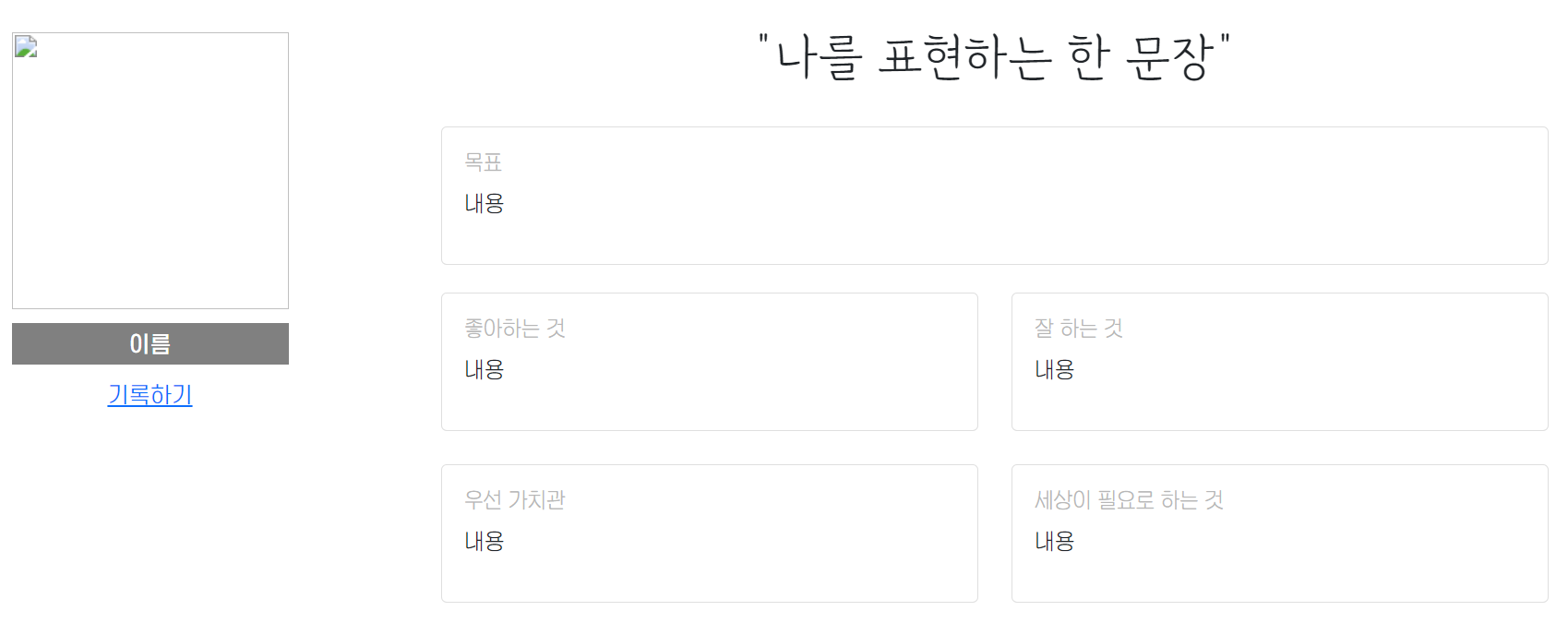
기록페이지('/record')
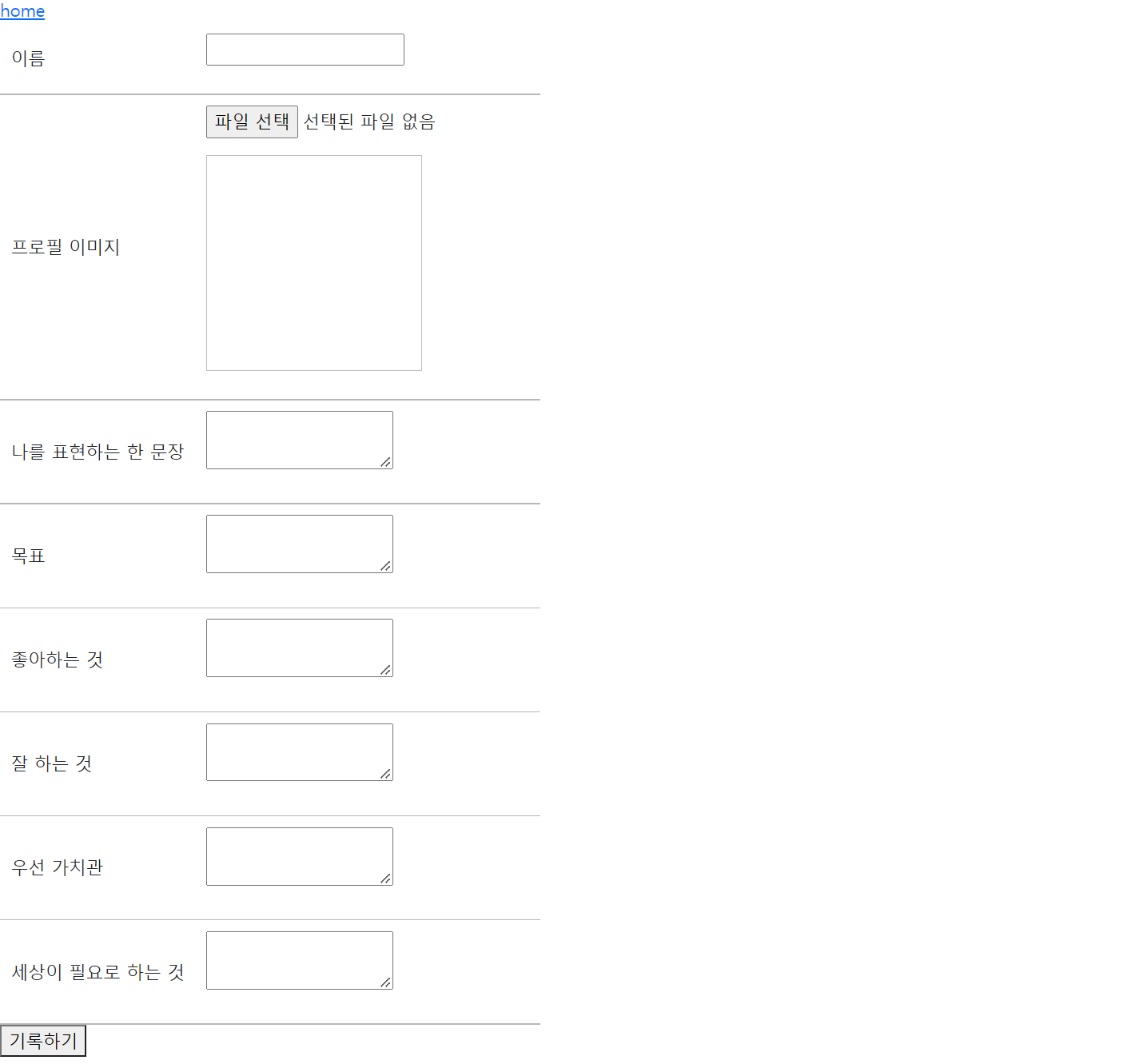
7주차 계획
- 프로필 이미지!
- GET 한 것 마이페이지에 내용 붙이기
- 기록페이지 CSS
- 홈페이지 만들기