app.js
import express from "express";
import helmet from "helmet";
import morgan from "morgan";
import cors from "cors";
import tweetController from "./tweet/tweet.controller.js";
const app = express();
app.use(express.json());
app.use(morgan("dev"));
app.use(helmet());
app.use(cors());
app.use("/tweet", tweetController);
app.use((req, res, next) => {
res.sendStatus(404);
});
app.use((err, req, res, next) => {
res.sendStatus(500);
});
app.listen(8000);
Controller
import express from "express";
import * as tweetService from "./tweet.service.js";
const tweetController = express.Router();
tweetController.get("/", tweetService.getTweets);
tweetController.get("/:id", tweetService.getTweet);
tweetController.post("/", tweetService.createTweet);
tweetController.put("/:id", tweetService.updateTweet);
tweetController.delete("/:id", tweetService.deleteTweet);
export default tweetController;
Service
import * as tweetRepository from "./tweet.repository.js";
export const getTweets = async (req, res) => {
const { username } = req.query;
const tempTweets = username
? await tweetRepository.getAllTweetsByUsername(username)
: await tweetRepository.getAllTweets();
res.status(200).json(tempTweets);
};
export const getTweet = async (req, res) => {
const { id } = req.params;
const tweet = await tweetRepository.getTweetById(id);
if (tweet) {
res.status(200).json(tweet);
} else {
res.status(404).json({ status: 404, message: `id ${id} NOT FOUND` });
}
};
export const createTweet = async (req, res) => {
await tweetRepository.createTweet(req.body);
res.sendStatus(201);
};
export const updateTweet = async (req, res) => {
const { id } = req.params;
const { text } = req.body;
const tweet = await tweetRepository.updateTweet(id, text);
if (tweet) {
tweet.text = text;
res.status(200).json(tweet);
} else {
res.status(404).json({ status: 404, message: `id ${id} NOT FOUND` });
}
};
export const deleteTweet = async (req, res) => {
const { id } = req.params;
const deleteResult = await tweetRepository.deleteTweet(id);
if (deleteResult) {
res.sendStatus(204);
} else {
res.status(404).json({ status: 404, message: `id ${id} NOT FOUND` });
}
};
Repository
let tweets = [
{
id: 1,
createdAt: new Date().toLocaleString(),
name: "SeokHyun Yu",
username: "ysh",
text: "Hello",
},
];
export const getAllTweets = async () => {
return tweets;
};
export const getAllTweetsByUsername = async (username) => {
return tweets.filter((tweet) => tweet.username === username);
};
export const getTweetById = async (id) => {
return tweets.find((tweet) => `${tweet.id}` === id);
};
export const createTweet = async ({ name, username, text }) => {
const newTweet = {
id: (tweets[0]?.id || 0) + 1,
createdAt: Date.now().toLocaleString(),
name,
username,
text,
};
tweets = [newTweet, ...tweets];
return tweets;
};
export const updateTweet = async (id, text) => {
const tweet = tweets.find((tweet) => `${tweet.id}` === id);
if (tweet) {
tweet.text = text;
}
return tweet;
};
export const deleteTweet = async (id) => {
const prevLength = tweets.length;
tweets = tweets.filter((tweet) => `${tweet.id}` !== id);
return prevLength !== tweets.length;
};
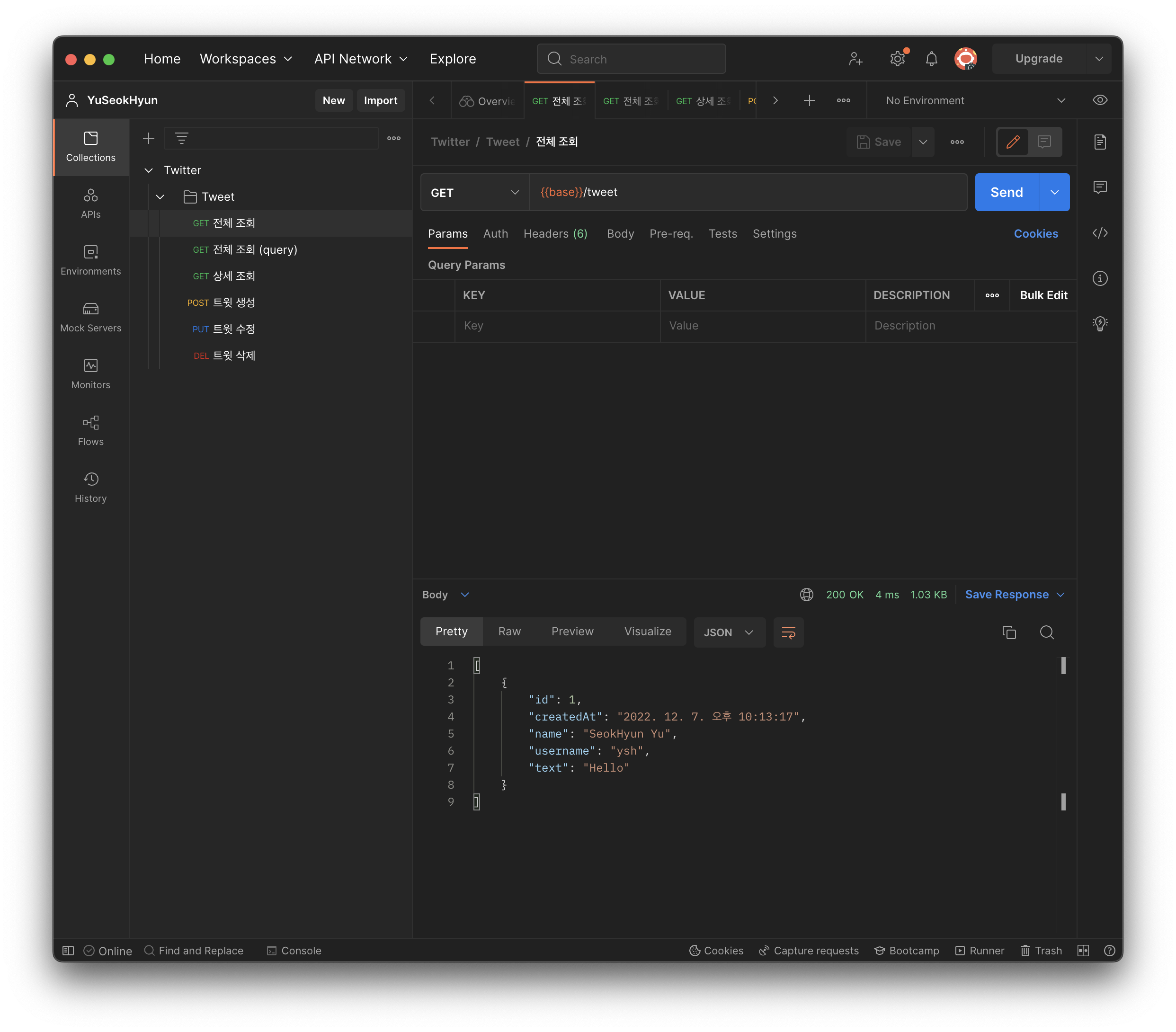
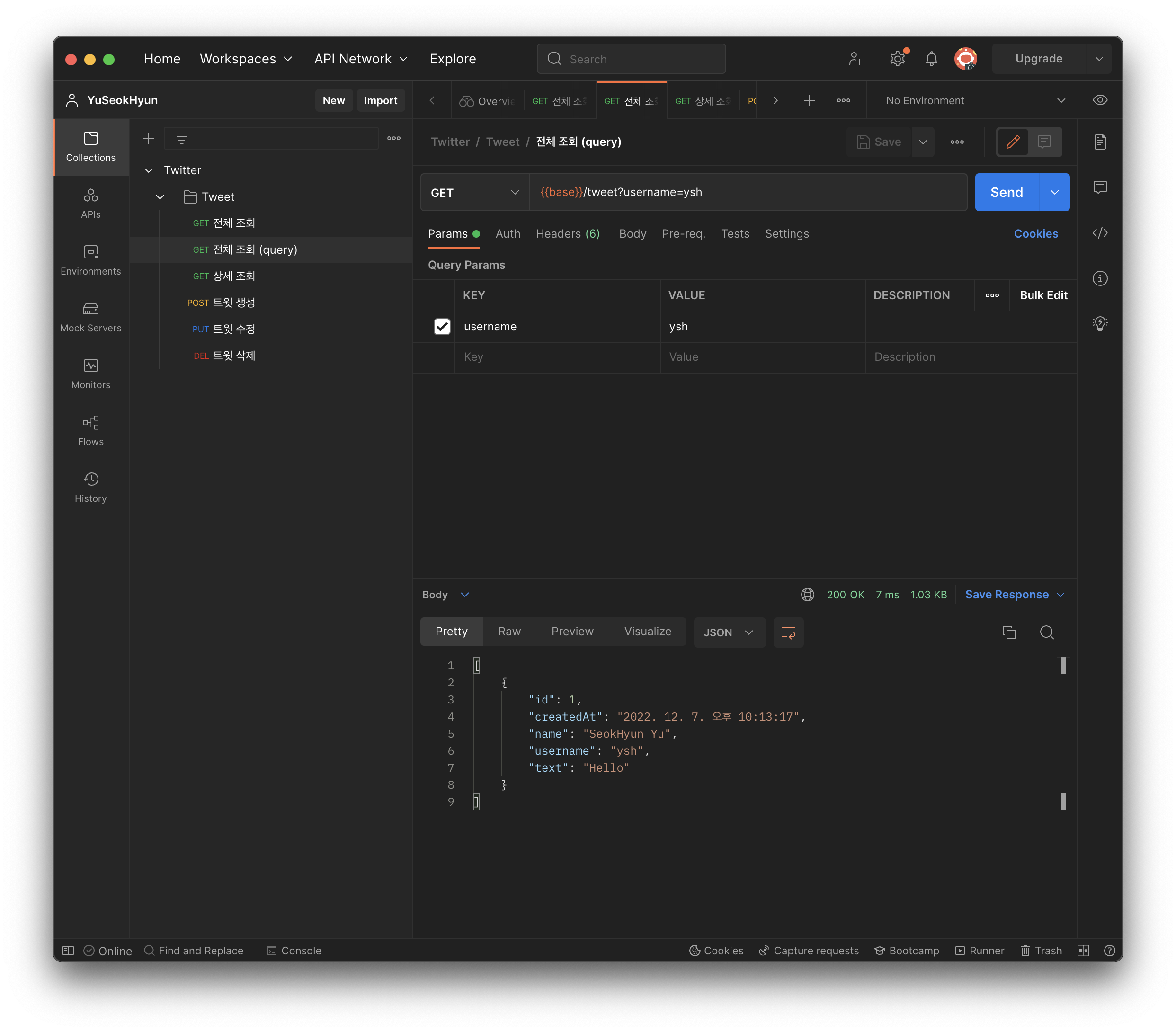
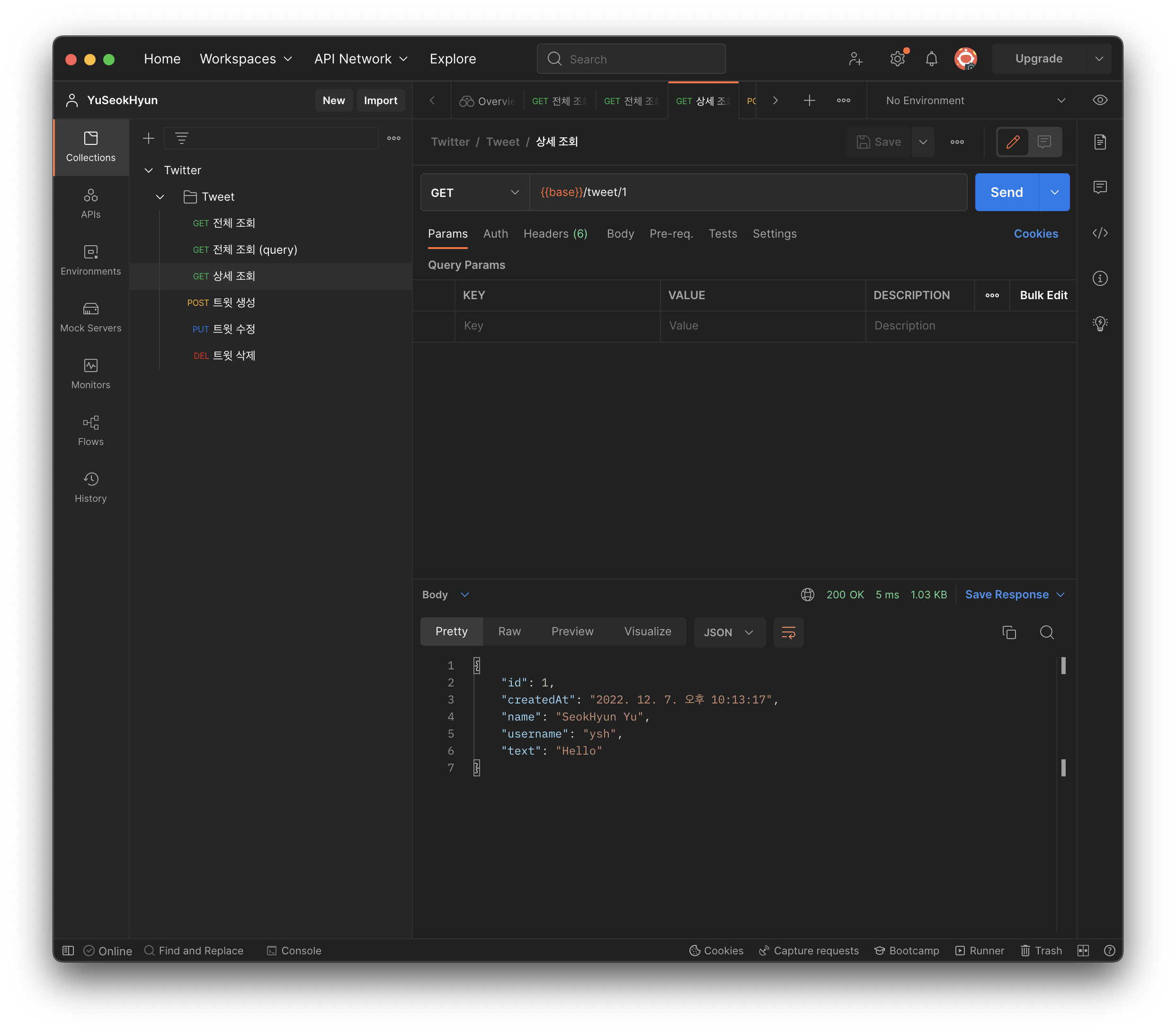
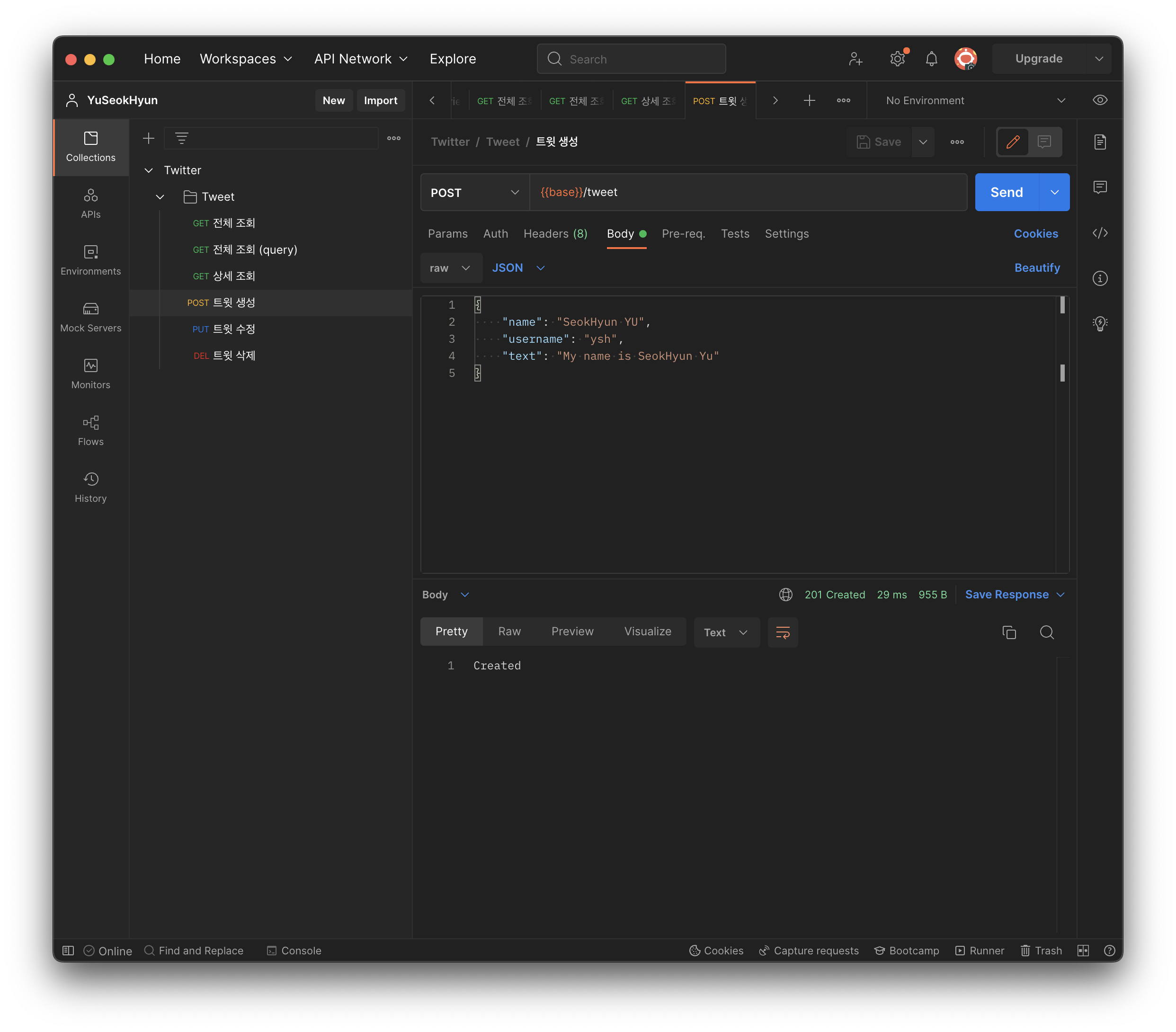
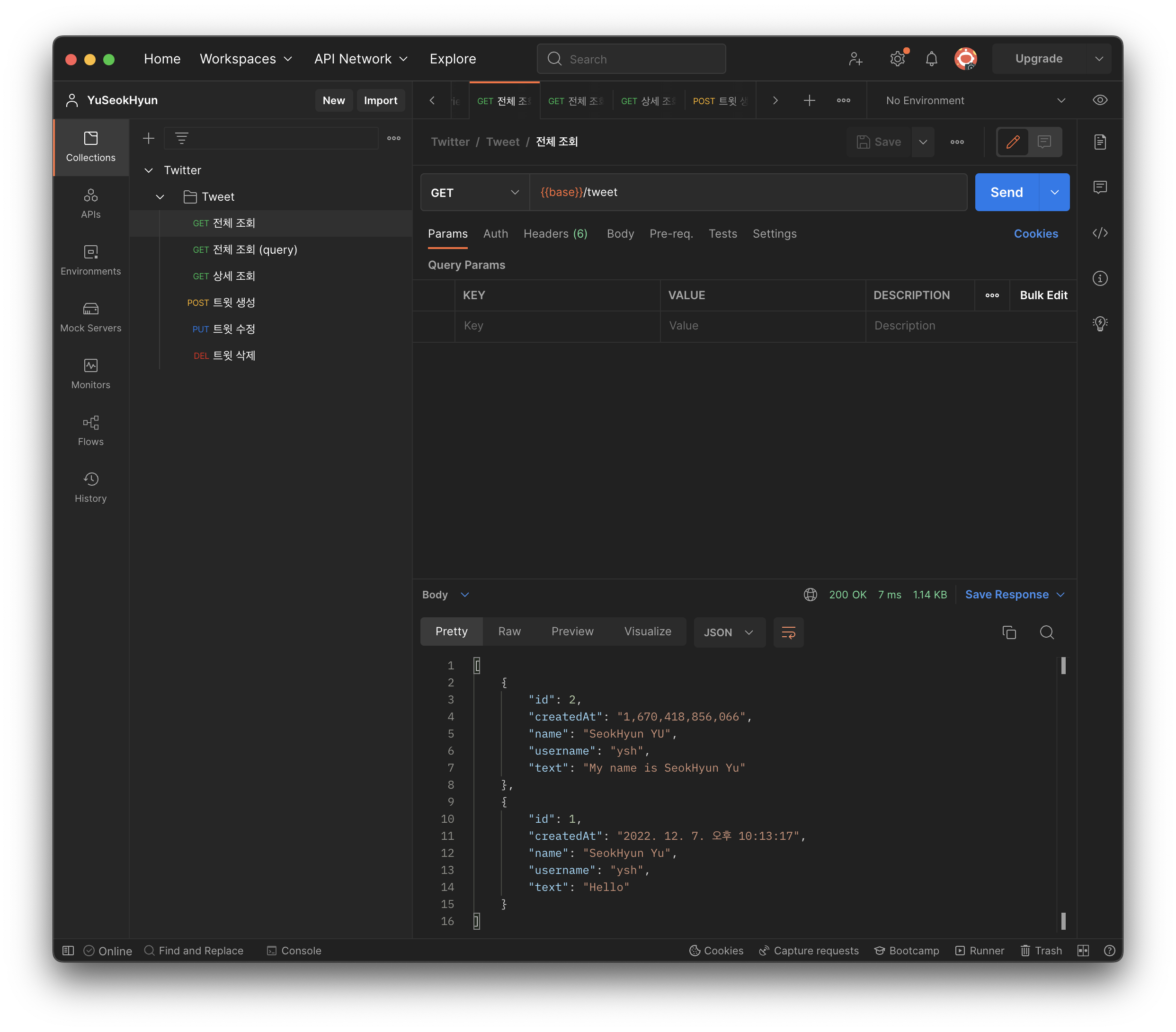
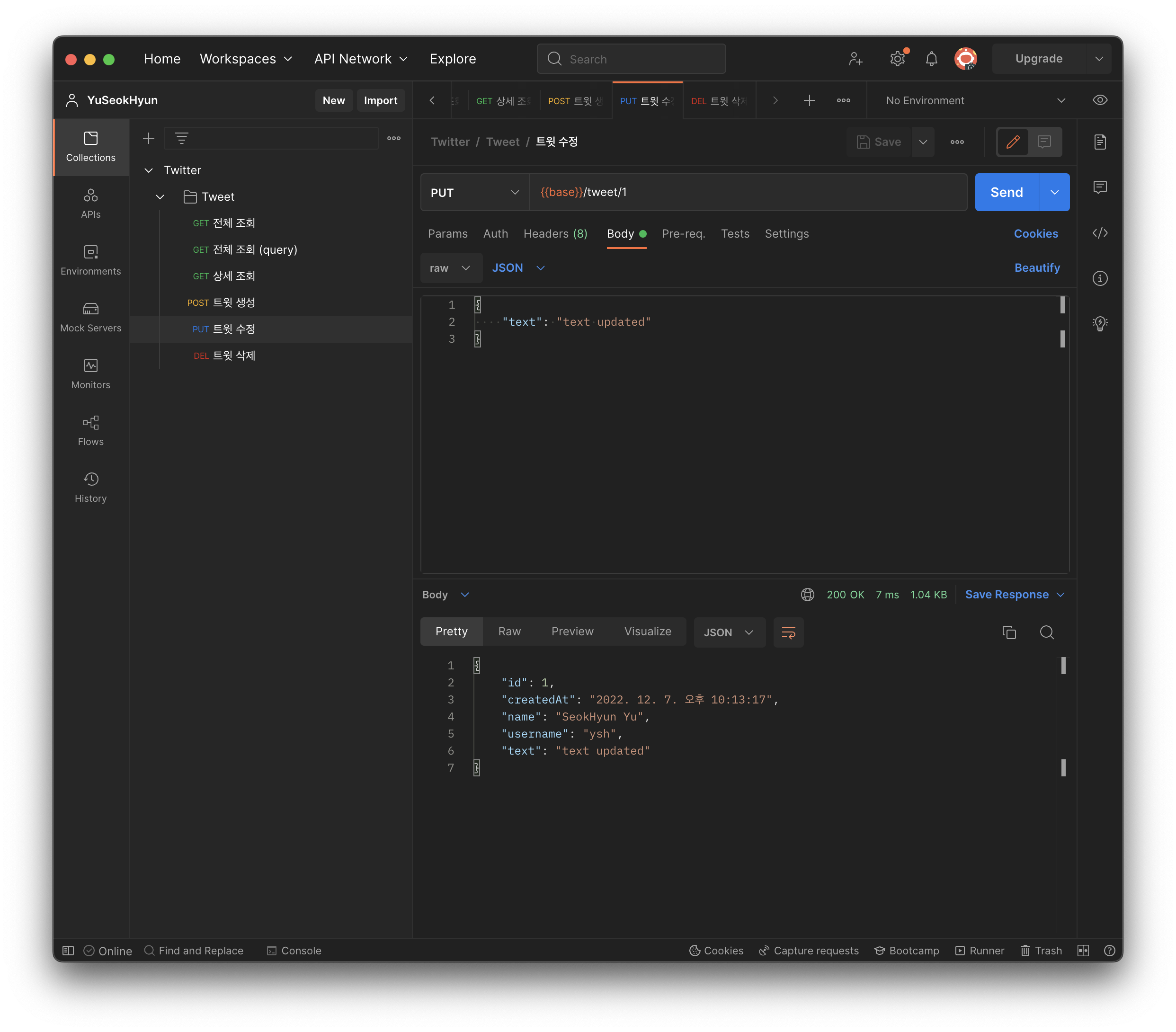
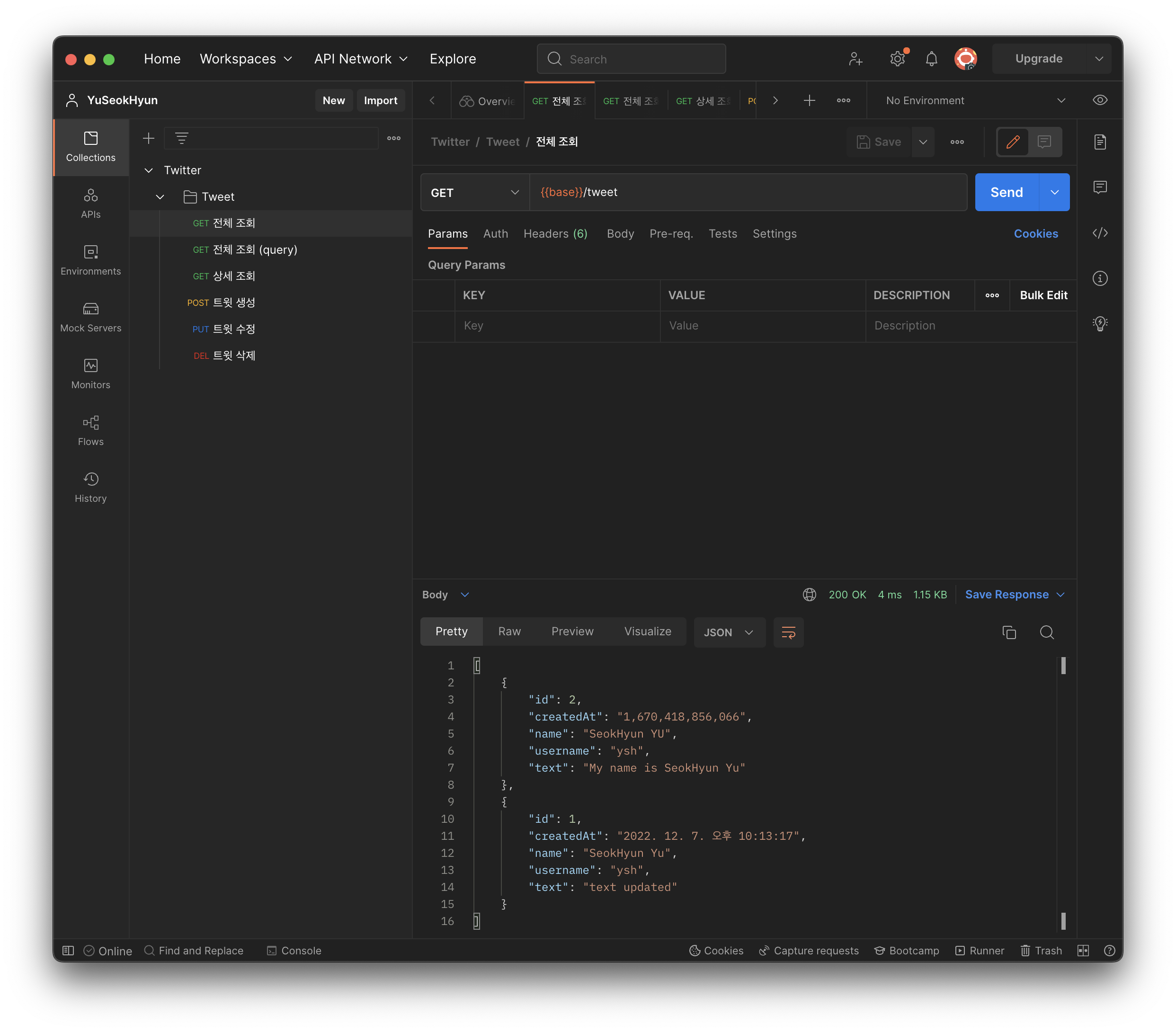
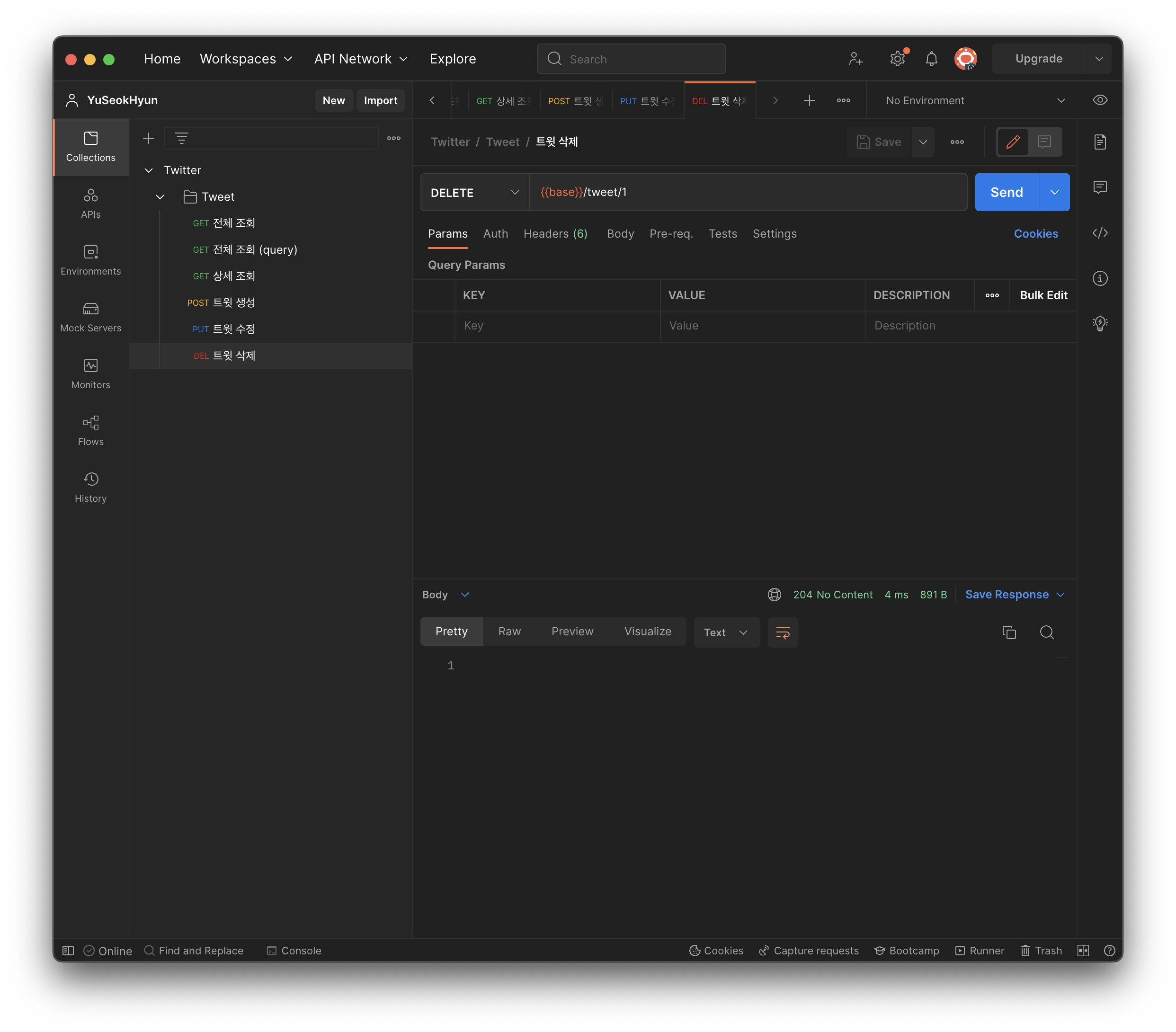
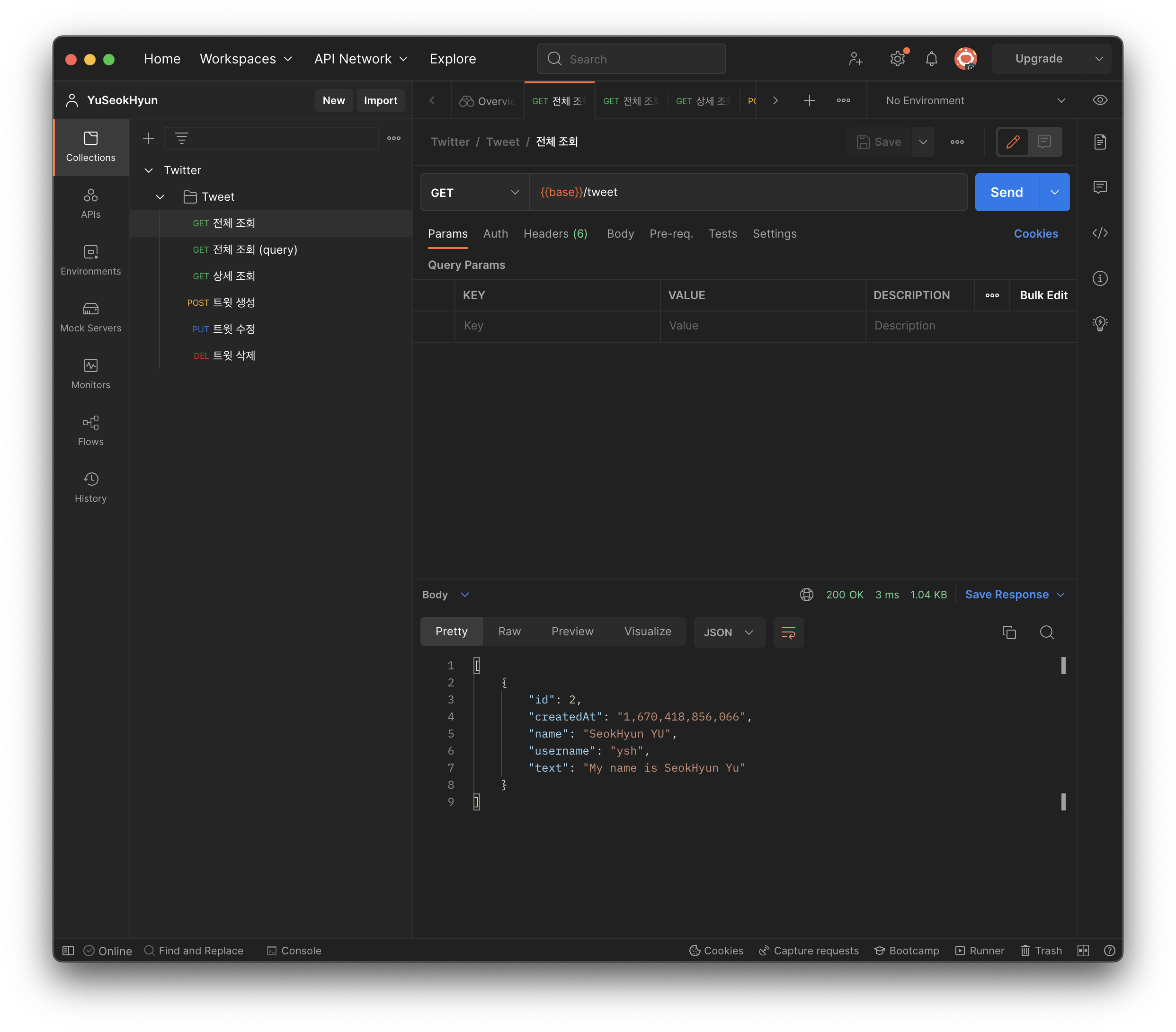